如何将回车符去掉 python:使用strip()方法、使用replace()方法、使用re模块。使用strip()方法是其中一种常用且简单的方法。使用strip()方法可以去掉字符串两端的空白字符,包括回车符和换行符。下面是详细描述:
使用strip()方法是去掉回车符的最简单和直接的方法。strip()方法可以去掉字符串两端的空白字符,包括回车符、换行符、空格等。只需调用该方法即可。例如,对于字符串string = "Hello World\n"
,可以通过调用string.strip()
来去掉末尾的换行符,得到"Hello World"
。这一方法特别适合用于处理从文件中读取的每一行文本,因为文件读取时每一行通常会包含换行符。
一、使用strip()方法去掉回车符
strip()方法是Python内置的字符串方法,能够去除字符串开头和结尾的空白字符,包括空格、换行符(\n)、回车符(\r)等。以下是详细的使用方法:
string_with_newline = "Hello, world!\n"
cleaned_string = string_with_newline.strip()
print(cleaned_string)
在这个例子中,strip()
方法去除了字符串末尾的换行符,得到的cleaned_string
是"Hello, world!"
。如果需要去除特定字符,可以在strip()
方法中传入这些字符。例如:
string_with_newline = "Hello, world!\r\n"
cleaned_string = string_with_newline.strip("\r\n")
print(cleaned_string)
二、使用replace()方法去掉回车符
replace()方法可以替换字符串中的特定子字符串。在处理回车符时,可以使用replace()方法将回车符替换为空字符串。以下是详细的使用方法:
string_with_newline = "Hello, world!\n"
cleaned_string = string_with_newline.replace("\n", "")
print(cleaned_string)
在这个例子中,replace("\n", "")
将字符串中的所有换行符替换为空字符串,从而去掉了换行符。类似地,可以去掉回车符:
string_with_newline = "Hello, world!\r"
cleaned_string = string_with_newline.replace("\r", "")
print(cleaned_string)
三、使用re模块去掉回车符
re模块是Python的正则表达式模块,可以用于复杂的字符串处理任务。使用re模块,可以通过正则表达式去掉回车符。以下是详细的使用方法:
import re
string_with_newline = "Hello, world!\r\n"
cleaned_string = re.sub(r'[\r\n]', '', string_with_newline)
print(cleaned_string)
在这个例子中,re.sub(r'[\r\n]', '', string_with_newline)
使用正则表达式将字符串中的所有回车符和换行符替换为空字符串,从而去掉了这些字符。
四、处理多行文本
在处理多行文本时,上述方法同样适用。可以对每一行使用strip()、replace()或re模块进行处理。以下是详细的示例:
使用strip()方法处理多行文本
multi_line_string = "Hello, world!\nThis is a test.\r\nAnother line."
lines = multi_line_string.splitlines()
cleaned_lines = [line.strip() for line in lines]
cleaned_string = '\n'.join(cleaned_lines)
print(cleaned_string)
在这个例子中,首先使用splitlines()
方法将多行字符串分割成行列表,然后对每一行使用strip()
方法去掉回车符和换行符,最后将处理后的行列表重新组合成单个字符串。
使用replace()方法处理多行文本
multi_line_string = "Hello, world!\nThis is a test.\r\nAnother line."
cleaned_string = multi_line_string.replace("\r", "").replace("\n", "")
print(cleaned_string)
在这个例子中,直接使用replace()
方法将所有的回车符和换行符替换为空字符串。
使用re模块处理多行文本
import re
multi_line_string = "Hello, world!\nThis is a test.\r\nAnother line."
cleaned_string = re.sub(r'[\r\n]', '', multi_line_string)
print(cleaned_string)
在这个例子中,使用re.sub()
方法将所有的回车符和换行符替换为空字符串。
五、读取文件并去掉回车符
在处理从文件中读取的文本时,上述方法同样适用。以下是详细的示例:
使用strip()方法读取文件并去掉回车符
with open('file.txt', 'r') as file:
lines = file.readlines()
cleaned_lines = [line.strip() for line in lines]
cleaned_string = '\n'.join(cleaned_lines)
print(cleaned_string)
在这个例子中,首先使用readlines()
方法读取文件中的所有行,然后对每一行使用strip()
方法去掉回车符和换行符,最后将处理后的行列表重新组合成单个字符串。
使用replace()方法读取文件并去掉回车符
with open('file.txt', 'r') as file:
content = file.read()
cleaned_content = content.replace("\r", "").replace("\n", "")
print(cleaned_content)
在这个例子中,直接使用replace()
方法将文件内容中的所有回车符和换行符替换为空字符串。
使用re模块读取文件并去掉回车符
import re
with open('file.txt', 'r') as file:
content = file.read()
cleaned_content = re.sub(r'[\r\n]', '', content)
print(cleaned_content)
在这个例子中,使用re.sub()
方法将文件内容中的所有回车符和换行符替换为空字符串。
六、处理CSV文件中的回车符
在处理CSV文件时,回车符和换行符可能会对数据的解析造成影响。可以使用上述方法去掉CSV文件中的回车符。以下是详细的示例:
使用strip()方法处理CSV文件中的回车符
import csv
with open('file.csv', 'r') as csvfile:
reader = csv.reader(csvfile)
cleaned_rows = [[cell.strip() for cell in row] for row in reader]
for row in cleaned_rows:
print(row)
在这个例子中,首先使用csv.reader
读取CSV文件中的所有行,然后对每一行中的每一个单元格使用strip()
方法去掉回车符和换行符。
使用replace()方法处理CSV文件中的回车符
import csv
with open('file.csv', 'r') as csvfile:
reader = csv.reader(csvfile)
cleaned_rows = [[cell.replace("\r", "").replace("\n", "") for cell in row] for row in reader]
for row in cleaned_rows:
print(row)
在这个例子中,直接使用replace()
方法将CSV文件中的所有回车符和换行符替换为空字符串。
使用re模块处理CSV文件中的回车符
import csv
import re
with open('file.csv', 'r') as csvfile:
reader = csv.reader(csvfile)
cleaned_rows = [[re.sub(r'[\r\n]', '', cell) for cell in row] for row in reader]
for row in cleaned_rows:
print(row)
在这个例子中,使用re.sub()
方法将CSV文件中的所有回车符和换行符替换为空字符串。
七、使用内置函数与自定义函数结合去掉回车符
有时候,使用内置函数与自定义函数结合可以更灵活地处理回车符。以下是详细的示例:
自定义函数去掉回车符
def remove_carriage_returns(text):
return text.replace("\r", "").replace("\n", "")
string_with_newline = "Hello, world!\r\nThis is a test."
cleaned_string = remove_carriage_returns(string_with_newline)
print(cleaned_string)
在这个例子中,自定义函数remove_carriage_returns
使用replace()
方法去掉回车符和换行符。
自定义函数结合strip()方法去掉回车符
def remove_carriage_returns(text):
lines = text.splitlines()
cleaned_lines = [line.strip() for line in lines]
return '\n'.join(cleaned_lines)
string_with_newline = "Hello, world!\r\nThis is a test."
cleaned_string = remove_carriage_returns(string_with_newline)
print(cleaned_string)
在这个例子中,自定义函数remove_carriage_returns
结合strip()
方法和splitlines()
方法去掉回车符和换行符。
八、处理JSON数据中的回车符
在处理JSON数据时,回车符和换行符可能会对数据的解析造成影响。可以使用上述方法去掉JSON数据中的回车符。以下是详细的示例:
使用strip()方法处理JSON数据中的回车符
import json
json_data = '{"message": "Hello, world!\nThis is a test.\r\nAnother line."}'
parsed_data = json.loads(json_data)
cleaned_data = {key: value.strip() for key, value in parsed_data.items()}
cleaned_json = json.dumps(cleaned_data)
print(cleaned_json)
在这个例子中,首先使用json.loads
解析JSON数据,然后对每一个键值对的值使用strip()
方法去掉回车符和换行符,最后将处理后的数据重新转换为JSON字符串。
使用replace()方法处理JSON数据中的回车符
import json
json_data = '{"message": "Hello, world!\nThis is a test.\r\nAnother line."}'
parsed_data = json.loads(json_data)
cleaned_data = {key: value.replace("\r", "").replace("\n", "") for key, value in parsed_data.items()}
cleaned_json = json.dumps(cleaned_data)
print(cleaned_json)
在这个例子中,直接使用replace()
方法将JSON数据中的所有回车符和换行符替换为空字符串。
使用re模块处理JSON数据中的回车符
import json
import re
json_data = '{"message": "Hello, world!\nThis is a test.\r\nAnother line."}'
parsed_data = json.loads(json_data)
cleaned_data = {key: re.sub(r'[\r\n]', '', value) for key, value in parsed_data.items()}
cleaned_json = json.dumps(cleaned_data)
print(cleaned_json)
在这个例子中,使用re.sub()
方法将JSON数据中的所有回车符和换行符替换为空字符串。
九、总结
去掉回车符是Python字符串处理中的一个常见任务。本文介绍了使用strip()方法、replace()方法和re模块去掉回车符的多种方法,并详细描述了如何处理多行文本、文件、CSV文件和JSON数据中的回车符。根据具体的需求,可以选择适合的方法来处理回车符,从而保证数据的正确性和一致性。
相关问答FAQs:
如何在Python中去除字符串中的换行符?
在Python中,可以使用str.replace()
方法或str.splitlines()
方法来去掉字符串中的换行符。例如,使用replace()
方法可以将换行符替换为空字符串:my_string.replace('\n', '')
。如果想要处理多种换行符,可以使用正则表达式,通过re.sub()
来替换所有换行符。
在Python中去掉回车符会影响字符串的其他部分吗?
去掉回车符只会影响字符串中换行符的存在,不会对其他字符产生影响。字符串的其他内容将保持不变,所以你可以放心地去除不需要的换行符,而不会改变其他数据。
有没有其他方法可以清理字符串中的空白字符?
除了去除换行符,还有其他方法可以清理字符串中的空白字符,比如使用str.strip()
方法去除字符串两端的空白,或者使用str.split()
方法将字符串分割成列表,去除所有空白字符。对于更复杂的情况,可以使用正则表达式的re.sub()
来匹配并替换特定的空白字符。
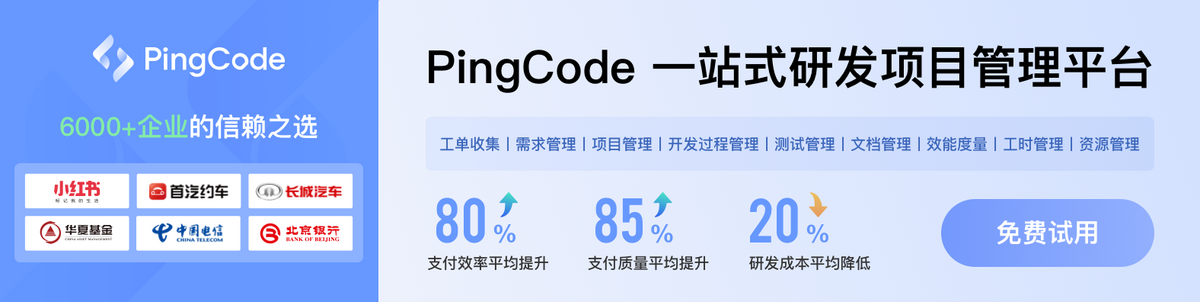