使用Python批量下载文件的过程包括:选择合适的库、设置下载链接、处理并发下载、错误处理、保存文件。这些步骤确保你可以高效地进行批量下载。 其中,选择合适的库非常关键,因为不同的库提供不同的功能,可以满足不同的下载需求。比如,requests
库适合简单的HTTP请求,而aiohttp
库则适合需要高并发的下载任务。
选择合适的库时,首先需要明确下载任务的性质。如果你的任务是简单的HTTP下载,requests
库是一个很好的选择。它易于使用,适合初学者。而如果你的任务涉及大量的并发下载,aiohttp
或者httpx
库则更为适合,它们支持异步操作,可以显著提高下载效率。在使用这些库时,还需要注意目标网站的反爬虫机制,合理设置请求头和间隔时间,以避免被封禁。
接下来,我们将详细介绍如何使用Python进行批量下载。
一、选择合适的库
在Python中,有多种库可以用于批量下载。选择合适的库是成功完成任务的第一步。
1. Requests库
requests
是Python中最流行的HTTP库之一,非常适合初学者使用。它提供了简洁的API,可以轻松地发送HTTP请求和下载文件。
import requests
def download_file(url, filename):
response = requests.get(url)
with open(filename, 'wb') as file:
file.write(response.content)
download_file('http://example.com/file.txt', 'file.txt')
2. Aiohttp库
对于需要处理大量并发请求的任务,aiohttp
库是一个不错的选择。它支持异步HTTP请求,可以显著提高下载速度。
import aiohttp
import asyncio
async def download_file(session, url, filename):
async with session.get(url) as response:
with open(filename, 'wb') as file:
file.write(await response.read())
async def main(urls):
async with aiohttp.ClientSession() as session:
tasks = [download_file(session, url, f'file_{i}.txt') for i, url in enumerate(urls)]
await asyncio.gather(*tasks)
urls = ['http://example.com/file1.txt', 'http://example.com/file2.txt']
asyncio.run(main(urls))
二、设置下载链接
在批量下载时,需要提前准备好所有的下载链接。这些链接可以存储在列表、文件或数据库中。
1. 从列表中获取链接
如果你的下载链接较少,可以直接将它们存储在一个列表中。
urls = ['http://example.com/file1.txt', 'http://example.com/file2.txt']
2. 从文件中读取链接
如果链接数量较多,可以将它们存储在一个文本文件中,然后逐行读取。
def load_urls_from_file(filename):
with open(filename, 'r') as file:
return [line.strip() for line in file]
urls = load_urls_from_file('urls.txt')
三、处理并发下载
并发下载可以显著提高下载效率,特别是在处理大量文件时。
1. 使用线程池
对于不支持异步操作的库,可以使用concurrent.futures.ThreadPoolExecutor
来实现并发下载。
from concurrent.futures import ThreadPoolExecutor
import requests
def download_file(url):
response = requests.get(url)
filename = url.split('/')[-1]
with open(filename, 'wb') as file:
file.write(response.content)
urls = ['http://example.com/file1.txt', 'http://example.com/file2.txt']
with ThreadPoolExecutor() as executor:
executor.map(download_file, urls)
2. 使用异步库
对于支持异步操作的库,如aiohttp
,可以直接利用它的异步特性来处理并发下载。
# 示例代码同上aiohttp部分
四、错误处理
在批量下载过程中,可能会遇到网络错误、文件不存在等问题。合理的错误处理可以提高程序的健壮性。
1. 捕获异常
在下载文件时,使用try-except
块捕获异常,并记录错误信息。
import requests
def download_file(url):
try:
response = requests.get(url)
response.raise_for_status() # 检查请求是否成功
filename = url.split('/')[-1]
with open(filename, 'wb') as file:
file.write(response.content)
except requests.RequestException as e:
print(f"Error downloading {url}: {e}")
urls = ['http://example.com/file1.txt', 'http://example.com/file2.txt']
for url in urls:
download_file(url)
2. 重试机制
对于临时的网络错误,可以实现一个简单的重试机制。
import time
import requests
def download_file(url, retries=3):
for i in range(retries):
try:
response = requests.get(url)
response.raise_for_status()
filename = url.split('/')[-1]
with open(filename, 'wb') as file:
file.write(response.content)
return
except requests.RequestException as e:
print(f"Attempt {i+1} failed for {url}: {e}")
time.sleep(2) # 等待2秒后重试
urls = ['http://example.com/file1.txt', 'http://example.com/file2.txt']
for url in urls:
download_file(url)
五、保存文件
在下载完成后,需要将文件保存到指定的位置。可以根据需求选择保存路径和文件名。
1. 动态生成文件名
可以根据URL动态生成文件名,或者根据时间戳、序号等生成唯一的文件名。
import os
from datetime import datetime
def download_file(url):
response = requests.get(url)
filename = url.split('/')[-1]
timestamp = datetime.now().strftime('%Y%m%d%H%M%S')
unique_filename = f"{timestamp}_{filename}"
with open(unique_filename, 'wb') as file:
file.write(response.content)
urls = ['http://example.com/file1.txt', 'http://example.com/file2.txt']
for url in urls:
download_file(url)
2. 指定保存路径
可以指定一个目录来保存所有下载的文件,确保目录存在,如果不存在则创建它。
import os
def download_file(url, save_dir='downloads'):
if not os.path.exists(save_dir):
os.makedirs(save_dir)
response = requests.get(url)
filename = os.path.join(save_dir, url.split('/')[-1])
with open(filename, 'wb') as file:
file.write(response.content)
urls = ['http://example.com/file1.txt', 'http://example.com/file2.txt']
for url in urls:
download_file(url)
通过以上步骤,你可以使用Python高效地进行批量下载。选择合适的库和方法,处理好并发和错误,可以让你的下载任务更加顺利。
相关问答FAQs:
如何使用Python批量下载文件?
使用Python批量下载文件通常可以通过requests库结合多线程或异步编程实现。首先,安装requests库并确保你有文件的URL列表。接着,可以编写一个简单的脚本,遍历URL列表,使用requests.get()方法下载文件。为了提高效率,可以考虑使用ThreadPoolExecutor或asyncio库来并发下载。
使用哪些Python库可以简化批量下载的过程?
除了requests库,Beautiful Soup和Pandas也可以帮助简化批量下载过程。Beautiful Soup用于从网页中提取文件的链接,而Pandas可以处理数据,方便批量下载多个文件的URL。通过结合这些库,可以构建一个更为高效的下载流程。
在下载过程中如何处理错误和异常?
在下载文件时,处理错误和异常是非常重要的。可以使用try-except语句捕捉请求中的异常,例如网络问题或404错误。此外,可以为下载过程设置重试机制,确保在遇到临时性问题时能够重新尝试下载。通过记录错误日志,用户可以在后续对下载失败的文件进行处理。
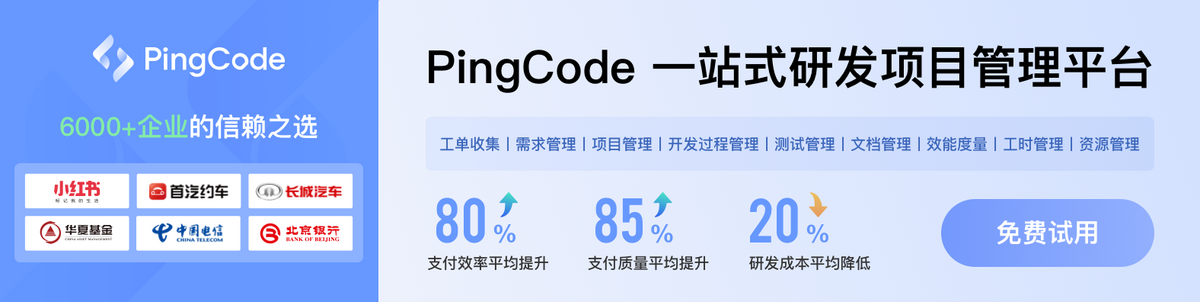