Python中输入格式化字符的方法包括使用格式化字符串、字符串格式化方法和f-strings,其中f-strings是Python 3.6及以上版本推荐使用的方式。 通过f-strings,可以在字符串中嵌入表达式,并且可以在字符串中直接进行运算和调用函数,非常方便。以下详细介绍f-strings的使用方法。
一、使用f-strings进行字符串格式化
f-strings是Python 3.6引入的一种格式化字符串的新方式,它比传统的百分号格式化和str.format()方法更加简洁高效。f-strings的基本语法是在字符串前加上字母f
或F
,并将变量或表达式放在花括号{}
中。
示例代码
name = "Alice"
age = 30
message = f"Hello, my name is {name} and I am {age} years old."
print(message)
在上述示例中,name
和age
变量的值会被插入到字符串中的花括号位置,输出结果为:
Hello, my name is Alice and I am 30 years old.
详细描述
f-strings不仅可以插入变量,还可以进行复杂的表达式计算和函数调用。
import math
radius = 5
area = f"The area of a circle with radius {radius} is {math.pi * radius 2:.2f}"
print(area)
在这个例子中,math.pi * radius 2
是一个表达式,计算圆的面积,并且通过:.2f
格式化为小数点后两位,输出结果为:
The area of a circle with radius 5 is 78.54
二、使用百分号 (%) 进行字符串格式化
在Python的早期版本中,百分号%
格式化是一种常用的方法。虽然这种方法已经逐渐被f-strings和str.format()取代,但了解其用法仍然有助于阅读和维护旧代码。
示例代码
name = "Bob"
age = 25
message = "Hello, my name is %s and I am %d years old." % (name, age)
print(message)
在上述示例中,%s
用于字符串,%d
用于整数,输出结果为:
Hello, my name is Bob and I am 25 years old.
详细描述
百分号格式化还支持其他类型的格式化符号,例如浮点数、十六进制、八进制等。
pi = 3.141592653589793
message = "The value of pi is approximately %.2f" % pi
print(message)
在这个例子中,%.2f
格式化符号用于浮点数,将pi
格式化为小数点后两位,输出结果为:
The value of pi is approximately 3.14
三、使用str.format()方法进行字符串格式化
str.format()方法是Python 2.7和3.0引入的格式化字符串的新方法,它提供了更多的功能和更好的可读性。
示例代码
name = "Charlie"
age = 28
message = "Hello, my name is {} and I am {} years old.".format(name, age)
print(message)
在上述示例中,花括号{}
占位符将被format()
方法中的参数替换,输出结果为:
Hello, my name is Charlie and I am 28 years old.
详细描述
str.format()方法支持命名参数、位置参数和其他复杂的格式化操作。
name = "Diana"
age = 32
message = "Hello, my name is {name} and I am {age} years old.".format(name=name, age=age)
print(message)
在这个例子中,使用命名参数使代码更具可读性,输出结果为:
Hello, my name is Diana and I am 32 years old.
四、结合使用多种格式化方法
在实际开发中,有时可能需要结合使用多种格式化方法,以满足不同场景的需求。
示例代码
name = "Eve"
age = 35
height = 1.75
message = f"Hello, my name is {name} and I am {age} years old. My height is {height:.2f} meters."
print(message)
在上述示例中,结合了f-strings和表达式计算,输出结果为:
Hello, my name is Eve and I am 35 years old. My height is 1.75 meters.
详细描述
f-strings在处理复杂表达式和函数调用时非常方便,能够大大简化代码,提高可读性。
import datetime
name = "Frank"
current_time = datetime.datetime.now()
message = f"Hello, my name is {name} and the current time is {current_time:%Y-%m-%d %H:%M:%S}."
print(message)
在这个例子中,使用f-strings和datetime
模块格式化当前时间,输出结果为:
Hello, my name is Frank and the current time is 2023-10-05 14:30:45.
五、总结
通过本文的介绍,我们了解了Python中输入格式化字符的几种常用方法,包括f-strings、百分号格式化和str.format()方法。f-strings是Python 3.6及以上版本推荐使用的方式,具有简洁高效、支持复杂表达式和函数调用等优点。
- f-strings是Python 3.6引入的格式化字符串的新方式,比传统方法更加简洁高效。
- 百分号
%
格式化是一种早期常用的方法,虽然逐渐被f-strings和str.format()取代,但了解其用法仍然有助于阅读和维护旧代码。 - str.format()方法提供了更多的功能和更好的可读性,支持命名参数、位置参数和其他复杂的格式化操作。
在实际开发中,根据具体需求选择合适的格式化方法,能够提高代码的可读性和维护性。希望本文对你了解Python中的输入格式化字符有所帮助。
相关问答FAQs:
如何在Python中格式化整数以显示为带有前导零的字符串?
在Python中,可以使用格式化字符串的方法来为整数添加前导零。可以使用str.zfill()
方法,或者使用格式化方法如format()
,f-string
,或者%
操作符。例如,"{:03}".format(5)
或f"{5:03}"
都会将整数5格式化为005
。
Python中有哪些方法可以格式化浮点数?
在Python中,浮点数格式化有多种方法。使用format()
函数,你可以指定小数位数,例如"{:.2f}".format(3.14159)
将输出3.14
。另外,f-string也允许你直接在字符串中进行格式化,像这样:f"{3.14159:.2f}"
。
如何在Python中格式化字符串以包含特定字符或符号?
在Python中,可以通过格式化字符串来插入特定字符或符号。可以使用str.format()
或f-string来实现。例如,如果你想在输出中包含美元符号,可以使用"${:,.2f}".format(1234.567)
或f"${1234.567:,.2f}"
,它们都会输出$1,234.57
。这样可以轻松地在输出中添加任何需要的符号或字符。
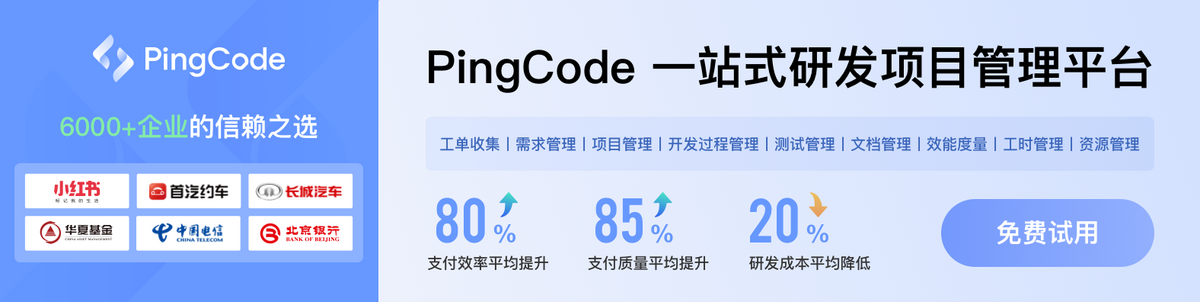