使用Python读取路径下的文件可以通过多种方式实现,常见的方法包括使用内置的open()
函数、os
模块、pathlib
模块等。通过这些方法,你可以轻松读取文件内容、处理文件路径以及进行错误处理等操作。下面,我将详细解释其中一种方法,并提供一些示例代码来帮助你理解。
一、使用open()
函数读取文件
open()
函数是Python内置的文件操作函数,它能够以多种模式打开文件,例如读取('r')、写入('w')、追加('a')等。以下是使用open()
函数读取路径下的文件的示例:
def read_file(file_path):
try:
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
return content
except FileNotFoundError:
print(f"The file at {file_path} does not exist.")
except Exception as e:
print(f"An error occurred: {e}")
file_path = 'path/to/your/file.txt'
file_content = read_file(file_path)
if file_content:
print(file_content)
在这个示例中,我们定义了一个函数read_file
,它接受一个文件路径作为参数,并尝试以读取模式('r')打开文件。如果文件不存在,会捕获FileNotFoundError
并打印错误消息。如果发生其他异常,也会捕获并打印错误消息。使用with
语句可以确保文件在读取完成后自动关闭。
二、使用os
模块处理文件路径
os
模块提供了一些处理文件路径和文件操作的有用函数,例如os.path.join()
、os.path.exists()
等。以下是使用os
模块读取文件的示例:
import os
def read_file_with_os(file_path):
if os.path.exists(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
return content
else:
print(f"The file at {file_path} does not exist.")
return None
file_path = os.path.join('path', 'to', 'your', 'file.txt')
file_content = read_file_with_os(file_path)
if file_content:
print(file_content)
在这个示例中,我们使用os.path.exists()
函数来检查文件是否存在,然后使用open()
函数读取文件内容。os.path.join()
函数用于构建跨平台的文件路径。
三、使用pathlib
模块处理文件路径
pathlib
模块是Python 3.4引入的用于面向对象地处理文件和目录路径的模块。它提供了比os
模块更直观和易用的接口。以下是使用pathlib
模块读取文件的示例:
from pathlib import Path
def read_file_with_pathlib(file_path):
path = Path(file_path)
if path.exists():
try:
content = path.read_text(encoding='utf-8')
return content
except Exception as e:
print(f"An error occurred: {e}")
else:
print(f"The file at {file_path} does not exist.")
return None
file_path = Path('path/to/your/file.txt')
file_content = read_file_with_pathlib(file_path)
if file_content:
print(file_content)
在这个示例中,我们使用Path
对象来表示文件路径,并使用Path.exists()
方法检查文件是否存在。Path.read_text()
方法用于读取文件内容,并且可以指定编码格式。
四、读取大文件的处理
当文件较大时,直接读取整个文件内容可能会导致内存不足的情况。此时,可以逐行读取文件内容:
def read_large_file(file_path):
try:
with open(file_path, 'r', encoding='utf-8') as file:
for line in file:
print(line.strip())
except FileNotFoundError:
print(f"The file at {file_path} does not exist.")
except Exception as e:
print(f"An error occurred: {e}")
file_path = 'path/to/your/large_file.txt'
read_large_file(file_path)
在这个示例中,我们使用for line in file
逐行读取文件内容,每次只将一行内容加载到内存中,并使用print(line.strip())
打印每行内容。
五、处理文件读取过程中的异常
在读取文件时,可能会遇到各种异常情况,例如文件不存在、权限不足等。我们可以使用try
/except
块来捕获并处理这些异常:
def read_file_with_exception_handling(file_path):
try:
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
return content
except FileNotFoundError:
print(f"The file at {file_path} does not exist.")
except PermissionError:
print(f"Permission denied to read the file at {file_path}.")
except Exception as e:
print(f"An unexpected error occurred: {e}")
file_path = 'path/to/your/file.txt'
file_content = read_file_with_exception_handling(file_path)
if file_content:
print(file_content)
在这个示例中,我们捕获了FileNotFoundError
和PermissionError
异常,并分别打印不同的错误消息。对于其他未预料到的异常,我们使用通用的Exception
类进行捕获并打印错误消息。
六、读取二进制文件
有时我们需要读取二进制文件,例如图片、音频文件等。可以通过在open()
函数中指定模式为'rb'
(读取二进制)来读取二进制文件:
def read_binary_file(file_path):
try:
with open(file_path, 'rb') as file:
content = file.read()
return content
except FileNotFoundError:
print(f"The file at {file_path} does not exist.")
except Exception as e:
print(f"An error occurred: {e}")
file_path = 'path/to/your/binary_file.bin'
file_content = read_binary_file(file_path)
if file_content:
print(f"Binary content of the file: {file_content[:20]}...") # 打印前20个字节
在这个示例中,我们以二进制模式打开文件,并读取其内容。为了展示读取到的内容,我们打印了二进制文件的前20个字节。
七、总结
综上所述,Python提供了多种方法来读取路径下的文件,包括使用内置的open()
函数、os
模块和pathlib
模块等。通过合理选择和组合这些方法,可以实现高效、灵活的文件读取操作。在实际应用中,根据具体需求选择适当的方法,并注意处理可能出现的异常情况,可以确保文件读取操作的可靠性和稳定性。
相关问答FAQs:
如何在Python中读取特定路径下的文件?
在Python中,可以使用内置的open()
函数来读取特定路径下的文件。通过提供文件的完整路径作为参数,您可以打开文件并使用read()
方法读取其内容。例如,with open('C:/path/to/your/file.txt', 'r') as file:
可以安全地打开文件并在使用后自动关闭它。
在读取文件时,如何处理文件编码问题?
文件编码可能会影响读取内容的方式。如果您知道文件的编码方式,可以在使用open()
函数时指定encoding
参数,例如open('file.txt', 'r', encoding='utf-8')
。如果不确定,可以使用chardet
库来检测文件编码,从而确保正确读取文件内容。
如何读取文件中的特定行或内容?
如果您只想读取文件中的特定行或内容,可以使用readlines()
方法获取所有行的列表,然后通过索引访问特定行。例如,lines = file.readlines()
将把所有行存储在lines
列表中,您可以通过lines[0]
获取第一行内容。此外,使用循环可以逐行处理文件内容,实现更灵活的读取方式。
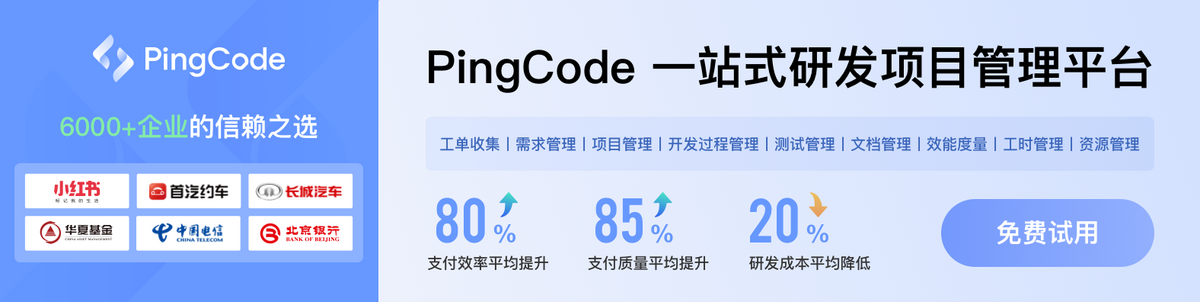