Python 识别字符串的方法有:使用内置函数、正则表达式、字符串方法、类型检查等。其中,正则表达式是一种非常强大的工具,可以用来匹配复杂的字符串模式。下面将详细描述使用正则表达式来识别字符串的方法。
Python中的字符串识别和处理是非常重要的功能,特别是在数据处理和文本分析领域。了解并掌握这些方法可以帮助我们更高效地处理和分析数据。接下来,我们将深入探讨Python识别字符串的几种方法,并逐步展开每种方法的详细说明和示例。
一、内置函数
Python提供了许多内置函数,可以用来识别和处理字符串。这些函数通常是最简单、最直接的方法,适合初学者和处理简单字符串的场景。
1、len() 函数
len()
函数用于返回字符串的长度。这个函数非常基础,但在很多场景下都非常有用。
string = "Hello, World!"
print(len(string)) # 输出: 13
2、str() 函数
str()
函数可以将其他类型的数据转换为字符串。这在需要对非字符串类型的数据进行字符串操作时非常有用。
number = 123
string_number = str(number)
print(string_number) # 输出: '123'
3、ord() 和 chr() 函数
ord()
函数用于返回字符的Unicode码,chr()
函数则用于将Unicode码转换为字符。这两个函数在需要进行字符编码和解码时非常有用。
char = 'A'
unicode = ord(char)
print(unicode) # 输出: 65
unicode = 65
char = chr(unicode)
print(char) # 输出: 'A'
二、正则表达式
正则表达式是一种强大的工具,用于匹配复杂的字符串模式。在Python中,可以使用re
模块来处理正则表达式。
1、re.match() 函数
re.match()
函数用于从字符串的起始位置匹配一个模式。如果匹配成功,返回一个匹配对象;否则,返回None
。
import re
pattern = r'Hello'
string = 'Hello, World!'
match = re.match(pattern, string)
if match:
print('匹配成功')
else:
print('匹配失败')
2、re.search() 函数
re.search()
函数用于在整个字符串中搜索模式。如果找到匹配,返回一个匹配对象;否则,返回None
。
import re
pattern = r'World'
string = 'Hello, World!'
search = re.search(pattern, string)
if search:
print('匹配成功')
else:
print('匹配失败')
3、re.findall() 函数
re.findall()
函数用于在字符串中查找所有匹配的模式,并以列表的形式返回所有匹配的子字符串。
import re
pattern = r'\d+'
string = 'There are 123 apples and 456 oranges.'
findall = re.findall(pattern, string)
print(findall) # 输出: ['123', '456']
三、字符串方法
Python的字符串类提供了许多方法,用于检查字符串的内容和模式。
1、startswith() 和 endswith() 方法
startswith()
方法用于检查字符串是否以指定的子字符串开头,endswith()
方法用于检查字符串是否以指定的子字符串结尾。
string = 'Hello, World!'
print(string.startswith('Hello')) # 输出: True
print(string.endswith('World!')) # 输出: True
2、find() 和 rfind() 方法
find()
方法用于返回指定子字符串在字符串中的首次出现位置,如果未找到,返回-1
。rfind()
方法用于返回指定子字符串在字符串中的最后一次出现位置。
string = 'Hello, World! Hello, Python!'
print(string.find('Hello')) # 输出: 0
print(string.rfind('Hello')) # 输出: 14
3、isalpha(), isdigit() 和 isalnum() 方法
这些方法用于检查字符串是否只包含字母、数字或字母和数字的组合。
string_alpha = 'Hello'
string_digit = '12345'
string_alnum = 'Hello123'
print(string_alpha.isalpha()) # 输出: True
print(string_digit.isdigit()) # 输出: True
print(string_alnum.isalnum()) # 输出: True
四、类型检查
在某些情况下,我们需要判断一个变量是否为字符串类型。Python提供了多种方法来进行类型检查。
1、使用 isinstance() 函数
isinstance()
函数用于检查一个变量是否为特定类型。
string = 'Hello, World!'
print(isinstance(string, str)) # 输出: True
2、使用 type() 函数
type()
函数用于返回变量的类型。
string = 'Hello, World!'
print(type(string) == str) # 输出: True
五、字符串格式化
字符串格式化是指根据特定格式生成新的字符串。Python提供了多种字符串格式化的方法。
1、使用 % 操作符
%
操作符用于格式化字符串,类似于C语言中的printf函数。
name = 'John'
age = 30
formatted_string = 'My name is %s and I am %d years old.' % (name, age)
print(formatted_string) # 输出: My name is John and I am 30 years old.
2、使用 str.format() 方法
str.format()
方法是Python推荐的字符串格式化方法,功能更强大,语法更简洁。
name = 'John'
age = 30
formatted_string = 'My name is {} and I am {} years old.'.format(name, age)
print(formatted_string) # 输出: My name is John and I am 30 years old.
3、使用 f-string (格式化字符串)
f-string是Python 3.6引入的一种新的字符串格式化方法,语法更加简洁直观。
name = 'John'
age = 30
formatted_string = f'My name is {name} and I am {age} years old.'
print(formatted_string) # 输出: My name is John and I am 30 years old.
六、字符串分割和连接
在处理字符串时,分割和连接字符串是非常常见的操作。Python提供了多种方法来实现这些操作。
1、split() 方法
split()
方法用于将字符串按照指定的分隔符分割成多个子字符串,并以列表的形式返回这些子字符串。
string = 'Hello, World! Hello, Python!'
split_string = string.split(' ')
print(split_string) # 输出: ['Hello,', 'World!', 'Hello,', 'Python!']
2、join() 方法
join()
方法用于将多个子字符串连接成一个字符串,子字符串之间使用指定的分隔符。
words = ['Hello', 'World', 'Python']
joined_string = ' '.join(words)
print(joined_string) # 输出: 'Hello World Python'
七、字符串替换
字符串替换是指将字符串中的某些子字符串替换为新的子字符串。Python提供了多种方法来实现字符串替换。
1、replace() 方法
replace()
方法用于将字符串中的指定子字符串替换为新的子字符串。
string = 'Hello, World! Hello, Python!'
replaced_string = string.replace('Hello', 'Hi')
print(replaced_string) # 输出: 'Hi, World! Hi, Python!'
2、re.sub() 函数
re.sub()
函数用于使用正则表达式进行字符串替换。
import re
string = 'Hello, World! Hello, Python!'
pattern = r'Hello'
replacement = 'Hi'
replaced_string = re.sub(pattern, replacement, string)
print(replaced_string) # 输出: 'Hi, World! Hi, Python!'
八、字符串去除空白字符
在处理字符串时,经常需要去除字符串两端或中间的空白字符。Python提供了多种方法来实现这一功能。
1、strip(), lstrip() 和 rstrip() 方法
strip()
方法用于去除字符串两端的空白字符,lstrip()
方法用于去除字符串左端的空白字符,rstrip()
方法用于去除字符串右端的空白字符。
string = ' Hello, World! '
print(string.strip()) # 输出: 'Hello, World!'
print(string.lstrip()) # 输出: 'Hello, World! '
print(string.rstrip()) # 输出: ' Hello, World!'
九、字符串大小写转换
Python提供了多种方法来转换字符串的大小写,这在文本处理和分析中非常常见。
1、upper() 和 lower() 方法
upper()
方法用于将字符串中的所有字母转换为大写字母,lower()
方法用于将字符串中的所有字母转换为小写字母。
string = 'Hello, World!'
print(string.upper()) # 输出: 'HELLO, WORLD!'
print(string.lower()) # 输出: 'hello, world!'
2、capitalize() 和 title() 方法
capitalize()
方法用于将字符串的第一个字母转换为大写字母,其余字母转换为小写字母。title()
方法用于将字符串中的每个单词的第一个字母转换为大写字母,其余字母转换为小写字母。
string = 'hello, world!'
print(string.capitalize()) # 输出: 'Hello, world!'
print(string.title()) # 输出: 'Hello, World!'
十、字符串编码和解码
在处理不同编码的文本数据时,字符串编码和解码是非常重要的操作。Python提供了多种方法来实现字符串的编码和解码。
1、encode() 方法
encode()
方法用于将字符串编码为指定编码格式的字节对象。
string = 'Hello, World!'
encoded_string = string.encode('utf-8')
print(encoded_string) # 输出: b'Hello, World!'
2、decode() 方法
decode()
方法用于将字节对象解码为指定编码格式的字符串。
encoded_string = b'Hello, World!'
decoded_string = encoded_string.decode('utf-8')
print(decoded_string) # 输出: 'Hello, World!'
综上所述,Python提供了丰富多样的字符串识别和处理方法,包括内置函数、正则表达式、字符串方法、类型检查、字符串格式化、字符串分割和连接、字符串替换、字符串去除空白字符、字符串大小写转换以及字符串编码和解码等。掌握这些方法可以帮助我们更高效地处理和分析文本数据,从而提升编程能力和工作效率。
相关问答FAQs:
如何在Python中检查字符串是否包含特定子串?
在Python中,您可以使用in
关键字来检查一个字符串是否包含另一个字符串。例如,if '子串' in '主字符串':
可以用来判断'主字符串'中是否包含'子串'。此外,您也可以使用字符串的find()
或index()
方法来获取子串的位置,如果返回值为-1,说明子串不存在。
Python中如何对字符串进行大小写转换?
您可以使用lower()
和upper()
方法来转换字符串的大小写。调用字符串.lower()
将所有字母转换为小写,而调用字符串.upper()
则会将所有字母转换为大写。如果需要将首字母大写,可以使用字符串.capitalize()
方法。
如何在Python中判断字符串是否是数字?
在Python中,您可以使用str.isdigit()
方法来判断字符串是否只包含数字字符。如果字符串只包含数字,该方法将返回True;否则返回False。例如,'123'.isdigit()
将返回True,而'123abc'.isdigit()
将返回False。对于包含小数的数字,可以使用try-except
结构结合float()
函数进行判断。
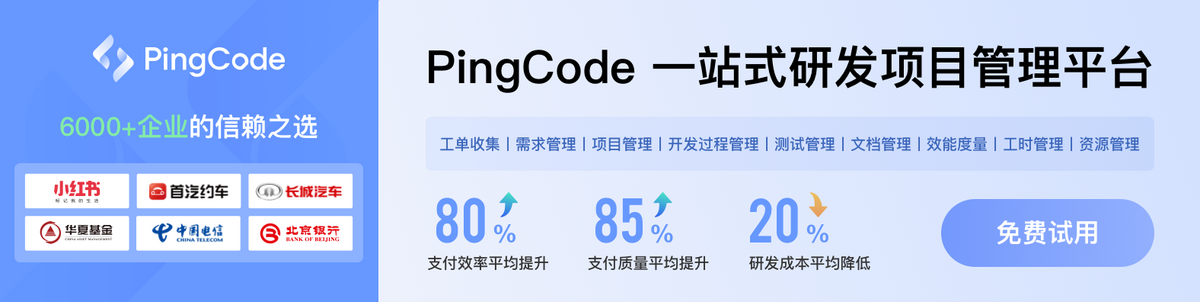