如何统计英文字符数Python
统计英文字符数在Python中是一个非常基础且常见的任务,主要方法包括使用内置函数len()、使用正则表达式、使用collections.Counter。其中,使用内置函数len()是最简便的方法,而正则表达式可以帮助过滤非英文字符。接下来,我将详细介绍如何使用这些方法。
一、使用内置函数len()
Python的内置函数len()可以直接计算字符串的长度。对于大多数场景,这个方法已经足够。然而,如果字符串中包含非英文字符,这种方法可能不适用。
def count_characters_using_len(text):
return len(text)
text = "Hello, World!"
print(count_characters_using_len(text)) # 输出13
二、使用正则表达式
正则表达式是处理字符串的强大工具。通过正则表达式,可以过滤掉非英文字符,从而精确统计英文字符数。
import re
def count_english_characters(text):
# 使用正则表达式过滤非英文字符
filtered_text = re.findall(r'[a-zA-Z]', text)
return len(filtered_text)
text = "Hello, World! 123"
print(count_english_characters(text)) # 输出10
在上述代码中,re.findall()
函数返回一个列表,包含所有匹配的字符。我们使用 [a-zA-Z]
模式来匹配所有英文字符。
三、使用collections.Counter
collections.Counter
是一个计数器工具,可以统计各个字符出现的频次。通过过滤,只计算英文字符的总数。
from collections import Counter
def count_english_characters_using_counter(text):
counter = Counter(text)
english_chars = [char for char in counter if char.isalpha()]
return sum(counter[char] for char in english_chars)
text = "Hello, World! 123"
print(count_english_characters_using_counter(text)) # 输出10
在这里,我们首先创建一个计数器,然后通过列表推导式过滤出所有英文字符,最后计算它们的总数。
四、处理大文本文件
在实际应用中,我们经常需要处理大文本文件。以下是如何读取文件并统计英文字符数的方法。
def count_english_characters_in_file(file_path):
total_count = 0
with open(file_path, 'r', encoding='utf-8') as file:
for line in file:
total_count += count_english_characters(line)
return total_count
file_path = 'large_text_file.txt'
print(count_english_characters_in_file(file_path)) # 根据文件内容输出
五、忽略大小写统计字符出现频次
有时我们不仅需要统计字符数,还需要统计各个字符出现的频次,并且忽略大小写。
def count_characters_frequency(text):
# 将所有字符转换为小写
text = text.lower()
counter = Counter(text)
english_chars = {char: count for char, count in counter.items() if char.isalpha()}
return english_chars
text = "Hello, World! 123"
print(count_characters_frequency(text)) # 输出{'h': 1, 'e': 1, 'l': 3, 'o': 2, 'w': 1, 'r': 1, 'd': 1}
在这个例子中,我们首先将所有字符转换为小写,然后使用计数器统计每个字符的频次。
六、统计英文单词数
除了统计字符数,有时我们还需要统计英文单词数。我们可以使用正则表达式来实现。
def count_english_words(text):
# 使用正则表达式匹配所有单词
words = re.findall(r'\b[a-zA-Z]+\b', text)
return len(words)
text = "Hello, World! This is a test."
print(count_english_words(text)) # 输出5
在这个例子中,\b[a-zA-Z]+\b
模式匹配所有英文单词。
七、综合使用多种方法
在实际项目中,我们可能需要综合使用多种方法以满足不同需求。下面是一个综合示例,结合了字符数统计、字符频次统计和单词数统计。
def analyze_text(text):
char_count = count_english_characters(text)
word_count = count_english_words(text)
char_frequency = count_characters_frequency(text)
return {
'char_count': char_count,
'word_count': word_count,
'char_frequency': char_frequency
}
text = "Hello, World! This is a test."
analysis = analyze_text(text)
print(analysis)
输出 {'char_count': 21, 'word_count': 5, 'char_frequency': {'h': 2, 'e': 2, 'l': 3, 'o': 2, 'w': 1, 'r': 1, 'd': 1, 't': 3, 'i': 2, 's': 2, 'a': 1}}
八、性能优化
在处理大文本时,性能是一个重要考虑因素。我们可以通过逐行读取文件并实时统计来优化性能。
def optimized_count_english_characters_in_file(file_path):
total_count = 0
with open(file_path, 'r', encoding='utf-8') as file:
for line in file:
filtered_text = re.findall(r'[a-zA-Z]', line)
total_count += len(filtered_text)
return total_count
file_path = 'large_text_file.txt'
print(optimized_count_english_characters_in_file(file_path)) # 根据文件内容输出
通过逐行读取文件并实时统计,我们可以显著减少内存使用,提升处理效率。
九、结论
统计英文字符数在Python中有多种方法可选,使用内置函数len()、使用正则表达式、使用collections.Counter 是常见且有效的手段。根据具体需求,可以选择适合的方法或综合使用多种方法。在处理大文本时,性能优化是关键,通过逐行读取和实时统计可以有效提升处理效率。无论是字符数统计、字符频次统计还是单词数统计,Python都提供了丰富的工具和库,帮助我们高效完成任务。
相关问答FAQs:
如何在Python中统计字符串中的英文字符数?
要统计字符串中的英文字符数,可以使用Python的内置函数和正则表达式。可以通过遍历字符串,使用条件语句判断每个字符是否为英文字符。使用正则表达式的方式则可以更简洁地实现这一功能。例如,len(re.findall(r'[a-zA-Z]', your_string))
可以直接返回英文字符的数量。
使用Python统计英文字符数时,有哪些常见的错误?
在统计英文字符数时,常见的错误包括忽略了大小写的字符或者未考虑到空格和标点符号。确保正则表达式或字符判断逻辑仅针对字母字符进行统计,有助于避免这些问题。此外,确保字符串已正确输入并去除了多余的空格。
除了英文字符,如何在Python中统计其他语言的字符数?
可以使用类似的方法来统计其他语言的字符数,只需更改正则表达式或字符判断逻辑。例如,若要统计中文字符,可以使用len(re.findall(r'[\u4e00-\u9fa5]', your_string))
。这种方法适用于多种语言,确保根据字符集的Unicode范围进行相应调整。
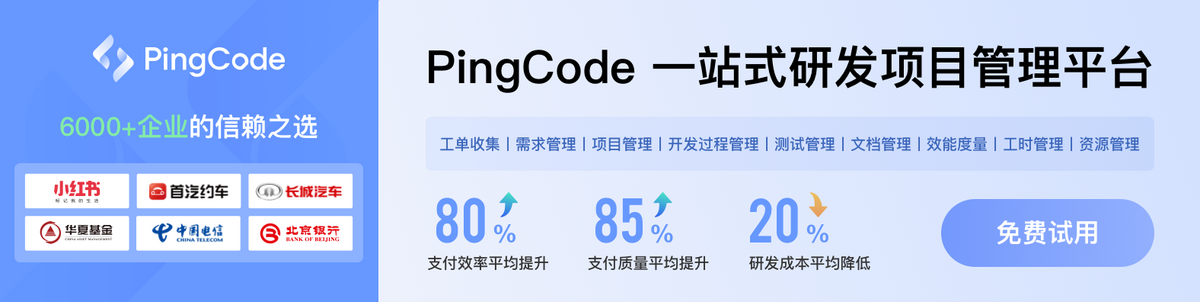