Python可以通过内置的open()
函数来创建和打开txt文件、指定文件模式、使用上下文管理器来确保文件正确关闭和处理文件内容。
Python提供了多种方式来创建和打开txt文件,其中最常用的是使用open()
函数。通过指定不同的文件模式,我们可以创建新文件、读取文件内容、写入文件内容或追加内容。此外,使用上下文管理器(with
语句)可以确保文件在操作完成后正确关闭,避免资源泄漏。下面我们将详细介绍这些方法,并提供示例代码。
一、open()
函数介绍
open()
函数是Python中用于打开文件的内置函数。其基本语法如下:
open(file, mode='r', buffering=-1, encoding=None, errors=None, newline=None, closefd=True, opener=None)
参数说明:
file
:文件路径(字符串)。mode
:文件打开模式(字符串),默认为只读模式'r'。buffering
:缓冲策略,默认为-1(自动选择)。encoding
:文件编码,默认为None(平台默认编码)。errors
:错误处理策略,默认为None。newline
:控制行结束符的处理方式,默认为None。closefd
:是否关闭文件描述符,默认为True。opener
:自定义打开器,默认为None。
常用的文件模式:
'r'
:只读模式(默认)。'w'
:写入模式(会覆盖已有文件)。'a'
:追加模式(在文件末尾追加内容)。'b'
:二进制模式(与其他模式结合使用,如'rb')。't'
:文本模式(与其他模式结合使用,如'rt',默认)。
二、创建和打开txt文件
- 使用
'w'
模式创建和写入文件
# 使用'w'模式创建并写入文件
file_path = 'example.txt'
with open(file_path, 'w', encoding='utf-8') as file:
file.write('Hello, World!\n')
file.write('This is a new line.\n')
print(f"File '{file_path}' created and written successfully.")
- 使用
'a'
模式创建和追加内容
# 使用'a'模式创建并追加内容
file_path = 'example.txt'
with open(file_path, 'a', encoding='utf-8') as file:
file.write('This is an appended line.\n')
print(f"Content appended to file '{file_path}' successfully.")
- 使用
'r'
模式读取文件内容
# 使用'r'模式读取文件内容
file_path = 'example.txt'
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
print(f"Content of file '{file_path}':\n{content}")
三、上下文管理器的使用
使用上下文管理器(with
语句)可以确保文件在操作完成后正确关闭,避免资源泄漏。示例如下:
# 使用上下文管理器创建并写入文件
file_path = 'example.txt'
with open(file_path, 'w', encoding='utf-8') as file:
file.write('Hello, World!\n')
file.write('This is a new line.\n')
使用上下文管理器读取文件内容
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
print(f"Content of file '{file_path}':\n{content}")
使用上下文管理器追加内容
with open(file_path, 'a', encoding='utf-8') as file:
file.write('This is an appended line.\n')
再次读取文件内容
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
print(f"Updated content of file '{file_path}':\n{content}")
四、处理文件操作中的常见问题
- 文件路径问题
确保文件路径正确。如果文件在当前目录下,直接使用文件名即可;如果文件在其他目录下,需要提供相对路径或绝对路径。例如:
# 相对路径
file_path = './subdirectory/example.txt'
绝对路径
file_path = 'C:/Users/Username/Documents/example.txt'
- 文件编码问题
读取和写入文件时,确保文件编码一致。常见编码包括'utf-8'、'ascii'、'latin-1'等。如果文件编码不一致,可能会导致读取或写入失败。例如:
# 写入文件时使用utf-8编码
file_path = 'example.txt'
with open(file_path, 'w', encoding='utf-8') as file:
file.write('你好,世界!\n')
读取文件时也使用utf-8编码
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
print(f"Content of file '{file_path}':\n{content}")
- 文件不存在问题
尝试读取不存在的文件时,会引发FileNotFoundError
异常。可以使用try-except
块处理该异常。例如:
file_path = 'nonexistent.txt'
try:
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
except FileNotFoundError:
print(f"File '{file_path}' does not exist.")
五、总结
通过使用Python的open()
函数,我们可以轻松地创建、打开、读取和写入txt文件。使用上下文管理器可以确保文件在操作完成后正确关闭,避免资源泄漏。此外,处理文件操作时常见的问题,如文件路径、编码和文件不存在问题,可以提高代码的健壮性。希望这篇文章能够帮助您更好地理解和掌握Python中文件操作的相关知识。
相关问答FAQs:
如何在Python中创建并打开一个新的TXT文件?
在Python中,可以使用内置的open()
函数来创建和打开TXT文件。通过指定文件名和模式,您可以创建一个新文件并立即开始写入内容。例如,使用open('example.txt', 'w')
可以创建一个名为example.txt
的文件。如果文件已经存在,它会被清空。您可以使用'a'
模式来打开文件并在现有内容后添加新内容,而不删除原有数据。
在Python中如何安全地写入TXT文件?
为了确保在写入TXT文件时不发生数据丢失,建议使用with
语句。这种方法可以自动处理文件的关闭。例如:
with open('example.txt', 'w') as file:
file.write("这是一个示例文本。")
使用with
语句可以确保即使在写入过程中出现异常,文件也会被正确关闭。
如何在Python中读取已存在的TXT文件内容?
要读取TXT文件的内容,可以使用open()
函数与'r'
模式。例如,使用open('example.txt', 'r')
可以打开文件并读取其内容。可以通过read()
方法一次性读取整个文件,或者使用readline()
逐行读取。以下是一个简单的示例:
with open('example.txt', 'r') as file:
content = file.read()
print(content)
这种方法非常适合处理文本文件的内容,无论文件大小如何。
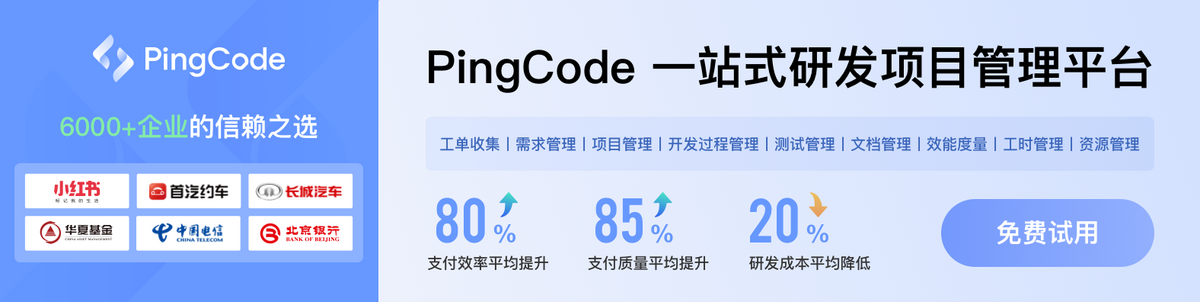