通过Python爬虫建立数据库的核心步骤包括:选择合适的爬虫工具、编写爬虫脚本、提取数据、清洗数据、选择数据库、将数据存入数据库。本文将详细描述如何通过Python爬虫从网页获取数据并存储到数据库中,重点介绍每个步骤的具体实现方法。
一、选择合适的爬虫工具
Python提供了多个优秀的爬虫工具,如BeautifulSoup、Scrapy和Selenium等。选择合适的工具取决于具体需求:
- BeautifulSoup:适用于简单的HTML页面解析。
- Scrapy:功能强大且高效,适用于大规模数据抓取。
- Selenium:适用于需要模拟用户操作的动态网页抓取。
BeautifulSoup
BeautifulSoup是一个用于解析HTML和XML的库,它与requests库配合使用,可以方便地抓取网页内容。
from bs4 import BeautifulSoup
import requests
url = 'http://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
print(soup.title)
Scrapy
Scrapy是一个强大的爬虫框架,适用于复杂的网站爬取。
import scrapy
class ExampleSpider(scrapy.Spider):
name = 'example'
start_urls = ['http://example.com']
def parse(self, response):
title = response.css('title::text').get()
yield {'title': title}
Selenium
Selenium适用于处理需要JavaScript渲染的动态网页。
from selenium import webdriver
driver = webdriver.Chrome()
driver.get('http://example.com')
title = driver.title
print(title)
driver.quit()
二、编写爬虫脚本
在选择了合适的工具后,下一步是编写爬虫脚本。以下是一个使用BeautifulSoup和requests库的示例。
import requests
from bs4 import BeautifulSoup
url = 'http://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
data = []
for item in soup.find_all('div', class_='item'):
title = item.find('h2').text
link = item.find('a')['href']
data.append({'title': title, 'link': link})
print(data)
三、提取数据
数据提取是爬虫的核心任务,通过解析网页内容,提取所需的信息。
for item in soup.find_all('div', class_='item'):
title = item.find('h2').text
link = item.find('a')['href']
data.append({'title': title, 'link': link})
四、清洗数据
在提取数据后,通常需要对数据进行清洗,以确保数据的准确性和一致性。
cleaned_data = []
for item in data:
if item['title'] and item['link']:
cleaned_data.append(item)
五、选择数据库
常见的数据库包括MySQL、PostgreSQL、SQLite等。选择合适的数据库取决于具体需求。
SQLite
SQLite是一个轻量级的嵌入式数据库,适用于小型项目。
import sqlite3
conn = sqlite3.connect('example.db')
c = conn.cursor()
c.execute('''CREATE TABLE IF NOT EXISTS data
(title TEXT, link TEXT)''')
for item in cleaned_data:
c.execute("INSERT INTO data (title, link) VALUES (?, ?)", (item['title'], item['link']))
conn.commit()
conn.close()
MySQL
MySQL适用于中大型项目,支持高并发和大数据量。
import mysql.connector
conn = mysql.connector.connect(user='user', password='password', host='127.0.0.1', database='example')
c = conn.cursor()
c.execute('''CREATE TABLE IF NOT EXISTS data
(title VARCHAR(255), link VARCHAR(255))''')
for item in cleaned_data:
c.execute("INSERT INTO data (title, link) VALUES (%s, %s)", (item['title'], item['link']))
conn.commit()
conn.close()
六、将数据存入数据库
将清洗后的数据存入数据库是爬虫工作的最后一步。
for item in cleaned_data:
c.execute("INSERT INTO data (title, link) VALUES (?, ?)", (item['title'], item['link']))
七、完整示例
以下是一个完整的示例,展示如何使用BeautifulSoup和SQLite从网页获取数据并存储到数据库中。
import requests
from bs4 import BeautifulSoup
import sqlite3
获取网页内容
url = 'http://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
提取数据
data = []
for item in soup.find_all('div', class_='item'):
title = item.find('h2').text
link = item.find('a')['href']
data.append({'title': title, 'link': link})
清洗数据
cleaned_data = []
for item in data:
if item['title'] and item['link']:
cleaned_data.append(item)
存储数据到SQLite数据库
conn = sqlite3.connect('example.db')
c = conn.cursor()
c.execute('''CREATE TABLE IF NOT EXISTS data
(title TEXT, link TEXT)''')
for item in cleaned_data:
c.execute("INSERT INTO data (title, link) VALUES (?, ?)", (item['title'], item['link']))
conn.commit()
conn.close()
通过以上步骤和示例代码,我们完成了从网页获取数据并存储到数据库的整个流程。希望这些内容能够帮助您更好地理解和实现Python爬虫与数据库的结合。
相关问答FAQs:
如何使用Python爬虫获取数据并存储到数据库中?
要通过Python爬虫获取数据并存储到数据库中,您需要首先选择合适的爬虫库,如BeautifulSoup或Scrapy,以提取网页内容。接下来,利用Python的数据库连接库(如SQLite、MySQL或PostgreSQL)来创建数据库和表格。您可以编写代码,将爬取到的数据格式化后插入到数据库中。确保在程序中处理异常情况,以避免在数据存储过程中出现错误。
在建立数据库之前,应该考虑哪些数据结构?
在建立数据库之前,需要仔细设计数据结构,包括确定数据表的字段、数据类型及其关系。这将有助于确保数据存储的高效性和查询的便捷性。常见的数据结构包括一对多和多对多关系,您还需要考虑索引的使用,以提高查询速度。根据爬取的数据特性,选择合适的字段,并合理规划主键和外键。
如何处理爬虫过程中遇到的反爬虫机制?
许多网站会实施反爬虫机制来保护其数据。在进行爬虫时,可以采取一些策略来绕过这些机制,例如使用随机的用户代理、设置请求间隔、使用代理IP或模拟人类行为。此外,解析网页时还应注意遵循网站的robots.txt文件,遵守相关法律法规,以避免不必要的麻烦。使用合适的工具和技术,可以有效地提高爬虫的成功率。
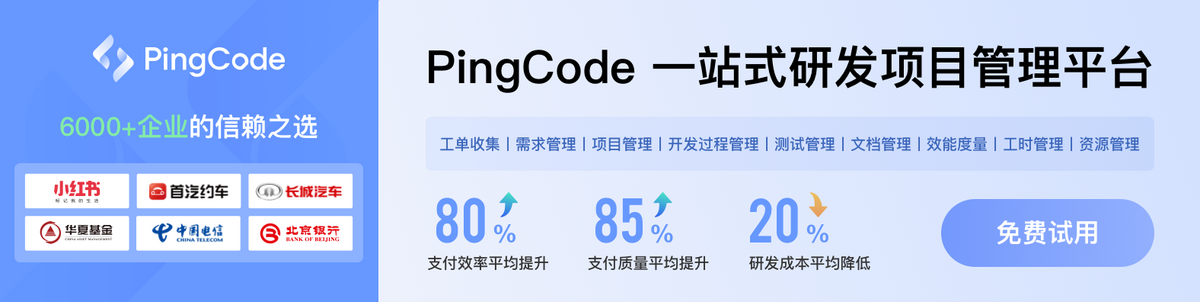