在Python中,变量继承是通过类的继承来实现的,类中的属性和方法可以被子类继承、子类可以重写父类中的变量、子类可以通过super()函数调用父类的变量。 在Python中,通过创建类和继承关系,可以实现变量和方法的继承。下面将详细介绍Python中变量继承的原理和实现方法。
一、类与继承的基本概念
在深入探讨变量继承之前,了解Python中的类和继承是至关重要的。类是面向对象编程中的核心概念,它是对象的蓝图或模板。继承则是类与类之间的一种关系,通过这种关系,一个类可以继承另一个类的属性和方法。
- 类的定义与实例化
在Python中,类是通过class关键字定义的,一个类可以包含多个属性和方法。属性是类的变量,而方法是类中的函数。在定义类时,可以为类指定初始属性值,这些属性在类的实例化过程中被初始化。
class Parent:
def __init__(self, name):
self.name = name
def greet(self):
print(f"Hello, my name is {self.name}")
实例化类
parent = Parent("Alice")
parent.greet() # 输出: Hello, my name is Alice
- 继承的实现
在Python中,类的继承是通过在定义子类时将父类作为参数传递给子类来实现的。子类会自动继承父类的所有属性和方法,并可以根据需要重写父类的方法。
class Child(Parent):
def __init__(self, name, age):
super().__init__(name)
self.age = age
def greet(self):
print(f"Hello, my name is {self.name} and I am {self.age} years old")
实例化子类
child = Child("Bob", 12)
child.greet() # 输出: Hello, my name is Bob and I am 12 years old
二、变量继承的实现与重写
在Python中,变量继承是通过类的继承机制实现的。子类不仅可以继承父类的变量,还可以重写这些变量或方法以实现自己的功能。
- 继承父类的变量
子类在继承父类时,可以直接访问父类的变量。这是因为子类继承了父类的属性和方法。通过这种方式,子类可以使用父类的变量而无需重复定义。
class Parent:
def __init__(self, name):
self.name = name
class Child(Parent):
def print_name(self):
print(f"The name is {self.name}")
child = Child("Charlie")
child.print_name() # 输出: The name is Charlie
- 子类重写父类的变量
在子类中,可以重写父类中的变量。重写是指在子类中为父类的变量赋予新的值或功能,以满足子类的特定需求。
class Parent:
def __init__(self, name):
self.name = name
class Child(Parent):
def __init__(self, name, age):
super().__init__(name)
self.age = age
def print_info(self):
print(f"The name is {self.name} and the age is {self.age}")
child = Child("Dave", 15)
child.print_info() # 输出: The name is Dave and the age is 15
三、使用super()函数调用父类变量
在子类中,如果需要调用或使用父类的构造函数或其他方法,可以使用super()函数。super()函数用于调用父类的方法和属性,特别是在重写父类的方法时,super()函数非常有用。
- super()函数的基本用法
super()函数主要用于调用父类的构造函数,以便在子类中初始化父类的属性。
class Parent:
def __init__(self, name):
self.name = name
class Child(Parent):
def __init__(self, name, age):
super().__init__(name)
self.age = age
child = Child("Eve", 10)
print(child.name) # 输出: Eve
- 在子类中调用父类的方法
在子类中,可以使用super()函数调用父类的方法。这可以确保父类的方法被正确执行,并且允许子类在调用父类的方法后进行额外的处理。
class Parent:
def greet(self):
print("Hello from the Parent class")
class Child(Parent):
def greet(self):
super().greet()
print("Hello from the Child class")
child = Child()
child.greet()
输出:
Hello from the Parent class
Hello from the Child class
四、变量的访问权限与继承
在Python中,变量的访问权限通过命名约定进行管理。虽然Python没有严格的访问控制机制,但可以通过变量命名规则实现变量的私有化和保护。
- 公有变量与私有变量
在Python中,默认情况下,类的变量是公有的,可以在类的外部访问和修改。如果需要将变量设置为私有,可以在变量名前加上双下划线(__),这将使变量仅在类内部可访问。
class Parent:
def __init__(self, name):
self.__name = name # 私有变量
def get_name(self):
return self.__name
parent = Parent("Frank")
print(parent.get_name()) # 输出: Frank
- 受保护的变量
受保护的变量在变量名前加单下划线(_)标识。这种变量可以在类和子类中访问,但不应在类的外部直接访问。这是一种约定俗成的保护机制。
class Parent:
def __init__(self, name):
self._name = name # 受保护的变量
class Child(Parent):
def print_name(self):
print(f"The name is {self._name}")
child = Child("Grace")
child.print_name() # 输出: The name is Grace
五、继承中的多态与变量
多态性是面向对象编程中的一个重要概念,它允许不同的类以相同的接口实现不同的功能。在Python中,多态性通过继承和方法重写实现。
- 多态的概念与实现
在继承关系中,子类可以重写父类的方法,这使得相同的方法在不同的类中可以表现出不同的行为。这种能力称为多态。
class Animal:
def sound(self):
pass
class Dog(Animal):
def sound(self):
print("Woof")
class Cat(Animal):
def sound(self):
print("Meow")
def animal_sound(animal: Animal):
animal.sound()
dog = Dog()
cat = Cat()
animal_sound(dog) # 输出: Woof
animal_sound(cat) # 输出: Meow
- 多态在变量继承中的应用
在变量继承中,多态性可以通过重写父类的变量和方法来实现,使得子类能够根据需要修改继承自父类的行为。
class Shape:
def __init__(self, name):
self.name = name
def area(self):
pass
class Square(Shape):
def __init__(self, side_length):
super().__init__("Square")
self.side_length = side_length
def area(self):
return self.side_length 2
class Circle(Shape):
def __init__(self, radius):
super().__init__("Circle")
self.radius = radius
def area(self):
return 3.14 * self.radius 2
def print_area(shape: Shape):
print(f"The area of the {shape.name} is {shape.area()}")
square = Square(4)
circle = Circle(3)
print_area(square) # 输出: The area of the Square is 16
print_area(circle) # 输出: The area of the Circle is 28.26
六、继承中的变量初始化与方法调用
在继承关系中,变量的初始化和方法调用是重要的环节。通过正确初始化变量和调用方法,可以确保类的实例化和功能的正确性。
- 子类的变量初始化
在子类中,可以通过调用父类的构造函数来初始化父类的变量。这样可以确保父类的属性在子类中得到正确的初始化。
class Parent:
def __init__(self, name):
self.name = name
class Child(Parent):
def __init__(self, name, age):
super().__init__(name)
self.age = age
child = Child("Hank", 14)
print(f"Name: {child.name}, Age: {child.age}")
输出: Name: Hank, Age: 14
- 调用父类的方法
在子类中,可以通过super()函数调用父类的方法。这种调用机制允许子类在执行自己的逻辑之前或之后调用父类的逻辑。
class Parent:
def greet(self):
print("Greetings from the Parent class")
class Child(Parent):
def greet(self):
super().greet()
print("Greetings from the Child class")
child = Child()
child.greet()
输出:
Greetings from the Parent class
Greetings from the Child class
通过以上内容的详细介绍,我们可以看到,在Python中实现变量继承主要依赖于类的继承机制。通过理解类的定义、继承、变量的初始化与重写、访问权限、多态性等概念,可以更好地在Python中进行面向对象编程。继承不仅提高了代码的重用性,还增强了代码的结构性和可读性,为开发复杂的软件系统提供了有力的支持。
相关问答FAQs:
如何在Python中使用变量进行继承?
在Python中,继承通常是通过类实现的。变量的继承是通过类的属性来完成的。当一个类继承另一个类时,子类将自动获取父类的属性和方法。如果想要在子类中使用父类的变量,可以直接通过self
引用父类的属性。
Python中变量的作用域如何影响继承?
变量的作用域指的是变量在程序中可访问的范围。在Python中,局部变量在函数内部可用,而类属性则是全局可用的。在继承中,子类可以访问父类的全局属性,但不能访问父类内部定义的局部变量。如果希望在子类中使用父类的局部变量,可以通过方法来实现。
在Python中如何重写父类变量?
在Python中,子类可以重写父类的变量,这样可以修改其值以满足特定需求。重写是通过在子类中定义与父类同名的变量实现的。当子类实例化时,访问该变量将返回子类定义的值,而不是父类的值。注意,重写变量可能会导致子类与父类之间的关系变得复杂,因此在设计时需谨慎考虑。
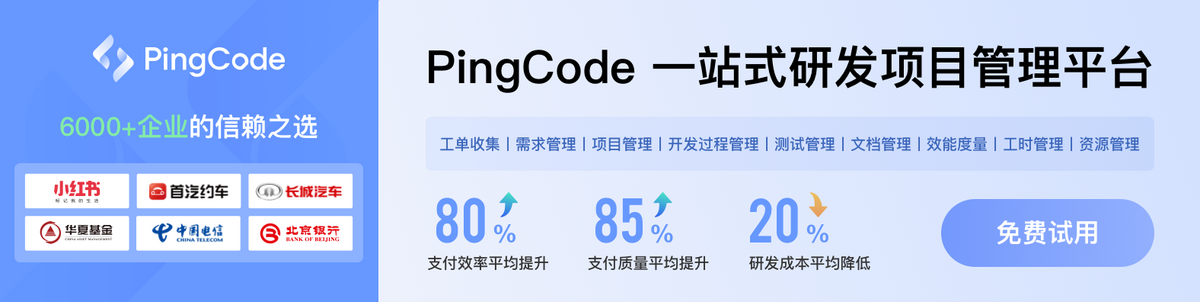