查看Python字符串的方法可以通过使用内置的dir()
函数、查看官方文档、使用help()
函数。其中,使用dir()
函数是最直接的方法,它可以列出字符串对象的所有属性和方法。通过使用 dir()
函数,我们可以快速了解字符串对象支持哪些方法,并利用这些方法进行各种字符串操作。接下来,我们将详细介绍这几种方法,并提供一些示例代码以帮助理解。
一、使用dir()
函数
dir()
函数是Python的内置函数之一,用于列出对象的属性和方法。对于字符串对象,它可以列出所有可用的方法。下面是一个示例:
string_methods = dir(str)
print(string_methods)
上述代码将输出一个包含所有字符串方法的列表。这些方法包括但不限于 split()
, join()
, replace()
, find()
, upper()
, lower()
, 等等。
示例说明
example_string = "Hello, World!"
print(dir(example_string))
运行上述代码,将会输出如下内容:
['__add__', '__class__', '__contains__', '__delattr__', '__dir__', '__doc__', '__eq__',
'__format__', '__ge__', '__getattribute__', '__getitem__', '__getnewargs__', '__gt__',
'__hash__', '__init__', '__init_subclass__', '__iter__', '__le__', '__len__', '__lt__',
'__mod__', '__mul__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__',
'__rmod__', '__rmul__', '__setattr__', '__sizeof__', '__str__', '__subclasshook__',
'capitalize', 'casefold', 'center', 'count', 'encode', 'endswith', 'expandtabs',
'find', 'format', 'format_map', 'index', 'isalnum', 'isalpha', 'isascii', 'isdecimal',
'isdigit', 'isidentifier', 'islower', 'isnumeric', 'isprintable', 'isspace', 'istitle',
'isupper', 'join', 'ljust', 'lower', 'lstrip', 'maketrans', 'partition', 'replace',
'rfind', 'rindex', 'rjust', 'rpartition', 'rsplit', 'rstrip', 'split', 'splitlines',
'startswith', 'strip', 'swapcase', 'title', 'translate', 'upper', 'zfill']
这些方法可以帮助我们在处理字符串时进行各种操作,例如替换字符、查找子字符串、转换大小写等。
二、查看官方文档
Python的官方文档是了解字符串方法的可靠来源。官方文档详细介绍了每个方法的用途、参数、返回值以及示例。访问Python的官方文档,可以获得最新的、最权威的信息。
访问官方文档
你可以通过访问以下链接查看Python字符串方法的官方文档:
在官方文档中,每个方法都有详细的说明和示例代码,帮助你理解如何使用这些方法。
三、使用help()
函数
help()
函数是Python提供的另一种查看对象信息的方式。它不仅可以列出对象的方法,还可以提供每个方法的详细描述。对于字符串对象,使用 help()
函数可以获得每个方法的详细信息。
示例使用
example_string = "Hello, World!"
help(example_string)
运行上述代码,将会输出如下内容:
Help on class str in module builtins:
class str(object)
| str(object='') -> str
| str(bytes_or_buffer[, encoding[, errors]]) -> str
|
| Create a new string object from the given object. If encoding or
| errors is specified, then the object must expose a data buffer
| that will be decoded using the given encoding and error handler.
| Otherwise, returns the result of object.__str__() (if defined)
| or repr(object).
| If no object is given, returns the empty string.
| ...
| Methods defined here:
|
| capitalize(self, /)
| Return a capitalized version of the string.
|
| More precisely, make the first character have upper case and the rest lower
| case.
|
| casefold(self, /)
| Return a version of the string suitable for caseless comparisons.
|
| center(self, width, fillchar=' ', /)
| Return a centered string of length width.
|
| Padding is done using the specified fill character (default is a space).
| ...
通过 help()
函数,我们可以深入了解每个方法的具体用法和示例,方便在实际编程中应用。
四、Python字符串常用方法详解
在了解如何查看字符串方法后,我们来详细介绍一些常用的字符串方法及其应用场景。
capitalize() 方法
capitalize() 方法用于将字符串的第一个字符转换为大写,其余字符转换为小写。
示例
example = "hello, world!"
capitalized_example = example.capitalize()
print(capitalized_example) # 输出: "Hello, world!"
upper() 和 lower() 方法
upper() 方法用于将字符串中的所有字符转换为大写,而 lower() 方法则将所有字符转换为小写。
示例
example = "Hello, World!"
upper_example = example.upper()
lower_example = example.lower()
print(upper_example) # 输出: "HELLO, WORLD!"
print(lower_example) # 输出: "hello, world!"
strip(), lstrip() 和 rstrip() 方法
strip() 方法用于移除字符串开头和结尾的空白字符,lstrip() 用于移除字符串开头的空白字符,rstrip() 用于移除字符串结尾的空白字符。
示例
example = " Hello, World! "
stripped_example = example.strip()
lstripped_example = example.lstrip()
rstripped_example = example.rstrip()
print(f"'{stripped_example}'") # 输出: "'Hello, World!'"
print(f"'{lstripped_example}'") # 输出: "'Hello, World! '"
print(f"'{rstripped_example}'") # 输出: "' Hello, World!'"
split() 和 join() 方法
split() 方法用于将字符串拆分为列表,join() 方法用于将列表中的元素连接成字符串。
示例
example = "Hello, World!"
split_example = example.split(", ")
print(split_example) # 输出: ['Hello', 'World!']
joined_example = ", ".join(split_example)
print(joined_example) # 输出: "Hello, World!"
replace() 方法
replace() 方法用于将字符串中的指定子字符串替换为另一个子字符串。
示例
example = "Hello, World!"
replaced_example = example.replace("World", "Python")
print(replaced_example) # 输出: "Hello, Python!"
五、总结
通过上述方法,我们可以轻松查看和使用Python字符串的各种方法。这些方法在字符串操作中非常有用,能够帮助我们实现各种文本处理需求。无论是使用 dir()
函数快速查看方法列表,还是通过官方文档和 help()
函数获取详细信息,都能够有效提升我们的编程效率和代码质量。希望本文能为你提供有价值的参考,帮助你更好地掌握Python字符串的操作技巧。
相关问答FAQs:
如何找到Python字符串的所有可用方法和属性?
可以使用内置的dir()
函数来获取一个字符串对象的所有可用方法和属性。例如,输入dir("your_string")
将返回一个列表,其中包含所有与字符串相关的方法和属性。这种方式可以帮助你快速了解可以对字符串执行的操作。
在Python中字符串方法的官方文档在哪里可以找到?
Python的官方文档提供了详细的字符串方法说明。你可以访问Python官网文档,在那里你会找到所有字符串方法的列表及其用法示例。这是学习和掌握字符串操作的重要资源。
如何有效利用Python字符串方法进行文本处理?
Python字符串方法提供了丰富的文本处理功能,例如strip()
去除空格、replace()
替换子串、split()
分割字符串等。理解每个方法的作用及其参数,可以帮助你在处理文本数据时提高效率。通过查看具体的例子,可以更好地掌握这些方法的实际应用。
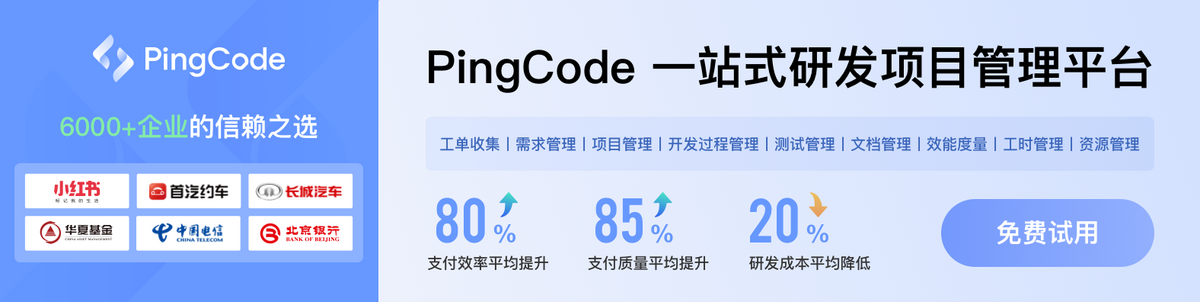