使用Python进入系统命令行可以通过 os
模块、 subprocess
模块、 sys
模块,推荐使用subprocess
模块,因为它更强大和灵活。
subprocess
模块允许你生成新的进程,连接它们的输入/输出/错误管道,并获取它们的返回码。它提供了一个更高级别的接口来执行系统命令。下面,我们将详细介绍如何在Python中使用不同的方法进入系统命令行,并执行命令。
一、使用os模块
os
模块是Python中用于与操作系统交互的模块之一,提供了一些简单的命令行调用方法。以下是如何使用os
模块执行系统命令:
1.1 os.system()
os.system()
是最简单的方法之一。它只接受一个字符串作为参数,该字符串是你想要执行的命令。这个方法适用于执行简单的命令:
import os
执行简单的系统命令
os.system('ls -l')
1.2 os.popen()
os.popen()
可以执行命令并获得其输出。它会返回一个文件对象,可以读取命令的输出:
import os
执行命令并读取输出
output = os.popen('ls -l').read()
print(output)
二、使用subprocess模块
subprocess
模块是Python中更强大和灵活的系统命令执行模块,推荐在大多数情况下使用它。
2.1 subprocess.run()
subprocess.run()
是Python 3.5引入的一个高级接口,适用于大多数用例。它返回一个CompletedProcess
对象,其中包含了命令的返回码、输出和错误信息:
import subprocess
执行命令并获取返回结果
result = subprocess.run(['ls', '-l'], capture_output=True, text=True)
print(result.stdout)
2.2 subprocess.Popen()
subprocess.Popen()
提供了更低级别的接口,适合需要更多控制的场景。可以使用它来执行命令并处理输入/输出/错误管道:
import subprocess
创建一个子进程
process = subprocess.Popen(['ls', '-l'], stdout=subprocess.PIPE, stderr=subprocess.PIPE)
获取输出和错误信息
stdout, stderr = process.communicate()
print(stdout.decode())
三、使用sys模块
sys
模块主要用于与Python解释器进行交互,不直接用于执行系统命令,但可以结合其他模块来实现更复杂的操作。
3.1 sys.argv
sys.argv
可以用来获取命令行参数,并结合其他模块执行命令:
import sys
import subprocess
获取命令行参数
command = sys.argv[1:]
执行命令并获取输出
result = subprocess.run(command, capture_output=True, text=True)
print(result.stdout)
3.2 sys.exit()
sys.exit()
可以用来终止Python脚本的执行,并返回一个状态码:
import sys
if len(sys.argv) < 2:
print("Usage: python script.py <command>")
sys.exit(1)
command = sys.argv[1]
四、处理命令输出
在执行系统命令时,处理命令的输出是一个常见的需求。以下是几种处理输出的方法:
4.1 捕获标准输出和错误输出
使用subprocess
模块,可以捕获标准输出和错误输出:
import subprocess
result = subprocess.run(['ls', '-l'], capture_output=True, text=True)
print("Standard Output:", result.stdout)
print("Error Output:", result.stderr)
4.2 将输出重定向到文件
可以将命令的输出重定向到文件:
import subprocess
with open('output.txt', 'w') as f:
subprocess.run(['ls', '-l'], stdout=f)
4.3 实时读取输出
在某些情况下,你可能需要实时读取命令的输出,可以使用subprocess.Popen()
实现:
import subprocess
process = subprocess.Popen(['ping', 'google.com'], stdout=subprocess.PIPE, text=True)
for line in process.stdout:
print(line.strip())
五、执行多个命令
有时需要在一个Python脚本中执行多个命令,可以使用subprocess
模块的不同方法来实现:
5.1 顺序执行命令
可以按顺序执行多个命令,并处理每个命令的输出:
import subprocess
commands = [
['ls', '-l'],
['pwd'],
['echo', 'Hello, World!']
]
for command in commands:
result = subprocess.run(command, capture_output=True, text=True)
print(result.stdout)
5.2 管道命令
可以使用管道将一个命令的输出作为另一个命令的输入:
import subprocess
创建第一个子进程
p1 = subprocess.Popen(['ls', '-l'], stdout=subprocess.PIPE)
创建第二个子进程,并将第一个子进程的输出作为输入
p2 = subprocess.Popen(['grep', '.py'], stdin=p1.stdout, stdout=subprocess.PIPE)
获取最终输出
output = p2.communicate()[0]
print(output.decode())
六、处理错误和异常
在执行系统命令时,处理可能发生的错误和异常是非常重要的。可以使用try
和except
语句来捕获和处理错误:
6.1 捕获异常
可以捕获subprocess
模块中可能发生的异常,并进行相应处理:
import subprocess
try:
result = subprocess.run(['ls', '-z'], capture_output=True, text=True, check=True)
except subprocess.CalledProcessError as e:
print("Command failed with return code", e.returncode)
print("Error output:", e.stderr)
6.2 检查命令返回码
可以检查命令的返回码,并根据返回码执行不同的操作:
import subprocess
result = subprocess.run(['ls', '-l'], capture_output=True, text=True)
if result.returncode == 0:
print("Command executed successfully")
else:
print("Command failed with return code", result.returncode)
七、跨平台兼容性
在编写Python脚本时,确保其在不同操作系统(如Windows、Linux、macOS)上兼容是非常重要的。可以使用platform
模块来检测操作系统,并根据操作系统执行不同的命令:
7.1 检测操作系统
使用platform
模块可以检测当前操作系统,并执行相应的命令:
import platform
import subprocess
os_type = platform.system()
if os_type == 'Windows':
command = ['dir']
elif os_type == 'Linux' or os_type == 'Darwin':
command = ['ls', '-l']
else:
raise NotImplementedError(f"Unsupported OS: {os_type}")
result = subprocess.run(command, capture_output=True, text=True)
print(result.stdout)
7.2 使用跨平台命令
可以使用一些跨平台的命令来提高兼容性,例如使用python
命令代替python3
或python.exe
:
import subprocess
command = ['python', '--version']
result = subprocess.run(command, capture_output=True, text=True)
print(result.stdout)
总结:通过上述方法,你可以在Python中灵活地进入系统命令行并执行各种命令。推荐使用subprocess
模块,因为它提供了更强大的功能和更高的灵活性。根据具体需求选择合适的方法,并确保处理好命令的输出、错误和异常,以编写健壮的Python脚本。
相关问答FAQs:
如何在Python中执行系统命令?
在Python中,可以使用内置的subprocess
模块来执行系统命令。通过这个模块,可以启动新进程、连接到它们的输入/输出/错误管道,并获取返回值。以下是一个简单的示例:
import subprocess
result = subprocess.run(['ls', '-l'], capture_output=True, text=True)
print(result.stdout)
这个代码会在Linux或Mac系统上列出当前目录的文件。
在Windows系统上如何使用Python运行命令提示符?
对于Windows用户,可以利用subprocess
模块打开命令提示符并执行命令。使用cmd
作为命令,并传递所需的参数。例如:
import subprocess
subprocess.run(['cmd', '/c', 'dir'])
这将列出当前目录中的文件。
如何处理Python中执行命令时的错误?
在使用subprocess
模块时,可以通过检查返回值来捕获和处理错误。将check=True
添加到subprocess.run()
方法中可以确保在命令执行失败时抛出异常。示例如下:
try:
subprocess.run(['ls', '-z'], check=True) # '-z'是一个无效参数
except subprocess.CalledProcessError as e:
print(f"命令执行失败: {e}")
这种方式可以帮助开发者在执行命令时更好地进行错误处理。
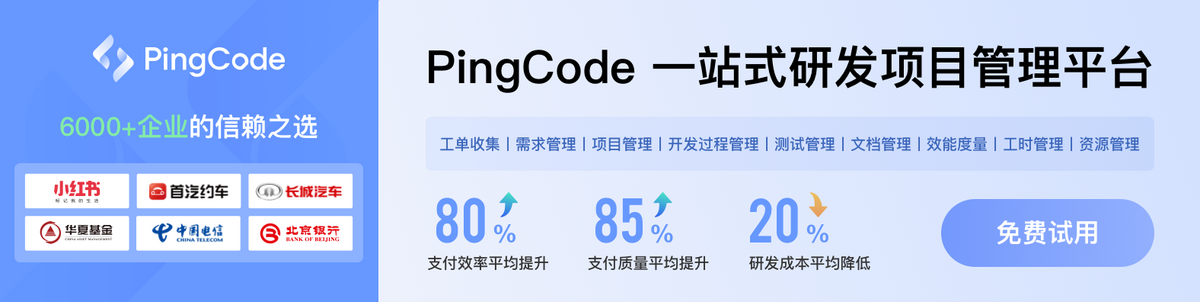