要用Python实现3D旋转,可以使用多个库来完成这项任务,包括Matplotlib、Pygame、PyOpenGL等。 在这些库中,Matplotlib因其简单易用且功能强大,非常适合初学者。通过Matplotlib,可以轻松创建并旋转3D图形。 在本文中,我们将详细介绍如何使用Matplotlib、Pygame和PyOpenGL来实现3D旋转。
一、MATPLOTLIB实现3D旋转
Matplotlib是一个广泛使用的绘图库,支持2D和3D图形的绘制。使用Matplotlib,我们可以创建一个3D图形并通过改变视角来实现旋转效果。
1. 安装Matplotlib
首先,确保你已经安装了Matplotlib。如果没有安装,可以使用以下命令安装:
pip install matplotlib
2. 导入必要的库
在你的Python脚本中,导入Matplotlib和其他必要的库:
import matplotlib.pyplot as plt
import numpy as np
from mpl_toolkits.mplot3d import Axes3D
3. 创建数据
接下来,创建一些3D数据来绘制。例如,我们可以创建一个3D正弦波:
x = np.linspace(-5, 5, 100)
y = np.linspace(-5, 5, 100)
x, y = np.meshgrid(x, y)
z = np.sin(np.sqrt(x<strong>2 + y</strong>2))
4. 绘制3D图形
使用Matplotlib的plot_surface
函数来绘制3D图形:
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ax.plot_surface(x, y, z, cmap='viridis')
5. 实现旋转
可以使用Matplotlib的动画功能来实现3D旋转。首先,导入FuncAnimation
:
from matplotlib.animation import FuncAnimation
然后,创建一个旋转函数并使用FuncAnimation
进行动画处理:
def rotate(angle):
ax.view_init(azim=angle)
ani = FuncAnimation(fig, rotate, frames=np.arange(0, 360, 1), interval=50)
plt.show()
这样,你就可以看到一个3D图形在不断旋转。
二、PYGAME实现3D旋转
Pygame是一个跨平台的Python库,专门用于开发视频游戏。它包含图像、声音等模块,可以用来实现3D图形旋转。虽然Pygame主要用于2D游戏开发,但它也可以通过一些技巧来实现3D图形。
1. 安装Pygame
首先,确保你已经安装了Pygame。如果没有安装,可以使用以下命令安装:
pip install pygame
2. 导入必要的库
在你的Python脚本中,导入Pygame和其他必要的库:
import pygame
import numpy as np
3. 初始化Pygame
初始化Pygame并设置显示窗口:
pygame.init()
screen = pygame.display.set_mode((800, 600))
pygame.display.set_caption("3D Rotation")
clock = pygame.time.Clock()
4. 创建3D数据
创建一些3D数据,例如一个立方体:
vertices = np.array([
[-1, -1, -1],
[1, -1, -1],
[1, 1, -1],
[-1, 1, -1],
[-1, -1, 1],
[1, -1, 1],
[1, 1, 1],
[-1, 1, 1]
])
edges = [
(0, 1), (1, 2), (2, 3), (3, 0),
(4, 5), (5, 6), (6, 7), (7, 4),
(0, 4), (1, 5), (2, 6), (3, 7)
]
5. 实现旋转
创建一个旋转函数并在主循环中调用:
def rotate(vertices, angle_x, angle_y):
rotation_x = np.array([
[1, 0, 0],
[0, np.cos(angle_x), -np.sin(angle_x)],
[0, np.sin(angle_x), np.cos(angle_x)]
])
rotation_y = np.array([
[np.cos(angle_y), 0, np.sin(angle_y)],
[0, 1, 0],
[-np.sin(angle_y), 0, np.cos(angle_y)]
])
return np.dot(vertices, rotation_x).dot(rotation_y)
angle_x, angle_y = 0, 0
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
angle_x += 0.01
angle_y += 0.01
rotated_vertices = rotate(vertices, angle_x, angle_y)
screen.fill((0, 0, 0))
for edge in edges:
points = []
for vertex in edge:
x, y = rotated_vertices[vertex][:2]
x = int(x * 100) + 400
y = int(y * 100) + 300
points.append((x, y))
pygame.draw.line(screen, (255, 255, 255), points[0], points[1])
pygame.display.flip()
clock.tick(60)
pygame.quit()
通过这种方式,你可以使用Pygame实现3D图形的旋转。
三、PYOPENGL实现3D旋转
PyOpenGL是Python的OpenGL绑定,提供了使用OpenGL进行3D图形编程的接口。使用PyOpenGL,可以实现更复杂和高效的3D图形渲染。
1. 安装PyOpenGL
首先,确保你已经安装了PyOpenGL。如果没有安装,可以使用以下命令安装:
pip install PyOpenGL PyOpenGL_accelerate
2. 导入必要的库
在你的Python脚本中,导入PyOpenGL和其他必要的库:
from OpenGL.GL import *
from OpenGL.GLUT import *
from OpenGL.GLU import *
import numpy as np
3. 初始化PyOpenGL
初始化PyOpenGL并设置显示窗口:
def init():
glClearColor(0.0, 0.0, 0.0, 1.0)
glEnable(GL_DEPTH_TEST)
def display():
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT)
glLoadIdentity()
gluLookAt(0, 0, 5, 0, 0, 0, 0, 1, 0)
# 旋转和绘制立方体
glRotatef(angle, 1, 1, 1)
draw_cube()
glutSwapBuffers()
def draw_cube():
vertices = [
[1, 1, -1], [1, -1, -1], [-1, -1, -1], [-1, 1, -1],
[1, 1, 1], [1, -1, 1], [-1, -1, 1], [-1, 1, 1]
]
edges = [
(0, 1), (1, 2), (2, 3), (3, 0),
(4, 5), (5, 6), (6, 7), (7, 4),
(0, 4), (1, 5), (2, 6), (3, 7)
]
glBegin(GL_LINES)
for edge in edges:
for vertex in edge:
glVertex3fv(vertices[vertex])
glEnd()
4. 实现旋转
创建一个旋转函数并在主循环中调用:
angle = 0
def update(value):
global angle
angle += 1
glutPostRedisplay()
glutTimerFunc(16, update, 0)
def main():
glutInit()
glutInitDisplayMode(GLUT_RGBA | GLUT_DOUBLE | GLUT_DEPTH)
glutInitWindowSize(800, 600)
glutCreateWindow("3D Rotation")
init()
glutDisplayFunc(display)
glutTimerFunc(16, update, 0)
glutMainLoop()
if __name__ == "__main__":
main()
通过这种方式,你可以使用PyOpenGL实现3D图形的旋转。
总结:
使用Python实现3D旋转的方法有很多,每种方法都有其独特的优势。Matplotlib适合绘制简单的3D图形并实现基本的旋转效果,Pygame虽然主要用于2D游戏开发,但也可以通过一些技巧实现3D图形,PyOpenGL则提供了更加高效和灵活的3D图形编程接口。根据你的需求,选择合适的工具可以帮助你更好地实现3D旋转效果。
相关问答FAQs:
用Python实现3D旋转的基本步骤是什么?
要在Python中实现3D旋转,首先需要选择一个适合的库,例如Matplotlib、Pygame或OpenGL。接下来,您需要定义要旋转的3D对象或图形,设置旋转中心和旋转角度。最后,通过更新图形的坐标和使用绘图函数来可视化旋转效果。
有哪些常用的Python库可以用来创建3D旋转效果?
在Python中,常用的库包括Matplotlib,它提供了简单的3D绘图功能;Pygame,适合游戏开发并能处理3D图形;以及OpenGL,这是一个强大的图形库,适合创建复杂的3D场景。您还可以考虑使用VPython,它专门用于3D编程,非常易于上手。
如何优化3D旋转的性能和流畅性?
要提高3D旋转的性能,可以减少绘制的多边形数量,简化模型的复杂度。此外,确保使用合适的渲染技术,比如使用双缓冲来减少闪烁。调整图形的更新频率,确保它与显示器的刷新率相匹配,也可以提升流畅性。此外,利用GPU加速可以显著提高性能。
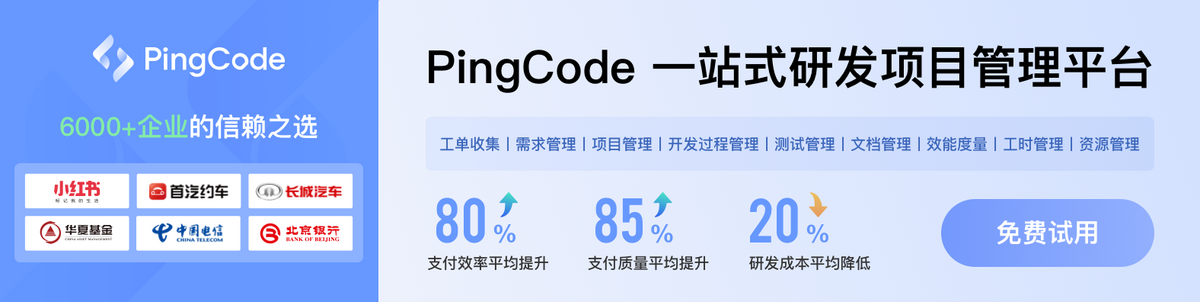