在Python中,表示行数的方法有多种,具体取决于您需要获取行数的对象或场景。可以使用文件对象的readlines()
方法获取文件中的行数、可以使用len()
函数结合列表理解获取列表中元素的行数、可以使用pandas
库的DataFrame
对象的shape
属性获取数据框的行数、可以使用enumerate()
函数结合循环获取文本行号。下面将详细介绍每种方法及其应用场景。
一、文件对象的行数
在处理文件时,获取文件的行数是常见的需求。Python提供了多种方法来实现这一点。
1.1 使用readlines()
readlines()
方法用于读取文件中的所有行,并将其存储在一个列表中。通过获取该列表的长度,可以得到文件的行数。
with open('example.txt', 'r') as file:
lines = file.readlines()
num_of_lines = len(lines)
print(f"Number of lines in the file: {num_of_lines}")
这种方法简单直接,但在处理大型文件时,由于需要将所有行加载到内存中,可能会导致内存占用过高。
1.2 使用循环计数
对于大型文件,可以使用循环逐行读取文件,并计数行数,以节省内存。
num_of_lines = 0
with open('example.txt', 'r') as file:
for line in file:
num_of_lines += 1
print(f"Number of lines in the file: {num_of_lines}")
这种方法在处理大型文件时更为高效,因为它不需要将整个文件加载到内存中。
二、列表中元素的行数
在Python中,列表是一种常用的数据结构,有时我们需要知道列表中有多少个元素,以表示行数。
2.1 使用len()
len()
函数是Python内置的用于获取对象长度的函数。对于列表,可以直接使用len()
来获取其中元素的数量。
my_list = ['line 1', 'line 2', 'line 3']
num_of_lines = len(my_list)
print(f"Number of elements in the list: {num_of_lines}")
这种方法简单明了,适用于各种列表。
2.2 结合列表理解
列表理解是一种简洁的创建列表的方法,有时我们需要根据某些条件创建列表并获取其长度。
my_list = ['line 1', 'line 2', 'line 3']
filtered_list = [line for line in my_list if '1' in line]
num_of_lines = len(filtered_list)
print(f"Number of elements in the filtered list: {num_of_lines}")
这种方法可以用于根据特定条件筛选元素,并获取筛选后的行数。
三、Pandas DataFrame的行数
在数据分析中,pandas
库是非常强大的工具,其DataFrame
对象提供了便捷的方法来获取数据框的行数。
3.1 使用shape
属性
DataFrame
的shape
属性返回一个包含行数和列数的元组。通过访问该元组的第一个元素,可以获取行数。
import pandas as pd
data = {'Column1': [1, 2, 3], 'Column2': [4, 5, 6]}
df = pd.DataFrame(data)
num_of_rows = df.shape[0]
print(f"Number of rows in the DataFrame: {num_of_rows}")
这种方法简单高效,是获取DataFrame
行数的标准方式。
3.2 使用len()
虽然shape
属性是推荐的方法,但在某些情况下,可以使用len()
函数结合DataFrame
的index
属性来获取行数。
num_of_rows = len(df.index)
print(f"Number of rows in the DataFrame using len: {num_of_rows}")
这种方法提供了另一种获取行数的途径,但在大多数情况下,使用shape
属性更为简洁。
四、文本处理中的行号
在文本处理中,有时需要在处理过程中获取当前行的行号。Python提供了多种方法来实现这一点。
4.1 使用enumerate()
enumerate()
函数用于在遍历可迭代对象时获取元素及其索引。对于文件对象,可以使用enumerate()
获取当前行的行号。
with open('example.txt', 'r') as file:
for line_number, line in enumerate(file, start=1):
print(f"Line {line_number}: {line.strip()}")
这种方法非常适合在处理文件时同时获取行内容和行号。
4.2 自定义计数器
虽然enumerate()
是推荐的方法,但在某些情况下,可以使用自定义计数器来获取行号。
line_number = 0
with open('example.txt', 'r') as file:
for line in file:
line_number += 1
print(f"Line {line_number}: {line.strip()}")
这种方法稍显繁琐,但提供了对行号计数的完全控制。
五、字符串中的行数
在某些应用中,我们可能需要确定字符串中有多少行。可以通过分割字符串来实现。
5.1 使用splitlines()
splitlines()
方法用于将字符串按行分割为一个列表,进而可以获取字符串的行数。
multi_line_string = """Line 1
Line 2
Line 3"""
lines = multi_line_string.splitlines()
num_of_lines = len(lines)
print(f"Number of lines in the string: {num_of_lines}")
这种方法简单直接,适用于多行字符串。
5.2 使用split('\n')
尽管splitlines()
是更合适的方法,但也可以使用split('\n')
来分割字符串并获取行数。
lines = multi_line_string.split('\n')
num_of_lines = len(lines)
print(f"Number of lines in the string using split: {num_of_lines}")
这种方法提供了另一种选择,但在大多数情况下,splitlines()
更为推荐。
六、日志文件的行数
在处理日志文件时,获取日志的行数通常是必要的步骤。可以使用多种方法来实现这一点。
6.1 使用readlines()
与普通文件类似,可以使用readlines()
方法来获取日志文件的行数。
with open('logfile.log', 'r') as file:
lines = file.readlines()
num_of_lines = len(lines)
print(f"Number of lines in the log file: {num_of_lines}")
这种方法适合小型日志文件的处理。
6.2 使用生成器表达式
在处理大型日志文件时,可以使用生成器表达式逐行计数,以节省内存。
num_of_lines = sum(1 for line in open('logfile.log'))
print(f"Number of lines in the log file using generator: {num_of_lines}")
这种方法高效且内存占用低,适合大型日志文件。
七、数据流的行数
在处理实时数据流时,获取当前接收到的行数可能是重要的需求。可以使用流式处理的方法来实现。
7.1 使用计数器
在处理数据流时,可以维护一个计数器来记录接收到的行数。
line_number = 0
def process_stream(stream):
global line_number
for line in stream:
line_number += 1
# Process the line
print(f"Total lines processed: {line_number}")
这种方法简单且有效,适用于流式数据的处理。
7.2 使用回调函数
在某些数据流处理框架中,可以使用回调函数来记录接收到的行数。
def on_line_received(line):
on_line_received.line_number += 1
# Process the line
on_line_received.line_number = 0
Assuming `stream` is a data stream
for line in stream:
on_line_received(line)
print(f"Total lines processed: {on_line_received.line_number}")
这种方法提供了灵活的行数记录机制,适用于复杂的数据流处理场景。
八、JSON数据的行数
在处理JSON数据时,获取数据结构中包含的行数或记录数可能是必要的。可以使用Python内置的json
模块来实现。
8.1 解析JSON数组
对于包含数组的JSON数据,可以通过解析数组并获取其长度来确定行数。
import json
json_data = '''
[
{"name": "Alice", "age": 30},
{"name": "Bob", "age": 25}
]
'''
data = json.loads(json_data)
num_of_records = len(data)
print(f"Number of records in JSON: {num_of_records}")
这种方法适用于简单的JSON数组。
8.2 处理嵌套结构
对于更复杂的嵌套JSON结构,可以使用递归方法来计算总行数或记录数。
def count_records(data):
if isinstance(data, list):
return sum(count_records(item) for item in data)
elif isinstance(data, dict):
return sum(count_records(value) for value in data.values())
else:
return 1
json_data = '''
{
"users": [
{"name": "Alice", "age": 30},
{"name": "Bob", "age": 25}
],
"admins": [
{"name": "Charlie", "age": 35}
]
}
'''
data = json.loads(json_data)
num_of_records = count_records(data)
print(f"Total number of records in JSON: {num_of_records}")
这种方法灵活且强大,适用于复杂的JSON数据结构。
九、XML数据的行数
在处理XML数据时,确定元素或节点的数量可以通过多种方法实现。
9.1 使用xml.etree.ElementTree
Python的xml.etree.ElementTree
模块提供了处理XML数据的基本方法,可以用来获取元素的数量。
import xml.etree.ElementTree as ET
xml_data = '''
<root>
<user>
<name>Alice</name>
<age>30</age>
</user>
<user>
<name>Bob</name>
<age>25</age>
</user>
</root>
'''
root = ET.fromstring(xml_data)
num_of_users = len(root.findall('user'))
print(f"Number of user elements: {num_of_users}")
这种方法简单易用,适合基本的XML处理。
9.2 使用lxml
库
对于更复杂的XML处理,可以使用功能更强大的lxml
库。
from lxml import etree
xml_data = '''
<root>
<user>
<name>Alice</name>
<age>30</age>
</user>
<user>
<name>Bob</name>
<age>25</age>
</user>
</root>
'''
root = etree.fromstring(xml_data)
num_of_users = len(root.xpath('//user'))
print(f"Number of user elements using lxml: {num_of_users}")
这种方法提供了强大的XPath支持,适用于复杂的XML查询和处理。
十、数据库查询结果的行数
在处理数据库查询时,获取查询结果的行数通常是必要的步骤。可以使用数据库连接库来实现。
10.1 使用sqlite3
对于SQLite数据库,可以使用sqlite3
模块进行查询,并获取结果的行数。
import sqlite3
conn = sqlite3.connect('example.db')
cursor = conn.cursor()
cursor.execute('SELECT * FROM users')
rows = cursor.fetchall()
num_of_rows = len(rows)
print(f"Number of rows in query result: {num_of_rows}")
conn.close()
这种方法适用于简单的SQLite数据库查询。
10.2 使用pandas
对于更复杂的数据库查询,可以使用pandas
库将查询结果加载到DataFrame
中,并使用其shape
属性获取行数。
import pandas as pd
conn = sqlite3.connect('example.db')
df = pd.read_sql_query('SELECT * FROM users', conn)
num_of_rows = df.shape[0]
print(f"Number of rows in query result using pandas: {num_of_rows}")
conn.close()
这种方法适用于更复杂的数据库操作,并提供了数据分析的额外功能。
十一、图像数据的行数
在处理图像数据时,确定图像的行数(即像素高度)可能是必要的。这可以通过图像处理库来实现。
11.1 使用PIL
库
Python Imaging Library(PIL)或其派生版本Pillow
可以用于获取图像的尺寸。
from PIL import Image
image = Image.open('example.jpg')
width, height = image.size
print(f"Image height (number of rows): {height}")
这种方法简单直接,适合基本的图像处理。
11.2 使用OpenCV
对于更复杂的图像处理,可以使用OpenCV
库。
import cv2
image = cv2.imread('example.jpg')
height, width, channels = image.shape
print(f"Image height (number of rows) using OpenCV: {height}")
这种方法强大且高效,适用于复杂的图像处理任务。
十二、音频数据的行数
在处理音频数据时,获取音频文件的帧数(行数)可能是必要的。这可以通过音频处理库来实现。
12.1 使用wave
模块
Python的wave
模块可以用于处理WAV格式的音频文件,并获取帧数。
import wave
with wave.open('example.wav', 'rb') as audio:
num_of_frames = audio.getnframes()
print(f"Number of frames in audio: {num_of_frames}")
这种方法简单且适用于WAV格式的基本音频处理。
12.2 使用pydub
库
对于更复杂的音频处理,可以使用pydub
库。
from pydub import AudioSegment
audio = AudioSegment.from_file('example.wav')
num_of_frames = len(audio.get_array_of_samples())
print(f"Number of frames in audio using pydub: {num_of_frames}")
这种方法提供了更广泛的音频格式支持和处理能力。
十三、视频数据的行数
在处理视频数据时,获取视频帧数(行数)可能是必要的。这可以通过视频处理库来实现。
13.1 使用cv2
库
OpenCV的cv2
模块可以用于处理视频文件,并获取总帧数。
import cv2
video = cv2.VideoCapture('example.mp4')
num_of_frames = int(video.get(cv2.CAP_PROP_FRAME_COUNT))
print(f"Number of frames in video: {num_of_frames}")
video.release()
这种方法强大且高效,适用于基本的视频处理。
13.2 使用imageio
库
对于更复杂的视频处理,可以使用imageio
库。
import imageio
video = imageio.get_reader('example.mp4')
num_of_frames = video.count_frames()
print(f"Number of frames in video using imageio: {num_of_frames}")
这种方法提供了更广泛的视频格式支持和处理能力。
十四、科学数据的行数
在科学计算中,获取数据集的行数通常是必要的。这可以通过科学计算库来实现。
14.1 使用numpy
库
对于多维数组,可以使用numpy
库获取行数。
import numpy as np
array = np.array([[1, 2, 3], [4, 5, 6]])
num_of_rows = array.shape[0]
print(f"Number of rows in numpy array: {num_of_rows}")
这种方法简单且高效,适用于基本的科学计算。
14.2 使用scipy
库
对于更复杂的科学数据集,可以使用scipy
库。
from scipy import io
mat_data = io.loadmat('example.mat')
num_of_rows = mat_data['variable'].shape[0]
print(f"Number of rows in scipy data: {num_of_rows}")
这种方法提供了对多种科学数据格式的支持,适用于复杂的科学计算任务。
总结:在Python中,获取行数的方法多种多样,具体选择取决于数据的类型和处理场景。了解每种方法的优缺点和适用场合,可以帮助您更有效地解决问题。通过不断实践,您将能够熟练地选择和应用这些方法来满足不同的需求。
相关问答FAQs:
如何在Python中获取文件的总行数?
在Python中,可以通过使用文件的读取方法来计算总行数。打开文件后,逐行读取并计数,或者使用readlines()
方法将所有行读入列表,然后计算列表的长度。示例代码如下:
with open('filename.txt', 'r') as file:
line_count = sum(1 for line in file)
print(f'文件总行数: {line_count}')
在Python中如何跳过特定行数的读取?
若希望在读取文件时跳过特定的行数,可以使用enumerate()
结合continue
语句。例如,若要跳过文件的前两行,可以这样实现:
with open('filename.txt', 'r') as file:
for index, line in enumerate(file):
if index < 2:
continue
print(line.strip())
这段代码将跳过前两行并输出后续的所有行。
如何在Python中统计特定内容的行数?
若需要统计文件中包含特定关键词的行数,可以在读取文件的同时进行条件判断。以下是一个示例,统计包含“Python”这个词的行数:
keyword = 'Python'
with open('filename.txt', 'r') as file:
keyword_count = sum(1 for line in file if keyword in line)
print(f'包含"{keyword}"的行数: {keyword_count}')
这种方式在处理大文件时非常高效,可以快速得到所需的信息。
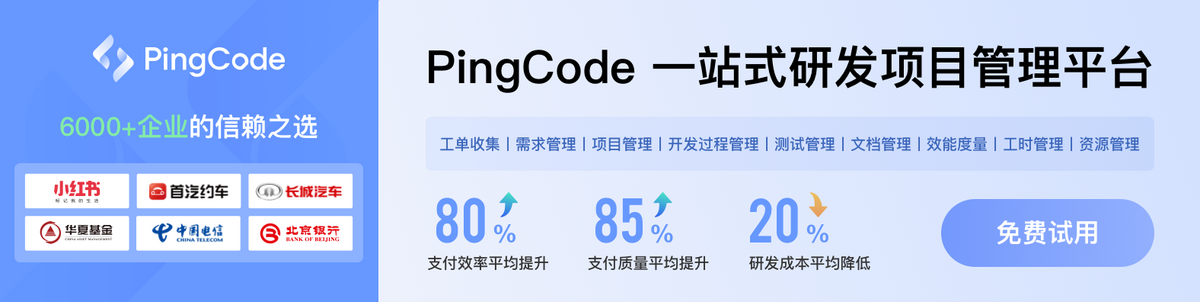