在Python中,使用Telnet连接服务器可以通过内置的telnetlib
库来实现。主要步骤包括:创建Telnet对象、连接到服务器、发送命令、读取响应。 下面我们将详细描述这些步骤,并给出一个完整的示例代码来展示如何实现这些操作。
一、连接服务器
使用telnetlib
库,首先需要创建一个Telnet对象并连接到指定的服务器。以下是详细步骤:
- 导入
telnetlib
库。 - 使用
Telnet()
方法创建一个Telnet对象。 - 使用
open()
方法连接到指定的服务器和端口。
import telnetlib
创建Telnet对象
tn = telnetlib.Telnet()
连接到服务器
tn.open('hostname', port)
二、发送命令
连接到服务器后,可以通过write()
方法发送命令。需要注意的是,发送的命令需要以字节串的形式,并且以换行符结尾。
# 发送命令
command = 'your_command\n'
tn.write(command.encode('ascii'))
三、读取响应
可以使用read_until()
或read_all()
方法来读取服务器的响应。read_until()
方法会一直读取,直到遇到指定的结束符;read_all()
方法会读取所有的数据,直到连接关闭。
# 读取响应
response = tn.read_until(b"expected_end_string").decode('ascii')
print(response)
四、关闭连接
完成操作后,记得关闭连接。
# 关闭连接
tn.close()
示例代码
下面是一个完整的示例代码,展示了如何使用telnetlib
库连接到服务器、发送命令并读取响应。
import telnetlib
def telnet_connect(hostname, port, command, end_string):
try:
# 创建Telnet对象
tn = telnetlib.Telnet()
# 连接到服务器
tn.open(hostname, port)
# 发送命令
tn.write((command + '\n').encode('ascii'))
# 读取响应
response = tn.read_until(end_string.encode('ascii')).decode('ascii')
print(response)
except Exception as e:
print(f"An error occurred: {e}")
finally:
# 关闭连接
tn.close()
示例使用
telnet_connect('your_hostname', 23, 'your_command', 'expected_end_string')
五、错误处理
在实际应用中,连接服务器的过程中可能会遇到各种错误,例如无法连接到服务器、发送命令失败等。为了提高代码的健壮性,需要进行错误处理。
import telnetlib
def telnet_connect(hostname, port, command, end_string):
try:
# 创建Telnet对象
tn = telnetlib.Telnet()
# 连接到服务器
tn.open(hostname, port)
# 发送命令
tn.write((command + '\n').encode('ascii'))
# 读取响应
response = tn.read_until(end_string.encode('ascii')).decode('ascii')
print(response)
except telnetlib.TelnetError as te:
print(f"Telnet error: {te}")
except ConnectionRefusedError:
print("Connection refused by the server")
except Exception as e:
print(f"An error occurred: {e}")
finally:
# 关闭连接
try:
tn.close()
except:
pass
示例使用
telnet_connect('your_hostname', 23, 'your_command', 'expected_end_string')
六、使用上下文管理器
为了确保连接能够正确关闭,可以使用上下文管理器来管理Telnet连接。通过定义一个上下文管理器类来封装Telnet连接和操作。
import telnetlib
class TelnetClient:
def __init__(self, hostname, port):
self.hostname = hostname
self.port = port
self.tn = None
def __enter__(self):
self.tn = telnetlib.Telnet(self.hostname, self.port)
return self.tn
def __exit__(self, exc_type, exc_value, traceback):
if self.tn:
self.tn.close()
def telnet_connect(hostname, port, command, end_string):
try:
with TelnetClient(hostname, port) as tn:
# 发送命令
tn.write((command + '\n').encode('ascii'))
# 读取响应
response = tn.read_until(end_string.encode('ascii')).decode('ascii')
print(response)
except telnetlib.TelnetError as te:
print(f"Telnet error: {te}")
except ConnectionRefusedError:
print("Connection refused by the server")
except Exception as e:
print(f"An error occurred: {e}")
示例使用
telnet_connect('your_hostname', 23, 'your_command', 'expected_end_string')
七、处理登录过程
在某些情况下,连接到Telnet服务器后需要进行登录。以下是一个处理登录过程的示例代码。
import telnetlib
def telnet_connect(hostname, port, username, password, command, end_string):
try:
# 创建Telnet对象
tn = telnetlib.Telnet(hostname, port)
# 读取登录提示并输入用户名
tn.read_until(b"login: ")
tn.write((username + '\n').encode('ascii'))
# 读取密码提示并输入密码
tn.read_until(b"Password: ")
tn.write((password + '\n').encode('ascii'))
# 发送命令
tn.write((command + '\n').encode('ascii'))
# 读取响应
response = tn.read_until(end_string.encode('ascii')).decode('ascii')
print(response)
except telnetlib.TelnetError as te:
print(f"Telnet error: {te}")
except ConnectionRefusedError:
print("Connection refused by the server")
except Exception as e:
print(f"An error occurred: {e}")
finally:
# 关闭连接
tn.close()
示例使用
telnet_connect('your_hostname', 23, 'your_username', 'your_password', 'your_command', 'expected_end_string')
八、使用外部库
除了telnetlib
,还可以使用第三方库如Exscript
来简化Telnet操作。Exscript
库支持更多高级功能,例如自动处理登录过程、命令调度等。
from Exscript.protocols import Telnet
def telnet_connect(hostname, port, username, password, command, end_string):
try:
# 创建Telnet对象
tn = Telnet()
tn.connect(hostname, port)
tn.login(username, password)
# 发送命令
tn.execute(command)
# 读取响应
response = tn.response
print(response)
except Exception as e:
print(f"An error occurred: {e}")
finally:
# 关闭连接
tn.close()
示例使用
telnet_connect('your_hostname', 23, 'your_username', 'your_password', 'your_command', 'expected_end_string')
总结
通过上述步骤和示例代码,我们详细介绍了如何在Python中使用Telnet连接服务器。无论是通过内置的telnetlib
库,还是使用第三方库如Exscript
,都可以实现连接服务器、发送命令并读取响应的功能。在实际应用中,根据具体需求选择合适的方法,并注意处理可能出现的错误和异常情况。
相关问答FAQs:
如何使用Python实现Telnet连接到服务器?
要通过Python连接到Telnet服务器,您可以使用telnetlib
库。首先,确保您的Python环境中已经安装了这个库。以下是一个简单的示例代码:
import telnetlib
# 设置服务器地址和端口
HOST = "your_server_address"
PORT = 23 # 默认Telnet端口
# 创建Telnet对象并连接
tn = telnetlib.Telnet(HOST, PORT)
# 登录,如果需要的话
tn.read_until(b"login: ")
tn.write(b"your_username\n")
tn.read_until(b"Password: ")
tn.write(b"your_password\n")
# 发送命令并获取输出
tn.write(b"your_command\n")
output = tn.read_all()
print(output.decode('utf-8'))
# 关闭连接
tn.close()
在Python中使用Telnet时需要注意哪些安全问题?
使用Telnet进行通信时,数据在网络中以明文形式传输,这意味着您的用户名、密码和其他敏感信息可能会被窃听。因此,建议在不安全的网络环境中使用SSH协议来代替Telnet,确保数据传输的安全性。如果必须使用Telnet,尽量在可信的网络环境中进行。
是否有其他Python库可以替代telnetlib?
除了telnetlib
,您还可以使用paramiko
库进行更安全的SSH连接。虽然paramiko
主要用于SSH,但它的功能更强大,能够提供更好的安全性和灵活性。如果您需要进行更复杂的操作,建议考虑使用该库。
如何处理Telnet连接中的异常情况?
在使用Telnet连接时,可能会遇到网络问题或身份验证失败等异常情况。建议在代码中添加异常处理,例如使用try-except
语句来捕获和处理EOFError
、socket.error
等异常,以便在出现问题时能够优雅地处理并给出用户友好的提示。
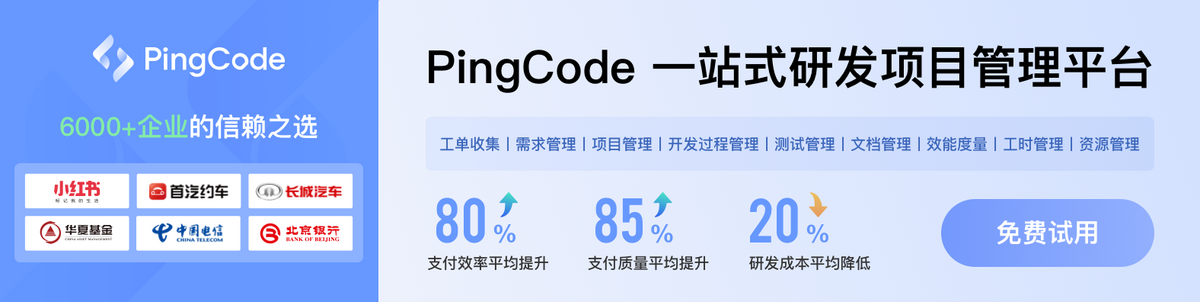