一、Python如何做时间为X轴
在Python中,可以使用matplotlib、pandas、seaborn等库来将时间作为X轴进行可视化、其中matplotlib是最常用的库。Matplotlib是一个强大的绘图库,可以处理多种数据类型,并且能够方便地进行时间序列的数据可视化。我们可以通过将时间数据格式化为日期时间对象,并将其设置为X轴来实现这一点。
在使用matplotlib时,可以通过将时间数据转换为datetime对象来确保正确地绘制时间轴。以下是一个详细描述:首先,我们需要导入必要的库,并确保时间数据被正确地读取和转换为datetime对象。然后,我们可以使用matplotlib的plot函数来绘制数据,并使用date_format来设置X轴的时间格式。
import matplotlib.pyplot as plt
import matplotlib.dates as mdates
import pandas as pd
生成示例数据
dates = pd.date_range('2023-01-01', periods=100)
data = pd.Series(range(100), index=dates)
绘图
fig, ax = plt.subplots()
ax.plot(data.index, data.values)
设置日期格式
ax.xaxis.set_major_formatter(mdates.DateFormatter('%Y-%m-%d'))
plt.xlabel('Date')
plt.ylabel('Values')
plt.title('Sample Time Series Data')
plt.xticks(rotation=45)
plt.show()
二、使用Matplotlib绘制时间序列
Matplotlib是Python中最常用的绘图库之一,用于创建静态、动画和交互式的可视化图表。它提供了许多功能,可以处理不同类型的数据和图表。在绘制时间序列图表时,Matplotlib提供了许多便捷的工具来处理时间轴和日期格式。
- 导入必要的库
在绘制时间序列图表之前,我们需要导入必要的库。通常需要导入matplotlib.pyplot、matplotlib.dates和pandas。
import matplotlib.pyplot as plt
import matplotlib.dates as mdates
import pandas as pd
- 准备数据
接下来,我们需要准备时间序列数据。可以使用pandas生成日期范围,并创建一个数据序列。
dates = pd.date_range('2023-01-01', periods=100)
data = pd.Series(range(100), index=dates)
- 创建图表
使用Matplotlib的plot函数创建图表,并将时间序列数据绘制在图表上。
fig, ax = plt.subplots()
ax.plot(data.index, data.values)
- 设置日期格式
为了使时间轴易于阅读,可以使用matplotlib.dates.DateFormatter设置日期格式,并旋转X轴标签以避免重叠。
ax.xaxis.set_major_formatter(mdates.DateFormatter('%Y-%m-%d'))
plt.xlabel('Date')
plt.ylabel('Values')
plt.title('Sample Time Series Data')
plt.xticks(rotation=45)
- 显示图表
最后,使用show函数显示图表。
plt.show()
三、使用Pandas和Matplotlib结合绘制时间序列
Pandas是一个强大的数据处理库,常与Matplotlib结合使用,用于处理和可视化时间序列数据。Pandas提供了许多便捷的方法来处理日期和时间数据,并与Matplotlib无缝集成。
- 读取时间序列数据
通常,时间序列数据存储在CSV文件中。可以使用pandas的read_csv函数读取CSV文件,并将日期列解析为日期时间对象。
import pandas as pd
data = pd.read_csv('time_series_data.csv', parse_dates=['Date'], index_col='Date')
- 绘制时间序列图表
使用Matplotlib绘制时间序列图表,并设置日期格式。
import matplotlib.pyplot as plt
import matplotlib.dates as mdates
fig, ax = plt.subplots()
ax.plot(data.index, data['Values'])
ax.xaxis.set_major_formatter(mdates.DateFormatter('%Y-%m-%d'))
plt.xlabel('Date')
plt.ylabel('Values')
plt.title('Time Series Data')
plt.xticks(rotation=45)
plt.show()
四、使用Seaborn绘制时间序列
Seaborn是基于Matplotlib的高级可视化库,提供了更加简洁和美观的接口来创建复杂的图表。在处理时间序列数据时,Seaborn也提供了许多便捷的功能。
- 导入必要的库
导入Seaborn、Matplotlib和Pandas库。
import seaborn as sns
import matplotlib.pyplot as plt
import pandas as pd
- 准备数据
生成时间序列数据。
dates = pd.date_range('2023-01-01', periods=100)
data = pd.DataFrame({'Date': dates, 'Values': range(100)})
- 绘制时间序列图表
使用Seaborn的lineplot函数绘制时间序列图表,并设置日期格式。
sns.set(style="darkgrid")
plt.figure(figsize=(12, 6))
sns.lineplot(x='Date', y='Values', data=data)
plt.xlabel('Date')
plt.ylabel('Values')
plt.title('Time Series Data')
plt.xticks(rotation=45)
plt.show()
五、使用Plotly进行交互式时间序列可视化
Plotly是一个用于创建交互式图表的库,支持多种图表类型,包括时间序列图表。与Matplotlib和Seaborn不同,Plotly生成的图表是交互式的,可以在网页中进行缩放、平移等操作。
- 导入必要的库
导入Plotly库。
import plotly.express as px
import pandas as pd
- 准备数据
生成时间序列数据。
dates = pd.date_range('2023-01-01', periods=100)
data = pd.DataFrame({'Date': dates, 'Values': range(100)})
- 绘制交互式时间序列图表
使用Plotly的express模块绘制交互式时间序列图表。
fig = px.line(data, x='Date', y='Values', title='Time Series Data')
fig.update_xaxes(rangeslider_visible=True)
fig.show()
六、处理时间序列数据的常见操作
在处理时间序列数据时,常常需要进行一些预处理操作,例如时间数据的解析、时间戳的转换、时间序列的重采样等。这些操作可以帮助我们更好地处理和可视化时间序列数据。
- 解析时间数据
在读取CSV文件时,可以使用pandas的parse_dates参数将时间列解析为日期时间对象。
data = pd.read_csv('time_series_data.csv', parse_dates=['Date'], index_col='Date')
- 时间戳转换
可以使用pandas的to_datetime函数将字符串格式的时间数据转换为日期时间对象。
data['Date'] = pd.to_datetime(data['Date'])
- 时间序列重采样
可以使用pandas的resample函数对时间序列数据进行重采样。例如,可以将数据从日频率重采样为月频率。
monthly_data = data.resample('M').sum()
七、时间序列数据的平滑和去噪
在实际应用中,时间序列数据可能会受到噪声的影响,使得数据看起来不平滑。在这种情况下,可以使用一些平滑和去噪技术来处理数据,使得数据更加平滑和易于分析。
- 移动平均
移动平均是一种常用的平滑技术,通过计算滑动窗口内的数据平均值来平滑时间序列数据。
data['Smoothed'] = data['Values'].rolling(window=5).mean()
- 指数平滑
指数平滑是一种加权移动平均方法,较新的数据点赋予更高的权重。
data['Exponentially_Smoothed'] = data['Values'].ewm(span=5).mean()
- 绘制平滑后的时间序列图表
使用Matplotlib绘制平滑后的时间序列图表。
fig, ax = plt.subplots()
ax.plot(data.index, data['Values'], label='Original')
ax.plot(data.index, data['Smoothed'], label='Smoothed')
ax.plot(data.index, data['Exponentially_Smoothed'], label='Exponentially Smoothed')
ax.xaxis.set_major_formatter(mdates.DateFormatter('%Y-%m-%d'))
plt.xlabel('Date')
plt.ylabel('Values')
plt.title('Smoothed Time Series Data')
plt.legend()
plt.xticks(rotation=45)
plt.show()
八、时间序列数据的分解
时间序列数据通常包含多个组成部分,例如趋势、季节性和残差。时间序列分解是一种将时间序列数据分解为这些组成部分的方法,有助于更好地理解和分析数据。
- 使用statsmodels进行时间序列分解
statsmodels是一个用于统计建模的Python库,提供了多种时间序列分析工具,包括时间序列分解。
import statsmodels.api as sm
decomposition = sm.tsa.seasonal_decompose(data['Values'], model='additive')
trend = decomposition.trend
seasonal = decomposition.seasonal
residual = decomposition.resid
- 绘制分解结果
使用Matplotlib绘制时间序列分解的结果。
fig, (ax1, ax2, ax3, ax4) = plt.subplots(4, 1, figsize=(12, 8), sharex=True)
ax1.plot(data.index, data['Values'], label='Original')
ax1.legend(loc='best')
ax2.plot(data.index, trend, label='Trend')
ax2.legend(loc='best')
ax3.plot(data.index, seasonal, label='Seasonal')
ax3.legend(loc='best')
ax4.plot(data.index, residual, label='Residual')
ax4.legend(loc='best')
ax4.xaxis.set_major_formatter(mdates.DateFormatter('%Y-%m-%d'))
plt.xlabel('Date')
plt.tight_layout()
plt.show()
九、时间序列的预测
时间序列预测是时间序列分析的一个重要应用。常见的时间序列预测方法包括ARIMA、SARIMA和LSTM等。
- 使用ARIMA进行时间序列预测
ARIMA(AutoRegressive Integrated Moving Average)是一种常用的时间序列预测方法,适用于稳定的时间序列数据。
from statsmodels.tsa.arima_model import ARIMA
model = ARIMA(data['Values'], order=(5, 1, 0))
model_fit = model.fit(disp=0)
forecast = model_fit.forecast(steps=10)
- 绘制预测结果
使用Matplotlib绘制时间序列预测的结果。
fig, ax = plt.subplots()
ax.plot(data.index, data['Values'], label='Original')
ax.plot(pd.date_range(data.index[-1], periods=10, freq='D'), forecast[0], label='Forecast')
ax.xaxis.set_major_formatter(mdates.DateFormatter('%Y-%m-%d'))
plt.xlabel('Date')
plt.ylabel('Values')
plt.title('Time Series Forecast')
plt.legend()
plt.xticks(rotation=45)
plt.show()
十、总结
通过使用Python中的Matplotlib、Pandas、Seaborn和Plotly等库,我们可以轻松地将时间作为X轴,进行时间序列数据的可视化。无论是简单的时间序列绘图,还是复杂的时间序列分解和平滑,Python都提供了丰富的工具和方法。掌握这些技术和方法,可以帮助我们更好地分析和理解时间序列数据,并进行有效的预测和决策。
相关问答FAQs:
如何在Python中将时间数据绘制为X轴?
在Python中,可以使用Matplotlib库将时间数据作为X轴进行绘图。首先,你需要确保时间数据是以适合的格式(如datetime对象)存储。使用pd.to_datetime()
将字符串转换为datetime格式,之后可以直接使用Matplotlib的plot
函数进行绘制。例如,plt.plot(time_data, value_data)
,其中time_data
是时间列表,value_data
是对应的数值列表。
使用Python绘制时间序列图需要哪些库?
为了绘制时间序列图,最常用的库包括Matplotlib和Pandas。Matplotlib用于绘图,Pandas则提供了强大的数据处理能力,尤其是在处理时间序列数据时。此外,Seaborn也是一个很好的选择,可以在Matplotlib的基础上提供更美观的图形。安装这些库可以通过pip install matplotlib pandas seaborn
命令完成。
如何处理不规则时间间隔的数据以绘制图表?
处理不规则时间间隔的数据可以使用Pandas的resample
或groupby
功能,首先将时间数据转换为Pandas的时间索引。通过dataframe.resample('频率').mean()
可以对时间序列数据进行重新采样,填补缺失值并生成均值等统计数据。这样可以确保绘制的图表更加平滑和准确。
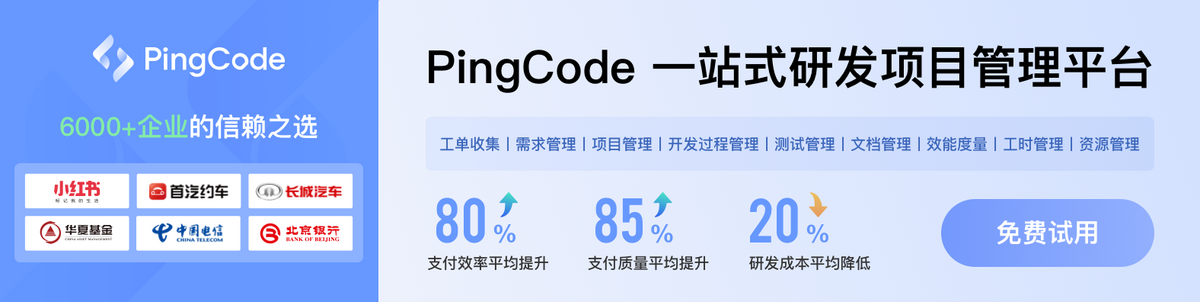