使用Python做图片浏览器的主要步骤包括:使用Tkinter创建GUI、使用Pillow处理图像、实现图像导航功能。
Tkinter是Python的标准GUI库,适合快速开发桌面应用。通过Tkinter,我们可以创建一个窗口来显示图像并添加按钮以浏览图像。Pillow是Python Imaging Library (PIL) 的分支,提供了强大的图像处理功能。我们可以使用Pillow加载和处理图像。
接下来,我们将详细描述如何使用Python制作一个简单的图片浏览器。
一、环境准备
在开始之前,需要确保安装了所需的Python库。可以使用以下命令安装Tkinter和Pillow:
pip install pillow
二、创建主窗口
首先,我们需要创建一个基础的Tkinter窗口。这个窗口将包含一个用于显示图像的Label和用于导航的按钮。
import tkinter as tk
from tkinter import filedialog
from PIL import Image, ImageTk
class ImageBrowser(tk.Tk):
def __init__(self):
super().__init__()
self.title("Python 图片浏览器")
self.geometry("800x600")
self.image_label = tk.Label(self)
self.image_label.pack(expand=True)
self.prev_button = tk.Button(self, text="上一张", command=self.show_prev_image)
self.prev_button.pack(side=tk.LEFT, padx=10, pady=10)
self.next_button = tk.Button(self, text="下一张", command=self.show_next_image)
self.next_button.pack(side=tk.RIGHT, padx=10, pady=10)
self.image_list = []
self.current_image_index = 0
self.load_images()
def load_images(self):
file_paths = filedialog.askopenfilenames(filetypes=[("Image files", "*.jpg *.jpeg *.png *.gif")])
self.image_list = [Image.open(file_path) for file_path in file_paths]
if self.image_list:
self.show_image(self.current_image_index)
def show_image(self, index):
image = self.image_list[index]
photo = ImageTk.PhotoImage(image)
self.image_label.config(image=photo)
self.image_label.image = photo
def show_prev_image(self):
if self.image_list:
self.current_image_index = (self.current_image_index - 1) % len(self.image_list)
self.show_image(self.current_image_index)
def show_next_image(self):
if self.image_list:
self.current_image_index = (self.current_image_index + 1) % len(self.image_list)
self.show_image(self.current_image_index)
if __name__ == "__main__":
app = ImageBrowser()
app.mainloop()
三、详细说明代码实现
1、主窗口和基本控件
我们首先创建了一个继承自tk.Tk
的ImageBrowser
类,并在初始化方法中设置了窗口的标题和大小。然后,我们创建了一个Label用于显示图像,两个按钮用于浏览图像,并将它们放置在窗口的不同位置。
2、加载图像
我们定义了一个load_images
方法,该方法使用filedialog.askopenfilenames
打开一个文件选择对话框,并让用户选择要浏览的图像文件。选择的文件路径保存在file_paths
中,我们使用Pillow的Image.open
方法加载这些图像,并将它们保存在self.image_list
中。
3、显示图像
我们定义了一个show_image
方法,该方法从self.image_list
中获取当前索引的图像,并使用ImageTk.PhotoImage
将其转换为Tkinter可以显示的格式。然后,我们将转换后的图像设置为self.image_label
的内容。
4、导航功能
我们定义了show_prev_image
和show_next_image
方法,这两个方法分别用于显示上一张和下一张图像。在这两个方法中,我们通过调整self.current_image_index
的值来实现图像的导航,并调用show_image
方法更新显示的图像。
四、改进和扩展
以上代码实现了一个基本的图片浏览器,我们可以根据需求进一步改进和扩展这个应用。
1、添加缩放功能
我们可以添加按钮或鼠标事件来实现图像的放大和缩小。通过调整图像的大小,可以实现缩放功能。
def zoom_in(self):
if self.image_list:
image = self.image_list[self.current_image_index]
width, height = image.size
image = image.resize((int(width * 1.2), int(height * 1.2)), Image.ANTIALIAS)
self.image_list[self.current_image_index] = image
self.show_image(self.current_image_index)
def zoom_out(self):
if self.image_list:
image = self.image_list[self.current_image_index]
width, height = image.size
image = image.resize((int(width * 0.8), int(height * 0.8)), Image.ANTIALIAS)
self.image_list[self.current_image_index] = image
self.show_image(self.current_image_index)
在初始化方法中添加对应的按钮:
self.zoom_in_button = tk.Button(self, text="放大", command=self.zoom_in)
self.zoom_in_button.pack(side=tk.LEFT, padx=10, pady=10)
self.zoom_out_button = tk.Button(self, text="缩小", command=self.zoom_out)
self.zoom_out_button.pack(side=tk.RIGHT, padx=10, pady=10)
2、添加旋转功能
我们可以添加按钮来实现图像的旋转功能。通过旋转图像,可以实现不同角度的查看。
def rotate_left(self):
if self.image_list:
image = self.image_list[self.current_image_index]
image = image.rotate(90, expand=True)
self.image_list[self.current_image_index] = image
self.show_image(self.current_image_index)
def rotate_right(self):
if self.image_list:
image = self.image_list[self.current_image_index]
image = image.rotate(-90, expand=True)
self.image_list[self.current_image_index] = image
self.show_image(self.current_image_index)
在初始化方法中添加对应的按钮:
self.rotate_left_button = tk.Button(self, text="左旋", command=self.rotate_left)
self.rotate_left_button.pack(side=tk.LEFT, padx=10, pady=10)
self.rotate_right_button = tk.Button(self, text="右旋", command=self.rotate_right)
self.rotate_right_button.pack(side=tk.RIGHT, padx=10, pady=10)
3、添加全屏查看功能
我们可以添加一个按钮来切换全屏模式,这样用户可以全屏查看图像。
def toggle_fullscreen(self):
self.attributes("-fullscreen", not self.attributes("-fullscreen"))
def exit_fullscreen(self, event=None):
self.attributes("-fullscreen", False)
在初始化方法中添加对应的按钮和绑定键盘事件:
self.fullscreen_button = tk.Button(self, text="全屏", command=self.toggle_fullscreen)
self.fullscreen_button.pack(side=tk.BOTTOM, padx=10, pady=10)
self.bind("<Escape>", self.exit_fullscreen)
五、总结
通过上述步骤,我们实现了一个基本的图片浏览器,并添加了一些实用的功能,如缩放、旋转和全屏查看。这个应用示例展示了如何使用Tkinter和Pillow库来创建一个功能丰富的图像浏览应用。根据实际需求,我们可以进一步扩展和改进这个应用,使其更加完善和实用。
相关问答FAQs:
如何用Python制作一个简单的图片浏览器?
制作一个简单的图片浏览器可以使用Python的Tkinter库来创建图形用户界面(GUI)。您可以通过加载图片文件并在窗口中显示它们来实现。首先,安装Pillow库以处理图像文件,然后使用Tkinter构建界面,添加按钮来浏览文件并显示选定的图片。
支持哪些图片格式?
大多数情况下,使用Pillow库可以支持多种图片格式,包括JPEG、PNG、GIF和BMP等。确保您在程序中处理这些格式时,使用适当的函数来加载和显示图像,以保证兼容性和良好的用户体验。
如何优化图片浏览器的性能?
为了提升图片浏览器的性能,可以考虑以下几点:使用缩略图来减少内存占用,延迟加载图片以提高初始加载速度,以及在显示大图时使用异步加载技术。此外,合理管理内存和文件缓存也能显著提高应用的响应速度。
可以添加哪些额外功能来增强图片浏览器的用户体验?
在图片浏览器中,可以加入多种功能来提升用户体验。例如,支持图片的放大缩小、旋转、幻灯片播放模式、文件夹浏览选择、以及收藏夹功能。此外,提供简单的图像编辑工具(如裁剪、调整亮度和对比度)也是一种很好的选择。
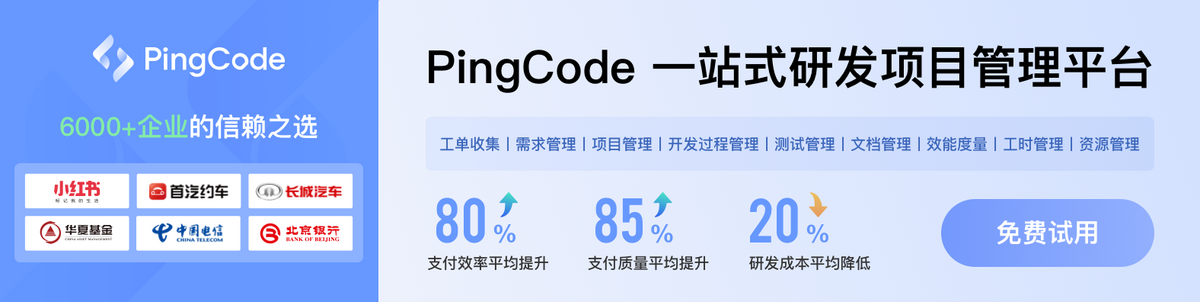