在Python中保存爬取的图片,可以使用requests库下载图片、os库处理文件路径、以及PIL库进行图像处理。首先,使用requests库下载图片,确保图片能够正确获取;其次,使用os库确保保存路径存在;最后,可以使用PIL库进行图像处理和存储。接下来,我们详细介绍这些步骤。
一、使用requests库下载图片
requests库是Python中非常常用的HTTP请求库,可以轻松地发出HTTP请求。下载图片的基本方法是通过requests.get()函数获取图片内容,然后将其写入文件。
import requests
def download_image(url, save_path):
try:
response = requests.get(url, stream=True)
if response.status_code == 200:
with open(save_path, 'wb') as file:
for chunk in response.iter_content(1024):
file.write(chunk)
print(f"Image successfully downloaded: {save_path}")
else:
print("Failed to retrieve image")
except Exception as e:
print(f"An error occurred: {e}")
Example usage
download_image('https://example.com/image.jpg', 'path/to/save/image.jpg')
二、使用os库处理文件路径
在保存图片时,确保保存路径存在是非常重要的。os库提供了许多处理文件路径的方法,例如os.makedirs()可以递归创建目录。
import os
def ensure_directory_exists(directory):
if not os.path.exists(directory):
os.makedirs(directory)
Example usage
directory = 'path/to/save'
ensure_directory_exists(directory)
三、使用PIL库进行图像处理和存储
PIL(Pillow)库是Python Imaging Library的分支,提供了丰富的图像处理功能。使用PIL可以方便地打开、修改和保存图像。
from PIL import Image
import requests
from io import BytesIO
def download_and_save_image(url, save_path):
try:
response = requests.get(url)
if response.status_code == 200:
image = Image.open(BytesIO(response.content))
image.save(save_path)
print(f"Image successfully downloaded and saved: {save_path}")
else:
print("Failed to retrieve image")
except Exception as e:
print(f"An error occurred: {e}")
Example usage
download_and_save_image('https://example.com/image.jpg', 'path/to/save/image.jpg')
四、综合示例
综合以上内容,我们可以创建一个综合示例,将所有步骤结合起来。
import os
import requests
from PIL import Image
from io import BytesIO
def ensure_directory_exists(directory):
if not os.path.exists(directory):
os.makedirs(directory)
def download_image(url, save_path):
try:
response = requests.get(url, stream=True)
if response.status_code == 200:
with open(save_path, 'wb') as file:
for chunk in response.iter_content(1024):
file.write(chunk)
print(f"Image successfully downloaded: {save_path}")
else:
print("Failed to retrieve image")
except Exception as e:
print(f"An error occurred: {e}")
def download_and_save_image(url, save_path):
try:
response = requests.get(url)
if response.status_code == 200:
image = Image.open(BytesIO(response.content))
image.save(save_path)
print(f"Image successfully downloaded and saved: {save_path}")
else:
print("Failed to retrieve image")
except Exception as e:
print(f"An error occurred: {e}")
def main(url, save_directory, filename):
ensure_directory_exists(save_directory)
save_path = os.path.join(save_directory, filename)
download_and_save_image(url, save_path)
Example usage
main('https://example.com/image.jpg', 'path/to/save', 'image.jpg')
通过以上步骤,我们可以轻松地在Python中保存爬取的图片。首先,确保保存路径存在,然后使用requests库下载图片,最后使用PIL库处理和保存图像。这样可以确保图片能够正确下载并保存到指定路径。
相关问答FAQs:
如何在Python中下载和保存图片?
在Python中,您可以使用requests库轻松下载图片。首先,您需要安装requests库(如果尚未安装),可以通过命令pip install requests
来完成。然后,您可以使用以下代码示例来下载并保存图片:
import requests
url = '图片的URL地址'
response = requests.get(url)
if response.status_code == 200:
with open('保存的文件名.jpg', 'wb') as file:
file.write(response.content)
else:
print('无法下载图片,状态码:', response.status_code)
如何处理爬取过程中出现的异常?
在爬取图片时,可能会遇到网络问题或URL无效等情况。为了提高程序的健壮性,您可以使用try-except块来捕获和处理这些异常。以下是一个简单的示例:
try:
response = requests.get(url)
response.raise_for_status() # 如果响应状态码不是200,会抛出异常
with open('保存的文件名.jpg', 'wb') as file:
file.write(response.content)
except requests.exceptions.RequestException as e:
print('发生错误:', e)
如何在爬取多张图片时保存它们?
如果您需要爬取多张图片,可以将图片URL放入一个列表中,并使用循环来下载和保存每一张图片。下面是一个示例:
urls = ['图片1的URL', '图片2的URL', '图片3的URL']
for i, url in enumerate(urls):
try:
response = requests.get(url)
response.raise_for_status()
with open(f'图片_{i+1}.jpg', 'wb') as file:
file.write(response.content)
except requests.exceptions.RequestException as e:
print(f'下载图片{i+1}时发生错误:', e)
通过这些方法,您可以有效地保存爬取的图片,同时处理可能出现的异常情况。
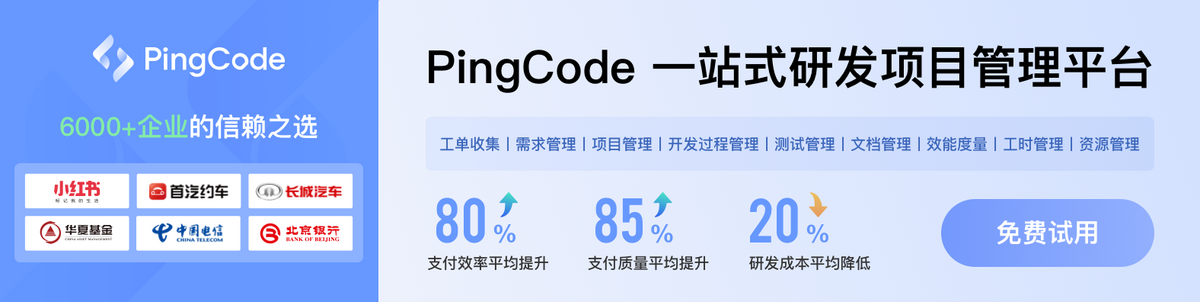