Python去除字符串双引号的方法有多种,包括使用strip()方法、replace()方法、正则表达式等。 其中,replace()方法是最常用且简单的方式,它能够将字符串中的所有双引号替换为空字符串,从而达到去除双引号的目的。具体实现方法如下:
string_with_quotes = '"Hello, World!"'
string_without_quotes = string_with_quotes.replace('"', '')
print(string_without_quotes)
这个代码会输出:Hello, World!
,即去除了双引号后的字符串。接下来,我们将详细介绍其他方法及其实现方式。
一、使用strip()方法
strip()方法用于移除字符串头尾指定的字符(默认为空格)。它不能直接去除字符串中间的双引号,但可以用于去除字符串两端的双引号。
string_with_quotes = '"Hello, World!"'
string_without_quotes = string_with_quotes.strip('"')
print(string_without_quotes)
这个代码会输出:Hello, World!
。需要注意的是,strip()方法仅移除字符串两端的双引号,对于字符串中间的双引号无效。
二、使用replace()方法
replace()方法是最常用的方式,它可以替换字符串中的所有匹配项。
string_with_quotes = '"Hello, "World!""'
string_without_quotes = string_with_quotes.replace('"', '')
print(string_without_quotes)
这个代码会输出:Hello, World!
,即去除了所有双引号。
三、使用正则表达式
正则表达式是一种强大的字符串处理工具,可以用于复杂的字符串匹配和替换。使用re模块中的sub()方法可以去除字符串中的双引号。
import re
string_with_quotes = '"Hello, "World!""'
string_without_quotes = re.sub(r'"', '', string_with_quotes)
print(string_without_quotes)
这个代码会输出:Hello, World!
。正则表达式虽然强大,但对于简单的替换操作来说显得有些复杂。
四、使用列表解析
列表解析是一种简洁的方式,通过条件筛选保留字符串中非双引号的字符。
string_with_quotes = '"Hello, "World!""'
string_without_quotes = ''.join([char for char in string_with_quotes if char != '"'])
print(string_without_quotes)
这个代码会输出:Hello, World!
。列表解析适用于简单的字符过滤操作。
五、使用translate()方法
translate()方法通过字符映射表进行替换操作,需要结合str.maketrans()方法创建映射表。
string_with_quotes = '"Hello, "World!""'
translation_table = str.maketrans('', '', '"')
string_without_quotes = string_with_quotes.translate(translation_table)
print(string_without_quotes)
这个代码会输出:Hello, World!
。translate()方法适用于大规模的字符替换操作。
六、使用递归方法
递归方法是一种递归地处理字符串的方法,适用于需要逐个字符处理的情况。
def remove_quotes(s):
if not s:
return s
if s[0] == '"':
return remove_quotes(s[1:])
return s[0] + remove_quotes(s[1:])
string_with_quotes = '"Hello, "World!""'
string_without_quotes = remove_quotes(string_with_quotes)
print(string_without_quotes)
这个代码会输出:Hello, World!
。递归方法虽然灵活,但对于大字符串的处理性能较低。
七、使用字符串切片
字符串切片是一种通过索引操作来截取字符串的方法,适用于去除字符串两端的双引号。
string_with_quotes = '"Hello, World!"'
string_without_quotes = string_with_quotes[1:-1]
print(string_without_quotes)
这个代码会输出:Hello, World!
。字符串切片只能去除固定位置的双引号。
八、使用生成器
生成器是一种延迟计算的迭代器,适用于大规模的字符串处理。
def remove_quotes_generator(s):
for char in s:
if char != '"':
yield char
string_with_quotes = '"Hello, "World!""'
string_without_quotes = ''.join(remove_quotes_generator(string_with_quotes))
print(string_without_quotes)
这个代码会输出:Hello, World!
。生成器能够高效地处理大字符串,但代码较为复杂。
九、使用字符串拼接
字符串拼接是一种通过逐个字符拼接成新字符串的方法,适用于逐个字符处理的情况。
string_with_quotes = '"Hello, "World!""'
string_without_quotes = ''.join(char for char in string_with_quotes if char != '"')
print(string_without_quotes)
这个代码会输出:Hello, World!
。字符串拼接适用于简单的字符过滤操作。
十、使用字符串替换的组合方法
组合使用多种方法,可以提高代码的灵活性和鲁棒性。
string_with_quotes = '"Hello, "World!""'
string_without_quotes = string_with_quotes.replace('"', '').strip()
print(string_without_quotes)
这个代码会输出:Hello, World!
。组合方法适用于复杂的字符串处理需求。
综上所述,Python提供了多种方法去除字符串中的双引号,每种方法都有其适用的场景和优缺点。根据具体需求选择合适的方法,可以提高代码的可读性和执行效率。
相关问答FAQs:
如何在Python中去除字符串中的双引号?
在Python中,可以使用字符串的replace()
方法来去除字符串中的双引号。例如,my_string.replace('"', '')
会将所有双引号替换为空字符,从而实现去除的效果。另一种方法是使用字符串切片或正则表达式来处理更复杂的情况。
使用正则表达式去除字符串中的双引号有什么优势?
使用正则表达式可以处理更复杂的字符串模式,例如同时去除双引号和其他特殊字符。通过re.sub(r'"', '', my_string)
,你可以灵活地定义需要移除的字符,适合处理多种格式的数据。
在处理用户输入时,如何安全地去除双引号?
处理用户输入时,使用strip()
和replace()
结合是一个好方法。my_input.strip().replace('"', '')
可以去除字符串两端的空白字符和内部的双引号,确保输入的清洁性和安全性,防止潜在的注入攻击或格式错误。
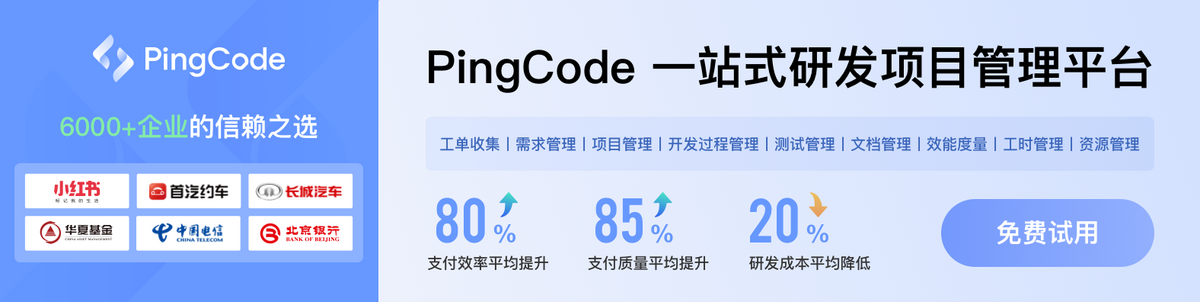