在Python中删除特定的一行可以使用多种方法,主要包括读取文件内容、删除特定行、写回文件内容等步骤。常用的方法有:使用文件操作、使用pandas库、使用正则表达式等。以下是通过读取文件内容、删除特定行、写回文件内容的方法进行详细描述。
文件操作方法
1. 读取文件内容
首先,我们需要读取文件的内容并将其存储在列表中,以便后续操作。可以使用readlines()
方法将文件内容读取为一个列表。
def read_file(file_path):
with open(file_path, 'r') as file:
lines = file.readlines()
return lines
2. 删除特定行
接下来,我们可以通过行号或者特定条件来删除指定的行。以下是通过行号删除特定行的示例:
def delete_line(lines, line_number):
if 0 <= line_number < len(lines):
del lines[line_number]
return lines
如果需要根据特定条件删除行,比如删除包含特定字符串的行,可以使用以下代码:
def delete_line_by_condition(lines, condition):
return [line for line in lines if condition not in line]
3. 写回文件内容
在删除特定行后,我们需要将修改后的内容写回到文件中。可以使用writelines()
方法将列表内容写回文件。
def write_file(file_path, lines):
with open(file_path, 'w') as file:
file.writelines(lines)
4. 完整示例
以下是完整的示例代码,包括读取文件、删除特定行、写回文件内容的步骤:
def delete_specific_line(file_path, line_number):
# 读取文件内容
lines = read_file(file_path)
# 删除特定行
lines = delete_line(lines, line_number)
# 写回文件内容
write_file(file_path, lines)
def read_file(file_path):
with open(file_path, 'r') as file:
lines = file.readlines()
return lines
def delete_line(lines, line_number):
if 0 <= line_number < len(lines):
del lines[line_number]
return lines
def write_file(file_path, lines):
with open(file_path, 'w') as file:
file.writelines(lines)
示例用法
file_path = 'example.txt'
line_number = 2
delete_specific_line(file_path, line_number)
使用Pandas库
1. 读取文件内容
使用pandas库读取文件内容,可以方便地对数据进行操作。以下是读取CSV文件的示例:
import pandas as pd
def read_csv(file_path):
return pd.read_csv(file_path)
2. 删除特定行
可以根据行号或者特定条件删除行。以下是通过行号删除特定行的示例:
def delete_row_by_index(df, index):
return df.drop(index)
如果需要根据特定条件删除行,比如删除包含特定字符串的行,可以使用以下代码:
def delete_row_by_condition(df, column_name, condition):
return df[df[column_name] != condition]
3. 写回文件内容
在删除特定行后,我们需要将修改后的内容写回到文件中。可以使用to_csv()
方法将DataFrame内容写回文件。
def write_csv(file_path, df):
df.to_csv(file_path, index=False)
4. 完整示例
以下是完整的示例代码,包括读取文件、删除特定行、写回文件内容的步骤:
import pandas as pd
def delete_specific_row(file_path, index):
# 读取文件内容
df = read_csv(file_path)
# 删除特定行
df = delete_row_by_index(df, index)
# 写回文件内容
write_csv(file_path, df)
def read_csv(file_path):
return pd.read_csv(file_path)
def delete_row_by_index(df, index):
return df.drop(index)
def write_csv(file_path, df):
df.to_csv(file_path, index=False)
示例用法
file_path = 'example.csv'
index = 2
delete_specific_row(file_path, index)
使用正则表达式
1. 读取文件内容
首先,我们需要读取文件的内容并将其存储在字符串中,以便后续操作。可以使用read()
方法将文件内容读取为字符串。
def read_file(file_path):
with open(file_path, 'r') as file:
content = file.read()
return content
2. 删除特定行
接下来,我们可以使用正则表达式来匹配并删除特定行。以下是删除包含特定字符串的行的示例:
import re
def delete_line_by_regex(content, pattern):
return re.sub(pattern, '', content)
3. 写回文件内容
在删除特定行后,我们需要将修改后的内容写回到文件中。可以使用write()
方法将字符串内容写回文件。
def write_file(file_path, content):
with open(file_path, 'w') as file:
file.write(content)
4. 完整示例
以下是完整的示例代码,包括读取文件、删除特定行、写回文件内容的步骤:
import re
def delete_specific_line(file_path, pattern):
# 读取文件内容
content = read_file(file_path)
# 删除特定行
content = delete_line_by_regex(content, pattern)
# 写回文件内容
write_file(file_path, content)
def read_file(file_path):
with open(file_path, 'r') as file:
content = file.read()
return content
def delete_line_by_regex(content, pattern):
return re.sub(pattern, '', content)
def write_file(file_path, content):
with open(file_path, 'w') as file:
file.write(content)
示例用法
file_path = 'example.txt'
pattern = r'^.*specific string.*$\n'
delete_specific_line(file_path, pattern)
总结
在Python中删除特定的一行可以使用多种方法,包括文件操作、pandas库、正则表达式等。每种方法都有其适用的场景和优势。通过结合具体需求选择合适的方法,可以高效地完成删除特定行的操作。无论是处理小型文本文件还是大型数据集,这些方法都能提供灵活和强大的解决方案。
相关问答FAQs:
如何在Python中删除特定行的内容?
要在Python中删除特定行,您可以通过读取文件内容到一个列表中,然后根据行号或行内容删除所需的行,最后将修改后的内容写回文件。使用with open()
语句可以更方便地处理文件,确保文件在操作后会自动关闭。
使用Python删除特定行时,是否需要考虑文件的大小?
是的,文件的大小会影响处理效率。对于较大的文件,建议使用逐行读取的方法,避免将整个文件加载到内存中。例如,您可以使用for
循环逐行遍历文件,找到需要删除的行并跳过它,而不是将所有行存入列表。
有没有现成的Python库可以帮助删除特定行?
是的,有一些现成的库可以帮助处理文件操作,比如pandas
。通过pandas
,您可以将文件读取为DataFrame对象,使用简单的方法删除特定行,然后再将结果写回文件。这种方式特别适合处理结构化数据,如CSV文件。
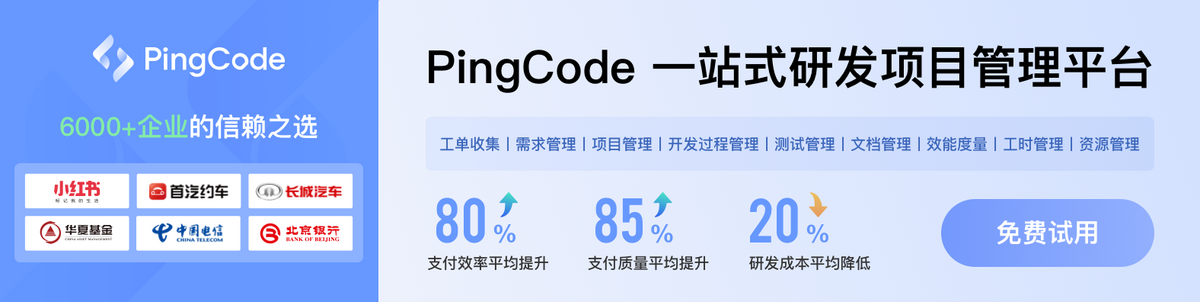