要调用Python中的类的成员函数,可以通过实例化类对象、使用类名调用静态方法、通过类方法的装饰器等方式,最常见的是通过实例化类对象来调用。 例如:创建一个类并实例化它,然后使用实例对象调用成员函数。
class MyClass:
def __init__(self, value):
self.value = value
def display_value(self):
print(f'The value is: {self.value}')
Create an instance of MyClass
obj = MyClass(10)
Call the display_value method
obj.display_value()
在上面的例子中,MyClass
类定义了一个构造函数__init__
和一个成员函数display_value
。通过创建MyClass
的实例obj
,我们可以调用display_value
成员函数。
一、类的定义与实例化
在Python中,类是使用class
关键字定义的。类可以包含成员变量和成员函数。成员函数用于定义对象的行为,而成员变量用于存储对象的状态。要调用类的成员函数,首先需要实例化类,即创建类的对象。
class MyClass:
def __init__(self, value):
self.value = value
def display_value(self):
print(f'The value is: {self.value}')
Create an instance of MyClass
obj = MyClass(10)
在这个示例中,MyClass
类有一个构造函数__init__
,它接受一个参数value
并将其存储在实例变量self.value
中。然后,我们创建一个MyClass
的实例obj
,并将10
传递给构造函数。
二、调用实例方法
实例方法是类中的成员函数,可以通过实例对象来调用。实例方法的第一个参数通常是self
,它指代调用方法的实例对象。
class MyClass:
def __init__(self, value):
self.value = value
def display_value(self):
print(f'The value is: {self.value}')
Create an instance of MyClass
obj = MyClass(10)
Call the display_value method
obj.display_value()
在这个例子中,我们使用实例对象obj
调用display_value
方法。self
参数自动传递了obj
实例,因此self.value
指的是obj.value
,即10
。调用该方法会输出The value is: 10
。
三、类方法和静态方法
除了实例方法外,Python还支持类方法和静态方法。类方法使用@classmethod
装饰器定义,第一个参数是cls
,指代类本身。静态方法使用@staticmethod
装饰器定义,不需要self
或cls
参数。
class MyClass:
value = 0
@classmethod
def set_value(cls, new_value):
cls.value = new_value
@staticmethod
def display_class_value():
print(f'The class value is: {MyClass.value}')
Call class method
MyClass.set_value(20)
Call static method
MyClass.display_class_value()
在这个例子中,set_value
是类方法,display_class_value
是静态方法。类方法可以通过类名直接调用,并且可以访问和修改类变量。静态方法也可以通过类名直接调用,但不能访问或修改类变量。
四、私有成员函数
在类中,可以通过在函数名前加下划线_
或双下划线__
来定义私有成员函数。私有成员函数只能在类的内部调用,不能通过实例对象直接访问。
class MyClass:
def __init__(self, value):
self.__value = value
def __display_value(self):
print(f'The value is: {self.__value}')
def call_private_method(self):
self.__display_value()
Create an instance of MyClass
obj = MyClass(10)
Call public method that calls the private method
obj.call_private_method()
在这个例子中,__display_value
是一个私有成员函数,不能通过实例对象obj
直接调用。我们定义了一个公有方法call_private_method
,在类的内部调用私有成员函数,从而间接调用私有成员函数。
五、继承与重写
继承是面向对象编程的重要特性。子类可以继承父类的成员函数,并可以选择性地重写它们。
class ParentClass:
def display_message(self):
print('This is the parent class.')
class ChildClass(ParentClass):
def display_message(self):
print('This is the child class.')
Create instances of ParentClass and ChildClass
parent = ParentClass()
child = ChildClass()
Call the display_message method
parent.display_message()
child.display_message()
在这个例子中,ChildClass
继承了ParentClass
,并重写了display_message
方法。调用parent.display_message()
输出This is the parent class.
,调用child.display_message()
输出This is the child class.
。
六、多态与动态绑定
多态是面向对象编程的另一个重要特性。它允许在不同类中定义同名的成员函数,通过动态绑定在运行时确定实际调用的函数。
class Animal:
def make_sound(self):
print('Some generic animal sound')
class Dog(Animal):
def make_sound(self):
print('Bark')
class Cat(Animal):
def make_sound(self):
print('Meow')
def play_sound(animal):
animal.make_sound()
Create instances of Dog and Cat
dog = Dog()
cat = Cat()
Call the play_sound function
play_sound(dog)
play_sound(cat)
在这个例子中,Dog
和Cat
类继承了Animal
类,并重写了make_sound
方法。play_sound
函数接受一个Animal
类型的参数,并调用其make_sound
方法。由于多态和动态绑定,调用play_sound(dog)
输出Bark
,调用play_sound(cat)
输出Meow
。
七、组合与委托
组合是通过包含对象来实现类之间的关系。委托是将某些功能委托给另一个类的实例来实现。
class Engine:
def start(self):
print('Engine started')
class Car:
def __init__(self):
self.engine = Engine()
def start(self):
self.engine.start()
print('Car started')
Create an instance of Car
car = Car()
Call the start method
car.start()
在这个例子中,Car
类包含一个Engine
类的实例,并将start
方法委托给Engine
实例来实现。调用car.start()
输出Engine started
和Car started
。
八、成员函数的命名约定
在编写类时,遵循一些命名约定可以使代码更易读和维护。以下是一些常见的命名约定:
- 公有成员函数:使用小写字母和下划线分隔单词,例如
display_value
。 - 私有成员函数:使用单下划线或双下划线开头,例如
_private_method
或__private_method
。 - 类方法:使用
@classmethod
装饰器,通常命名为set_something
或get_something
。 - 静态方法:使用
@staticmethod
装饰器,通常命名为perform_action
或calculate_something
。
遵循这些命名约定可以提高代码的可读性和可维护性,使其他开发者更容易理解和使用类的成员函数。
九、使用文档字符串
在定义类和成员函数时,使用文档字符串(docstring)可以帮助其他开发者理解类的用途和功能。
class MyClass:
"""This is a sample class."""
def __init__(self, value):
"""
Initialize the MyClass instance.
Args:
value (int): The value to be stored in the instance.
"""
self.value = value
def display_value(self):
"""
Display the value stored in the instance.
"""
print(f'The value is: {self.value}')
Create an instance of MyClass
obj = MyClass(10)
Call the display_value method
obj.display_value()
在这个示例中,我们使用文档字符串为类和成员函数添加了注释。文档字符串通常位于定义的第一行,用三重引号("""
)括起来。使用文档字符串可以帮助开发者快速了解类和成员函数的用途和参数。
十、总结
调用Python中类的成员函数主要有以下几种方式:
- 实例化类对象:通过创建类的实例对象来调用成员函数,这是最常见的方式。
- 类方法和静态方法:使用
@classmethod
和@staticmethod
装饰器定义类方法和静态方法,可以通过类名直接调用。 - 私有成员函数:通过在类内部定义私有成员函数,只能在类的内部调用,不能通过实例对象直接访问。
- 继承与重写:子类可以继承父类的成员函数,并选择性地重写它们。
- 多态与动态绑定:通过多态和动态绑定,可以在运行时确定实际调用的成员函数。
- 组合与委托:通过组合和委托,可以将某些功能委托给另一个类的实例来实现。
掌握这些技巧可以帮助你在Python中更灵活地调用类的成员函数,提高代码的可读性和可维护性。
相关问答FAQs:
如何在Python中创建一个类并调用其成员函数?
在Python中,创建一个类可以使用class
关键字。类中可以定义成员函数,使用self
参数来访问类的属性和其他方法。调用成员函数时,首先需要实例化该类,然后通过实例对象调用相应的成员函数。例如:
class MyClass:
def my_method(self):
print("Hello from my_method!")
# 实例化类
obj = MyClass()
# 调用成员函数
obj.my_method()
如果类中有多个成员函数,如何选择性地调用?
在一个类中可以定义多个成员函数,可以根据需要选择性地调用它们。通过实例对象,可以直接调用任何已定义的成员函数,例如:
class MyClass:
def method_one(self):
print("This is method one.")
def method_two(self):
print("This is method two.")
obj = MyClass()
obj.method_one() # 调用method_one
obj.method_two() # 调用method_two
在调用成员函数时,如何传递参数?
成员函数可以接受参数,以便在调用时传递不同的数据。定义时在函数参数列表中添加参数,然后在调用时提供具体的值,例如:
class MyClass:
def greet(self, name):
print(f"Hello, {name}!")
obj = MyClass()
obj.greet("Alice") # 输出: Hello, Alice!
通过这种方式,您可以灵活地在成员函数中使用参数,实现更复杂的功能。
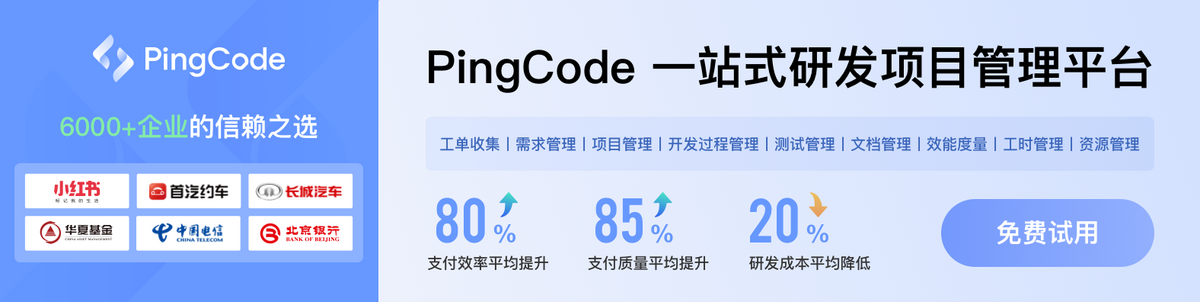