在Python中,可以使用Matplotlib库在大图上绘制子图、使用subplot()函数指定子图位置、使用GridSpec控制子图布局。这三点是实现这一功能的核心。下面将详细介绍其中一点,即使用subplot()函数指定子图位置。
使用subplot()函数指定子图位置是指通过Matplotlib库的subplot()函数来在一个大图上分割出多个子图区域,然后在每个子图区域内绘制各自的图形。subplot()函数的基本调用格式是subplot(nrows, ncols, index),其中nrows和ncols分别表示将大图分成的行数和列数,index表示子图的位置。比如,subplot(2, 3, 4)表示将大图分成2行3列的网格,并在第4个位置绘制子图。
接下来,将详细介绍如何使用Matplotlib库在大图上绘制子图的方法。
一、导入必要的库
首先,需要导入Matplotlib库以及其他可能需要的库,如NumPy。
import matplotlib.pyplot as plt
import numpy as np
二、使用subplot()函数绘制子图
1、创建简单的子图
最基本的方式是使用subplot()函数来创建多个子图。下面是一个简单的示例:
x = np.linspace(0, 10, 100)
fig = plt.figure()
第一个子图
plt.subplot(2, 2, 1)
plt.plot(x, np.sin(x))
plt.title('Sine Wave')
第二个子图
plt.subplot(2, 2, 2)
plt.plot(x, np.cos(x))
plt.title('Cosine Wave')
第三个子图
plt.subplot(2, 2, 3)
plt.plot(x, np.tan(x))
plt.title('Tangent Wave')
第四个子图
plt.subplot(2, 2, 4)
plt.plot(x, np.exp(x))
plt.title('Exponential Curve')
plt.tight_layout()
plt.show()
在这个例子中,我们将大图分成了2行2列的网格,并在每个位置上绘制了不同的图形。
2、调整子图之间的间距
使用subplot()函数时,可以通过tight_layout()函数来自动调整子图之间的间距,避免子图重叠。
fig = plt.figure()
plt.subplot(2, 3, 1)
plt.plot(x, np.sin(x))
plt.title('Sine Wave')
plt.subplot(2, 3, 2)
plt.plot(x, np.cos(x))
plt.title('Cosine Wave')
plt.subplot(2, 3, 3)
plt.plot(x, np.tan(x))
plt.title('Tangent Wave')
plt.subplot(2, 3, 4)
plt.plot(x, np.exp(x))
plt.title('Exponential Curve')
plt.subplot(2, 3, 5)
plt.plot(x, np.log(x + 1))
plt.title('Logarithmic Curve')
plt.tight_layout()
plt.show()
三、使用GridSpec控制子图布局
GridSpec是Matplotlib中更高级的子图布局控制方式,可以实现更加灵活的子图排列。
1、基本用法
import matplotlib.gridspec as gridspec
fig = plt.figure()
gs = gridspec.GridSpec(2, 3)
ax1 = fig.add_subplot(gs[0, 0])
ax1.plot(x, np.sin(x))
ax1.set_title('Sine Wave')
ax2 = fig.add_subplot(gs[0, 1])
ax2.plot(x, np.cos(x))
ax2.set_title('Cosine Wave')
ax3 = fig.add_subplot(gs[0, 2])
ax3.plot(x, np.tan(x))
ax3.set_title('Tangent Wave')
ax4 = fig.add_subplot(gs[1, :])
ax4.plot(x, np.exp(x))
ax4.set_title('Exponential Curve')
plt.tight_layout()
plt.show()
在这个例子中,我们使用GridSpec将大图分成2行3列的网格,并通过索引来指定每个子图的位置。其中,gs[1, :]表示将第二行合并成一个大子图。
2、调整子图比例
GridSpec还可以通过调整子图的比例来实现不同大小的子图。
fig = plt.figure()
gs = gridspec.GridSpec(2, 3, width_ratios=[1, 2, 1], height_ratios=[1, 2])
ax1 = fig.add_subplot(gs[0, 0])
ax1.plot(x, np.sin(x))
ax1.set_title('Sine Wave')
ax2 = fig.add_subplot(gs[0, 1])
ax2.plot(x, np.cos(x))
ax2.set_title('Cosine Wave')
ax3 = fig.add_subplot(gs[0, 2])
ax3.plot(x, np.tan(x))
ax3.set_title('Tangent Wave')
ax4 = fig.add_subplot(gs[1, :])
ax4.plot(x, np.exp(x))
ax4.set_title('Exponential Curve')
plt.tight_layout()
plt.show()
在这个例子中,我们通过width_ratios和height_ratios参数来设置每列和每行的比例,从而实现不同大小的子图。
四、使用subplot2grid()函数控制子图布局
subplot2grid()函数是另一个灵活的子图布局控制方式,可以更加方便地指定子图的位置和大小。
1、基本用法
fig = plt.figure()
ax1 = plt.subplot2grid((2, 3), (0, 0))
ax1.plot(x, np.sin(x))
ax1.set_title('Sine Wave')
ax2 = plt.subplot2grid((2, 3), (0, 1))
ax2.plot(x, np.cos(x))
ax2.set_title('Cosine Wave')
ax3 = plt.subplot2grid((2, 3), (0, 2))
ax3.plot(x, np.tan(x))
ax3.set_title('Tangent Wave')
ax4 = plt.subplot2grid((2, 3), (1, 0), colspan=3)
ax4.plot(x, np.exp(x))
ax4.set_title('Exponential Curve')
plt.tight_layout()
plt.show()
在这个例子中,我们使用subplot2grid()函数将大图分成2行3列的网格,并通过指定起始位置和跨度来控制子图的位置和大小。
2、调整子图比例
同样,可以通过调整子图的比例来实现不同大小的子图。
fig = plt.figure()
ax1 = plt.subplot2grid((3, 3), (0, 0))
ax1.plot(x, np.sin(x))
ax1.set_title('Sine Wave')
ax2 = plt.subplot2grid((3, 3), (0, 1), colspan=2)
ax2.plot(x, np.cos(x))
ax2.set_title('Cosine Wave')
ax3 = plt.subplot2grid((3, 3), (1, 0), rowspan=2)
ax3.plot(x, np.tan(x))
ax3.set_title('Tangent Wave')
ax4 = plt.subplot2grid((3, 3), (1, 1), colspan=2, rowspan=2)
ax4.plot(x, np.exp(x))
ax4.set_title('Exponential Curve')
plt.tight_layout()
plt.show()
在这个例子中,我们通过调整colspan和rowspan参数来设置子图的跨度,从而实现不同大小的子图。
五、总结
在Python中,可以通过Matplotlib库的subplot()函数、GridSpec模块和subplot2grid()函数等多种方式在大图上绘制子图。其中,subplot()函数是最简单和基础的方式,适用于基本的子图布局;GridSpec模块提供了更加灵活的子图布局控制,可以实现复杂的子图排列;subplot2grid()函数则更加直观,适用于需要精确控制子图位置和大小的场景。根据具体需求选择合适的方法,可以更加高效地在大图上绘制子图。
相关问答FAQs:
如何在Python中创建子图以便在大图上进行可视化?
在Python中,可以使用Matplotlib库来创建子图。首先,你需要导入Matplotlib并设置一个大的图形窗口。接着,可以使用plt.subplot()
或者plt.subplots()
方法来定义子图的布局。通过调整参数,可以灵活地控制每个子图的位置和大小,适应不同的显示需求。此外,使用ax
对象来绘制每个子图的内容,确保每个子图的元素清晰可见。
在绘制子图时,如何确保每个子图的可读性?
为了确保子图的可读性,可以考虑以下几点:使用合适的字体大小和样式,确保图例和坐标轴标签不重叠,适当调整子图的间距(使用plt.subplots_adjust()
函数),并选择合适的颜色和样式来区分不同的数据。此外,考虑在每个子图中加入标题,帮助观众快速理解每个部分的内容。
如何在Python中调整子图的大小和比例?
在创建子图时,可以通过figsize
参数来设置整个图形的大小,单位为英寸。例如,plt.figure(figsize=(12, 8))
可以创建一个宽12英寸、高8英寸的画布。在定义子图时,还可以通过gridspec
模块来自定义每个子图的宽高比,进一步调整布局,以实现更好的视觉效果和信息传递。
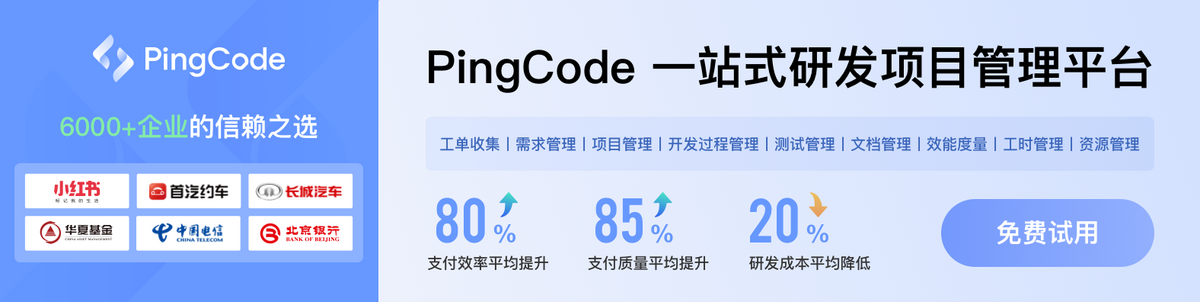