批量处理图像在Python中可以通过多种方法实现,通常使用Python的图像处理库如PIL(Pillow)、OpenCV、以及结合os库进行文件操作。这些库提供了图像读取、处理和保存的功能。PIL易于使用、支持多种图像格式,OpenCV则提供了更强大的计算机视觉功能。
在这些方法中,利用PIL库是最为常见的选择。PIL(Pillow)是一个功能强大的图像处理库,支持多种图像格式,易于使用。下面我们详细探讨如何使用Python进行批量图像处理。
一、PIL(Pillow)库的使用
Pillow是PIL的一个分支,提供了简单易用的接口来进行图像的打开、操作和保存。
- 安装和导入Pillow
在开始使用Pillow之前,需要确保已经安装了该库。可以通过以下命令安装:
pip install pillow
然后,在Python脚本中导入所需的模块:
from PIL import Image
import os
- 批量处理图像
首先,需要定义一个函数来处理单个图像。可以在该函数中执行如调整大小、转换格式、旋转等操作。
def process_image(input_path, output_path):
with Image.open(input_path) as img:
# 示例操作:调整大小
img = img.resize((800, 600))
# 示例操作:转换为灰度
img = img.convert('L')
# 保存处理后的图像
img.save(output_path)
然后,编写一个循环来遍历目录中的所有图像文件,并对每个文件调用上述函数。
def batch_process_images(input_dir, output_dir):
if not os.path.exists(output_dir):
os.makedirs(output_dir)
for filename in os.listdir(input_dir):
if filename.endswith(('.png', '.jpg', '.jpeg', '.bmp', '.gif')):
input_path = os.path.join(input_dir, filename)
output_path = os.path.join(output_dir, filename)
process_image(input_path, output_path)
- 示例调用
定义输入和输出目录,然后调用批量处理函数:
input_directory = 'path/to/input/directory'
output_directory = 'path/to/output/directory'
batch_process_images(input_directory, output_directory)
二、OpenCV库的使用
OpenCV是一个强大的计算机视觉库,支持丰富的图像处理功能。
- 安装和导入OpenCV
使用以下命令安装OpenCV:
pip install opencv-python
然后,在Python脚本中导入OpenCV:
import cv2
import os
- 批量处理图像
与Pillow类似,首先定义一个函数来处理单个图像:
def process_image_opencv(input_path, output_path):
img = cv2.imread(input_path)
# 示例操作:调整大小
img = cv2.resize(img, (800, 600))
# 示例操作:转换为灰度
img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 保存处理后的图像
cv2.imwrite(output_path, img)
然后,遍历目录并处理每个图像:
def batch_process_images_opencv(input_dir, output_dir):
if not os.path.exists(output_dir):
os.makedirs(output_dir)
for filename in os.listdir(input_dir):
if filename.endswith(('.png', '.jpg', '.jpeg', '.bmp', '.gif')):
input_path = os.path.join(input_dir, filename)
output_path = os.path.join(output_dir, filename)
process_image_opencv(input_path, output_path)
- 示例调用
input_directory = 'path/to/input/directory'
output_directory = 'path/to/output/directory'
batch_process_images_opencv(input_directory, output_directory)
三、结合其他库进行高级处理
在实际应用中,可能需要对图像执行更复杂的处理操作,例如使用NumPy进行数学计算,或使用scikit-image进行图像变换。
- 使用NumPy进行高级处理
NumPy是Python的一个强大的数学计算库,通常与OpenCV结合使用。
import numpy as np
def advanced_process_image(input_path, output_path):
img = cv2.imread(input_path)
# 示例操作:均值滤波
img = cv2.blur(img, (5, 5))
# 使用NumPy进行自定义操作
img = np.where(img > 128, 255, 0)
cv2.imwrite(output_path, img)
- 使用scikit-image进行图像变换
scikit-image是一个用于图像处理的Python库,特别适合科学计算和图像分析。
from skimage import io, filters
def process_image_skimage(input_path, output_path):
img = io.imread(input_path)
# 示例操作:应用Sobel边缘检测
edges = filters.sobel(img)
io.imsave(output_path, edges)
四、优化批量处理的性能
当处理大量图像时,性能可能成为一个问题。可以通过以下方法进行优化:
- 多线程与多进程
使用Python的多线程或多进程模块来并行处理图像,从而提高处理速度。
from multiprocessing import Pool
def batch_process_images_concurrently(input_dir, output_dir, num_workers=4):
if not os.path.exists(output_dir):
os.makedirs(output_dir)
files = [f for f in os.listdir(input_dir) if f.endswith(('.png', '.jpg', '.jpeg', '.bmp', '.gif'))]
pool = Pool(num_workers)
for filename in files:
input_path = os.path.join(input_dir, filename)
output_path = os.path.join(output_dir, filename)
pool.apply_async(process_image_opencv, args=(input_path, output_path))
pool.close()
pool.join()
- 批量读取和写入
减少I/O操作的次数可以显著提高性能。例如,可以使用内存映射文件来加速读取和写入。
五、处理不同格式的图像
在批量处理图像时,可能需要处理多种不同格式的图像。Pillow和OpenCV都支持多种格式,但处理方式略有不同。
- 读取和写入不同格式
确保在读取和保存图像时指定正确的格式,特别是在处理透明度(Alpha通道)时。
def process_image_with_alpha(input_path, output_path):
with Image.open(input_path) as img:
# 检查并处理Alpha通道
if img.mode in ('RGBA', 'LA') or (img.mode == 'P' and 'transparency' in img.info):
img = img.convert('RGBA')
img = img.resize((800, 600))
img.save(output_path, format='PNG')
- 处理不同色彩空间
在处理不同格式的图像时,可能需要转换色彩空间。例如,从RGB转换到YUV或灰度。
def convert_color_space(input_path, output_path):
img = cv2.imread(input_path)
# 转换为YUV色彩空间
yuv_img = cv2.cvtColor(img, cv2.COLOR_BGR2YUV)
cv2.imwrite(output_path, yuv_img)
总结:
批量处理图像是一个常见的需求,Python提供了多种库和工具来实现这一功能。Pillow和OpenCV是两种常用的图像处理库,各有优劣。通过结合使用这些库,并利用多线程、多进程等技术,可以有效地提高图像处理的效率。根据具体的需求,可以选择适合的库和方法来实现批量图像处理。
相关问答FAQs:
如何使用Python处理大量图像?
在Python中,您可以利用多个库来批量处理图像,常用的库包括PIL(Pillow)、OpenCV和ImageMagick。通过编写脚本,您可以轻松地读取、修改和保存图像。您可以使用循环遍历指定文件夹中的所有图像文件,并对其进行所需的处理,例如调整大小、格式转换或应用滤镜。
有哪些Python库适合图像批量处理?
有几个流行的Python库非常适合进行图像批量处理。Pillow是一个强大的图像处理库,支持多种图像格式,适合常规图像操作。OpenCV则更适合于复杂的图像处理需求,尤其是在计算机视觉方面。其他库如imageio和scikit-image也提供了丰富的图像处理功能,可以根据需求选择适合的库。
如何提高图像批量处理的效率?
在进行图像批量处理时,您可以采用多线程或多进程的方法来提高效率。使用Python的concurrent.futures模块,您可以并行处理多个图像。此外,合理选择图像的处理方式和保存格式也能显著提高处理速度。比如,选择合适的压缩算法和调整图像的分辨率,可以在保持图像质量的同时减少处理时间。
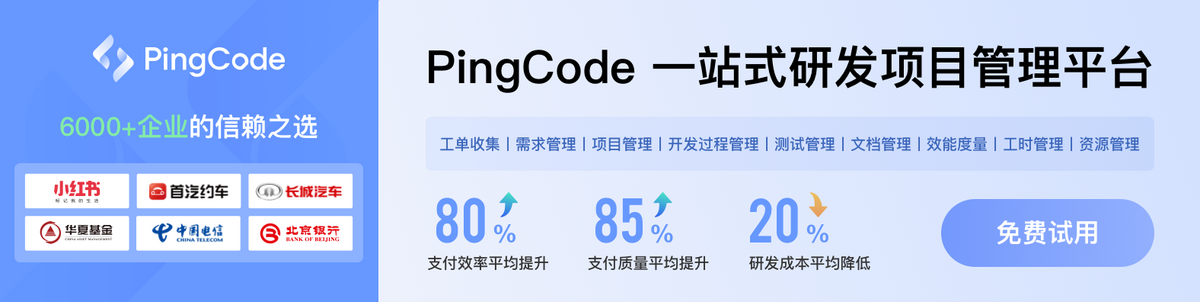