Python中实现图形旋转可以通过以下几种方式:使用Pygame库进行图形旋转、利用PIL库对图像进行旋转、借助Matplotlib库进行数据可视化图形的旋转。这些方法各有其适用场景,例如Pygame适合用于游戏开发中的实时图形旋转,而PIL适用于静态图像处理。下面将详细介绍如何通过这些方法实现图形的旋转。
一、PYGAME实现图形旋转
Pygame是一个用于开发2D游戏的Python库,其内置了许多处理图形和声音的功能。使用Pygame可以很方便地实现图形旋转。
-
Pygame安装与基本设置
首先需要安装Pygame库,可以通过pip进行安装:
pip install pygame
安装完成后,我们可以创建一个简单的窗口来显示图形。
import pygame
import sys
pygame.init()
screen = pygame.display.set_mode((800, 600))
pygame.display.set_caption('Pygame Rotation Example')
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
screen.fill((255, 255, 255))
pygame.display.flip()
-
加载和旋转图形
在Pygame中,可以使用
pygame.transform.rotate
方法来旋转图形。首先需要加载一张图形,然后应用旋转。import pygame
import sys
pygame.init()
screen = pygame.display.set_mode((800, 600))
pygame.display.set_caption('Pygame Rotation Example')
加载图像
image = pygame.image.load('path/to/image.png')
angle = 0
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
# 每帧旋转一定角度
angle += 1
rotated_image = pygame.transform.rotate(image, angle)
# 清空屏幕
screen.fill((255, 255, 255))
screen.blit(rotated_image, (400, 300))
pygame.display.flip()
-
调整旋转中心
默认情况下,图形会围绕其左上角进行旋转。为了实现围绕中心旋转,需要在旋转后调整图形的显示位置。
import pygame
import sys
pygame.init()
screen = pygame.display.set_mode((800, 600))
pygame.display.set_caption('Pygame Rotation Example')
image = pygame.image.load('path/to/image.png')
rect = image.get_rect()
angle = 0
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
angle += 1
rotated_image = pygame.transform.rotate(image, angle)
new_rect = rotated_image.get_rect(center=rect.center)
screen.fill((255, 255, 255))
screen.blit(rotated_image, new_rect.topleft)
pygame.display.flip()
二、PIL实现图像旋转
PIL(Python Imaging Library)是一个强大的图像处理库,可以用于处理静态图像。PIL中的rotate
方法可以用来旋转图像。
-
PIL安装与基本用法
首先安装PIL库(Pillow是PIL的一个分支):
pip install pillow
使用PIL可以很容易地加载和旋转图像。
from PIL import Image
加载图像
image = Image.open('path/to/image.jpg')
旋转图像
rotated_image = image.rotate(45)
保存旋转后的图像
rotated_image.save('path/to/rotated_image.jpg')
-
指定旋转中心
PIL的
rotate
方法默认会围绕图像的中心进行旋转。如果需要指定旋转中心,可以通过先扩大画布,然后进行旋转的方式实现。from PIL import Image
def rotate_image(image_path, angle, center):
image = Image.open(image_path)
# 扩大画布
expanded_image = Image.new('RGBA', (image.width * 2, image.height * 2))
expanded_image.paste(image, (image.width // 2, image.height // 2))
# 旋转图像
rotated_image = expanded_image.rotate(angle, center=center, expand=True)
return rotated_image
rotated_image = rotate_image('path/to/image.jpg', 45, (100, 100))
rotated_image.save('path/to/rotated_image.jpg')
三、MATPLOTLIB实现数据可视化图形旋转
Matplotlib是一个用于绘制数据可视化图形的Python库。在Matplotlib中,可以通过变换功能实现图形的旋转。
-
Matplotlib安装与基本绘图
首先安装Matplotlib库:
pip install matplotlib
使用Matplotlib绘制基本图形:
import matplotlib.pyplot as plt
import numpy as np
创建数据
x = np.linspace(0, 10, 100)
y = np.sin(x)
绘制图形
plt.plot(x, y)
plt.title('Original Plot')
plt.show()
-
旋转图形
在Matplotlib中,可以通过
Affine2D
变换实现图形旋转。import matplotlib.pyplot as plt
import numpy as np
from matplotlib.transforms import Affine2D
x = np.linspace(0, 10, 100)
y = np.sin(x)
fig, ax = plt.subplots()
创建旋转变换
trans = Affine2D().rotate_deg(45)
应用变换
ax.plot(x, y, transform=trans + ax.transData)
ax.set_xlim(-10, 10)
ax.set_ylim(-10, 10)
plt.title('Rotated Plot')
plt.show()
-
在特定点旋转
可以通过指定旋转的中心点来进行更灵活的图形旋转。
import matplotlib.pyplot as plt
import numpy as np
from matplotlib.transforms import Affine2D
x = np.linspace(0, 10, 100)
y = np.sin(x)
fig, ax = plt.subplots()
指定旋转中心
trans = Affine2D().rotate_deg_around(5, 0, 45)
ax.plot(x, y, transform=trans + ax.transData)
ax.set_xlim(-10, 10)
ax.set_ylim(-10, 10)
plt.title('Rotated Plot Around Point (5, 0)')
plt.show()
通过以上几种方法,Python用户可以在不同场景下实现图形的旋转操作,无论是游戏开发、图像处理还是数据可视化,都能找到适合的解决方案。选择合适的方法可以提高开发效率和代码可读性,帮助实现更复杂的图形处理需求。
相关问答FAQs:
如何在Python中实现图形旋转的基本步骤是什么?
在Python中实现图形旋转通常涉及使用图形处理库,如PIL(Pillow)或OpenCV。基本步骤包括:加载图像,创建一个旋转矩阵,应用该矩阵进行旋转,最后保存或展示旋转后的图像。具体实现可以参考相关库的文档和示例代码。
有哪些Python库可以用于图形旋转?
在Python中,有多个库可以实现图形旋转功能。Pillow是一个强大的图像处理库,支持多种图像格式并提供简单的旋转方法。OpenCV则更适合处理复杂的图像处理任务,同时支持高效的图像旋转。此外,Matplotlib也可以用于旋转图形,尤其是在绘制图形时。
旋转图形时,如何保持图形的质量?
在旋转图形时,保持图形质量的关键在于选择合适的旋转角度和插值方法。例如,使用双线性插值或立方插值可以在旋转过程中减少锯齿和模糊。此外,确保图像在进行旋转前为高分辨率,也能有效提高最终效果的质量。
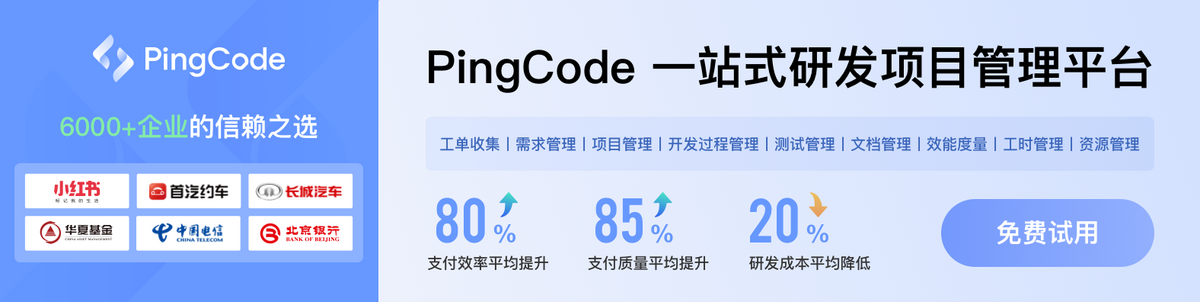