如何用Python爬取微博指数
要用Python爬取微博指数,可以采取以下几种方法:使用微博开放API、模拟登录微博并获取相关数据、通过第三方工具或库,如Selenium或BeautifulSoup。使用微博开放API、模拟登录微博、通过第三方工具(如Selenium),这些方法能够帮助你有效地获取微博指数数据。接下来,我们详细介绍其中一种方法:使用Selenium模拟浏览器操作。
一、使用微博开放API
微博开放API提供了丰富的接口,可以直接获取微博上的数据。首先,你需要在微博开放平台上注册并申请API权限。以下是使用微博开放API的步骤:
- 注册并申请API权限:在微博开放平台上创建一个应用,获取App Key和App Secret。
- 获取Access Token:通过OAuth2.0授权,获取Access Token。
- 调用API接口:使用获取的Access Token,调用微博开放API获取微博指数相关数据。
示例代码:
import requests
APP_KEY = 'your_app_key'
APP_SECRET = 'your_app_secret'
REDIRECT_URI = 'your_redirect_uri'
获取Access Token
def get_access_token():
auth_url = f"https://api.weibo.com/oauth2/authorize?client_id={APP_KEY}&response_type=code&redirect_uri={REDIRECT_URI}"
print(f"Please go to this URL and authorize the app: {auth_url}")
authorization_code = input("Enter the authorization code: ")
token_url = "https://api.weibo.com/oauth2/access_token"
data = {
"client_id": APP_KEY,
"client_secret": APP_SECRET,
"grant_type": "authorization_code",
"code": authorization_code,
"redirect_uri": REDIRECT_URI
}
response = requests.post(token_url, data=data)
return response.json()["access_token"]
获取微博指数
def get_weibo_index(keyword, access_token):
url = f"https://api.weibo.com/2/search/topics.json?q={keyword}&access_token={access_token}"
response = requests.get(url)
return response.json()
access_token = get_access_token()
keyword = "your_keyword"
weibo_index = get_weibo_index(keyword, access_token)
print(weibo_index)
二、模拟登录微博
由于微博的反爬机制较强,通常需要模拟登录获取相关数据。以下是使用Selenium模拟登录并爬取微博指数的步骤:
- 安装Selenium和浏览器驱动:在本地安装Selenium库和对应的浏览器驱动(如ChromeDriver)。
- 登录微博:使用Selenium模拟浏览器操作,自动登录微博。
- 获取微博指数数据:登录后,访问微博指数页面并获取相关数据。
示例代码:
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
import time
设置浏览器驱动
driver = webdriver.Chrome(executable_path='/path/to/chromedriver')
打开微博登录页面
driver.get('https://weibo.com/login.php')
输入用户名和密码并登录
username = driver.find_element(By.NAME, 'username')
password = driver.find_element(By.NAME, 'password')
username.send_keys('your_username')
password.send_keys('your_password')
password.send_keys(Keys.RETURN)
等待页面加载
time.sleep(5)
访问微博指数页面
driver.get('https://data.weibo.com/index')
输入关键词并获取指数数据
keyword_input = driver.find_element(By.ID, 'search-input')
keyword_input.send_keys('your_keyword')
keyword_input.send_keys(Keys.RETURN)
等待页面加载
time.sleep(5)
获取微博指数数据
index_data = driver.find_element(By.CLASS_NAME, 'index-data').text
print(index_data)
关闭浏览器
driver.quit()
三、通过第三方工具(如Selenium)
Selenium是一个强大的浏览器自动化工具,能够模拟用户操作,适用于处理复杂的JavaScript渲染页面。以下是使用Selenium爬取微博指数的步骤:
- 安装Selenium和浏览器驱动:在本地安装Selenium库和对应的浏览器驱动(如ChromeDriver)。
- 模拟浏览器操作:使用Selenium模拟浏览器操作,访问微博指数页面并获取相关数据。
示例代码:
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
import time
设置浏览器驱动
driver = webdriver.Chrome(executable_path='/path/to/chromedriver')
打开微博指数页面
driver.get('https://data.weibo.com/index')
输入关键词并获取指数数据
keyword_input = driver.find_element(By.ID, 'search-input')
keyword_input.send_keys('your_keyword')
keyword_input.send_keys(Keys.RETURN)
等待页面加载
time.sleep(5)
获取微博指数数据
index_data = driver.find_element(By.CLASS_NAME, 'index-data').text
print(index_data)
关闭浏览器
driver.quit()
四、数据清洗和存储
在获取到微博指数数据后,可以对数据进行清洗和存储。常用的方法包括:
- 数据清洗:去除无关字符、处理缺失值等。
- 数据存储:将清洗后的数据存储到数据库或文件中(如CSV、Excel)。
示例代码:
import pandas as pd
示例微博指数数据
index_data = [
{"date": "2023-01-01", "index": 100},
{"date": "2023-01-02", "index": 110},
{"date": "2023-01-03", "index": 105},
]
将数据转换为DataFrame
df = pd.DataFrame(index_data)
数据清洗
df['index'] = df['index'].astype(int)
数据存储
df.to_csv('weibo_index.csv', index=False)
五、数据分析和可视化
在获取并清洗存储微博指数数据后,可以进行数据分析和可视化。常用的方法包括:
- 数据分析:使用统计方法或机器学习算法对数据进行分析。
- 数据可视化:使用Matplotlib、Seaborn等库进行数据可视化。
示例代码:
import pandas as pd
import matplotlib.pyplot as plt
读取存储的微博指数数据
df = pd.read_csv('weibo_index.csv')
数据分析
print(df.describe())
数据可视化
plt.plot(df['date'], df['index'])
plt.xlabel('Date')
plt.ylabel('Index')
plt.title('Weibo Index Over Time')
plt.show()
通过以上方法,可以用Python爬取微博指数数据,并进行数据清洗、存储、分析和可视化。根据实际需求选择合适的方法,确保数据的准确性和完整性。
相关问答FAQs:
如何使用Python爬取微博指数的基本步骤是什么?
爬取微博指数通常需要使用Python的网络请求库,如Requests,以及解析库,如BeautifulSoup或lxml。首先,你需要分析微博指数页面的结构,找到需要提取的数据元素。接下来,使用Requests库向微博指数的URL发送HTTP请求,获取页面内容。然后,使用解析库从HTML中提取所需数据,最后将结果保存到CSV或数据库中以便后续分析。
我需要掌握哪些Python库来实现微博指数的爬取?
在爬取微博指数时,最常用的Python库包括Requests和BeautifulSoup。Requests用于发送网络请求和处理响应,而BeautifulSoup则用于解析HTML和XML文档,方便提取所需信息。此外,Pandas库也很有用,它可以帮助你将爬取的数据整理成表格形式,便于分析和展示。
如何处理爬取过程中可能遇到的反爬虫机制?
为了应对微博的反爬虫机制,可以采取多种策略。首先,可以使用代理IP来隐藏真实IP地址,从而减少被封禁的风险。其次,合理设置请求间隔,模拟人类行为,避免发送过于频繁的请求。此外,使用浏览器自动化工具如Selenium,可以模拟真实用户的操作,从而绕过一些简单的反爬虫措施。最后,保持对网页结构变化的关注,及时调整爬虫代码。
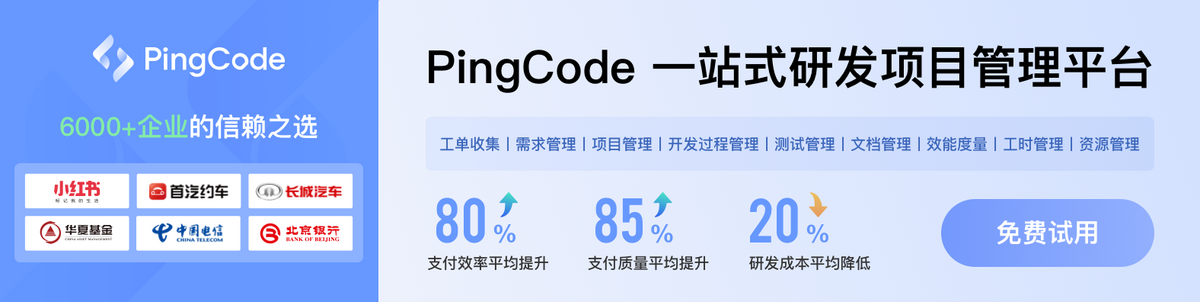