Python 实现大屏可视化的方法有很多,可以使用如 Matplotlib、Plotly、Dash、Bokeh、Streamlit 等库来创建交互式和动态的可视化图表。 在这些库中,Dash 是一个特别强大和灵活的工具,它可以结合 Plotly 图表和 Flask 后端来创建复杂的大屏可视化应用。下面我们将详细介绍如何使用 Dash 来实现 Python 大屏可视化。
一、安装和初步设置
在开始之前,确保你已经安装了 Dash 和 Plotly。这些库可以通过 pip 安装:
pip install dash plotly
安装完成后,你可以开始创建你的第一个 Dash 应用程序。
二、创建基本的 Dash 应用
Dash 应用程序由两个主要部分组成:布局(layout)和 回调函数(callbacks)。布局定义了应用程序的外观,而回调函数则定义了应用程序的交互逻辑。
import dash
import dash_core_components as dcc
import dash_html_components as html
from dash.dependencies import Input, Output
app = dash.Dash(__name__)
app.layout = html.Div(children=[
html.H1(children='Hello Dash'),
html.Div(children='''
Dash: A web application framework for Python.
'''),
dcc.Graph(
id='example-graph',
figure={
'data': [
{'x': [1, 2, 3], 'y': [4, 1, 2], 'type': 'bar', 'name': 'SF'},
{'x': [1, 2, 3], 'y': [2, 4, 5], 'type': 'bar', 'name': 'NYC'},
],
'layout': {
'title': 'Dash Data Visualization'
}
}
)
])
if __name__ == '__main__':
app.run_server(debug=True)
这段代码创建了一个简单的 Dash 应用程序,它包含一个标题和一个条形图。
三、动态数据和交互
为了使图表动态化,我们可以使用回调函数。回调函数允许我们根据用户输入来更新图表。
import dash
import dash_core_components as dcc
import dash_html_components as html
from dash.dependencies import Input, Output
import plotly.express as px
import pandas as pd
app = dash.Dash(__name__)
Sample data
df = pd.DataFrame({
"Fruit": ["Apples", "Oranges", "Bananas", "Apples", "Oranges", "Bananas"],
"Amount": [4, 1, 2, 2, 4, 5],
"City": ["SF", "SF", "SF", "NYC", "NYC", "NYC"]
})
fig = px.bar(df, x="Fruit", y="Amount", color="City", barmode="group")
app.layout = html.Div(children=[
html.H1(children='Dynamic Dash Visualization'),
dcc.Dropdown(
id='city-dropdown',
options=[
{'label': 'San Francisco', 'value': 'SF'},
{'label': 'New York City', 'value': 'NYC'}
],
value='SF'
),
dcc.Graph(
id='dynamic-graph',
figure=fig
)
])
@app.callback(
Output('dynamic-graph', 'figure'),
[Input('city-dropdown', 'value')]
)
def update_graph(selected_city):
filtered_df = df[df['City'] == selected_city]
fig = px.bar(filtered_df, x="Fruit", y="Amount", color="City", barmode="group")
return fig
if __name__ == '__main__':
app.run_server(debug=True)
在这个例子中,用户可以通过下拉菜单选择不同的城市,图表将动态更新以显示所选城市的数据。
四、布局美化和响应式设计
Dash 提供了多种 HTML 组件和 CSS 样式选项,使得创建响应式和美观的布局变得简单。
app.layout = html.Div(children=[
html.H1(children='Responsive Dash Application', style={'textAlign': 'center', 'color': '#7FDBFF'}),
html.Div(children='''
Dash: A web application framework for Python.
''', style={'textAlign': 'center'}),
dcc.Dropdown(
id='city-dropdown',
options=[
{'label': 'San Francisco', 'value': 'SF'},
{'label': 'New York City', 'value': 'NYC'}
],
value='SF',
style={'width': '50%', 'margin': 'auto'}
),
dcc.Graph(
id='dynamic-graph'
)
], style={'width': '80%', 'margin': 'auto'})
五、集成更多图表和组件
除了条形图,Dash 还支持多种其他类型的图表,如散点图、折线图、饼图等。你可以通过组合多个图表和组件来创建复杂的可视化应用。
app.layout = html.Div(children=[
html.H1(children='Complex Dash Application'),
html.Div(children=[
dcc.Graph(
id='line-chart',
figure=px.line(df, x="Fruit", y="Amount", color="City", title="Line Chart")
),
dcc.Graph(
id='scatter-plot',
figure=px.scatter(df, x="Fruit", y="Amount", color="City", title="Scatter Plot")
)
], style={'display': 'flex', 'flex-direction': 'row'}),
dcc.Dropdown(
id='city-dropdown',
options=[
{'label': 'San Francisco', 'value': 'SF'},
{'label': 'New York City', 'value': 'NYC'}
],
value='SF',
style={'width': '50%', 'margin': 'auto'}
),
dcc.Graph(
id='dynamic-graph'
)
])
六、部署 Dash 应用
一旦你完成了 Dash 应用的开发,可以将其部署到服务器上,如 Heroku、AWS 或其他云平台。
pip install gunicorn
创建一个 Procfile
文件:
web: gunicorn app:server
然后使用 git 部署到 Heroku:
git init
heroku create
git add .
git commit -m "Initial commit"
git push heroku master
七、总结
通过以上步骤,我们展示了如何使用 Dash 创建一个 Python 大屏可视化应用。从基本的安装和设置,到创建动态数据和交互,再到布局美化和响应式设计,最终到部署应用,整个过程涵盖了 Dash 的核心功能和使用方法。Dash 是一个强大且灵活的工具,适用于各种大屏可视化需求,无论是简单的图表展示还是复杂的交互式应用,都可以轻松实现。
相关问答FAQs:
如何选择适合大屏可视化的Python库?
在进行大屏可视化时,选择合适的Python库至关重要。常用的库包括Matplotlib、Seaborn、Plotly和Bokeh等。Matplotlib适合基本的图表绘制,Seaborn则能够增强数据的美观性。Plotly和Bokeh则提供了交互性和动态效果,特别适合大屏展示。根据项目需求和数据复杂性,选择最适合的库可以显著提升可视化效果。
大屏可视化中如何处理实时数据?
大屏可视化通常需要展示实时数据,这要求程序能够动态更新图表。可以使用Flask或Django等Web框架构建后端服务,通过WebSocket或定时轮询实现数据的实时更新。在前端,可以借助JavaScript库如D3.js与Python后端结合,创建流畅的动态效果。这种方法能保证用户在观看时获得最新的数据展示。
在大屏可视化项目中,如何优化性能?
为了确保大屏可视化的流畅性,优化性能是必须考虑的因素。可以通过减少绘制的图形数量、简化图形复杂度以及使用合适的分辨率来提升性能。此外,使用数据抽样技术和缓存机制也能有效降低系统负担,确保在高负载情况下依然能够流畅运行。合理的性能优化策略将直接影响用户的体验和数据展示的效果。
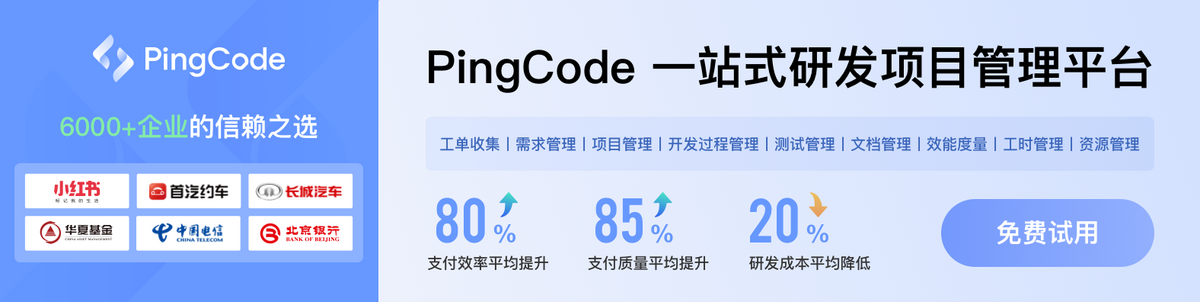