Python批处理Excel文件夹的方法包括使用pandas、os库、glob库进行文件操作、批量读取与处理。 其中,使用pandas库可以方便地读取和写入Excel文件,os库可以处理文件路径,而glob库可以批量获取指定文件夹中的文件列表。下面将详细介绍如何使用这些工具来批处理Excel文件。
一、设置环境
在开始之前,我们需要确保已经安装了所需的Python库。可以使用以下命令安装pandas库:
pip install pandas
二、导入所需库
在处理Excel文件之前,我们需要先导入相关的Python库:
import pandas as pd
import os
import glob
三、获取Excel文件列表
使用glob
库,我们可以批量获取指定文件夹中的所有Excel文件:
def get_excel_files(directory):
files = glob.glob(os.path.join(directory, "*.xlsx"))
return files
通过此函数,我们可以获取指定目录下所有扩展名为.xlsx
的Excel文件。
四、读取Excel文件并处理
接下来,我们可以逐个读取Excel文件,并对其内容进行处理。假设我们要对每个Excel文件的内容进行某种数据处理操作,例如对某些列进行求和,我们可以这样做:
def process_excel_file(file_path):
df = pd.read_excel(file_path)
# 进行数据处理操作,例如对某些列求和
df['Total'] = df.sum(axis=1)
return df
五、批量处理Excel文件
我们可以将上述步骤结合起来,创建一个函数来批量处理Excel文件并将结果保存到新的Excel文件中:
def batch_process_excel_files(directory, output_directory):
files = get_excel_files(directory)
for file in files:
df = process_excel_file(file)
# 获取文件名
file_name = os.path.basename(file)
# 保存处理后的数据到新的Excel文件
output_path = os.path.join(output_directory, file_name)
df.to_excel(output_path, index=False)
六、执行批处理操作
最后,我们可以调用批处理函数来处理指定文件夹中的所有Excel文件,并将结果保存到指定的输出文件夹中:
input_directory = "path/to/input/directory"
output_directory = "path/to/output/directory"
batch_process_excel_files(input_directory, output_directory)
批处理Excel文件实战案例
接下来,让我们通过一个具体的实战案例来演示如何批处理Excel文件。假设我们有一个包含多个Excel文件的文件夹,每个文件中都有一个名为"Sales"的工作表。我们的目标是计算每个文件中销售数据的总和,并将结果保存到新的Excel文件中。
1. 设置环境
确保已经安装了所需的Python库:
pip install pandas
2. 导入所需库
import pandas as pd
import os
import glob
3. 获取Excel文件列表
def get_excel_files(directory):
files = glob.glob(os.path.join(directory, "*.xlsx"))
return files
4. 读取Excel文件并处理
def process_excel_file(file_path):
df = pd.read_excel(file_path, sheet_name="Sales")
# 计算销售数据的总和
df['TotalSales'] = df.sum(axis=1)
return df
5. 批量处理Excel文件
def batch_process_excel_files(directory, output_directory):
files = get_excel_files(directory)
for file in files:
df = process_excel_file(file)
# 获取文件名
file_name = os.path.basename(file)
# 保存处理后的数据到新的Excel文件
output_path = os.path.join(output_directory, file_name)
df.to_excel(output_path, index=False)
6. 执行批处理操作
input_directory = "path/to/input/directory"
output_directory = "path/to/output/directory"
batch_process_excel_files(input_directory, output_directory)
通过上述步骤,我们可以方便地批量处理Excel文件夹中的所有文件,并将处理结果保存到新的Excel文件中。无论是处理销售数据、财务数据还是其他类型的数据,这种方法都非常高效和实用。
处理多个工作表的Excel文件
有时,我们可能需要处理包含多个工作表的Excel文件。在这种情况下,我们可以使用pd.ExcelFile
类来读取整个Excel文件,并分别处理每个工作表。下面是一个示例:
def process_excel_file(file_path):
excel_file = pd.ExcelFile(file_path)
processed_data = {}
for sheet_name in excel_file.sheet_names:
df = pd.read_excel(file_path, sheet_name=sheet_name)
# 进行数据处理操作,例如对某些列求和
df['Total'] = df.sum(axis=1)
processed_data[sheet_name] = df
return processed_data
def batch_process_excel_files(directory, output_directory):
files = get_excel_files(directory)
for file in files:
processed_data = process_excel_file(file)
# 获取文件名
file_name = os.path.basename(file)
# 保存处理后的数据到新的Excel文件
with pd.ExcelWriter(os.path.join(output_directory, file_name)) as writer:
for sheet_name, df in processed_data.items():
df.to_excel(writer, sheet_name=sheet_name, index=False)
通过这种方法,我们可以分别处理Excel文件中的每个工作表,并将结果保存到新的Excel文件中。
结论
通过使用Python的pandas库和os、glob库,我们可以非常高效地批处理Excel文件夹中的所有文件。无论是读取、处理还是保存Excel文件,这些工具都提供了极大的便利和灵活性。希望通过本文的详细介绍和实战案例,能够帮助您更好地理解和应用Python批处理Excel文件的方法。
相关问答FAQs:
如何使用Python批量处理多个Excel文件?
Python提供了许多强大的库来处理Excel文件,如pandas和openpyxl。通过编写脚本,您可以轻松地遍历一个文件夹中的所有Excel文件,执行数据清理、格式转换或数据分析等操作。例如,您可以使用pandas的read_excel
函数读取每个文件,对数据进行处理后,再使用to_excel
函数将结果保存为新的Excel文件。
是否需要安装额外的库来处理Excel文件?
是的,处理Excel文件通常需要安装pandas和openpyxl等库。可以通过pip命令轻松安装,例如pip install pandas openpyxl
。此外,根据您的需求,可能还需要其他库来处理特定的数据分析任务或文件格式转换。
如何处理不同格式的Excel文件?
在Python中,您可以使用pandas库来处理多种Excel格式,包括.xls
和.xlsx
。在读取文件时,只需指定文件路径,pandas会自动识别文件格式。对于处理不同工作表或特定区域的数据,您可以使用sheet_name
参数指定所需的工作表,或者通过usecols
参数选择特定的列。这样,您可以灵活地从多种格式的Excel文件中提取和处理数据。
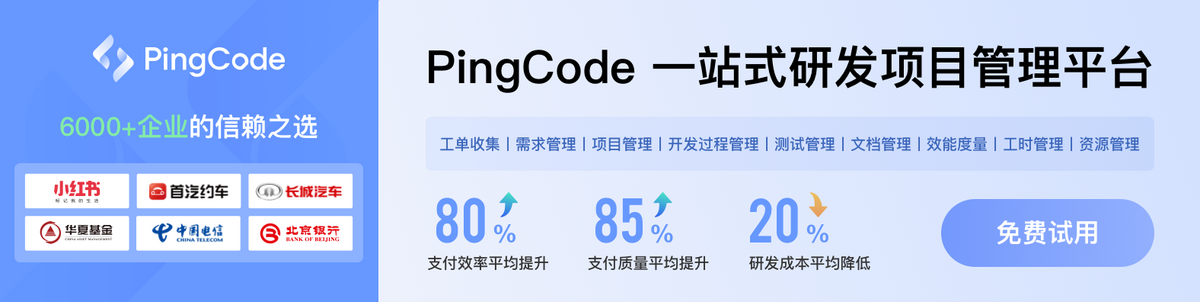