Python显示上一条语录的方法包括:使用列表、使用文件存储、利用数据库。 在本文中,我们将详细探讨这几种方法,并给出相应的代码示例。
一、使用列表存储并显示上一条语录
使用列表存储语录是一种简单而有效的方法。列表可以轻松添加、访问和修改元素,适合小规模的语录存储。
- 创建并操作列表
首先,我们需要创建一个包含语录的列表,并编写函数来显示上一条语录。
quotes = [
"The only limit to our realization of tomorrow is our doubts of today.",
"The future belongs to those who believe in the beauty of their dreams.",
"The best way to predict the future is to invent it."
]
current_index = 0
def show_previous_quote():
global current_index
if current_index > 0:
current_index -= 1
else:
print("This is the first quote.")
print(quotes[current_index])
Example usage
show_previous_quote() # Output: This is the first quote.
current_index = 2 # Let's assume we're at the last quote
show_previous_quote() # Output: The future belongs to those who believe in the beauty of their dreams.
在这个例子中,我们定义了一个包含语录的列表和一个当前索引变量。通过函数show_previous_quote
,我们可以显示上一条语录。
- 循环引用
为了使代码更具交互性,我们可以通过循环引用语录,确保用户始终可以查看上一条语录。
def show_previous_quote_circular():
global current_index
current_index = (current_index - 1) % len(quotes)
print(quotes[current_index])
Example usage
current_index = 0
show_previous_quote_circular() # Output: The best way to predict the future is to invent it.
在这个例子中,我们使用取模运算符%
来实现循环引用,使得列表可以无限循环。
二、使用文件存储并显示上一条语录
对于更大规模的语录存储,使用文件存储是一种有效的方法。我们可以将语录存储在一个文本文件中,然后读取并显示上一条语录。
- 创建并操作文件
首先,我们需要创建一个包含语录的文本文件,并编写函数来读取和显示上一条语录。
# Write quotes to a file
with open('quotes.txt', 'w') as file:
file.write("The only limit to our realization of tomorrow is our doubts of today.\n")
file.write("The future belongs to those who believe in the beauty of their dreams.\n")
file.write("The best way to predict the future is to invent it.\n")
Read quotes from the file
def read_quotes_from_file():
with open('quotes.txt', 'r') as file:
return file.readlines()
quotes = read_quotes_from_file()
current_index = 0
def show_previous_quote_from_file():
global current_index
if current_index > 0:
current_index -= 1
else:
print("This is the first quote.")
print(quotes[current_index].strip())
Example usage
show_previous_quote_from_file() # Output: This is the first quote.
current_index = 2 # Let's assume we're at the last quote
show_previous_quote_from_file() # Output: The future belongs to those who believe in the beauty of their dreams.
在这个例子中,我们首先将语录写入一个文本文件,然后读取文件内容并存储在一个列表中。通过函数show_previous_quote_from_file
,我们可以显示上一条语录。
- 循环引用
同样,我们可以通过循环引用来增强文件存储方法的交互性。
def show_previous_quote_from_file_circular():
global current_index
current_index = (current_index - 1) % len(quotes)
print(quotes[current_index].strip())
Example usage
current_index = 0
show_previous_quote_from_file_circular() # Output: The best way to predict the future is to invent it.
在这个例子中,我们使用取模运算符%
来实现循环引用,使得文件存储的语录可以无限循环。
三、利用数据库存储并显示上一条语录
对于更复杂的应用,使用数据库存储语录是一种高效且灵活的方法。我们可以使用SQLite数据库来存储语录,并编写函数来读取和显示上一条语录。
- 创建并操作数据库
首先,我们需要创建一个包含语录的数据库,并编写函数来读取和显示上一条语录。
import sqlite3
Create a database and a table
conn = sqlite3.connect('quotes.db')
c = conn.cursor()
c.execute('''CREATE TABLE IF NOT EXISTS quotes
(id INTEGER PRIMARY KEY, quote TEXT)''')
Insert quotes into the table
quotes_data = [
(1, "The only limit to our realization of tomorrow is our doubts of today."),
(2, "The future belongs to those who believe in the beauty of their dreams."),
(3, "The best way to predict the future is to invent it.")
]
c.executemany('INSERT OR REPLACE INTO quotes VALUES (?,?)', quotes_data)
conn.commit()
Read quotes from the database
def read_quotes_from_db():
c.execute('SELECT quote FROM quotes ORDER BY id')
return [row[0] for row in c.fetchall()]
quotes = read_quotes_from_db()
current_index = 0
def show_previous_quote_from_db():
global current_index
if current_index > 0:
current_index -= 1
else:
print("This is the first quote.")
print(quotes[current_index])
Example usage
show_previous_quote_from_db() # Output: This is the first quote.
current_index = 2 # Let's assume we're at the last quote
show_previous_quote_from_db() # Output: The future belongs to those who believe in the beauty of their dreams.
Close the database connection
conn.close()
在这个例子中,我们首先创建一个SQLite数据库并插入语录数据,然后读取数据库内容并存储在一个列表中。通过函数show_previous_quote_from_db
,我们可以显示上一条语录。
- 循环引用
同样,我们可以通过循环引用来增强数据库存储方法的交互性。
def show_previous_quote_from_db_circular():
global current_index
current_index = (current_index - 1) % len(quotes)
print(quotes[current_index])
Example usage
current_index = 0
show_previous_quote_from_db_circular() # Output: The best way to predict the future is to invent it.
在这个例子中,我们使用取模运算符%
来实现循环引用,使得数据库存储的语录可以无限循环。
总结
Python显示上一条语录的方法包括:使用列表、使用文件存储、利用数据库。 使用列表是一种简单而有效的方法,适合小规模的语录存储;使用文件存储适合中等规模的语录存储,可以通过循环引用增强交互性;利用数据库存储则适合更复杂的应用,具有高效且灵活的特点。通过以上方法,用户可以根据自身需求选择合适的存储和显示方式。
相关问答FAQs:
如何在Python中获取和显示上一条语录?
在Python中,可以使用列表或字典来存储语录。通过索引或键的方式,可以方便地获取到上一条语录。例如,您可以将语录存储在一个列表中,使用索引 -1 来访问最后一条语录,或者使用一个变量来追踪当前的语录索引,从而轻松显示上一条。
可以用什么方法来存储多条语录以便后续访问?
存储多条语录的常见方法包括使用列表、字典或数据库。列表适合简单的语录存储,而字典允许您使用键值对的方式存储语录,使得访问更为灵活。如果需要持久化存储,可以考虑使用SQLite等数据库,方便后续的检索和管理。
如何处理用户请求以显示上一条语录?
处理用户请求可以通过定义一个函数来实现。该函数可以接受用户输入,并根据当前语录的索引来计算上一条语录的位置。通过判断用户的输入,可以灵活地显示相应的语录,并提供反馈。例如,如果用户输入“上一条”,函数将返回当前索引减一的语录内容。
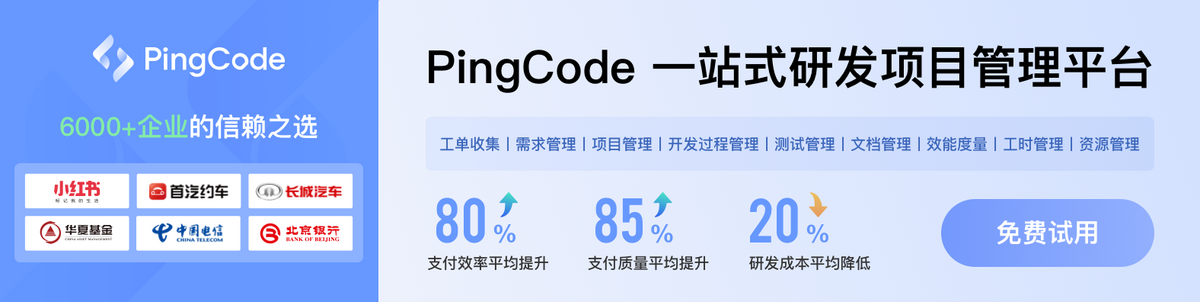