在 Python 中显示数据库中的图像类型数据可以通过以下几个步骤来实现:
连接数据库、获取图像数据、将图像数据转换为可显示的格式、显示图像。 其中,连接数据库可以使用 pymysql
或 sqlite3
等库,获取图像数据后需要将其转换成适合显示的格式,可以使用 PIL
(Python Imaging Library)或 opencv
等库来处理图像数据,最后在窗口或网页上显示图像。以下详细介绍这些步骤。
一、连接数据库
在开始之前,确保你已经安装了相关的库,可以通过以下命令安装:
pip install pymysql pillow
然后,使用 pymysql
库连接到 MySQL 数据库:
import pymysql
连接到数据库
connection = pymysql.connect(
host='localhost',
user='yourusername',
password='yourpassword',
database='yourdatabase'
)
二、获取图像数据
假设我们有一个名为 images
的表,其中存储了图像数据:
CREATE TABLE images (
id INT AUTO_INCREMENT PRIMARY KEY,
image LONGBLOB
);
我们可以编写一个函数来获取图像数据:
def get_image_data(image_id):
with connection.cursor() as cursor:
sql = "SELECT image FROM images WHERE id = %s"
cursor.execute(sql, (image_id,))
result = cursor.fetchone()
return result[0] if result else None
三、将图像数据转换为可显示的格式
使用 PIL
将二进制图像数据转换为可显示的格式:
from PIL import Image
import io
def convert_image_data(image_data):
image = Image.open(io.BytesIO(image_data))
return image
四、显示图像
使用 PIL
显示图像:
def display_image(image):
image.show()
示例:获取并显示图像
image_id = 1
image_data = get_image_data(image_id)
if image_data:
image = convert_image_data(image_data)
display_image(image)
else:
print("No image found with the given ID.")
五、使用 OpenCV 显示图像
如果你更喜欢使用 opencv
,可以通过以下方式显示图像:
pip install opencv-python
然后,使用 opencv
显示图像:
import cv2
import numpy as np
def display_image_with_opencv(image_data):
# 将二进制数据转换为 NumPy 数组
nparr = np.frombuffer(image_data, np.uint8)
# 使用 OpenCV 解码图像
image = cv2.imdecode(nparr, cv2.IMREAD_COLOR)
# 显示图像
cv2.imshow('Image', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
示例:获取并显示图像
image_id = 1
image_data = get_image_data(image_id)
if image_data:
display_image_with_opencv(image_data)
else:
print("No image found with the given ID.")
六、在网页上显示图像
如果你需要在网页上显示图像,可以使用 Flask 框架将图像数据发送到前端页面。首先,安装 Flask:
pip install flask
然后,编写一个简单的 Flask 应用:
from flask import Flask, send_file
import io
app = Flask(__name__)
@app.route('/image/<int:image_id>')
def get_image(image_id):
image_data = get_image_data(image_id)
if image_data:
return send_file(io.BytesIO(image_data), mimetype='image/jpeg')
else:
return "No image found with the given ID.", 404
if __name__ == '__main__':
app.run(debug=True)
在浏览器中访问 http://127.0.0.1:5000/image/1
即可查看图像。
七、总结
通过以上步骤,我们能够在 Python 中从数据库中提取图像数据并进行显示。无论是使用 PIL
、opencv
还是在网页上显示图像,这些方法都能帮助你处理和展示数据库中的图像数据。希望这些内容对你有所帮助!
相关问答FAQs:
如何在Python中连接到数据库并读取image类型的数据?
在Python中,可以使用库如sqlite3
、MySQLdb
或SQLAlchemy
来连接不同类型的数据库。通过执行查询并使用适当的光标方法,可以读取存储为image类型的数据。通常,您需要将二进制数据转换为适合显示的格式,例如使用PIL库将其转换为图像对象。
在Python中处理和显示数据库中的图像需要哪些库?
为了处理和显示存储在数据库中的图像,您可以使用Pillow
库来处理图像数据,以及matplotlib
或Tkinter
等库来显示图像。Pillow
允许您打开、编辑和保存图像,而matplotlib
和Tkinter
提供了简单的图形界面来展示这些图像。
如何将图像从Python数据库中提取并保存为文件?
提取图像数据的过程通常包括从数据库中读取二进制数据,并将其写入文件。可以使用Python的文件操作功能,将读取到的二进制数据写入到指定路径的文件中。确保在写入时使用适当的模式(如wb
)来避免数据损坏。
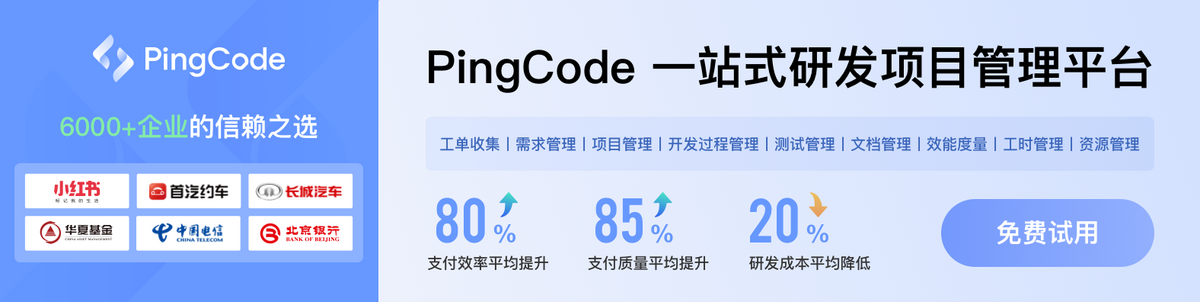