使用Python的format方法可以实现字符串的格式化输出,通过占位符、位置参数、关键字参数等方式,可以灵活地控制输出格式、精确控制小数点、调整字符串对齐方式等。 其中,占位符是最基本的格式化方式,通过在字符串中指定大括号{}
来表示需要替换的内容;位置参数则允许根据顺序或索引来替换占位符;而关键字参数则可以通过指定变量名来替换占位符。例如,你可以使用"{0} is {1}".format("Python", "awesome")
这种形式来格式化输出。下面将详细介绍Python中format的使用方法。
一、占位符与基本用法
使用Python的format
方法,最基本的形式是通过占位符来指定要替换的内容。占位符使用大括号{}
表示,可以在字符串中放置任意位置。
-
简单替换
在最简单的形式下,
format
方法会按照传入参数的顺序来替换占位符。例如:message = "Hello, {}. Welcome to {}!"
formatted_message = message.format("Alice", "Wonderland")
print(formatted_message) # 输出: Hello, Alice. Welcome to Wonderland!
-
索引位置
可以在占位符中指定位置索引,以确定使用哪个参数来替换该占位符:
message = "The {0} is stronger than the {1}."
formatted_message = message.format("pen", "sword")
print(formatted_message) # 输出: The pen is stronger than the sword.
索引位置可以重复使用:
message = "The {0} is mightier than the {1}, and the {0} writes history."
formatted_message = message.format("pen", "sword")
print(formatted_message) # 输出: The pen is mightier than the sword, and the pen writes history.
二、关键字参数
除了使用位置参数外,format
方法还可以使用关键字参数,这样可以使代码更加清晰和易读。通过在占位符中指定变量名来替换内容。
-
基本用法
使用关键字参数可以直接在占位符中指定参数名:
message = "Hello, {name}. Welcome to {place}!"
formatted_message = message.format(name="Alice", place="Wonderland")
print(formatted_message) # 输出: Hello, Alice. Welcome to Wonderland!
-
混合使用
位置参数和关键字参数可以混合使用,但位置参数必须在关键字参数之前:
message = "Hello, {}. Welcome to {place}!"
formatted_message = message.format("Alice", place="Wonderland")
print(formatted_message) # 输出: Hello, Alice. Welcome to Wonderland!
三、格式化字符串的高级用法
format
方法提供了强大的格式化功能,可以用于控制输出的对齐、宽度、精度等。
-
对齐方式
使用冒号
:
后接对齐方式符号可以控制字符串的对齐。常用的对齐方式包括:<
:左对齐>
:右对齐^
:居中对齐
message = "{:<10} is left-aligned.".format("text")
print(message) # 输出: text is left-aligned.
message = "{:>10} is right-aligned.".format("text")
print(message) # 输出: text is right-aligned.
message = "{:^10} is centered.".format("text")
print(message) # 输出: text is centered.
-
设置宽度
可以在对齐方式符号后指定一个数字,表示输出的最小宽度:
message = "{:10} is the width.".format("text")
print(message) # 输出: text is the width.
-
控制数值精度
对于浮点数,可以通过
format
方法控制小数点后的位数:value = 3.14159
message = "Pi is approximately {:.2f}".format(value)
print(message) # 输出: Pi is approximately 3.14
-
使用千位分隔符
通过在格式化字符串中使用逗号可以实现千位分隔符:
value = 1234567890
message = "The number is {:,}".format(value)
print(message) # 输出: The number is 1,234,567,890
四、嵌套与复合格式化
在某些情况下,你可能需要在格式化字符串中嵌套使用format
方法,这种方法可以用于更复杂的字符串构建。
-
嵌套使用
nested_message = "Coordinates: {latitude}, {longitude}".format(
latitude="{:.2f}".format(37.7749),
longitude="{:.2f}".format(-122.4194)
)
print(nested_message) # 输出: Coordinates: 37.77, -122.42
-
复合格式化
复合格式化允许在一个占位符中同时使用多种格式化功能:
message = "The value is {value:,.2f}".format(value=12345.6789)
print(message) # 输出: The value is 12,345.68
五、使用字典进行格式化
在某些情况下,数据可能以字典形式存储,format
方法允许直接使用字典进行格式化。
-
字典解包
可以通过在
format
方法中使用双星号来解包字典:
data = {"name": "Alice", "place": "Wonderland"}
message = "Hello, {name}. Welcome to {place}!".format(data)
print(message) # 输出: Hello, Alice. Welcome to Wonderland!
-
结合关键字参数
字典解包可以与关键字参数结合使用,以提供更灵活的格式化选项:
data = {"place": "Wonderland"}
message = "Hello, {name}. Welcome to {place}!".format(name="Alice", data)
print(message) # 输出: Hello, Alice. Welcome to Wonderland!
六、使用类与对象进行格式化
format
方法不仅可以用于基本数据类型,还可以用于类和对象。通过在类中定义__format__
方法,可以自定义对象的格式化行为。
-
定义__format__方法
自定义对象的格式化行为需要在类中定义
__format__
方法:class Point:
def __init__(self, x, y):
self.x = x
self.y = y
def __format__(self, format_spec):
if format_spec == "r":
return "({:.2f}, {:.2f})".format(self.x, self.y)
else:
return "Point({}, {})".format(self.x, self.y)
point = Point(1.23456, 7.89012)
print("Formatted: {:r}".format(point)) # 输出: Formatted: (1.23, 7.89)
-
灵活使用对象格式化
通过在类中实现
__format__
方法,可以实现更灵活的对象格式化:class Car:
def __init__(self, make, model):
self.make = make
self.model = model
def __format__(self, format_spec):
if format_spec == "short":
return "{} {}".format(self.make, self.model)
else:
return "Car(make='{}', model='{}')".format(self.make, self.model)
car = Car("Toyota", "Corolla")
print("Formatted: {:short}".format(car)) # 输出: Formatted: Toyota Corolla
七、总结
Python的format
方法提供了一种强大而灵活的字符串格式化方式。通过占位符、位置参数、关键字参数以及对齐、宽度、精度控制等高级功能,用户可以实现多种多样的字符串格式化需求。此外,通过结合使用字典、类和对象,format
方法更是为复杂数据结构的格式化提供了有力支持。在Python的实际编程中,熟练掌握format
方法的使用将极大提高代码的可读性和可维护性。
相关问答FAQs:
如何在Python中使用format方法格式化字符串?
在Python中,使用format
方法可以轻松地格式化字符串。你可以通过在字符串中使用大括号 {}
来指定要插入的位置,并调用字符串的format()
方法来填充这些位置。例如,"Hello, {}".format("world")
将输出 Hello, world
。此外,可以使用索引、关键字参数或格式说明符来进一步控制输出的格式。
format方法与f-strings有什么区别?format
方法与f-strings(格式化字符串字面量)之间的主要区别在于语法和可读性。f-strings在Python 3.6及以上版本中引入,允许你在字符串前加上字母f
,并直接在字符串中嵌入表达式,比如 name = "Alice"
,可以写成 f"Hello, {name}"
。f-strings通常更简洁且易于阅读,但format
方法在处理复杂的格式时更为灵活。
如何使用format方法进行数字格式化?format
方法允许对数字进行多种格式化操作,例如控制小数位数、添加千位分隔符等。你可以在大括号中添加格式说明符,例如 "{:.2f}".format(3.14159)
,这将输出 3.14
,表示保留两位小数。对于千位分隔符,可以使用 "{:,}".format(1000000)
,输出结果为 1,000,000
。这种灵活性使得format
方法在处理财务数据或统计信息时非常实用。
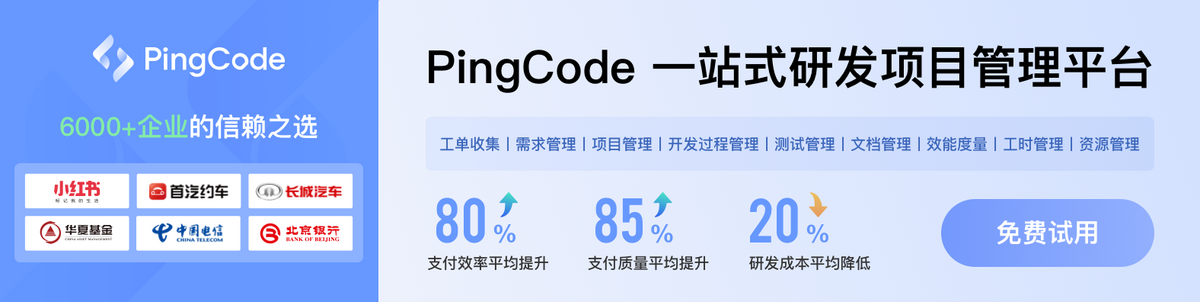