如何用Python实现对图像的分割
图像分割是计算机视觉中的一个重要任务,它可以将图像分割成具有意义的区域。常用的方法有阈值分割、边缘检测、区域生长、聚类算法、深度学习等。本文将详细介绍这些方法,并提供Python代码示例。
阈值分割是最简单的图像分割方法之一。它根据像素值的阈值将图像分为前景和背景。边缘检测通过检测图像中的边缘来实现分割,这些边缘通常表示图像中的物体边界。区域生长是基于像素相似性的一种图像分割方法,聚类算法如K-means则将图像像素聚类成不同的组。深度学习方法,如U-Net和Mask R-CNN,利用神经网络实现高级的图像分割。
一、阈值分割
阈值分割是将图像中的像素值与某个阈值进行比较,进而将像素分类为前景或背景。这种方法适用于简单的场景。
import cv2
import numpy as np
from matplotlib import pyplot as plt
读取图像
image = cv2.imread('image.jpg', 0)
应用全局阈值
_, thresholded = cv2.threshold(image, 127, 255, cv2.THRESH_BINARY)
显示图像
plt.subplot(1, 2, 1)
plt.imshow(image, cmap='gray')
plt.title('Original Image')
plt.subplot(1, 2, 2)
plt.imshow(thresholded, cmap='gray')
plt.title('Thresholded Image')
plt.show()
二、边缘检测
边缘检测通过检测图像中的显著变化来实现分割。常用的边缘检测算法有Canny边缘检测器。
# 应用Canny边缘检测
edges = cv2.Canny(image, 100, 200)
显示图像
plt.subplot(1, 2, 1)
plt.imshow(image, cmap='gray')
plt.title('Original Image')
plt.subplot(1, 2, 2)
plt.imshow(edges, cmap='gray')
plt.title('Edge Image')
plt.show()
三、区域生长
区域生长方法从一个或多个种子点开始,逐步将与种子点相似的像素加入区域。
def region_growing(img, seed):
h, w = img.shape
segmented = np.zeros_like(img)
segmented[seed[0], seed[1]] = 255
stack = [seed]
while stack:
x, y = stack.pop()
for dx, dy in [(-1, 0), (1, 0), (0, -1), (0, 1)]:
nx, ny = x + dx, y + dy
if 0 <= nx < h and 0 <= ny < w and segmented[nx, ny] == 0 and img[nx, ny] == img[x, y]:
segmented[nx, ny] = 255
stack.append((nx, ny))
return segmented
seed_point = (100, 100)
segmented_image = region_growing(image, seed_point)
显示图像
plt.subplot(1, 2, 1)
plt.imshow(image, cmap='gray')
plt.title('Original Image')
plt.subplot(1, 2, 2)
plt.imshow(segmented_image, cmap='gray')
plt.title('Segmented Image')
plt.show()
四、聚类算法
聚类算法如K-means可以将图像像素分成多个簇,每个簇表示一个分割区域。
from sklearn.cluster import KMeans
将图像转换为二维数组
pixels = image.reshape(-1, 1)
应用K-means聚类
kmeans = KMeans(n_clusters=2)
kmeans.fit(pixels)
segmented = kmeans.labels_.reshape(image.shape)
显示图像
plt.subplot(1, 2, 1)
plt.imshow(image, cmap='gray')
plt.title('Original Image')
plt.subplot(1, 2, 2)
plt.imshow(segmented, cmap='gray')
plt.title('Segmented Image')
plt.show()
五、深度学习
深度学习方法在图像分割领域取得了显著进展。U-Net和Mask R-CNN是两种常用的深度学习模型。
U-Net
U-Net是一种卷积神经网络,专门用于生物医学图像分割。它由一个编码器和一个解码器组成,能够高效地捕捉图像细节。
import tensorflow as tf
from tensorflow.keras import layers, models
def unet_model(input_size=(128, 128, 1)):
inputs = layers.Input(input_size)
c1 = layers.Conv2D(64, (3, 3), activation='relu', padding='same')(inputs)
c1 = layers.Conv2D(64, (3, 3), activation='relu', padding='same')(c1)
p1 = layers.MaxPooling2D((2, 2))(c1)
c2 = layers.Conv2D(128, (3, 3), activation='relu', padding='same')(p1)
c2 = layers.Conv2D(128, (3, 3), activation='relu', padding='same')(c2)
p2 = layers.MaxPooling2D((2, 2))(c2)
c3 = layers.Conv2D(256, (3, 3), activation='relu', padding='same')(p2)
c3 = layers.Conv2D(256, (3, 3), activation='relu', padding='same')(c3)
u1 = layers.UpSampling2D((2, 2))(c3)
merge1 = layers.concatenate([c2, u1], axis=3)
c4 = layers.Conv2D(128, (3, 3), activation='relu', padding='same')(merge1)
c4 = layers.Conv2D(128, (3, 3), activation='relu', padding='same')(c4)
u2 = layers.UpSampling2D((2, 2))(c4)
merge2 = layers.concatenate([c1, u2], axis=3)
c5 = layers.Conv2D(64, (3, 3), activation='relu', padding='same')(merge2)
c5 = layers.Conv2D(64, (3, 3), activation='relu', padding='same')(c5)
outputs = layers.Conv2D(1, (1, 1), activation='sigmoid')(c5)
model = models.Model(inputs=[inputs], outputs=[outputs])
return model
model = unet_model()
model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
model.summary()
Mask R-CNN
Mask R-CNN是一种用于实例分割的深度学习模型,它不仅可以检测物体,还可以为每个物体生成一个高质量的分割掩码。
import mrcnn.config
import mrcnn.utils
import mrcnn.model as modellib
from mrcnn import visualize
class InferenceConfig(mrcnn.config.Config):
NAME = "coco_inference"
GPU_COUNT = 1
IMAGES_PER_GPU = 1
NUM_CLASSES = 1 + 80 # COCO has 80 classes
config = InferenceConfig()
model = modellib.MaskRCNN(mode="inference", model_dir='./', config=config)
model.load_weights('mask_rcnn_coco.h5', by_name=True)
读取并处理图像
image = cv2.imread('image.jpg')
results = model.detect([image], verbose=1)
r = results[0]
显示结果
visualize.display_instances(image, r['rois'], r['masks'], r['class_ids'],
r['scores'], title="Predictions")
总结
图像分割是计算机视觉中的基本任务,方法多种多样。从简单的阈值分割和边缘检测,到复杂的深度学习模型,每种方法都有其适用的场景。本文详细介绍了几种常见的图像分割方法,并提供了相应的Python代码示例。通过这些方法,您可以根据具体需求选择适合的图像分割技术。
相关问答FAQs:
如何选择合适的图像分割算法?
在进行图像分割时,选择合适的算法至关重要。常见的算法包括阈值分割、区域生长、边缘检测和深度学习方法(如U-Net)。选择算法时需考虑图像的特性、分割的精度需求以及计算资源。对于简单的图像,阈值分割和边缘检测可能效果良好,而复杂场景下,深度学习方法通常能够提供更好的结果。
如何使用Python库进行图像分割?
Python中有多种库可以实现图像分割,如OpenCV、scikit-image和TensorFlow。使用OpenCV可以通过简单的函数调用进行阈值分割和轮廓检测,而scikit-image提供了更丰富的图像处理工具和算法。对于深度学习方法,则可以使用TensorFlow或PyTorch来构建和训练模型,进行更高级的分割任务。
图像分割后的结果如何评估?
评估图像分割效果的方法有多种,常见的包括交并比(IoU)、Dice系数和边界重合度。交并比通过计算预测分割与真实分割的交集与并集的比值来衡量分割的准确性,而Dice系数则更加关注重叠区域的大小。这些指标可以帮助开发者了解算法的性能,并在需要时进行参数调整或算法替换。
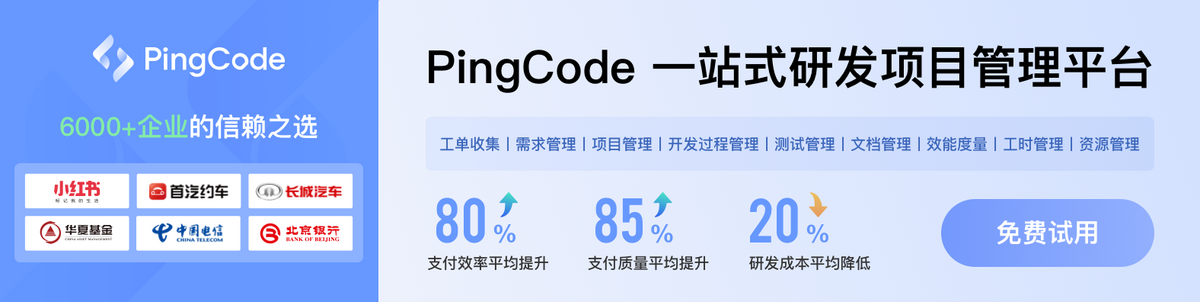