Python中检查字符串的方法有很多,主要包括:使用in
关键字、find()
方法、index()
方法、正则表达式、startswith()
和endswith()
方法、isalpha()
、isdigit()
等方法。 其中,使用in
关键字是最为常用的一种方法,因为它简洁且高效。下面详细展开说明如何使用这些方法。
一、使用in
关键字
in
关键字是Python中最简洁的检查字符串的方法之一。它用于检查一个字符串是否包含在另一个字符串中。如果包含,则返回True,否则返回False。例如:
text = "Hello, world!"
if "world" in text:
print("Substring found!")
else:
print("Substring not found!")
详细描述:
使用in
关键字的好处在于其简洁性和可读性。它使代码更加直观,易于理解。此外,in
关键字的性能也相对较高,适合在需要频繁进行字符串检查的场景中使用。
二、使用find()
方法
find()
方法用于返回子字符串在字符串中首次出现的位置。如果子字符串不在字符串中,则返回-1。例如:
text = "Hello, world!"
position = text.find("world")
if position != -1:
print(f"Substring found at position {position}")
else:
print("Substring not found!")
find()
方法的优点在于它不仅可以检查子字符串是否存在,还可以告诉你它的位置,这在某些应用场景中非常有用。
三、使用index()
方法
index()
方法与find()
方法类似,但不同的是,如果子字符串不在字符串中,index()
方法会抛出一个ValueError
异常。例如:
text = "Hello, world!"
try:
position = text.index("world")
print(f"Substring found at position {position}")
except ValueError:
print("Substring not found!")
index()
方法的优点在于可以通过异常处理机制捕获子字符串不存在的情况,这在需要对不存在情况进行特殊处理的场景中非常有用。
四、使用正则表达式
Python的re
模块提供了强大的正则表达式功能,可以用于复杂的字符串检查。例如,检查字符串是否包含数字:
import re
text = "Hello, world! 123"
if re.search(r'\d', text):
print("String contains a number!")
else:
print("String does not contain a number!")
正则表达式的优点在于其灵活性和强大功能,可以处理复杂的字符串模式匹配任务。
五、使用startswith()
和endswith()
方法
startswith()
和endswith()
方法分别用于检查字符串是否以特定的子字符串开头或结尾。例如:
text = "Hello, world!"
if text.startswith("Hello"):
print("String starts with 'Hello'")
if text.endswith("world!"):
print("String ends with 'world!'")
这两个方法的优点在于它们专门用于检查字符串的起始和结尾,简洁且高效。
六、使用isalpha()
、isdigit()
等方法
Python还提供了一些内置的方法用于检查字符串的特定特性,例如,isalpha()
检查字符串是否只包含字母,isdigit()
检查字符串是否只包含数字。例如:
text = "Hello"
if text.isalpha():
print("String contains only letters!")
else:
print("String contains non-letter characters!")
number = "12345"
if number.isdigit():
print("String contains only digits!")
else:
print("String contains non-digit characters!")
这些方法的优点在于它们专门用于特定类型的检查,简洁且高效。
七、使用any()
和all()
结合字符串方法
有时需要检查字符串中是否包含特定集合的字符,可以结合any()
和all()
函数实现。例如,检查字符串中是否包含任意一个数字:
text = "Hello, world! 123"
if any(char.isdigit() for char in text):
print("String contains a digit!")
else:
print("String does not contain a digit!")
或者检查字符串是否只包含字母和数字:
text = "Hello123"
if all(char.isalnum() for char in text):
print("String contains only letters and digits!")
else:
print("String contains special characters!")
八、使用count()
方法
count()
方法用于返回子字符串在字符串中出现的次数,如果为0则表示子字符串不在字符串中。例如:
text = "Hello, world! Hello again!"
count = text.count("Hello")
if count > 0:
print(f"Substring found {count} times!")
else:
print("Substring not found!")
count()
方法的优点在于它不仅可以检查子字符串是否存在,还可以统计子字符串出现的次数,这在某些统计分析场景中非常有用。
九、使用字符串切片
通过字符串切片也可以实现一些检查功能,例如检查字符串的特定部分是否包含子字符串:
text = "Hello, world!"
if "world" in text[7:12]:
print("Substring found in the specified range!")
else:
print("Substring not found in the specified range!")
字符串切片的优点在于它可以灵活地检查字符串的特定部分,适用于需要对字符串进行局部检查的场景。
十、使用partition()
方法
partition()
方法用于将字符串分割成三个部分:分隔符前的部分、分隔符本身、分隔符后的部分。通过检查分隔符是否存在,可以实现字符串检查。例如:
text = "Hello, world!"
before, sep, after = text.partition("world")
if sep:
print("Substring found!")
else:
print("Substring not found!")
partition()
方法的优点在于它可以在检查字符串的同时将字符串分割成多个部分,适用于需要对字符串进行分割和检查的场景。
十一、使用split()
方法
split()
方法用于将字符串分割成多个子字符串,然后可以检查这些子字符串是否包含特定的子字符串。例如:
text = "Hello, world! How are you?"
parts = text.split()
if "world!" in parts:
print("Substring found in the split parts!")
else:
print("Substring not found in the split parts!")
split()
方法的优点在于它可以将字符串分割成多个部分,然后对这些部分进行检查,适用于需要对字符串进行分割和检查的场景。
十二、使用replace()
方法
replace()
方法用于将字符串中的子字符串替换为另一个子字符串。通过检查替换前后的字符串是否相同,可以实现字符串检查。例如:
text = "Hello, world!"
new_text = text.replace("world", "")
if text != new_text:
print("Substring found and removed!")
else:
print("Substring not found!")
replace()
方法的优点在于它可以在检查字符串的同时对字符串进行替换,适用于需要对字符串进行替换和检查的场景。
十三、使用join()
方法
join()
方法通常用于将多个字符串连接成一个字符串,但也可以用于检查字符串。例如,通过join()
方法将字符串分割成多个部分,然后检查这些部分是否包含特定的子字符串:
text = "Hello, world! How are you?"
parts = text.split()
joined_text = " ".join(parts)
if "world!" in joined_text:
print("Substring found in the joined text!")
else:
print("Substring not found in the joined text!")
join()
方法的优点在于它可以将多个字符串连接成一个字符串,然后对这个字符串进行检查,适用于需要对多个字符串进行连接和检查的场景。
十四、使用strip()
方法
strip()
方法用于移除字符串两端的空白字符,通过检查移除前后的字符串是否相同,可以实现字符串检查。例如:
text = " Hello, world! "
stripped_text = text.strip()
if text != stripped_text:
print("String contains leading or trailing spaces!")
else:
print("String does not contain leading or trailing spaces!")
strip()
方法的优点在于它可以在检查字符串的同时移除字符串两端的空白字符,适用于需要对字符串进行清理和检查的场景。
十五、使用rfind()
方法
rfind()
方法与find()
方法类似,但不同的是,rfind()
方法从右向左查找子字符串的位置。例如:
text = "Hello, world! Hello again!"
position = text.rfind("Hello")
if position != -1:
print(f"Substring found at position {position}")
else:
print("Substring not found!")
rfind()
方法的优点在于它可以从右向左查找子字符串的位置,适用于需要从字符串末尾开始查找的场景。
十六、使用rindex()
方法
rindex()
方法与index()
方法类似,但不同的是,rindex()
方法从右向左查找子字符串的位置。如果子字符串不在字符串中,rindex()
方法会抛出一个ValueError
异常。例如:
text = "Hello, world! Hello again!"
try:
position = text.rindex("Hello")
print(f"Substring found at position {position}")
except ValueError:
print("Substring not found!")
rindex()
方法的优点在于它可以通过异常处理机制从右向左查找子字符串的位置,适用于需要从字符串末尾开始查找并处理异常的场景。
十七、使用format()
方法
format()
方法通常用于格式化字符串,但也可以用于检查字符串。例如,通过format()
方法将字符串格式化为特定的格式,然后检查格式化后的字符串是否包含特定的子字符串:
template = "Hello, {}!"
formatted_text = template.format("world")
if "world" in formatted_text:
print("Substring found in the formatted text!")
else:
print("Substring not found in the formatted text!")
format()
方法的优点在于它可以在检查字符串的同时对字符串进行格式化,适用于需要对字符串进行格式化和检查的场景。
十八、使用lower()
和upper()
方法
lower()
和upper()
方法用于将字符串转换为小写或大写,通过比较转换前后的字符串,可以实现字符串检查。例如:
text = "Hello, World!"
lower_text = text.lower()
if text != lower_text:
print("String contains uppercase letters!")
else:
print("String does not contain uppercase letters!")
lower()
和upper()
方法的优点在于它们可以在检查字符串的同时将字符串转换为小写或大写,适用于需要对字符串进行大小写转换和检查的场景。
十九、使用isalnum()
方法
isalnum()
方法用于检查字符串是否只包含字母和数字。例如:
text = "Hello123"
if text.isalnum():
print("String contains only letters and digits!")
else:
print("String contains special characters!")
isalnum()
方法的优点在于它可以专门用于检查字符串是否只包含字母和数字,适用于需要对字符串进行字母和数字检查的场景。
二十、使用isspace()
方法
isspace()
方法用于检查字符串是否只包含空白字符。例如:
text = " "
if text.isspace():
print("String contains only spaces!")
else:
print("String contains non-space characters!")
isspace()
方法的优点在于它可以专门用于检查字符串是否只包含空白字符,适用于需要对字符串进行空白字符检查的场景。
通过这些方法,您可以灵活地在Python中检查字符串,根据具体需求选择最合适的方法可以使代码更加简洁、高效和可读。
相关问答FAQs:
如何在Python中判断字符串是否为空?
在Python中,可以使用简单的条件语句来检查字符串是否为空。通过直接使用if
语句来判断字符串的真值,如果字符串为空,条件会返回False
。例如:
my_string = ""
if not my_string:
print("字符串为空")
上述代码会输出“字符串为空”,因为my_string
没有内容。
Python中有哪些方法可以检查字符串是否包含特定子串?
在Python中,可以使用in
关键字来检查字符串中是否包含某个子串。例如:
text = "Hello, world!"
if "world" in text:
print("字符串中包含 'world'")
该代码段会输出“字符串中包含 'world'”,因为“world”确实出现在text
中。此外,还可以使用str.find()
或str.index()
方法来获取子串的位置,如果返回值为-1,表示子串不存在。
怎样在Python中检查字符串的格式或类型?
对于字符串格式的检查,Python提供了多种内建方法。例如,使用str.isdigit()
可以判断字符串是否仅由数字组成,使用str.isalpha()
可以检查字符串是否仅由字母构成。以下是一个示例:
my_string = "12345"
if my_string.isdigit():
print("字符串仅包含数字")
这段代码会输出“字符串仅包含数字”。这些方法可以帮助开发者在处理用户输入时确保数据的有效性。
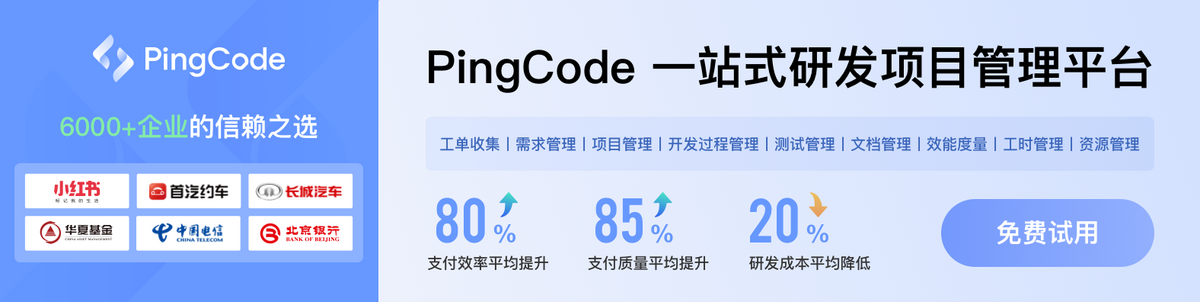