Python查询数据库非空的方法有多种,主要包括以下几个步骤:连接数据库、执行查询语句、处理结果集。为了详细介绍其中一个方法,我们将结合MySQL数据库和Python的MySQL连接库 mysql-connector-python
来演示具体操作。
一、连接数据库
要想查询数据库中的表是否为空,首先需要建立与数据库的连接。Python提供了多种连接数据库的库,比如 mysql-connector-python
, pymysql
, sqlalchemy
等。这里我们使用 mysql-connector-python
。
import mysql.connector
def create_connection(host_name, user_name, user_password, db_name):
connection = None
try:
connection = mysql.connector.connect(
host=host_name,
user=user_name,
passwd=user_password,
database=db_name
)
print("Connection to MySQL DB successful")
except mysql.connector.Error as e:
print(f"The error '{e}' occurred")
return connection
二、执行查询语句
连接建立成功后,接下来需要执行查询语句来判断表是否为空。可以通过 SELECT COUNT(*)
来获取表中的记录数,如果返回值为0,则表为空。
def is_table_empty(connection, table_name):
cursor = connection.cursor()
cursor.execute(f"SELECT COUNT(*) FROM {table_name}")
result = cursor.fetchone()
return result[0] == 0
三、处理结果集
根据查询结果集中的记录数,我们可以判断表是否为空,并据此做出相应的操作。
def main():
connection = create_connection("localhost", "root", "password", "database_name")
table_name = "your_table_name"
if is_table_empty(connection, table_name):
print(f"The table {table_name} is empty.")
else:
print(f"The table {table_name} is not empty.")
四、使用小标题将文章进行分段
一、连接数据库
为了查询数据库中的表是否为空,首先需要连接到数据库。Python提供了多种与MySQL数据库连接的库,其中 mysql-connector-python
是较为常用的一种。下面是一个简单的示例代码,用于建立与MySQL数据库的连接:
import mysql.connector
def create_connection(host_name, user_name, user_password, db_name):
connection = None
try:
connection = mysql.connector.connect(
host=host_name,
user=user_name,
passwd=user_password,
database=db_name
)
print("Connection to MySQL DB successful")
except mysql.connector.Error as e:
print(f"The error '{e}' occurred")
return connection
该函数 create_connection
接受四个参数:数据库主机名、用户名、密码和数据库名,并返回一个数据库连接对象。如果连接成功,打印成功信息;如果连接失败,打印错误信息。
二、执行查询语句
建立数据库连接后,下一步是执行查询语句来判断表是否为空。可以通过执行 SELECT COUNT(*)
来获取表中的记录数。如果记录数为0,则表为空。下面是一个示例代码:
def is_table_empty(connection, table_name):
cursor = connection.cursor()
cursor.execute(f"SELECT COUNT(*) FROM {table_name}")
result = cursor.fetchone()
return result[0] == 0
在这个函数中,我们通过 cursor.execute
执行查询语句,并使用 cursor.fetchone
获取查询结果。如果返回的记录数为0,表示表为空;否则,表示表不为空。
三、处理结果集
根据查询结果集中的记录数,我们可以判断表是否为空,并据此做出相应的操作。下面是一个示例代码,用于根据查询结果判断表是否为空:
def main():
connection = create_connection("localhost", "root", "password", "database_name")
table_name = "your_table_name"
if is_table_empty(connection, table_name):
print(f"The table {table_name} is empty.")
else:
print(f"The table {table_name} is not empty.")
在这个主函数中,我们首先建立与数据库的连接,然后调用 is_table_empty
函数判断表是否为空,并根据结果打印相应的消息。
四、处理多种数据库
除了MySQL数据库,Python还可以连接其他类型的数据库,例如PostgreSQL、SQLite、SQL Server等。对于不同类型的数据库,可以使用相应的连接库来实现连接和查询。下面是一些常用的数据库连接库及其示例代码:
1. PostgreSQL
使用 psycopg2
库连接PostgreSQL数据库并查询表是否为空:
import psycopg2
def create_connection_pg(host_name, user_name, user_password, db_name):
connection = None
try:
connection = psycopg2.connect(
host=host_name,
user=user_name,
password=user_password,
database=db_name
)
print("Connection to PostgreSQL DB successful")
except psycopg2.Error as e:
print(f"The error '{e}' occurred")
return connection
def is_table_empty_pg(connection, table_name):
cursor = connection.cursor()
cursor.execute(f"SELECT COUNT(*) FROM {table_name}")
result = cursor.fetchone()
return result[0] == 0
def main_pg():
connection = create_connection_pg("localhost", "postgres", "password", "database_name")
table_name = "your_table_name"
if is_table_empty_pg(connection, table_name):
print(f"The table {table_name} is empty.")
else:
print(f"The table {table_name} is not empty.")
2. SQLite
使用 sqlite3
库连接SQLite数据库并查询表是否为空:
import sqlite3
def create_connection_sqlite(db_file):
connection = None
try:
connection = sqlite3.connect(db_file)
print("Connection to SQLite DB successful")
except sqlite3.Error as e:
print(f"The error '{e}' occurred")
return connection
def is_table_empty_sqlite(connection, table_name):
cursor = connection.cursor()
cursor.execute(f"SELECT COUNT(*) FROM {table_name}")
result = cursor.fetchone()
return result[0] == 0
def main_sqlite():
connection = create_connection_sqlite("database.db")
table_name = "your_table_name"
if is_table_empty_sqlite(connection, table_name):
print(f"The table {table_name} is empty.")
else:
print(f"The table {table_name} is not empty.")
3. SQL Server
使用 pyodbc
库连接SQL Server数据库并查询表是否为空:
import pyodbc
def create_connection_sqlserver(server_name, db_name, user_name, user_password):
connection = None
try:
connection = pyodbc.connect(
'DRIVER={ODBC Driver 17 for SQL Server};'
f'SERVER={server_name};'
f'DATABASE={db_name};'
f'UID={user_name};'
f'PWD={user_password}'
)
print("Connection to SQL Server DB successful")
except pyodbc.Error as e:
print(f"The error '{e}' occurred")
return connection
def is_table_empty_sqlserver(connection, table_name):
cursor = connection.cursor()
cursor.execute(f"SELECT COUNT(*) FROM {table_name}")
result = cursor.fetchone()
return result[0] == 0
def main_sqlserver():
connection = create_connection_sqlserver("server_name", "database_name", "username", "password")
table_name = "your_table_name"
if is_table_empty_sqlserver(connection, table_name):
print(f"The table {table_name} is empty.")
else:
print(f"The table {table_name} is not empty.")
五、注意事项
在实际应用中,查询数据库表是否为空时需要注意以下几点:
- SQL注入风险:在构建SQL查询语句时,应避免直接使用用户输入的值,以防SQL注入攻击。可以使用参数化查询或预处理语句来防止SQL注入。
- 异常处理:在执行查询操作时,应考虑到可能出现的异常情况,如数据库连接失败、查询语句错误等。可以通过捕获异常并进行适当的处理来提高程序的健壮性。
- 资源释放:在操作数据库时,确保在操作完成后关闭游标和数据库连接,以释放资源,避免资源泄漏。
六、总结
本文详细介绍了如何使用Python查询数据库中的表是否为空。首先通过建立与数据库的连接,然后执行查询语句获取表中的记录数,最后根据查询结果判断表是否为空。此外,还介绍了如何连接和查询其他类型的数据库(如PostgreSQL、SQLite、SQL Server)以及在实际应用中需要注意的事项。希望这些内容对您使用Python进行数据库操作有所帮助。
相关问答FAQs:
如何使用Python查询数据库中非空的字段?
在Python中,可以使用SQL语句结合数据库连接库(如SQLite、MySQL Connector等)来查询非空字段。通常的SQL语句结构为:SELECT * FROM table_name WHERE column_name IS NOT NULL;
。这条语句会返回指定表中所有非空的记录。确保在执行查询之前,已正确设置数据库连接。
在Python中,如何处理查询结果以过滤非空值?
执行查询后,通常会得到一个结果集。可以通过循环遍历结果集,或者使用Python的列表推导式来过滤出非空值。例如,使用cursor.fetchall()
获取所有结果,然后使用条件语句判断哪些字段非空。这样可以有效地处理和分析数据。
Python中常用的数据库连接库有哪些?
在Python中,常见的数据库连接库包括sqlite3
(用于SQLite数据库)、mysql-connector-python
(用于MySQL数据库)、psycopg2
(用于PostgreSQL数据库)等。每种库都有不同的特性和用法,选择合适的库可以提高数据库操作的效率和便捷性。
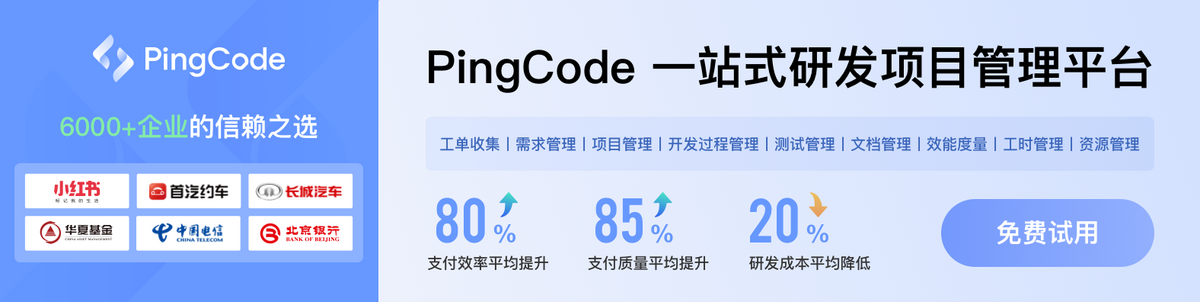