如何给文本文件加密python
在Python中给文本文件加密有多种方法,常见的包括使用对称加密(例如AES、DES)、非对称加密(例如RSA)以及简单的替换加密方法。使用AES加密、使用RSA加密、使用Fernet加密、简单替换加密是常见的文本文件加密方法。下面将详细介绍如何使用AES加密进行文本文件加密。
AES(高级加密标准)是一种对称加密算法,广泛应用于数据加密领域。AES使用相同的密钥进行加密和解密,因此需要确保密钥的安全性。使用Python的pycryptodome
库可以方便地实现AES加密。
一、安装所需库
首先,需要安装pycryptodome
库。可以通过以下命令安装:
pip install pycryptodome
二、AES加密和解密
1、生成密钥和初始化向量
AES加密需要一个密钥和一个初始化向量(IV)。密钥和IV的长度需要符合AES的要求。常见的密钥长度有128位、192位和256位,IV长度为128位。
from Crypto.Cipher import AES
from Crypto.Random import get_random_bytes
生成密钥和IV
key = get_random_bytes(32) # 256位密钥
iv = get_random_bytes(16) # 128位IV
2、加密文本文件
使用生成的密钥和IV,对文本文件进行加密。
from Crypto.Util.Padding import pad
def encrypt_file(file_path, key, iv):
with open(file_path, 'rb') as file:
plaintext = file.read()
# 创建AES加密器
cipher = AES.new(key, AES.MODE_CBC, iv)
# 对数据进行填充
padded_plaintext = pad(plaintext, AES.block_size)
# 加密数据
ciphertext = cipher.encrypt(padded_plaintext)
# 将密文写入文件
with open(file_path + '.enc', 'wb') as file:
file.write(iv + ciphertext)
3、解密文本文件
使用相同的密钥和IV,对加密后的文本文件进行解密。
from Crypto.Util.Padding import unpad
def decrypt_file(file_path, key):
with open(file_path, 'rb') as file:
iv = file.read(16) # 读取IV
ciphertext = file.read()
# 创建AES解密器
cipher = AES.new(key, AES.MODE_CBC, iv)
# 解密数据
plaintext = unpad(cipher.decrypt(ciphertext), AES.block_size)
# 将明文写入文件
with open(file_path.replace('.enc', ''), 'wb') as file:
file.write(plaintext)
三、示例代码
将上述代码整合在一起,形成一个完整的示例代码。
from Crypto.Cipher import AES
from Crypto.Util.Padding import pad, unpad
from Crypto.Random import get_random_bytes
def generate_key_iv():
key = get_random_bytes(32) # 256位密钥
iv = get_random_bytes(16) # 128位IV
return key, iv
def encrypt_file(file_path, key, iv):
with open(file_path, 'rb') as file:
plaintext = file.read()
cipher = AES.new(key, AES.MODE_CBC, iv)
padded_plaintext = pad(plaintext, AES.block_size)
ciphertext = cipher.encrypt(padded_plaintext)
with open(file_path + '.enc', 'wb') as file:
file.write(iv + ciphertext)
def decrypt_file(file_path, key):
with open(file_path, 'rb') as file:
iv = file.read(16)
ciphertext = file.read()
cipher = AES.new(key, AES.MODE_CBC, iv)
plaintext = unpad(cipher.decrypt(ciphertext), AES.block_size)
with open(file_path.replace('.enc', ''), 'wb') as file:
file.write(plaintext)
if __name__ == "__main__":
key, iv = generate_key_iv()
file_path = 'example.txt'
# 加密文件
encrypt_file(file_path, key, iv)
print(f"File '{file_path}' encrypted successfully.")
# 解密文件
encrypted_file_path = file_path + '.enc'
decrypt_file(encrypted_file_path, key)
print(f"File '{encrypted_file_path}' decrypted successfully.")
四、使用RSA加密
RSA是一种非对称加密算法,使用公钥加密和私钥解密。适用于加密小数据量,例如加密AES的密钥。
1、生成RSA密钥对
使用Python的Crypto.PublicKey
模块生成RSA密钥对。
from Crypto.PublicKey import RSA
def generate_rsa_key_pair():
key = RSA.generate(2048)
private_key = key.export_key()
public_key = key.publickey().export_key()
return private_key, public_key
2、加密和解密AES密钥
使用RSA公钥加密AES密钥,使用RSA私钥解密AES密钥。
from Crypto.Cipher import PKCS1_OAEP
def encrypt_aes_key(aes_key, public_key):
rsa_cipher = PKCS1_OAEP.new(RSA.import_key(public_key))
encrypted_key = rsa_cipher.encrypt(aes_key)
return encrypted_key
def decrypt_aes_key(encrypted_key, private_key):
rsa_cipher = PKCS1_OAEP.new(RSA.import_key(private_key))
aes_key = rsa_cipher.decrypt(encrypted_key)
return aes_key
五、使用Fernet加密
Fernet是一种对称加密方法,使用cryptography
库提供的Fernet类进行加密和解密。
1、安装cryptography库
pip install cryptography
2、使用Fernet加密和解密
from cryptography.fernet import Fernet
def generate_fernet_key():
key = Fernet.generate_key()
return key
def encrypt_file_with_fernet(file_path, key):
fernet = Fernet(key)
with open(file_path, 'rb') as file:
plaintext = file.read()
ciphertext = fernet.encrypt(plaintext)
with open(file_path + '.enc', 'wb') as file:
file.write(ciphertext)
def decrypt_file_with_fernet(file_path, key):
fernet = Fernet(key)
with open(file_path, 'rb') as file:
ciphertext = file.read()
plaintext = fernet.decrypt(ciphertext)
with open(file_path.replace('.enc', ''), 'wb') as file:
file.write(plaintext)
if __name__ == "__main__":
key = generate_fernet_key()
file_path = 'example.txt'
# 加密文件
encrypt_file_with_fernet(file_path, key)
print(f"File '{file_path}' encrypted successfully.")
# 解密文件
encrypted_file_path = file_path + '.enc'
decrypt_file_with_fernet(encrypted_file_path, key)
print(f"File '{encrypted_file_path}' decrypted successfully.")
六、简单替换加密
简单替换加密是一种基本的加密方法,使用一个字母表的替换表将每个字符替换为另一个字符。
1、生成替换表
import string
import random
def generate_substitution_table():
original_alphabet = string.ascii_letters + string.digits
shuffled_alphabet = list(original_alphabet)
random.shuffle(shuffled_alphabet)
substitution_table = dict(zip(original_alphabet, shuffled_alphabet))
return substitution_table
2、加密和解密文本文件
使用生成的替换表进行加密和解密。
def encrypt_file_with_substitution(file_path, substitution_table):
with open(file_path, 'r') as file:
plaintext = file.read()
ciphertext = ''.join([substitution_table.get(char, char) for char in plaintext])
with open(file_path + '.enc', 'w') as file:
file.write(ciphertext)
def decrypt_file_with_substitution(file_path, substitution_table):
reverse_substitution_table = {v: k for k, v in substitution_table.items()}
with open(file_path, 'r') as file:
ciphertext = file.read()
plaintext = ''.join([reverse_substitution_table.get(char, char) for char in ciphertext])
with open(file_path.replace('.enc', ''), 'w') as file:
file.write(plaintext)
if __name__ == "__main__":
substitution_table = generate_substitution_table()
file_path = 'example.txt'
# 加密文件
encrypt_file_with_substitution(file_path, substitution_table)
print(f"File '{file_path}' encrypted successfully.")
# 解密文件
encrypted_file_path = file_path + '.enc'
decrypt_file_with_substitution(encrypted_file_path, substitution_table)
print(f"File '{encrypted_file_path}' decrypted successfully.")
通过以上方法,可以在Python中实现多种文本文件加密,确保数据的安全性。根据具体需求选择合适的加密方法,并确保密钥的安全存储和管理。
相关问答FAQs:
如何使用Python保护我的文本文件内容?
使用Python加密文本文件的一个常见方法是使用对称加密库,如cryptography
。您可以安装这个库,并利用它的功能生成密钥、加密和解密文件内容。通过这种方式,您可以确保只有拥有正确密钥的人才能访问文件。
我需要哪些Python库来进行文本文件加密?
为了加密文本文件,推荐使用cryptography
库,它提供了简单易用的API,能够实现强大的加密功能。安装该库非常简单,只需运行pip install cryptography
命令即可。此外,您可能还需要os
和base64
等内置库来处理文件路径和编码。
加密后的文本文件如何进行解密?
解密加密的文本文件与加密过程相似,您需要使用相同的加密算法和密钥。使用cryptography
库时,可以通过提供密钥和加密的内容来恢复原始文本。确保在解密时,密钥与加密时使用的密钥一致,以确保能够成功恢复文件内容。
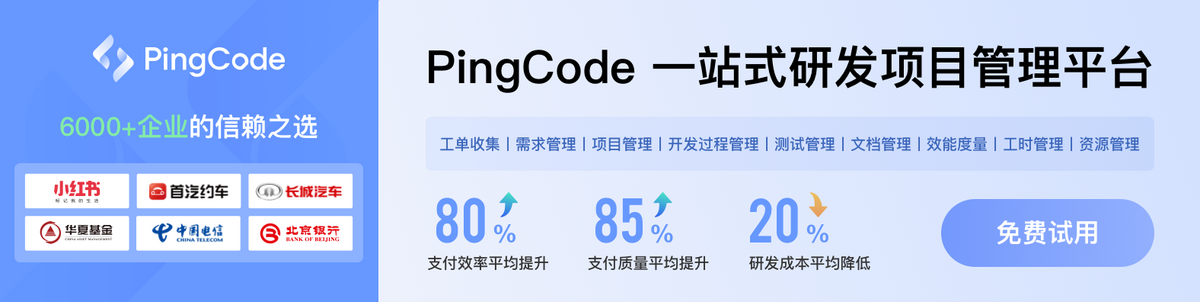