使用Python添加背景图片的方法有多种,包括使用PIL(Pillow)、OpenCV和Matplotlib库等。
PIL(Pillow)、OpenCV和Matplotlib库,这些库提供了丰富的图像处理功能,支持图像的加载、编辑和保存。在这篇文章中,我们将详细介绍如何使用这些库来添加背景图片。
一、PIL(Pillow)库
Pillow是Python Imaging Library(PIL)的一个分支,提供了许多图像处理功能。以下是使用Pillow添加背景图片的步骤:
- 安装Pillow库:
pip install pillow
- 使用Pillow加载和编辑图像:
from PIL import Image
加载前景图像和背景图像
foreground = Image.open("foreground.png")
background = Image.open("background.jpg")
确保背景图像和前景图像大小一致
background = background.resize(foreground.size)
创建一个新的图像,合并前景图像和背景图像
combined = Image.new("RGBA", foreground.size)
combined.paste(background, (0, 0))
combined.paste(foreground, (0, 0), mask=foreground)
保存结果
combined.save("combined_image.png")
在这个示例中,首先加载前景图像和背景图像,并将背景图像调整为与前景图像相同的大小。然后创建一个新的图像对象,使用paste
方法将背景图像和前景图像合并在一起,最后保存合成后的图像。
二、OpenCV库
OpenCV是一个强大的计算机视觉库,支持多种图像处理操作。以下是使用OpenCV添加背景图片的步骤:
- 安装OpenCV库:
pip install opencv-python
- 使用OpenCV加载和编辑图像:
import cv2
加载前景图像和背景图像
foreground = cv2.imread("foreground.png", cv2.IMREAD_UNCHANGED)
background = cv2.imread("background.jpg")
获取图像尺寸
fg_height, fg_width = foreground.shape[:2]
bg_height, bg_width = background.shape[:2]
调整背景图像大小
background = cv2.resize(background, (fg_width, fg_height))
分离前景图像的Alpha通道
b, g, r, a = cv2.split(foreground)
创建前景和背景的掩码
foreground_mask = cv2.merge((b, g, r))
alpha_mask = cv2.merge((a, a, a)) / 255.0
inverse_alpha_mask = 1.0 - alpha_mask
合并前景图像和背景图像
for c in range(0, 3):
background[:, :, c] = background[:, :, c] * inverse_alpha_mask[:, :, c] + foreground_mask[:, :, c] * alpha_mask[:, :, c]
保存结果
cv2.imwrite("combined_image.png", background)
在这个示例中,我们首先加载前景图像和背景图像,并调整背景图像的大小以匹配前景图像。然后,我们分离前景图像的Alpha通道,创建前景和背景的掩码,并使用这些掩码合并前景图像和背景图像。
三、Matplotlib库
Matplotlib主要用于数据可视化,但也可以用于简单的图像处理操作。以下是使用Matplotlib添加背景图片的步骤:
- 安装Matplotlib库:
pip install matplotlib
- 使用Matplotlib加载和编辑图像:
import matplotlib.pyplot as plt
import matplotlib.image as mpimg
import numpy as np
加载前景图像和背景图像
foreground = mpimg.imread("foreground.png")
background = mpimg.imread("background.jpg")
确保背景图像和前景图像大小一致
background = np.resize(background, foreground.shape)
创建前景和背景的掩码
alpha_mask = foreground[:, :, 3] / 255.0
inverse_alpha_mask = 1.0 - alpha_mask
合并前景图像和背景图像
for c in range(0, 3):
background[:, :, c] = background[:, :, c] * inverse_alpha_mask + foreground[:, :, c] * alpha_mask
显示和保存结果
plt.imshow(background)
plt.axis('off')
plt.savefig("combined_image.png", bbox_inches='tight', pad_inches=0)
plt.show()
在这个示例中,我们使用Matplotlib加载前景图像和背景图像,并调整背景图像的大小以匹配前景图像。然后,我们创建前景和背景的掩码,并使用这些掩码合并前景图像和背景图像。最后,显示并保存合成后的图像。
总结:
使用PIL(Pillow)、OpenCV和Matplotlib库,我们可以轻松地在Python中添加背景图片。每个库都有其独特的功能和优点,您可以根据具体需求选择合适的库进行图像处理。希望本文的详细介绍能帮助您理解和掌握这些方法,并应用到您的项目中。
相关问答FAQs:
如何在Python中使用图形库添加背景图片?
在Python中,常用的图形库如Pygame、Tkinter和Matplotlib等都可以用来添加背景图片。以Pygame为例,您可以使用pygame.image.load()
函数加载图片,并通过blit()
方法将其绘制到屏幕上。您只需确保在绘制其他图形之前先绘制背景图片,这样可以确保其显示在最底层。
添加背景图片时需要考虑哪些图片格式?
在Python中,常见的支持格式包括PNG、JPEG和BMP等。PNG格式通常是最佳选择,因为它支持透明度并能保持较高的图像质量。确保所使用的库支持您选择的图片格式,这样您就可以顺利加载和显示背景图片。
如何调整背景图片的大小以适应窗口?
如果您想调整背景图片的大小以适应窗口,您可以使用图形库提供的图像缩放功能。例如,在Pygame中,可以使用pygame.transform.scale()
函数来调整图像的尺寸。只需传入目标尺寸和原图像,即可获得缩放后的图像,方便您将其作为背景使用。
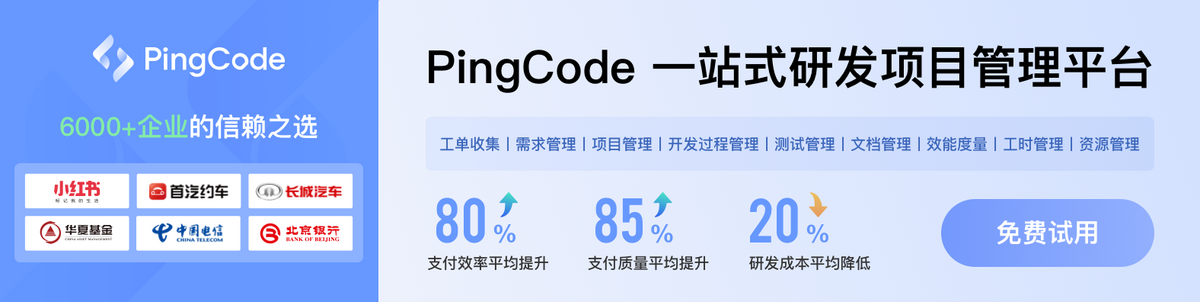