在Python中,可以通过多种方法将字符串的轮廓分清,主要包括:使用正则表达式、字符串方法(如split、join等)、以及第三方库(如nltk、spacy等)。其中,正则表达式是一种强大的工具,可以通过模式匹配来精确提取和分割字符串。字符串方法则提供了简单易用的内置方法来处理字符串。第三方库提供了更高级的自然语言处理功能,可以处理复杂的文本分析任务。下面将详细介绍这些方法。
一、使用正则表达式
正则表达式(Regular Expression,简称regex)是一种用来匹配字符串中字符组合的模式。Python 的 re
模块提供了对正则表达式的支持。
1.1 基本用法
正则表达式的基本功能包括匹配、查找、替换等。以下是一些常见的正则表达式操作:
import re
匹配字符串中的数字
pattern = r'\d+'
string = "The price is 100 dollars"
result = re.findall(pattern, string)
print(result) # 输出: ['100']
替换字符串中的数字
pattern = r'\d+'
replacement = "XXX"
result = re.sub(pattern, replacement, string)
print(result) # 输出: "The price is XXX dollars"
1.2 分割字符串
正则表达式还可以用来分割字符串。例如,将一个句子按照空格分割成单词:
import re
pattern = r'\s+'
string = "This is a sample sentence."
result = re.split(pattern, string)
print(result) # 输出: ['This', 'is', 'a', 'sample', 'sentence.']
1.3 提取特定模式
正则表达式可以用来提取特定模式的子字符串。例如,提取电子邮件地址:
import re
pattern = r'[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+\.[a-zA-Z0-9-.]+'
string = "Please contact us at support@example.com for further information."
result = re.findall(pattern, string)
print(result) # 输出: ['support@example.com']
二、使用字符串方法
Python 提供了一些内置的字符串方法,能够方便地对字符串进行操作。
2.1 split方法
split
方法可以将字符串按照指定的分隔符分割成一个列表:
string = "apple,banana,cherry"
result = string.split(',')
print(result) # 输出: ['apple', 'banana', 'cherry']
2.2 join方法
join
方法可以将一个列表中的元素连接成一个字符串,指定的分隔符会插入到每个元素之间:
words = ['apple', 'banana', 'cherry']
result = ','.join(words)
print(result) # 输出: "apple,banana,cherry"
2.3 strip方法
strip
方法可以去除字符串两端的空白字符:
string = " hello world "
result = string.strip()
print(result) # 输出: "hello world"
三、使用第三方库
Python 有许多第三方库可以用于文本处理和自然语言处理,这些库提供了高级的功能和更强大的处理能力。
3.1 nltk库
Natural Language Toolkit(nltk)是一个用于处理自然语言文本的库。它提供了丰富的工具和数据集来处理文本。
安装nltk库:
pip install nltk
使用示例:
import nltk
nltk.download('punkt')
from nltk.tokenize import word_tokenize
string = "This is a sample sentence."
result = word_tokenize(string)
print(result) # 输出: ['This', 'is', 'a', 'sample', 'sentence', '.']
3.2 spacy库
spaCy 是一个用于高级自然语言处理的库。它速度快,功能强大,适用于大规模文本处理。
安装spacy库:
pip install spacy
python -m spacy download en_core_web_sm
使用示例:
import spacy
nlp = spacy.load("en_core_web_sm")
doc = nlp("This is a sample sentence.")
for token in doc:
print(token.text) # 输出: This is a sample sentence .
3.3 TextBlob库
TextBlob 是一个简单易用的文本处理库,适用于基础的自然语言处理任务。
安装TextBlob库:
pip install textblob
使用示例:
from textblob import TextBlob
string = "This is a sample sentence."
blob = TextBlob(string)
result = blob.words
print(result) # 输出: ['This', 'is', 'a', 'sample', 'sentence']
四、综合应用
在实际项目中,我们可能需要综合使用上述方法来处理复杂的文本任务。以下是一个综合示例,展示如何使用正则表达式、字符串方法和第三方库来处理一个复杂的文本任务。
示例任务:从文本中提取电子邮件地址和电话号码
步骤1:使用正则表达式提取电子邮件地址和电话号码
import re
text = """
Please contact us at support@example.com or sales@example.com.
You can also reach us at (123) 456-7890 or +1-800-555-1234.
"""
email_pattern = r'[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+\.[a-zA-Z0-9-.]+'
phone_pattern = r'\+?\d[\d -]{8,12}\d'
emails = re.findall(email_pattern, text)
phones = re.findall(phone_pattern, text)
print("Emails:", emails) # 输出: Emails: ['support@example.com', 'sales@example.com']
print("Phones:", phones) # 输出: Phones: ['(123) 456-7890', '+1-800-555-1234']
步骤2:使用字符串方法清理提取的电话号码
cleaned_phones = [phone.replace(' ', '').replace('-', '').replace('(', '').replace(')', '') for phone in phones]
print("Cleaned Phones:", cleaned_phones) # 输出: Cleaned Phones: ['1234567890', '18005551234']
步骤3:使用nltk进行词汇分析
import nltk
nltk.download('punkt')
from nltk.tokenize import word_tokenize
text = "Please contact us at support@example.com or sales@example.com."
tokens = word_tokenize(text)
print("Tokens:", tokens) # 输出: Tokens: ['Please', 'contact', 'us', 'at', 'support', '@', 'example.com', 'or', 'sales', '@', 'example.com', '.']
步骤4:使用spacy进行命名实体识别(NER)
import spacy
nlp = spacy.load("en_core_web_sm")
doc = nlp(text)
for ent in doc.ents:
print(ent.text, ent.label_) # 输出: support@example.com ORG, sales@example.com ORG
通过上述步骤,我们可以看到如何综合使用正则表达式、字符串方法和第三方库来处理复杂的文本任务。这些方法在实际项目中非常实用,可以帮助我们高效地处理和分析文本数据。
相关问答FAQs:
如何在Python中识别字符串的不同轮廓或模式?
在Python中,可以使用正则表达式(regex)来识别字符串的不同轮廓或模式。通过re
模块,可以创建复杂的模式匹配,以提取或识别特定的字符串特征。例如,可以通过re.findall()
方法找到所有符合某种模式的子字符串。这使得提取信息变得简单高效。
在Python中,如何处理字符串的不同格式或结构?
处理字符串的不同格式可以使用字符串方法,如split()
、join()
、strip()
等。这些方法帮助分离、合并或清理字符串,使得数据处理更加灵活。此外,利用字典和列表等数据结构,可以将字符串的不同部分分组,从而更清晰地展现其轮廓。
如何使用Python对字符串进行分类和排序?
在Python中,可以使用列表推导式和内置的sorted()
函数对字符串进行分类和排序。通过定义自定义的排序键,可以根据字符串的某些特征(如长度、字母顺序等)对它们进行排序。使用这些方法,可以有效地管理和分析字符串数据,以便提取所需的轮廓信息。
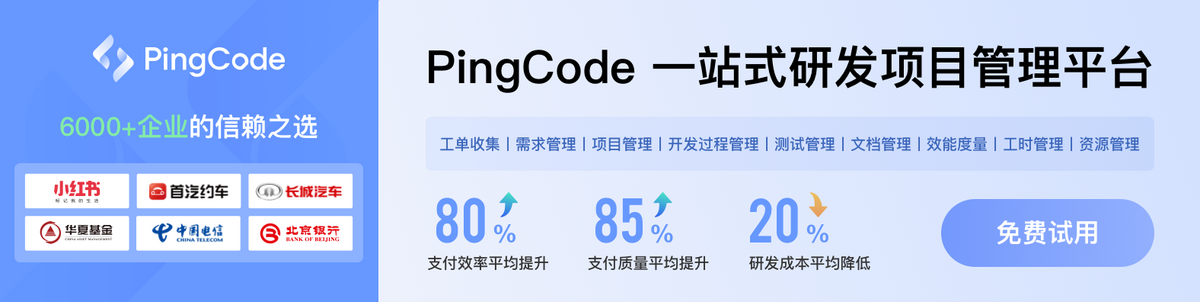