Python爬虫可以通过多种方式抓取网页数据库内容,主要方法包括使用requests库进行HTTP请求、BeautifulSoup库解析HTML内容、Selenium库进行动态网页抓取、Scrapy框架进行大规模数据抓取、以及利用API接口直接获取数据。在这些方法中,requests库进行HTTP请求、BeautifulSoup库解析HTML内容、Selenium库进行动态网页抓取是最常用的。接下来,我们详细介绍如何使用这些方法来爬取网页数据库。
一、使用requests库进行HTTP请求
requests库是Python中最常用的HTTP库,能够发送所有HTTP请求类型。以下是其使用步骤:
-
安装requests库:
pip install requests
-
发送HTTP请求:
import requests
url = "http://example.com"
response = requests.get(url)
html_content = response.content
-
处理响应内容:
if response.status_code == 200:
print("Request was successful")
print(html_content)
else:
print(f"Request failed with status code {response.status_code}")
二、使用BeautifulSoup库解析HTML内容
BeautifulSoup库用于解析HTML和XML文档。结合requests库,可以轻松解析网页内容。
-
安装BeautifulSoup库:
pip install beautifulsoup4
-
解析HTML内容:
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'html.parser')
-
提取数据:
titles = soup.find_all('h1')
for title in titles:
print(title.get_text())
三、使用Selenium库进行动态网页抓取
Selenium库能够模拟浏览器操作,适用于需要加载JavaScript内容的动态网页。
-
安装Selenium库及浏览器驱动:
pip install selenium
下载与浏览器版本匹配的webdriver,例如chromedriver
-
使用Selenium进行网页抓取:
from selenium import webdriver
driver = webdriver.Chrome(executable_path='/path/to/chromedriver')
driver.get("http://example.com")
page_source = driver.page_source
driver.quit()
-
结合BeautifulSoup解析内容:
soup = BeautifulSoup(page_source, 'html.parser')
data = soup.find_all('div', class_='data-class')
for item in data:
print(item.get_text())
四、使用Scrapy框架进行大规模数据抓取
Scrapy是一个功能强大的网页抓取框架,适用于大规模数据抓取任务。
-
安装Scrapy框架:
pip install scrapy
-
创建Scrapy项目:
scrapy startproject myproject
-
定义爬虫:
# myproject/spiders/my_spider.py
import scrapy
class MySpider(scrapy.Spider):
name = 'my_spider'
start_urls = ['http://example.com']
def parse(self, response):
for title in response.xpath('//h1/text()').getall():
yield {'title': title}
-
运行爬虫:
scrapy crawl my_spider
五、利用API接口直接获取数据
许多网站提供API接口,可以直接从API获取结构化数据,而不需要解析HTML。
-
发送API请求:
import requests
api_url = "http://api.example.com/data"
response = requests.get(api_url)
json_data = response.json()
-
处理API响应:
for item in json_data:
print(item)
结论
通过以上方法,我们可以使用Python爬取网页数据库内容。requests库与BeautifulSoup库的结合、Selenium库的动态网页抓取是实现网页爬虫的常用方法。根据具体需求,选择合适的工具和方法,能够高效地获取所需数据。
相关问答FAQs:
如何使用Python进行网页爬虫,获取数据库信息?
使用Python进行网页爬虫,可以通过库如BeautifulSoup和Scrapy来解析网页内容,并提取所需的数据。首先,使用requests库获取网页的HTML内容,然后利用BeautifulSoup对HTML进行解析,提取出需要的数据库信息。Scrapy则提供了更为强大的框架来处理大规模爬虫任务,包括数据存储和异步请求等功能。
在进行爬虫时,如何处理反爬虫机制?
反爬虫机制通常通过检测访问频率、IP地址、用户代理等来阻止爬虫行为。为了有效应对反爬虫措施,可以使用代理IP池、设置随机的用户代理、增加请求间隔等方法。此外,模拟浏览器行为,例如使用Selenium库来执行动态加载的网页,也可以帮助绕过一些简单的反爬虫技术。
获取的数据如何进行存储和管理?
爬取到的数据可以选择多种存储方式。对于小规模的数据,可以直接存储到CSV或JSON文件中,方便后续分析。如果数据量较大,建议使用数据库,如SQLite、MySQL或MongoDB等,以便于管理和查询。此外,可以使用Pandas库对数据进行进一步处理和分析,提升数据的利用效率。
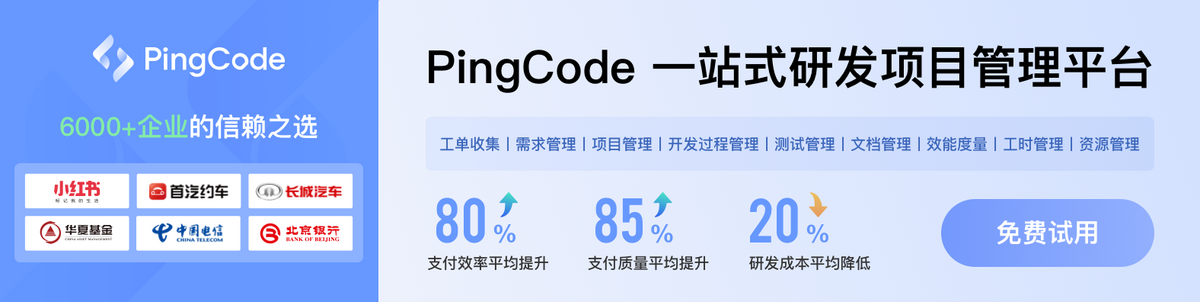