在Python中查找字符串的方法主要有:使用find()方法、使用index()方法、正则表达式、使用in操作符等。 这些方法各有优缺点,具体使用时可以根据需要选择适当的方法。以下是对find()方法的详细描述:
find()方法:这是一个内置方法,用于在字符串中查找子字符串,并返回子字符串在字符串中的最低索引,如果找不到子字符串,则返回-1。find()方法还可以指定搜索的开始和结束位置。find()方法是一个非常高效且简洁的字符串查找方式,适用于大多数简单的字符串查找需求。
str1 = "Hello, welcome to the world of Python."
result = str1.find("welcome")
if result != -1:
print(f"Substring found at index {result}")
else:
print("Substring not found")
一、使用find()方法查找字符串
find()方法是Python字符串对象的一个方法,用于查找子字符串在字符串中的位置。该方法返回子字符串的最低索引,如果找不到子字符串,则返回-1。find()方法还可以指定搜索的开始和结束位置。
# 示例代码
str1 = "Hello, welcome to the world of Python."
result = str1.find("welcome")
if result != -1:
print(f"Substring found at index {result}")
else:
print("Substring not found")
在上面的示例中,find()方法在字符串str1中查找子字符串"welcome",并返回其最低索引。如果找不到子字符串,则返回-1。
find()方法的语法如下:
str.find(sub[, start[, end]])
- sub:要查找的子字符串。
- start:可选参数,指定查找的起始位置。
- end:可选参数,指定查找的结束位置。
二、使用index()方法查找字符串
index()方法与find()方法类似,也用于查找子字符串在字符串中的位置。不同之处在于,如果找不到子字符串,index()方法会引发ValueError异常,而不是返回-1。
# 示例代码
str1 = "Hello, welcome to the world of Python."
try:
result = str1.index("welcome")
print(f"Substring found at index {result}")
except ValueError:
print("Substring not found")
在上面的示例中,index()方法在字符串str1中查找子字符串"welcome",并返回其最低索引。如果找不到子字符串,则引发ValueError异常。
index()方法的语法如下:
str.index(sub[, start[, end]])
- sub:要查找的子字符串。
- start:可选参数,指定查找的起始位置。
- end:可选参数,指定查找的结束位置。
三、使用正则表达式查找字符串
正则表达式(Regular Expression)是一个强大的工具,用于匹配字符串中的模式。Python的re模块提供了一组操作正则表达式的函数,可以用来查找字符串。
import re
示例代码
str1 = "Hello, welcome to the world of Python."
pattern = re.compile("welcome")
match = pattern.search(str1)
if match:
print(f"Substring found at index {match.start()}")
else:
print("Substring not found")
在上面的示例中,re.compile()函数用于编译正则表达式模式,返回一个模式对象。模式对象的search()方法在字符串str1中查找匹配的子字符串,并返回一个匹配对象。如果找不到匹配的子字符串,则返回None。
正则表达式的语法详见Python官方文档中的re模块。
四、使用in操作符查找字符串
in操作符用于检查子字符串是否存在于字符串中。如果子字符串存在,则返回True,否则返回False。
# 示例代码
str1 = "Hello, welcome to the world of Python."
if "welcome" in str1:
print("Substring found")
else:
print("Substring not found")
在上面的示例中,in操作符用于检查子字符串"welcome"是否存在于字符串str1中。如果存在,则返回True,否则返回False。
五、使用字符串方法count()查找字符串
count()方法用于返回子字符串在字符串中出现的次数。如果子字符串不存在于字符串中,则返回0。
# 示例代码
str1 = "Hello, welcome to the world of Python. Welcome again!"
count = str1.count("welcome")
print(f"Substring 'welcome' found {count} times")
在上面的示例中,count()方法用于统计子字符串"welcome"在字符串str1中出现的次数。
count()方法的语法如下:
str.count(sub[, start[, end]])
- sub:要查找的子字符串。
- start:可选参数,指定查找的起始位置。
- end:可选参数,指定查找的结束位置。
六、使用字符串方法startswith()和endswith()查找字符串
startswith()方法用于检查字符串是否以指定的子字符串开头,如果是则返回True,否则返回False。endswith()方法用于检查字符串是否以指定的子字符串结尾,如果是则返回True,否则返回False。
# 示例代码
str1 = "Hello, welcome to the world of Python."
if str1.startswith("Hello"):
print("String starts with 'Hello'")
if str1.endswith("Python."):
print("String ends with 'Python.'")
在上面的示例中,startswith()方法用于检查字符串str1是否以子字符串"Hello"开头,endswith()方法用于检查字符串str1是否以子字符串"Python."结尾。
七、使用列表推导式查找字符串
列表推导式是一种简洁的创建列表的方式,可以用于查找字符串中所有出现的子字符串的位置。
# 示例代码
str1 = "Hello, welcome to the world of Python. Welcome again!"
sub = "welcome"
positions = [i for i in range(len(str1)) if str1.startswith(sub, i)]
print(f"Substring '{sub}' found at positions {positions}")
在上面的示例中,列表推导式用于查找子字符串"welcome"在字符串str1中的所有出现位置,并返回这些位置的列表。
八、使用字符串方法partition()和rpartition()查找字符串
partition()方法用于将字符串分成三部分,第一部分是子字符串之前的部分,第二部分是子字符串,第三部分是子字符串之后的部分。如果子字符串不存在于字符串中,则返回字符串本身和两个空字符串。
rpartition()方法与partition()方法类似,不同之处在于它从字符串的右端开始查找子字符串。
# 示例代码
str1 = "Hello, welcome to the world of Python."
result = str1.partition("welcome")
print(result)
result = str1.rpartition("welcome")
print(result)
在上面的示例中,partition()方法用于将字符串str1分成三部分,rpartition()方法用于从右端开始查找子字符串"welcome",并将字符串分成三部分。
九、使用字符串方法split()和rsplit()查找字符串
split()方法用于将字符串按照指定的分隔符分割成列表。如果不指定分隔符,则默认使用空格分割。rsplit()方法与split()方法类似,不同之处在于它从字符串的右端开始分割。
# 示例代码
str1 = "Hello, welcome to the world of Python."
result = str1.split("welcome")
print(result)
result = str1.rsplit("welcome")
print(result)
在上面的示例中,split()方法用于将字符串str1按照子字符串"welcome"分割成列表,rsplit()方法用于从右端开始分割字符串str1。
十、使用字符串方法findall()查找字符串
findall()方法是re模块中的一个函数,用于查找字符串中所有匹配的子字符串,并返回一个列表。如果找不到匹配的子字符串,则返回一个空列表。
import re
示例代码
str1 = "Hello, welcome to the world of Python. Welcome again!"
pattern = re.compile("welcome", re.IGNORECASE)
matches = pattern.findall(str1)
print(f"Found {len(matches)} matches: {matches}")
在上面的示例中,re.compile()函数用于编译正则表达式模式,并指定忽略大小写的匹配,findall()方法用于查找字符串str1中所有匹配的子字符串,并返回一个包含所有匹配项的列表。
以上是Python中查找字符串的多种方法。根据实际需求和场景,可以选择最合适的方法来实现字符串查找。
相关问答FAQs:
如何在Python中查找字符串的特定子串?
在Python中,可以使用str.find()
、str.index()
或str.count()
等方法来查找特定的子串。find()
方法返回子串首次出现的索引,如果未找到则返回-1;而index()
方法在未找到时会引发异常。count()
方法则用来统计子串出现的次数。使用这些方法可以帮助你灵活地处理字符串查找的问题。
Python中是否支持正则表达式来查找字符串?
是的,Python的re
模块提供了强大的正则表达式支持,可以用来查找复杂模式的字符串。使用re.search()
、re.match()
和re.findall()
等函数,可以找到符合特定模式的字符串,非常适合处理更复杂的查找需求,例如匹配特定格式的文本或提取信息。
在查找字符串时,如何处理大小写敏感的问题?
如果需要忽略大小写进行字符串查找,可以使用str.lower()
或str.upper()
方法将字符串统一为小写或大写,然后再进行比较。例如,在查找时将目标字符串和待查找的字符串都转换为小写,这样就能确保大小写不同不会影响查找结果。这种方法非常实用,尤其是在处理用户输入时。
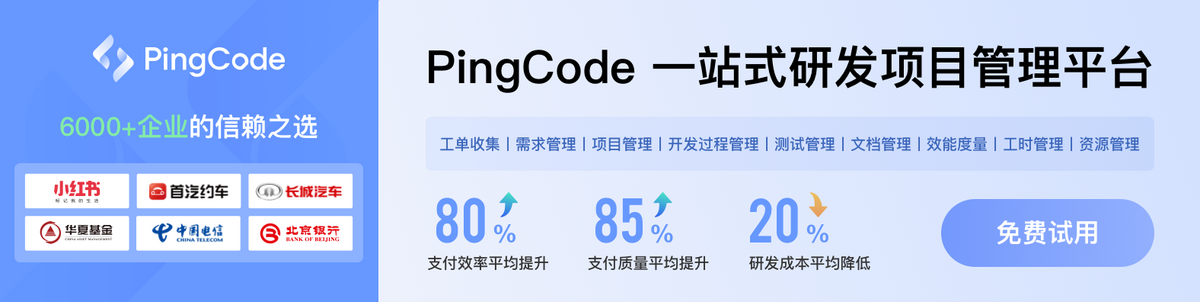