Python 批量做批注的方法包括使用批处理文件操作、自动化脚本、第三方库等。常用的方法有:使用Python的文件操作功能、利用第三方库如docx、openpyxl、PyPDF2等。其中,最推荐的是利用第三方库进行批量处理,因为这些库提供了更强大的功能和更简洁的接口。 接下来,我们将详细描述如何使用这些方法来批量做批注。
一、使用 Python 文件操作功能
Python 提供了强大的文件操作功能,可以通过读取、写入文件来实现批量做批注。
1.1 读取和写入文本文件
首先,我们来看一下如何读取和写入文本文件。以下是一个简单的示例,演示如何在文本文件的每一行后面添加批注:
def add_comments_to_file(input_file, output_file, comment):
with open(input_file, 'r') as infile, open(output_file, 'w') as outfile:
for line in infile:
outfile.write(line.strip() + ' ' + comment + '\n')
add_comments_to_file('input.txt', 'output.txt', '# This is a comment')
这个示例代码读取 input.txt
文件的每一行,并在每行的末尾添加注释,然后将结果写入 output.txt
文件。
1.2 处理多种文件类型
对于不同类型的文件(如CSV、JSON等),可以使用相应的库进行处理。例如,处理CSV文件可以使用 csv
库:
import csv
def add_comments_to_csv(input_file, output_file, comment):
with open(input_file, 'r') as infile, open(output_file, 'w', newline='') as outfile:
reader = csv.reader(infile)
writer = csv.writer(outfile)
for row in reader:
row.append(comment)
writer.writerow(row)
add_comments_to_csv('input.csv', 'output.csv', 'This is a comment')
这个示例代码读取 input.csv
文件的每一行,并在每行的末尾添加注释,然后将结果写入 output.csv
文件。
二、利用第三方库
使用第三方库可以更方便地处理不同类型的文件,并添加批注。
2.1 批量处理 Word 文档
可以使用 python-docx
库来处理 Word 文档,并在文档中添加批注。以下是一个示例,演示如何在 Word 文档的每一段后面添加批注:
from docx import Document
def add_comments_to_docx(input_file, output_file, comment):
doc = Document(input_file)
for paragraph in doc.paragraphs:
paragraph.add_run(' ' + comment)
doc.save(output_file)
add_comments_to_docx('input.docx', 'output.docx', 'This is a comment')
这个示例代码读取 input.docx
文件的每一段,并在每段的末尾添加注释,然后将结果保存到 output.docx
文件。
2.2 批量处理 Excel 文件
可以使用 openpyxl
库来处理 Excel 文件,并在表格中添加批注。以下是一个示例,演示如何在 Excel 表格的每一行后面添加批注:
import openpyxl
def add_comments_to_excel(input_file, output_file, comment):
workbook = openpyxl.load_workbook(input_file)
sheet = workbook.active
for row in sheet.iter_rows():
row[-1].value = str(row[-1].value) + ' ' + comment
workbook.save(output_file)
add_comments_to_excel('input.xlsx', 'output.xlsx', 'This is a comment')
这个示例代码读取 input.xlsx
文件的每一行,并在每行的末尾添加注释,然后将结果保存到 output.xlsx
文件。
2.3 批量处理 PDF 文件
可以使用 PyPDF2
库来处理 PDF 文件,并在文档中添加批注。以下是一个示例,演示如何在 PDF 文档的每一页添加批注:
import PyPDF2
def add_comments_to_pdf(input_file, output_file, comment):
pdf_reader = PyPDF2.PdfFileReader(input_file)
pdf_writer = PyPDF2.PdfFileWriter()
for page_num in range(pdf_reader.getNumPages()):
page = pdf_reader.getPage(page_num)
page.mergeText(comment, x=50, y=50) # 添加批注文本到页面
pdf_writer.addPage(page)
with open(output_file, 'wb') as output_pdf:
pdf_writer.write(output_pdf)
add_comments_to_pdf('input.pdf', 'output.pdf', 'This is a comment')
这个示例代码读取 input.pdf
文件的每一页,并在每页添加批注,然后将结果保存到 output.pdf
文件。
三、自动化批处理脚本
除了使用文件操作和第三方库,还可以编写自动化脚本来批量处理多个文件。例如,可以编写一个脚本,遍历指定目录下的所有文件,并为每个文件添加批注。
3.1 遍历目录并处理文件
以下是一个示例脚本,演示如何遍历目录并为每个文件添加批注:
import os
def add_comments_to_files(directory, comment):
for filename in os.listdir(directory):
input_file = os.path.join(directory, filename)
output_file = os.path.join(directory, 'annotated_' + filename)
if filename.endswith('.txt'):
add_comments_to_file(input_file, output_file, comment)
elif filename.endswith('.csv'):
add_comments_to_csv(input_file, output_file, comment)
elif filename.endswith('.docx'):
add_comments_to_docx(input_file, output_file, comment)
elif filename.endswith('.xlsx'):
add_comments_to_excel(input_file, output_file, comment)
elif filename.endswith('.pdf'):
add_comments_to_pdf(input_file, output_file, comment)
add_comments_to_files('files_directory', 'This is a comment')
这个脚本遍历 files_directory
目录下的所有文件,并为每个文件添加批注。根据文件的扩展名,调用相应的处理函数。
四、结合正则表达式处理批注
在某些情况下,可能需要更复杂的批注处理逻辑,例如在特定模式的文本后面添加批注。可以使用正则表达式来实现这样的需求。
4.1 在特定模式后添加批注
以下是一个示例,演示如何在匹配特定模式的文本后面添加批注:
import re
def add_comments_after_pattern(input_file, output_file, pattern, comment):
with open(input_file, 'r') as infile, open(output_file, 'w') as outfile:
for line in infile:
modified_line = re.sub(pattern, lambda match: match.group(0) + ' ' + comment, line)
outfile.write(modified_line)
pattern = r'\bpattern\b' # 匹配单词 'pattern'
add_comments_after_pattern('input.txt', 'output.txt', pattern, 'This is a comment')
这个示例代码读取 input.txt
文件的每一行,并在匹配模式 pattern
的文本后面添加注释,然后将结果写入 output.txt
文件。
五、处理大文件和并行处理
对于大文件或大量文件,可以使用并行处理来提高处理速度。Python 提供了 multiprocessing
库,可以方便地实现并行处理。
5.1 使用多进程处理文件
以下是一个示例,演示如何使用多进程批量处理文件:
import os
from multiprocessing import Pool
def process_file(args):
input_file, output_file, comment = args
if input_file.endswith('.txt'):
add_comments_to_file(input_file, output_file, comment)
elif input_file.endswith('.csv'):
add_comments_to_csv(input_file, output_file, comment)
elif input_file.endswith('.docx'):
add_comments_to_docx(input_file, output_file, comment)
elif input_file.endswith('.xlsx'):
add_comments_to_excel(input_file, output_file, comment)
elif input_file.endswith('.pdf'):
add_comments_to_pdf(input_file, output_file, comment)
def add_comments_to_files_parallel(directory, comment):
files = [(os.path.join(directory, filename), os.path.join(directory, 'annotated_' + filename), comment)
for filename in os.listdir(directory)]
with Pool() as pool:
pool.map(process_file, files)
add_comments_to_files_parallel('files_directory', 'This is a comment')
这个示例代码使用多进程并行处理 files_directory
目录下的所有文件,并为每个文件添加批注。
六、总结
通过以上方法,我们可以使用 Python 批量处理不同类型的文件,并添加批注。具体方法包括:使用文件操作功能处理文本文件、利用第三方库处理 Word、Excel 和 PDF 文件、编写自动化脚本遍历目录、结合正则表达式处理复杂模式、以及使用并行处理提高处理速度。根据具体需求选择合适的方法,可以高效地实现批量做批注的任务。
相关问答FAQs:
如何在Python中使用库来批量做批注?
在Python中,有多个库可以帮助你批量做批注。例如,你可以使用Pandas
库来处理数据,并利用Matplotlib
或Seaborn
库来可视化数据并添加批注。通过循环遍历数据集,你可以在图表中为多个数据点添加批注,提升可读性。
有哪些常见的批注方法可以在Python中实现?
在Python中,常见的批注方法包括文本批注、箭头指示和高亮显示。文本批注可以直接在图表中添加说明,箭头指示则可以用来强调特定的数据点,而高亮显示则能帮助用户快速找到关注的部分。这些方法可以通过Matplotlib
的annotate
函数轻松实现。
如何提高批量批注的效率?
为了提高批量批注的效率,可以预先定义批注内容和位置,将其存储在数据结构中(如列表或字典)。在绘制图表时,通过循环自动添加批注,避免手动输入,提高了代码的复用性和可维护性。此外,考虑使用函数来封装批注逻辑,这样可以在不同的图表中重复使用。
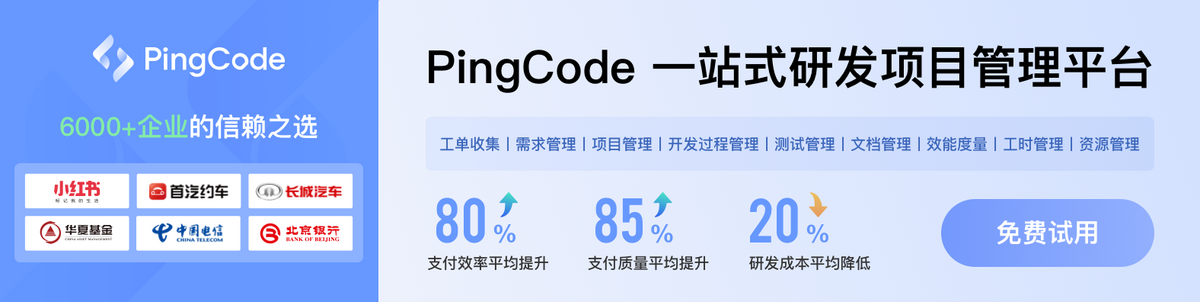