Python中输入字符串的长度,可以使用内置函数len()。使用len()函数可以方便地获取字符串的长度。
例如,假设你有一个字符串"Hello, world!",你想知道它的长度,可以使用如下代码:
string = "Hello, world!"
length = len(string)
print(f"The length of the string is: {length}")
上述代码将输出:The length of the string is: 13
。这是因为字符串"Hello, world!"包含13个字符,包括空格和标点符号。
为了更详细地描述如何在Python中处理字符串长度的相关内容,接下来我们将深入讨论字符串的基本操作、常见的字符串处理技巧,以及实际应用中的一些示例。
一、字符串的基本操作
1、定义字符串
在Python中,字符串可以使用单引号('
)、双引号("
)或三引号('''
或 """
)来定义。三引号通常用于定义多行字符串。
single_quote_string = 'Hello, world!'
double_quote_string = "Hello, world!"
multi_line_string = '''Hello,
world!'''
2、获取字符串长度
如前所述,获取字符串长度最常用的方法是使用内置函数len()
:
string = "Hello, world!"
length = len(string)
3、字符串连接
字符串可以使用加号(+
)进行连接:
str1 = "Hello"
str2 = "world"
combined = str1 + ", " + str2 + "!"
4、字符串重复
可以使用乘号(*
)来重复一个字符串:
repeat_str = "Hello" * 3
二、常见的字符串处理技巧
1、字符串切片
字符串切片是指从字符串中获取子字符串的方法。可以使用冒号(:
)来指定切片的起始和结束位置:
string = "Hello, world!"
substring = string[0:5] # 结果是"Hello"
2、字符串查找
可以使用find()
方法查找子字符串的位置:
string = "Hello, world!"
position = string.find("world") # 结果是7
3、字符串替换
可以使用replace()
方法替换字符串中的某个子字符串:
string = "Hello, world!"
new_string = string.replace("world", "Python") # 结果是"Hello, Python!"
4、字符串拆分
可以使用split()
方法将字符串拆分为列表:
string = "Hello, world!"
words = string.split(", ") # 结果是["Hello", "world!"]
5、去除字符串中的空白
可以使用strip()
方法去除字符串两端的空白字符:
string = " Hello, world! "
trimmed_string = string.strip() # 结果是"Hello, world!"
三、字符串处理的高级应用
1、格式化字符串
在Python中,有多种方法可以格式化字符串。最常用的方法之一是使用format()
方法:
name = "Alice"
age = 30
formatted_string = "My name is {} and I am {} years old.".format(name, age)
或者使用f-string(格式化字符串字面量),这是在Python 3.6引入的一种更简洁的方法:
name = "Alice"
age = 30
formatted_string = f"My name is {name} and I am {age} years old."
2、正则表达式
正则表达式是一个强大的工具,用于匹配复杂的字符串模式。在Python中,可以使用re
模块进行正则表达式操作:
import re
pattern = r"\b[A-Za-z]+\b"
string = "Hello, world!"
matches = re.findall(pattern, string) # 结果是["Hello", "world"]
3、字符串编码和解码
在处理文本数据时,可能需要进行字符串的编码和解码操作。可以使用encode()
和decode()
方法:
string = "Hello, world!"
encoded_string = string.encode("utf-8")
decoded_string = encoded_string.decode("utf-8")
4、使用字符串作为数据结构
字符串不仅仅是文本的集合,在某些情况下,字符串也可以用作数据结构。例如,可以将字符串视为字符数组,通过索引访问单个字符:
string = "Hello, world!"
first_char = string[0] # 结果是"H"
last_char = string[-1] # 结果是"!"
5、字符串的不可变性
在Python中,字符串是不可变的,这意味着一旦创建,字符串的内容就不能被更改。如果需要修改字符串的某一部分,可以创建一个新的字符串:
string = "Hello, world!"
new_string = string[:7] + "Python!" # 结果是"Hello, Python!"
四、字符串处理的实际应用
1、处理用户输入
在许多应用程序中,处理用户输入是一个常见的任务。可以使用input()
函数获取用户输入,并使用字符串操作函数对输入进行处理:
user_input = input("Enter your name: ")
formatted_input = user_input.strip().title()
print(f"Hello, {formatted_input}!")
2、文件操作
在处理文本文件时,字符串操作是必不可少的。可以使用内置的文件操作函数读取和写入文件:
# 读取文件
with open("example.txt", "r") as file:
content = file.read()
print(content)
写入文件
with open("example.txt", "w") as file:
file.write("Hello, world!")
3、数据清理和预处理
在数据科学和机器学习领域,数据清理和预处理是非常重要的步骤。字符串操作在这过程中起着关键作用。例如,可以使用字符串操作函数清理数据中的噪声,标准化文本格式等:
raw_data = [" Alice ", "Bob ", " Charlie"]
cleaned_data = [name.strip().title() for name in raw_data]
4、生成报告和文档
在生成报告和文档时,字符串操作也是必不可少的。可以使用字符串格式化函数生成动态内容,并将结果写入文件:
report_template = """
Report
======
Name: {name}
Age: {age}
"""
name = "Alice"
age = 30
report = report_template.format(name=name, age=age)
with open("report.txt", "w") as file:
file.write(report)
5、网络编程
在网络编程中,处理协议和数据传输时,经常需要对字符串进行编码、解码和解析。例如,处理HTTP请求和响应头部信息时,字符串操作是非常重要的:
import requests
response = requests.get("https://api.example.com/data")
headers = response.headers
content_type = headers.get("Content-Type")
if "application/json" in content_type:
data = response.json()
print(data)
通过以上详细介绍,我们可以看到字符串操作在Python编程中无处不在。掌握字符串的基本操作和高级技巧,可以帮助我们更高效地处理各种文本数据,解决实际问题。希望这篇文章对你在Python中处理字符串有所帮助!
相关问答FAQs:
如何在Python中获取字符串的长度?
在Python中,可以使用内置的len()
函数来获取字符串的长度。只需将字符串作为参数传递给该函数,例如:length = len("Hello, World!")
。这将返回字符串的字符数,包括空格和标点符号。
获取用户输入字符串的长度时需要注意什么?
在获取用户输入字符串的长度时,可以使用input()
函数来接收用户输入。示例代码如下:
user_input = input("请输入一个字符串:")
length = len(user_input)
print(f"您输入的字符串长度为:{length}")
请注意,输入的字符串可能包含空格,这也会计入长度。
Python中字符串长度的单位是什么?
在Python中,字符串长度的单位是字符。len()
函数返回的是字符串中字符的总数,包括所有字母、数字、空格和特殊符号。例如,字符串"abc 123!"的长度为8,因为它包含了6个字母/数字和2个空格和标点。
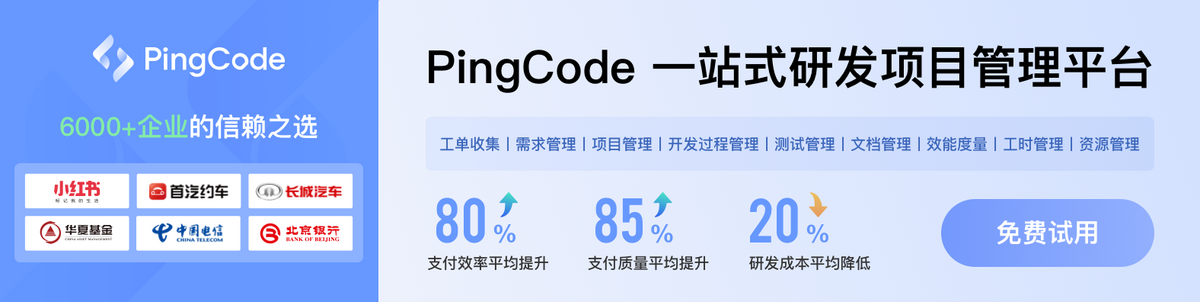