在Python中,可以使用Matplotlib库来绘制平面的反弹线。 Matplotlib是一个广泛使用的2D绘图库,能够生成各种图表和图形。绘制平面的反弹线涉及到物体碰撞边界后反弹的模拟,通常需要考虑物体的初始位置、速度、方向以及平面的边界条件。接下来,我将详细介绍如何使用Python和Matplotlib来绘制平面的反弹线。
一、安装和导入必要的库
在开始之前,请确保你已经安装了Matplotlib库。如果还没有安装,可以使用以下命令进行安装:
pip install matplotlib
导入Matplotlib库和其他可能需要的库:
import matplotlib.pyplot as plt
import numpy as np
二、定义反弹的物理模型
为了模拟平面的反弹线,我们需要定义一些物理模型的参数,包括物体的初始位置、速度、反弹条件等。
- 初始位置和速度:假设物体从二维平面的某个初始位置出发,具有初始速度和方向。
- 边界条件:定义平面的边界条件,当物体碰到边界时,计算反弹后的方向和速度。
- 时间步长:模拟过程中的时间步长,用于计算物体在每个时间步长内的位置变化。
三、实现反弹模型的函数
定义一个函数,用于计算物体在平面内的运动和反弹过程。
def simulate_bounce(initial_position, initial_velocity, bounds, time_steps):
"""
Simulate the bouncing of an object in a 2D plane.
Parameters:
- initial_position: tuple, (x, y) coordinates of the initial position.
- initial_velocity: tuple, (vx, vy) initial velocity components.
- bounds: tuple, (xmin, xmax, ymin, ymax) boundaries of the plane.
- time_steps: int, number of time steps to simulate.
Returns:
- positions: list of (x, y) tuples representing the object's positions over time.
"""
x, y = initial_position
vx, vy = initial_velocity
xmin, xmax, ymin, ymax = bounds
positions = [(x, y)]
for _ in range(time_steps):
# Update position
x += vx
y += vy
# Check for collision with boundaries and update velocity
if x <= xmin or x >= xmax:
vx = -vx # Reverse x velocity
if y <= ymin or y >= ymax:
vy = -vy # Reverse y velocity
# Append new position
positions.append((x, y))
return positions
四、绘制反弹线
使用Matplotlib绘制模拟过程中物体的运动轨迹。
def plot_bounce(positions, bounds):
"""
Plot the bouncing object positions using Matplotlib.
Parameters:
- positions: list of (x, y) tuples representing the object's positions over time.
- bounds: tuple, (xmin, xmax, ymin, ymax) boundaries of the plane.
"""
xs, ys = zip(*positions)
plt.figure(figsize=(10, 6))
plt.plot(xs, ys, marker='o', linestyle='-', color='b')
plt.xlim(bounds[0], bounds[1])
plt.ylim(bounds[2], bounds[3])
plt.title('2D Plane Bounce Simulation')
plt.xlabel('X Position')
plt.ylabel('Y Position')
plt.grid(True)
plt.show()
五、运行模拟和绘制图形
定义初始条件并运行模拟,然后绘制结果。
# Initial conditions
initial_position = (1, 1)
initial_velocity = (0.2, 0.3)
bounds = (0, 10, 0, 10)
time_steps = 100
Run simulation
positions = simulate_bounce(initial_position, initial_velocity, bounds, time_steps)
Plot results
plot_bounce(positions, bounds)
结论
通过上述步骤,我们已经成功地使用Python和Matplotlib绘制了一个平面内物体反弹线的模拟图。关键步骤包括定义物理模型、编写模拟函数、绘制图形。这种方法不仅可以用于简单的二维反弹模拟,还可以扩展到更复杂的物理系统和三维空间。通过不断调整初始条件和边界条件,你可以探索不同的反弹行为和轨迹,深入理解物理模拟的原理。
相关问答FAQs:
如何在Python中实现反弹线的绘制?
在Python中,可以使用Matplotlib库来绘制平面的反弹线。首先需要安装Matplotlib库,使用pip install matplotlib
命令。接着,创建一个图形窗口,并定义反弹线的起点和反弹角度,利用数学函数计算路径,最后使用plt.plot()
函数将路径绘制出来。
反弹线的绘制需要哪些数学基础?
绘制反弹线需要对几何和三角函数有一定的理解。具体来说,需要知道如何计算反弹角度,以及如何使用斜率公式来确定线段的方向。了解反射定律,即入射角等于反射角,将帮助更好地在平面上模拟反弹现象。
是否可以在Python中添加动画效果来展示反弹线的动态过程?
是的,Python的Matplotlib库支持动画效果。可以使用FuncAnimation
类来创建动态展示。通过设置更新函数和动画参数,能够实现反弹线在绘制过程中的逐步展示,从而更加生动地表现反弹的效果。
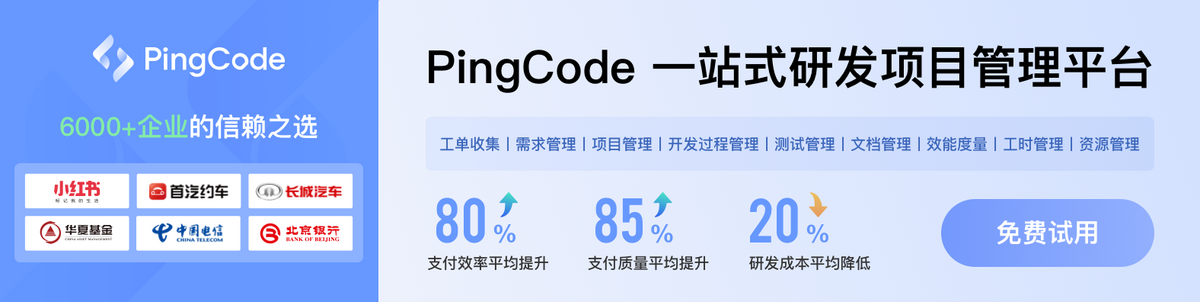