Python需要安装的库包括但不限于:NumPy、Pandas、Matplotlib、Scikit-learn、Requests、BeautifulSoup、TensorFlow、PyTorch、Django、Flask、SQLAlchemy、Scrapy、Pillow。这些库涵盖了数据处理、数据可视化、机器学习、网络请求、网页解析、深度学习、Web开发、数据库操作、网络爬虫、图像处理等多个领域,适用于不同的项目需求。以下将详细介绍其中一个常用库的安装与使用。
NumPy 是一个用于科学计算的基础库。它提供了支持高效操作的大型多维数组和矩阵,并附带了大量数学函数库。为了安装NumPy,你可以使用Python的包管理工具pip
。
pip install numpy
在安装完成后,可以通过以下代码验证是否安装成功:
import numpy as np
print(np.__version__)
下面将详细介绍如何使用NumPy进行常见的科学计算操作。
一、NumPy安装及基础操作
安装NumPy
在Python中,安装库通常使用包管理工具pip
。可以在命令行中输入以下命令来安装NumPy:
pip install numpy
安装完成后,可以通过以下代码验证是否安装成功:
import numpy as np
print(np.__version__)
基础操作
NumPy最核心的功能是其高效的多维数组对象ndarray
。以下是一些常见的操作:
创建数组
可以通过多种方式创建NumPy数组:
import numpy as np
从列表创建数组
arr1 = np.array([1, 2, 3, 4, 5])
print(arr1)
创建全零数组
arr2 = np.zeros((3, 4))
print(arr2)
创建全一数组
arr3 = np.ones((2, 3))
print(arr3)
创建指定值的数组
arr4 = np.full((2, 2), 7)
print(arr4)
创建单位矩阵
arr5 = np.eye(3)
print(arr5)
创建随机数组
arr6 = np.random.random((2, 2))
print(arr6)
数组操作
NumPy数组支持多种操作,包括切片、索引、形状操作等:
# 创建数组
arr = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
数组切片
print(arr[0:2, 1:3])
数组形状
print(arr.shape)
改变数组形状
arr_reshaped = arr.reshape((1, 9))
print(arr_reshaped)
数组元素类型
print(arr.dtype)
数组元素类型转换
arr_float = arr.astype(np.float64)
print(arr_float.dtype)
二、Pandas安装及数据处理
安装Pandas
Pandas是一个强大的数据分析和数据处理库。可以通过以下命令安装:
pip install pandas
安装完成后,可以通过以下代码验证是否安装成功:
import pandas as pd
print(pd.__version__)
数据处理
Pandas最常用的数据结构是DataFrame
和Series
。以下是一些常见的数据操作:
创建DataFrame
可以通过多种方式创建DataFrame:
import pandas as pd
从字典创建DataFrame
data = {
'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [24, 27, 22],
'City': ['New York', 'Los Angeles', 'Chicago']
}
df = pd.DataFrame(data)
print(df)
从列表创建DataFrame
data = [
['Alice', 24, 'New York'],
['Bob', 27, 'Los Angeles'],
['Charlie', 22, 'Chicago']
]
df = pd.DataFrame(data, columns=['Name', 'Age', 'City'])
print(df)
从CSV文件创建DataFrame
df = pd.read_csv('data.csv')
print(df)
数据操作
Pandas提供了丰富的数据操作功能:
# 选择列
print(df['Name'])
选择行
print(df.loc[0])
选择多行多列
print(df.loc[0:1, ['Name', 'City']])
添加新列
df['Salary'] = [50000, 60000, 55000]
print(df)
删除列
df = df.drop(columns=['Age'])
print(df)
数据统计
print(df.describe())
数据分组
grouped = df.groupby('City').mean()
print(grouped)
三、Matplotlib安装及数据可视化
安装Matplotlib
Matplotlib是一个数据可视化库。可以通过以下命令安装:
pip install matplotlib
安装完成后,可以通过以下代码验证是否安装成功:
import matplotlib.pyplot as plt
print(plt.__version__)
数据可视化
Matplotlib提供了多种绘图功能:
绘制折线图
import matplotlib.pyplot as plt
创建数据
x = [1, 2, 3, 4, 5]
y = [10, 20, 25, 30, 35]
绘制折线图
plt.plot(x, y)
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Line Plot')
plt.show()
绘制柱状图
import matplotlib.pyplot as plt
创建数据
categories = ['A', 'B', 'C', 'D']
values = [5, 7, 3, 8]
绘制柱状图
plt.bar(categories, values)
plt.xlabel('Categories')
plt.ylabel('Values')
plt.title('Bar Plot')
plt.show()
绘制散点图
import matplotlib.pyplot as plt
创建数据
x = [1, 2, 3, 4, 5]
y = [10, 20, 25, 30, 35]
绘制散点图
plt.scatter(x, y)
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Scatter Plot')
plt.show()
绘制直方图
import matplotlib.pyplot as plt
创建数据
data = [1, 2, 2, 3, 3, 3, 4, 4, 4, 4, 5, 5, 5, 5, 5]
绘制直方图
plt.hist(data, bins=5)
plt.xlabel('Value')
plt.ylabel('Frequency')
plt.title('Histogram')
plt.show()
四、Scikit-learn安装及机器学习
安装Scikit-learn
Scikit-learn是一个机器学习库。可以通过以下命令安装:
pip install scikit-learn
安装完成后,可以通过以下代码验证是否安装成功:
import sklearn
print(sklearn.__version__)
机器学习
Scikit-learn提供了丰富的机器学习算法和工具:
数据集加载
Scikit-learn提供了多种内置数据集:
from sklearn.datasets import load_iris
加载数据集
iris = load_iris()
print(iris.data)
print(iris.target)
数据预处理
Scikit-learn提供了多种数据预处理工具:
from sklearn.preprocessing import StandardScaler
创建数据
data = [[1, 2], [3, 4], [5, 6]]
标准化
scaler = StandardScaler()
scaled_data = scaler.fit_transform(data)
print(scaled_data)
训练模型
可以使用Scikit-learn进行模型训练:
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LogisticRegression
from sklearn.metrics import accuracy_score
加载数据集
iris = load_iris()
X_train, X_test, y_train, y_test = train_test_split(iris.data, iris.target, test_size=0.2, random_state=42)
创建模型
model = LogisticRegression()
训练模型
model.fit(X_train, y_train)
预测
y_pred = model.predict(X_test)
评估模型
accuracy = accuracy_score(y_test, y_pred)
print('Accuracy:', accuracy)
五、Requests安装及网络请求
安装Requests
Requests是一个简单易用的HTTP库。可以通过以下命令安装:
pip install requests
安装完成后,可以通过以下代码验证是否安装成功:
import requests
print(requests.__version__)
网络请求
Requests提供了丰富的HTTP请求功能:
发送GET请求
import requests
发送GET请求
response = requests.get('https://api.github.com')
输出响应内容
print(response.text)
发送POST请求
import requests
发送POST请求
data = {'key': 'value'}
response = requests.post('https://httpbin.org/post', data=data)
输出响应内容
print(response.text)
设置请求头
import requests
设置请求头
headers = {'User-Agent': 'Mozilla/5.0'}
response = requests.get('https://api.github.com', headers=headers)
输出响应内容
print(response.text)
处理响应
import requests
发送GET请求
response = requests.get('https://api.github.com')
输出响应状态码
print(response.status_code)
输出响应头
print(response.headers)
输出JSON响应内容
print(response.json())
六、BeautifulSoup安装及网页解析
安装BeautifulSoup
BeautifulSoup是一个用于解析HTML和XML的库。可以通过以下命令安装:
pip install beautifulsoup4
pip install lxml
安装完成后,可以通过以下代码验证是否安装成功:
from bs4 import BeautifulSoup
print(BeautifulSoup.__version__)
网页解析
BeautifulSoup提供了丰富的HTML和XML解析功能:
解析HTML
from bs4 import BeautifulSoup
创建HTML文档
html_doc = """
<html><head><title>The Dormouse's story</title></head>
<body>
<p class="title"><b>The Dormouse's story</b></p>
<p class="story">Once upon a time there were three little sisters; and their names were
<a href="http://example.com/elsie" class="sister" id="link1">Elsie</a>,
<a href="http://example.com/lacie" class="sister" id="link2">Lacie</a> and
<a href="http://example.com/tillie" class="sister" id="link3">Tillie</a>;
and they lived at the bottom of a well.</p>
<p class="story">...</p>
"""
解析HTML
soup = BeautifulSoup(html_doc, 'lxml')
输出解析结果
print(soup.prettify())
查找元素
from bs4 import BeautifulSoup
创建HTML文档
html_doc = """
<html><head><title>The Dormouse's story</title></head>
<body>
<p class="title"><b>The Dormouse's story</b></p>
<p class="story">Once upon a time there were three little sisters; and their names were
<a href="http://example.com/elsie" class="sister" id="link1">Elsie</a>,
<a href="http://example.com/lacie" class="sister" id="link2">Lacie</a> and
<a href="http://example.com/tillie" class="sister" id="link3">Tillie</a>;
and they lived at the bottom of a well.</p>
<p class="story">...</p>
"""
解析HTML
soup = BeautifulSoup(html_doc, 'lxml')
查找所有链接
links = soup.find_all('a')
for link in links:
print(link.get('href'))
查找特定ID的元素
link2 = soup.find(id='link2')
print(link2.text)
查找特定类的元素
story_paragraphs = soup.find_all('p', class_='story')
for paragraph in story_paragraphs:
print(paragraph.text)
七、TensorFlow安装及深度学习
安装TensorFlow
TensorFlow是一个开源的机器学习库,广泛用于深度学习任务。可以通过以下命令安装:
pip install tensorflow
安装完成后,可以通过以下代码验证是否安装成功:
import tensorflow as tf
print(tf.__version__)
深度学习
TensorFlow提供了丰富的深度学习功能:
创建神经网络
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense
创建模型
model = Sequential()
model.add(Dense(128, activation='relu', input_shape=(784,)))
model.add(Dense(64, activation='relu'))
model.add(Dense(10, activation='softmax'))
编译模型
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
输出模型摘要
model.summary()
训练模型
import tensorflow as tf
from tensorflow.keras.datasets import mnist
加载数据集
(x_train, y_train), (x_test, y_test) = mnist.load_data()
预处理数据
x_train = x_train.reshape(-1, 784).astype('float32') / 255
x_test = x_test.reshape(-1, 784).astype('float32') / 255
训练模型
model.fit(x_train, y_train, epochs=5, batch_size=32, validation_split=0.2)
评估模型
loss, accuracy = model.evaluate(x_test, y_test)
print('Test accuracy:', accuracy)
保存与加载模型
# 保存模型
model.save('my_model.h5')
加载模型
new_model = tf.keras.models.load_model('my_model.h5')
输出模型摘要
new_model.summary()
八、PyTorch安装及深度学习
安装PyTorch
PyTorch是一个开源的深度学习库,具有动态计算图的特性。可以通过以下命令安装:
pip install torch torchvision
安装完成后,可以通过以下代码验证是否安装成功:
import torch
print(torch.__version__)
深度学习
PyTorch提供了丰富的深度学习功能:
创建神经网络
import torch
import torch.nn as nn
import torch.optim as optim
定义模型
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.fc1 = nn.Linear(784, 128)
self.fc2 = nn.Linear(128, 64)
self.fc3 = nn.Linear(64, 10)
def forward(self, x):
x = torch.relu(self.fc1(x))
x = torch.relu(self.fc2(x))
x = torch.log_softmax(self.fc3(x), dim=1)
return x
创建模型
model = Net()
print(model)
训练模型
import torch
import torch.nn as nn
import torch.optim as optim
from torchvision import datasets, transforms
加载数据集
transform = transforms.Compose([transforms.ToTensor(), transforms.Normalize((0.5,), (0.5,))])
trainset = datasets.MNIST(root='./data', train=True, download=True, transform=transform)
trainloader = torch.utils.data.DataLoader(trainset, batch_size=32, shuffle=True)
定义损失函数和优化器
criterion = nn.NLLLoss()
optimizer = optim.Adam(model.parameters(), lr=0.001)
训练模型
epochs = 5
for epoch in range(epochs):
running_loss = 0
for images, labels in trainloader:
images = images.view(images.shape[0], -1)
optimizer.zero_grad()
output = model(images)
loss = criterion(output, labels)
loss.backward()
optimizer.step()
running_loss += loss.item()
print(f'Epoch {epoch+1}, Loss: {running_loss/len(trainloader)}')
评估模型
import torch
import torch.nn as nn
import torch.optim as optim
from torchvision import datasets, transforms
加载数据集
transform = transforms.Compose([transforms.ToTensor(), transforms.Normalize((0.5,), (0.5,))])
testset = datasets.MNIST(root='./data', train=False, download=True, transform=transform)
testloader = torch.utils.data.DataLoader(testset, batch_size=32, shuffle=False)
评估模型
correct = 0
total = 0
with torch.no_grad():
for images, labels in testloader:
images = images.view(images.shape[0], -1)
output = model(images)
_, predicted = torch.max(output,
相关问答FAQs:
在Python中常用的库有哪些?
Python拥有丰富的库生态系统,常用的库包括但不限于:
- NumPy:用于科学计算和数值处理,提供高效的多维数组对象。
- Pandas:强大的数据分析和数据处理工具,适合处理表格数据。
- Matplotlib:数据可视化库,用于生成各种图表和图像。
- Scikit-learn:机器学习库,提供各种算法和工具用于数据挖掘和数据分析。
- Requests:用于发送HTTP请求的库,非常适合网络编程。
- Flask/Django:用于Web开发的框架,Flask适合小型项目,而Django适合大型应用。
如何在Python中安装库?
安装库通常使用Python的包管理工具pip
。打开命令行或终端,输入以下命令来安装所需库:
pip install 包名
例如,安装NumPy可以使用:
pip install numpy
确保已安装pip
,如果没有,可以通过Python的官方网站或使用以下命令安装:
python -m ensurepip --default-pip
如何管理和更新已安装的库?
管理已安装的库可以使用以下命令:
- 查看已安装库:
pip list
- 更新库到最新版本:
pip install --upgrade 包名
例如,要更新Pandas,可以使用:
pip install --upgrade pandas
使用这些命令可以确保您的库是最新的,帮助您利用最新的功能和修复。
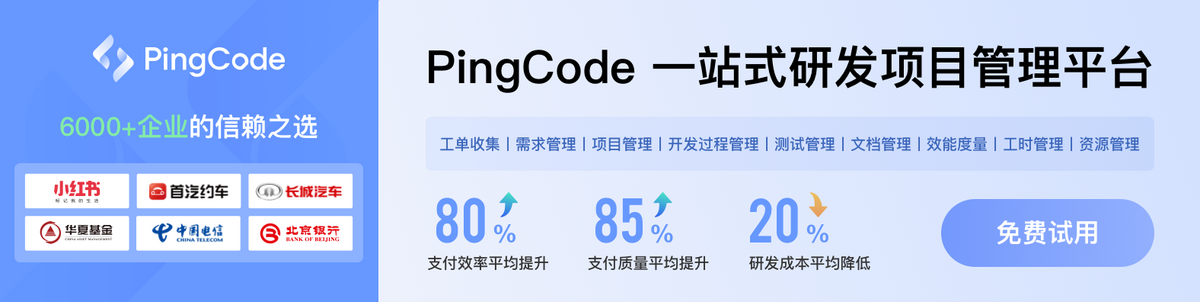