用Python爬取公司名单的方法有很多种,包括使用BeautifulSoup、Scrapy、Selenium等技术。首先,选择一个数据源网站,通过发送HTTP请求获取网页内容,解析内容提取公司名单,存储数据。关键步骤包括选择合适的网站、发送请求、解析数据、存储数据。以下是详细的步骤介绍。
一、选择数据源网站
选择一个包含公司名单的可靠网站是爬取数据的第一步。常见的选择包括商业目录网站、行业协会网站和政府注册公司数据库等。在选择网站时,需要注意网站的反爬策略和数据使用条款,确保合法合规。
寻找合适的网站
寻找合适的网站是爬取数据的第一步。你可以使用Google等搜索引擎查找包含公司名单的网站。例如,可以搜索“公司名单目录”或“商业目录”。找到合适的网站后,检查网站的结构和内容,确定是否适合爬取。
网站的反爬策略
许多网站都有反爬虫机制,以防止过多的自动化请求。常见的反爬策略包括限制请求频率、IP封禁、验证码等。在开始爬取数据之前,需要了解目标网站的反爬策略,并采取相应的措施。例如,可以使用代理IP轮换、设置合理的请求间隔等。
二、发送HTTP请求获取网页内容
使用Python的requests库发送HTTP请求,获取网页的HTML内容。
import requests
url = "https://example.com/companies"
response = requests.get(url)
检查请求是否成功
if response.status_code == 200:
html_content = response.text
else:
print(f"Failed to retrieve content, status code: {response.status_code}")
处理请求失败的情况
在实际操作中,请求可能会失败,如网络问题、目标网站禁止访问等。处理请求失败的情况非常重要,以确保爬虫的稳定性。可以使用try-except块捕获异常,并设置重试机制。
import requests
from requests.exceptions import RequestException
url = "https://example.com/companies"
def fetch_html(url):
try:
response = requests.get(url)
response.raise_for_status()
return response.text
except RequestException as e:
print(f"Error fetching {url}: {e}")
return None
html_content = fetch_html(url)
if html_content:
# 继续处理html_content
pass
三、解析网页内容提取公司名单
使用BeautifulSoup库解析HTML内容,提取公司名单。首先,安装BeautifulSoup库:
pip install beautifulsoup4
使用BeautifulSoup解析HTML
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'html.parser')
company_list = []
假设公司名称在class为company-name的标签中
for company in soup.find_all(class_='company-name'):
company_name = company.get_text(strip=True)
company_list.append(company_name)
print(company_list)
处理动态内容
有些网站使用JavaScript动态生成内容,直接获取HTML可能无法获得需要的数据。这时可以使用Selenium库模拟浏览器操作,加载动态内容。
首先,安装Selenium和浏览器驱动(如ChromeDriver):
pip install selenium
使用Selenium获取动态内容
from selenium import webdriver
from selenium.webdriver.chrome.service import Service as ChromeService
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
设置ChromeDriver路径
chrome_service = ChromeService(executable_path='/path/to/chromedriver')
启动浏览器
driver = webdriver.Chrome(service=chrome_service)
driver.get("https://example.com/companies")
等待页面加载完成
wait = WebDriverWait(driver, 10)
wait.until(EC.presence_of_element_located((By.CLASS_NAME, 'company-name')))
获取页面内容
html_content = driver.page_source
driver.quit()
解析HTML内容
soup = BeautifulSoup(html_content, 'html.parser')
company_list = []
for company in soup.find_all(class_='company-name'):
company_name = company.get_text(strip=True)
company_list.append(company_name)
print(company_list)
四、存储数据
将提取的公司名单存储到文件或数据库中。常见的存储方式包括CSV文件、JSON文件、SQLite数据库等。
存储到CSV文件
import csv
with open('companies.csv', 'w', newline='', encoding='utf-8') as csvfile:
writer = csv.writer(csvfile)
writer.writerow(['Company Name'])
for company_name in company_list:
writer.writerow([company_name])
存储到JSON文件
import json
with open('companies.json', 'w', encoding='utf-8') as jsonfile:
json.dump(company_list, jsonfile, ensure_ascii=False, indent=4)
存储到SQLite数据库
首先,安装SQLite库:
pip install sqlite3
import sqlite3
conn = sqlite3.connect('companies.db')
c = conn.cursor()
创建表
c.execute('''
CREATE TABLE IF NOT EXISTS companies (
id INTEGER PRIMARY KEY,
name TEXT NOT NULL
)
''')
插入数据
for company_name in company_list:
c.execute('INSERT INTO companies (name) VALUES (?)', (company_name,))
conn.commit()
conn.close()
五、处理大规模数据爬取
在爬取大量数据时,需要考虑爬虫的效率和稳定性。可以使用多线程或多进程技术加速爬取过程,使用代理IP防止被封禁,以及对数据进行去重和清洗。
使用多线程爬取
import threading
def fetch_company_names(url):
html_content = fetch_html(url)
if html_content:
soup = BeautifulSoup(html_content, 'html.parser')
for company in soup.find_all(class_='company-name'):
company_name = company.get_text(strip=True)
company_list.append(company_name)
urls = ["https://example.com/companies?page=1", "https://example.com/companies?page=2", ...]
threads = []
for url in urls:
thread = threading.Thread(target=fetch_company_names, args=(url,))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
print(company_list)
使用代理IP
proxies = {
'http': 'http://your_proxy_ip:port',
'https': 'https://your_proxy_ip:port'
}
def fetch_html(url):
try:
response = requests.get(url, proxies=proxies)
response.raise_for_status()
return response.text
except RequestException as e:
print(f"Error fetching {url}: {e}")
return None
数据去重和清洗
爬取的数据可能包含重复项或格式不一致的情况,需要进行去重和清洗。
cleaned_company_list = list(set(company_list))
cleaned_company_list = [name.strip() for name in cleaned_company_list if name.strip()]
print(cleaned_company_list)
六、定期更新数据
爬取的数据可能会随着时间变化,需要定期更新。可以设置定时任务,定期运行爬虫,获取最新的公司名单。
使用cron定时任务(Linux)
编辑crontab文件:
crontab -e
添加定时任务,每天凌晨1点运行爬虫脚本:
0 1 * * * /usr/bin/python3 /path/to/your_script.py
总结
使用Python爬取公司名单涉及选择数据源网站、发送HTTP请求获取网页内容、解析数据、存储数据和处理大规模数据爬取等步骤。通过合理选择工具和技术,可以高效地获取和管理公司名单数据。
相关问答FAQs:
如何选择合适的网站进行公司名单爬取?
在进行公司名单爬取时,选择合适的网站至关重要。首先,你可以考虑行业相关的商业目录、黄页或者公司注册信息网站。这些网站通常包含结构化的数据,便于提取。确保你遵守网站的使用条款,了解相关法律法规,以免侵犯版权或违反爬虫政策。
爬取公司名单时应该使用哪些Python库?
使用Python进行爬虫时,有几个常用的库可以帮助你高效地完成任务。Requests
库用于发送HTTP请求,BeautifulSoup
用于解析HTML和XML文档,Scrapy
则是一个强大的爬虫框架,适合大规模数据抓取。此外,Pandas
库可以帮助你整理和存储爬取的数据,方便后续分析。
如何处理爬取过程中遇到的反爬虫机制?
许多网站为了保护其数据,实施了反爬虫机制。如果在爬取过程中遇到限制,可以尝试使用代理服务器以更换IP地址,或使用延时策略来减缓请求频率,降低被检测的风险。此外,可以通过修改请求头信息,使其看起来像是来自浏览器的正常请求,从而减少被封禁的可能性。
爬取到的公司名单如何进行数据清洗和存储?
在爬取到公司名单后,数据清洗是非常重要的一步。可以使用Pandas
库对数据进行去重、填补缺失值和格式化处理。清洗后的数据可以存储为CSV文件、Excel文档或者数据库,以便后续的分析和使用。确保数据的准确性和完整性,以提高后续工作的效率。
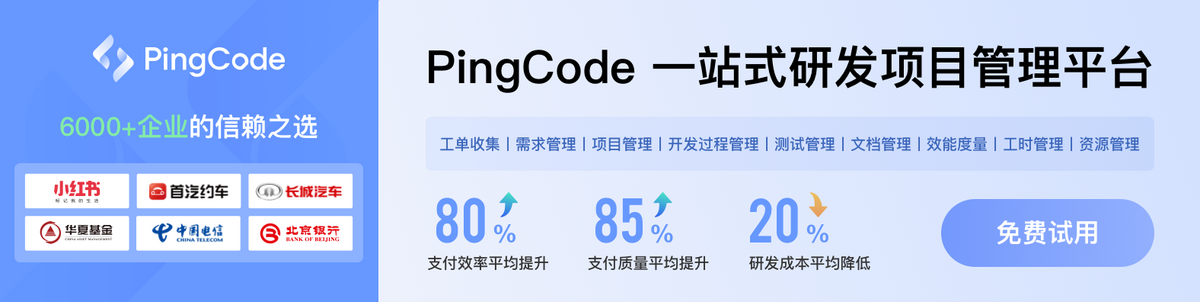