Python读取txt文件中指定内容的方法主要包括:使用文件操作函数、正则表达式、文本解析库等。 其中,使用文件操作函数是最基础且常用的方法,通过读取文件内容并进行字符串操作,可以实现对指定内容的提取。下面将详细介绍这种方法,并涵盖其他进阶方法。
一、文件操作函数
- 读取整个文件内容并查找
这种方法适用于文件内容较小的情况,可以一次性将整个文件内容读取到内存中,然后通过字符串操作查找指定内容。
def read_specific_content(file_path, keyword):
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
if keyword in content:
print(f"Keyword '{keyword}' found in file.")
else:
print(f"Keyword '{keyword}' not found in file.")
这种方法的优点是简单直接,但对于大文件来说,可能会占用大量内存。
- 逐行读取文件内容并查找
对于大文件,逐行读取是一种更好的选择,可以避免将整个文件内容读入内存。
def read_specific_content_line_by_line(file_path, keyword):
with open(file_path, 'r', encoding='utf-8') as file:
for line in file:
if keyword in line:
print(f"Keyword '{keyword}' found in line: {line.strip()}")
逐行读取可以有效节省内存,但需要注意处理文件指针的移动和关闭文件资源。
二、正则表达式
正则表达式是一种强大的文本处理工具,适用于从文件中提取复杂模式的内容。
- 使用正则表达式查找指定内容
import re
def read_specific_content_with_regex(file_path, pattern):
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
matches = re.findall(pattern, content)
if matches:
print(f"Pattern '{pattern}' found in file: {matches}")
else:
print(f"Pattern '{pattern}' not found in file.")
正则表达式可以实现灵活多样的匹配,但需要掌握一定的正则表达式语法。
三、文本解析库
对于特定格式的文件,如JSON、XML、CSV等,可以使用相应的解析库进行读取和提取。
- 读取JSON文件
import json
def read_specific_content_from_json(file_path, key):
with open(file_path, 'r', encoding='utf-8') as file:
data = json.load(file)
if key in data:
print(f"Key '{key}' found in JSON file: {data[key]}")
else:
print(f"Key '{key}' not found in JSON file.")
- 读取CSV文件
import csv
def read_specific_content_from_csv(file_path, keyword):
with open(file_path, 'r', encoding='utf-8') as file:
reader = csv.reader(file)
for row in reader:
if keyword in row:
print(f"Keyword '{keyword}' found in row: {row}")
- 读取XML文件
import xml.etree.ElementTree as ET
def read_specific_content_from_xml(file_path, tag):
tree = ET.parse(file_path)
root = tree.getroot()
for elem in root.iter(tag):
print(f"Tag '{tag}' found in XML file: {elem.text}")
四、结合使用多种方法
在实际应用中,可能需要结合多种方法来实现复杂的文件读取和内容提取需求。
- 结合文件操作和正则表达式
import re
def read_and_extract_with_combination(file_path, pattern):
with open(file_path, 'r', encoding='utf-8') as file:
for line in file:
matches = re.findall(pattern, line)
if matches:
print(f"Pattern '{pattern}' found in line: {line.strip()}")
- 结合文本解析和文件操作
例如,读取一个包含多种格式数据的文件,可以先逐行读取,再根据行内容选择合适的解析方法。
import json
import csv
import xml.etree.ElementTree as ET
def read_combined_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
for line in file:
if line.startswith('{'):
data = json.loads(line)
print(f"JSON data: {data}")
elif line.startswith('<'):
root = ET.fromstring(line)
print(f"XML data: {ET.tostring(root, encoding='unicode')}")
else:
reader = csv.reader([line])
for row in reader:
print(f"CSV data: {row}")
五、常见问题和解决方法
- 文件编码问题
读取文件时,可能会遇到编码问题,导致读取失败或内容乱码。可以通过指定编码参数解决。
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
- 文件不存在或无法访问
需要处理文件不存在或无法访问的情况,避免程序崩溃。
import os
file_path = 'example.txt'
if os.path.exists(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
else:
print(f"File '{file_path}' does not exist.")
- 文件读取性能优化
对于大文件,可以使用文件的内存映射(mmap)提高读取性能。
import mmap
def read_large_file_with_mmap(file_path, keyword):
with open(file_path, 'r+b') as file:
mmapped_file = mmap.mmap(file.fileno(), 0)
if keyword.encode('utf-8') in mmapped_file:
print(f"Keyword '{keyword}' found in file.")
mmapped_file.close()
通过上述方法,可以有效地读取txt文件中的指定内容,并根据实际需求选择合适的方法进行优化和扩展。无论是简单的关键词查找,还是复杂的模式匹配和数据解析,Python都提供了丰富的工具和库支持。
相关问答FAQs:
如何在Python中读取特定行的内容?
要读取txt文件中特定行的内容,可以使用Python的文件操作功能。首先打开文件,然后使用readlines()
方法将所有行读入一个列表。接着,可以通过索引访问特定行。例如,lines[2]
将返回第三行的内容。确保处理索引越界的情况,以免程序崩溃。
Python读取txt文件时,如何处理编码问题?
在读取txt文件时,编码问题可能会影响内容的正确显示。使用open()
函数时,可以指定encoding
参数,例如open('file.txt', 'r', encoding='utf-8')
。如果文件采用了其他编码格式,如latin1
或gbk
,请相应调整编码参数。这样可以确保读取的内容不会出现乱码。
有没有办法根据关键字从txt文件中筛选内容?
可以使用Python的字符串方法来筛选包含特定关键字的行。通过遍历文件的每一行,并使用if keyword in line
来检查关键字是否存在。如果存在,可以将该行存储到一个新的列表中或直接输出。这样便可轻松获取包含所需关键字的所有内容。
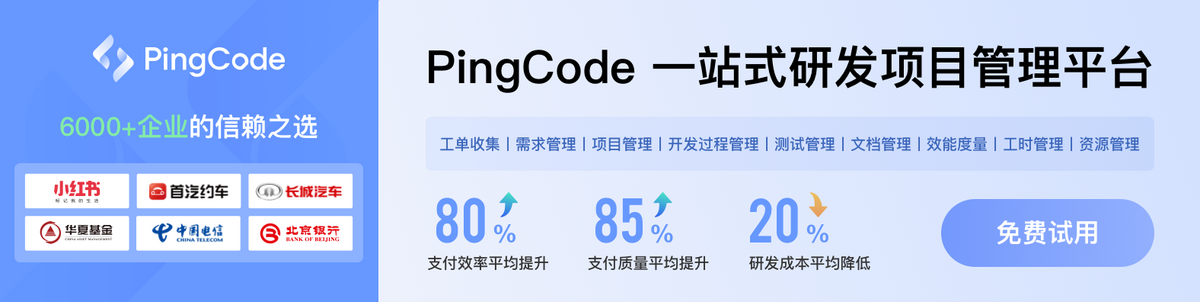