在Python中,要将温度符号放在前面,可以使用字符串格式化方法将温度符号和数值进行组合。 常见的方法包括使用f-strings、str.format()
方法以及字符串连接。以下是一些具体的方法和示例:
使用f-strings
f-strings是Python 3.6引入的一种字符串格式化方法,使用方便且高效。下面是一个示例:
temperature = 25
temperature_symbol = "°C"
formatted_temperature = f"{temperature_symbol} {temperature}"
print(formatted_temperature)
在这个示例中,f"{temperature_symbol} {temperature}"
会将温度符号和数值组合成一个字符串,然后输出为°C 25
。
使用str.format()
方法
str.format()
方法是另一种常见的字符串格式化方法,适用于Python 2.7及以上版本。以下是一个示例:
temperature = 25
temperature_symbol = "°C"
formatted_temperature = "{} {}".format(temperature_symbol, temperature)
print(formatted_temperature)
这里,"{} {}".format(temperature_symbol, temperature)
会将温度符号和数值组合成一个字符串,然后输出为°C 25
。
使用字符串连接
字符串连接是最基本的方法,通过将字符串和数值转换为字符串后进行连接。以下是一个示例:
temperature = 25
temperature_symbol = "°C"
formatted_temperature = temperature_symbol + " " + str(temperature)
print(formatted_temperature)
在这个示例中,通过temperature_symbol + " " + str(temperature)
将温度符号和数值连接成一个字符串,然后输出为°C 25
。
详细描述f-strings
f-strings(格式化字符串字面量)是Python 3.6及以上版本引入的一种字符串格式化方法,具有简洁和高效的特点。 它通过在字符串前添加字母f
或F
,然后在字符串内部使用大括号{}
包裹表达式来进行格式化。以下是一些使用f-strings的详细示例:
基本用法
temperature = 25
temperature_symbol = "°C"
formatted_temperature = f"{temperature_symbol} {temperature}"
print(formatted_temperature)
在这个示例中,f"{temperature_symbol} {temperature}"
会将temperature_symbol
和temperature
变量的值插入到字符串中,输出为°C 25
。
表达式求值
f-strings不仅可以插入变量,还可以在大括号内进行表达式求值:
temperature_celsius = 25
temperature_fahrenheit = (temperature_celsius * 9/5) + 32
formatted_temperature = f"{temperature_celsius}°C is {temperature_fahrenheit:.2f}°F"
print(formatted_temperature)
在这个示例中,大括号内包含了一个表达式temperature_fahrenheit:.2f
,表示将温度以两位小数的形式输出,结果为25°C is 77.00°F
。
使用函数调用
f-strings还可以在大括号内调用函数:
def convert_to_fahrenheit(celsius):
return (celsius * 9/5) + 32
temperature_celsius = 25
formatted_temperature = f"{temperature_celsius}°C is {convert_to_fahrenheit(temperature_celsius):.2f}°F"
print(formatted_temperature)
在这个示例中,大括号内调用了convert_to_fahrenheit
函数,将摄氏温度转换为华氏温度,结果为25°C is 77.00°F
。
其他格式化方法
除了上述三种方法外,还有一些其他的字符串格式化方法,如旧式的百分号%
格式化。虽然这些方法较少使用,但在某些情况下仍然有用:
百分号%
格式化
temperature = 25
temperature_symbol = "°C"
formatted_temperature = "%s %d" % (temperature_symbol, temperature)
print(formatted_temperature)
在这个示例中,"%s %d" % (temperature_symbol, temperature)
会将温度符号和数值插入到字符串中,输出为°C 25
。
小结
在Python中,有多种方法可以将温度符号放在前面。其中,f-strings是最简洁和高效的选择,适用于Python 3.6及以上版本。 其他方法如str.format()
、字符串连接和百分号%
格式化也可以根据具体需求使用。在实际开发中,可以根据代码的可读性和维护性选择合适的方法。
相关问答FAQs:
如何在Python中实现温度符号的前置显示?
在Python中,要将温度符号放在数值前面,可以通过字符串格式化来实现。例如,使用f-string
或format()
方法将符号与数字连接在一起。示例代码如下:
temperature = 25
formatted_temp = f"℃{temperature}"
print(formatted_temp)
这样可以确保符号在数字前面显示。
是否可以在Python中自定义温度符号的位置?
当然可以。在Python中,通过字符串操作,可以轻松地定义温度符号的位置。例如,可以通过以下代码将符号放在数字后面:
temperature = 25
formatted_temp = f"{temperature}℃"
print(formatted_temp)
这样可以灵活处理符号的位置。
在Python中如何处理不同的温度单位?
处理不同温度单位(如摄氏度、华氏度和开尔文)时,可以使用条件语句来格式化输出。例如:
temperature = 25
unit = "C" # 可以是 "C", "F", "K"
if unit == "C":
formatted_temp = f"℃{temperature}"
elif unit == "F":
formatted_temp = f"℉{(temperature * 9/5) + 32}"
else: # 假设单位为K
formatted_temp = f"K{temperature + 273.15}"
print(formatted_temp)
这样可以根据不同单位的需要进行相应的显示。
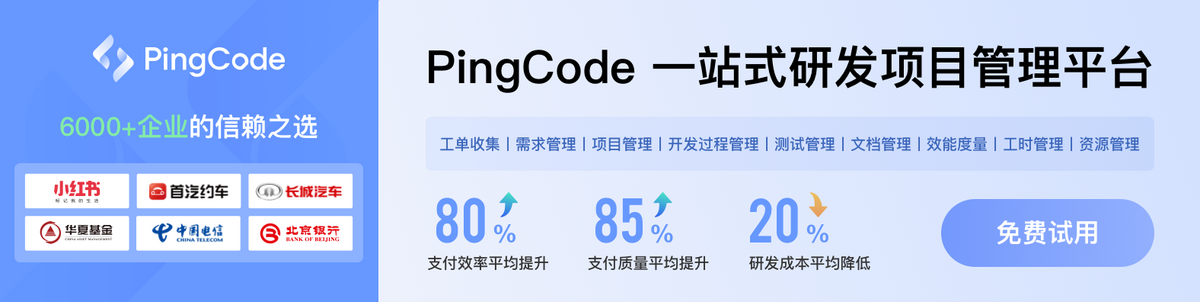