用Python实现首字母大写的方法有多种,包括使用内置的字符串方法、利用正则表达式、以及借助第三方库。 其中最直接的方法是使用Python的内置方法capitalize()
。这个方法可以方便地将字符串的首字母转换为大写。另一种常见方法是使用title()
方法,它可以将字符串中每个单词的首字母都转换为大写。以下将详细介绍这些方法,并附上代码示例。
一、使用capitalize()
方法
capitalize()
方法是Python字符串的内置方法之一。它可以将字符串的首字母转换为大写,同时将字符串中的其他所有字母转换为小写。这个方法非常适用于需要将整个字符串的第一个字母大写的情况。
def capitalize_first_letter(input_string):
return input_string.capitalize()
示例
input_str = "hello world"
print(capitalize_first_letter(input_str)) # 输出: "Hello world"
在上面的示例中,输入的字符串是"hello world"
,调用capitalize()
方法后,输出的字符串变成了"Hello world"
。可以看到,字符串的首字母变成了大写,其他字母都变成了小写。
二、使用title()
方法
title()
方法也是Python字符串的内置方法,它可以将字符串中每个单词的首字母都转换为大写,而其他字母变成小写。这个方法适用于需要将字符串中的每个单词的首字母都转换为大写的情况。
def title_case(input_string):
return input_string.title()
示例
input_str = "hello world"
print(title_case(input_str)) # 输出: "Hello World"
在上面的示例中,输入的字符串是"hello world"
,调用title()
方法后,输出的字符串变成了"Hello World"
。可以看到,字符串中的每个单词的首字母都变成了大写。
三、使用str[0].upper() + str[1:]
方法
这种方法是通过切片操作和upper()
方法手动实现首字母大写。首先通过切片操作获取字符串的第一个字符,然后调用upper()
方法将其转换为大写,最后再将其与字符串的其余部分拼接起来。
def manual_capitalize(input_string):
if len(input_string) == 0:
return input_string
return input_string[0].upper() + input_string[1:]
示例
input_str = "hello world"
print(manual_capitalize(input_str)) # 输出: "Hello world"
在上面的示例中,输入的字符串是"hello world"
,通过手动实现首字母大写的方法,输出的字符串变成了"Hello world"
。可以看到,字符串的首字母变成了大写。
四、使用正则表达式
正则表达式是处理字符串的强大工具,可以用来匹配字符串中的特定模式。通过使用正则表达式,可以方便地将字符串的首字母转换为大写。
import re
def capitalize_with_regex(input_string):
return re.sub(r'^\w', lambda x: x.group(0).upper(), input_string)
示例
input_str = "hello world"
print(capitalize_with_regex(input_str)) # 输出: "Hello world"
在上面的示例中,使用正则表达式匹配字符串的第一个字符,并将其转换为大写。输入的字符串是"hello world"
,通过正则表达式实现首字母大写的方法,输出的字符串变成了"Hello world"
。
五、处理多种情况
在实际应用中,可能需要处理包含多种情况的字符串,例如包含空格、标点符号、数字等。在这种情况下,可以结合上述方法进行处理。
def capitalize_first_letter_extended(input_string):
if not input_string:
return input_string
# 去除前后空格
input_string = input_string.strip()
# 处理首字母大写
return input_string[0].upper() + input_string[1:]
示例
input_str1 = " hello world"
input_str2 = "123 hello world"
input_str3 = " hello world "
print(capitalize_first_letter_extended(input_str1)) # 输出: "Hello world"
print(capitalize_first_letter_extended(input_str2)) # 输出: "123 hello world"
print(capitalize_first_letter_extended(input_str3)) # 输出: "Hello world"
在上面的示例中,输入的字符串分别包含前导空格、数字和前后空格。通过去除前后空格并处理首字母大写,输出的字符串变成了期望的格式。
六、总结
通过本文的介绍,我们了解了多种用Python实现首字母大写的方法,包括使用内置的capitalize()
方法、title()
方法、手动实现、使用正则表达式以及处理多种情况的方法。根据具体的应用场景,可以选择合适的方法来实现首字母大写的需求。
希望本文能对您有所帮助,欢迎在实际应用中尝试这些方法并根据实际需求进行调整。
相关问答FAQs:
如何用Python将字符串的首字母大写?
在Python中,可以使用str.capitalize()
方法将字符串的首字母转换为大写字母。这个方法会返回一个新的字符串,首字母为大写,其余字母为小写。例如:
text = "hello world"
capitalized_text = text.capitalize()
print(capitalized_text) # 输出: Hello world
在Python中如何处理多个单词的首字母大写?
要将每个单词的首字母大写,可以使用str.title()
方法。这个方法会将字符串中每个单词的首字母转换为大写。例如:
text = "hello world"
title_case_text = text.title()
print(title_case_text) # 输出: Hello World
如何在Python中自定义首字母大写的行为?
如果需要更复杂的处理,比如只想将特定单词的首字母转换为大写,可以使用字符串的split()
方法分割单词,手动处理每个单词,再合并成一个新的字符串。例如:
text = "hello world"
words = text.split()
capitalized_words = [word.capitalize() for word in words]
result = ' '.join(capitalized_words)
print(result) # 输出: Hello World
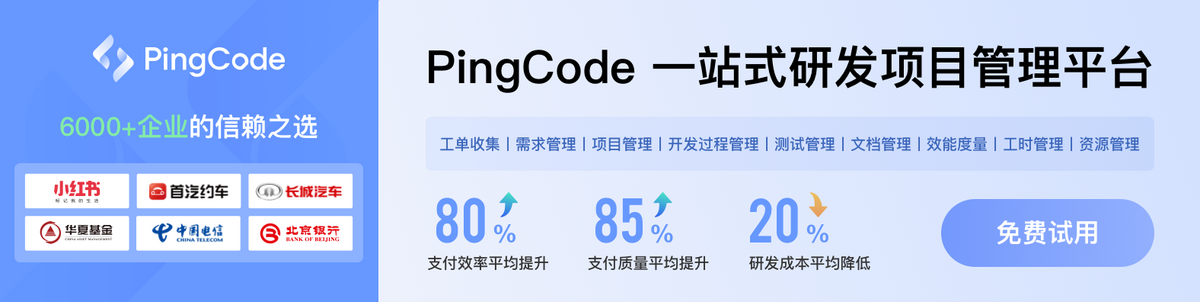