要用Python从堡垒机上复制数据,可以使用SSH连接、scp命令、paramiko库、文件处理等方法。 其中,paramiko库是一个非常强大的工具,它能够帮助我们通过SSH协议从远程服务器上复制文件,并且它在Python社区中广泛使用。下面将详细介绍如何使用paramiko库从堡垒机上复制数据。
一、安装paramiko库
在开始之前,确保已经安装了paramiko库。如果没有安装,可以使用pip进行安装:
pip install paramiko
二、连接堡垒机
连接堡垒机是实现复制数据的第一步。使用paramiko库可以非常方便地建立SSH连接。以下是一个示例代码:
import paramiko
def connect_to_bastion(hostname, port, username, password):
try:
client = paramiko.SSHClient()
client.set_missing_host_key_policy(paramiko.AutoAddPolicy())
client.connect(hostname, port, username, password)
print("Connected to bastion host")
return client
except Exception as e:
print(f"Failed to connect to bastion host: {e}")
return None
在这段代码中,connect_to_bastion
函数接受堡垒机的主机名、端口号、用户名和密码作为参数,并尝试建立SSH连接。如果连接成功,将返回SSH客户端对象。
三、通过堡垒机连接目标服务器
一旦连接到堡垒机,可以通过隧道连接到目标服务器。这里使用paramiko库的Channel
对象来创建隧道。以下是示例代码:
def create_tunnel(bastion_client, target_hostname, target_port, target_username, target_password):
try:
transport = bastion_client.get_transport()
dest_addr = (target_hostname, target_port)
local_addr = ('127.0.0.1', 0)
channel = transport.open_channel('direct-tcpip', dest_addr, local_addr)
target_client = paramiko.SSHClient()
target_client.set_missing_host_key_policy(paramiko.AutoAddPolicy())
target_client.connect(target_hostname, target_port, target_username, target_password, sock=channel)
print("Connected to target server through bastion host")
return target_client
except Exception as e:
print(f"Failed to connect to target server: {e}")
return None
在这段代码中,create_tunnel
函数通过堡垒机的SSH客户端对象建立到目标服务器的隧道连接,并返回目标服务器的SSH客户端对象。
四、从目标服务器复制数据
通过目标服务器的SSH客户端对象,可以使用SFTP协议复制文件。以下是示例代码:
def copy_data_from_target(target_client, remote_path, local_path):
try:
sftp = target_client.open_sftp()
sftp.get(remote_path, local_path)
sftp.close()
print(f"Copied data from {remote_path} to {local_path}")
except Exception as e:
print(f"Failed to copy data: {e}")
在这段代码中,copy_data_from_target
函数通过目标服务器的SSH客户端对象建立SFTP连接,并使用get
方法将远程文件复制到本地。
五、完整示例
以下是完整的示例代码,将前面的步骤整合到一起:
import paramiko
def connect_to_bastion(hostname, port, username, password):
try:
client = paramiko.SSHClient()
client.set_missing_host_key_policy(paramiko.AutoAddPolicy())
client.connect(hostname, port, username, password)
print("Connected to bastion host")
return client
except Exception as e:
print(f"Failed to connect to bastion host: {e}")
return None
def create_tunnel(bastion_client, target_hostname, target_port, target_username, target_password):
try:
transport = bastion_client.get_transport()
dest_addr = (target_hostname, target_port)
local_addr = ('127.0.0.1', 0)
channel = transport.open_channel('direct-tcpip', dest_addr, local_addr)
target_client = paramiko.SSHClient()
target_client.set_missing_host_key_policy(paramiko.AutoAddPolicy())
target_client.connect(target_hostname, target_port, target_username, target_password, sock=channel)
print("Connected to target server through bastion host")
return target_client
except Exception as e:
print(f"Failed to connect to target server: {e}")
return None
def copy_data_from_target(target_client, remote_path, local_path):
try:
sftp = target_client.open_sftp()
sftp.get(remote_path, local_path)
sftp.close()
print(f"Copied data from {remote_path} to {local_path}")
except Exception as e:
print(f"Failed to copy data: {e}")
def main():
bastion_hostname = 'bastion.example.com'
bastion_port = 22
bastion_username = 'bastion_user'
bastion_password = 'bastion_password'
target_hostname = 'target.example.com'
target_port = 22
target_username = 'target_user'
target_password = 'target_password'
remote_path = '/path/to/remote/file'
local_path = '/path/to/local/file'
bastion_client = connect_to_bastion(bastion_hostname, bastion_port, bastion_username, bastion_password)
if bastion_client:
target_client = create_tunnel(bastion_client, target_hostname, target_port, target_username, target_password)
if target_client:
copy_data_from_target(target_client, remote_path, local_path)
target_client.close()
bastion_client.close()
if __name__ == '__main__':
main()
在这个完整示例中,我们首先连接到堡垒机,然后通过堡垒机创建到目标服务器的隧道连接,最后从目标服务器复制数据到本地。确保将示例中的堡垒机和目标服务器的主机名、端口号、用户名、密码和文件路径替换为实际的值。
六、处理异常情况
在实际应用中,需要处理各种可能的异常情况,例如网络不稳定、认证失败、文件不存在等。可以在每个函数中添加更多的异常处理逻辑,以确保程序的健壮性。
七、定期任务
如果需要定期从堡垒机上复制数据,可以使用Python的schedule
库或操作系统的定时任务(如cron或Task Scheduler)来实现定时执行。
以下是使用schedule
库的示例代码:
import schedule
import time
def job():
main()
schedule.every().day.at("01:00").do(job)
while True:
schedule.run_pending()
time.sleep(1)
在这个示例中,schedule
库将每天在凌晨1点执行一次数据复制任务。
八、总结
使用Python从堡垒机上复制数据是一个实用的技术,可以帮助我们在安全的环境中高效地传输数据。通过paramiko库,可以方便地建立SSH连接、创建隧道连接,并使用SFTP协议复制文件。以上示例代码展示了如何逐步实现这些功能,并处理各种可能的异常情况。在实际应用中,可以根据具体需求进行调整和优化,以实现更加健壮和高效的数据传输。
希望这篇文章能帮助你理解如何用Python从堡垒机上复制数据,并为你的实际项目提供参考。
相关问答FAQs:
如何在Python中连接堡垒机以进行数据复制?
连接堡垒机的常用方法是使用SSH协议。您可以利用Python中的paramiko
库来建立SSH连接。首先,确保您已经安装了该库。连接时,需要提供堡垒机的IP地址、用户名和密码或密钥文件。通过建立连接后,您可以使用exec_command
方法执行命令,从而实现数据的复制。
在复制数据时,如何处理堡垒机的认证问题?
堡垒机通常会要求更高的安全性,您可能需要使用SSH密钥对而不是密码进行认证。如果您使用密钥文件,可以在paramiko
中指定密钥路径。同时,有些堡垒机可能要求多重认证,例如双因素认证,您需要根据具体要求调整连接方法,确保认证顺利。
使用Python从堡垒机复制数据时,有哪些常见的错误及解决方案?
常见的错误包括连接超时、认证失败和权限不足。连接超时可能是由于网络问题,建议检查网络状态。认证失败通常与用户名或密码错误有关,确保输入信息无误。权限不足的问题可以通过确认用户在堡垒机上具备适当的访问权限来解决。如果错误依旧,可以查看堡垒机的日志以获取更多信息。
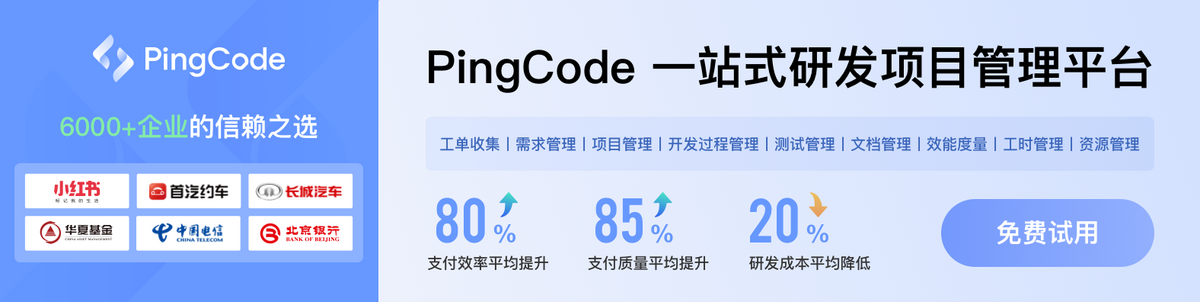