Python 输出字符的第几位
在 Python 中,我们可以通过多种方式来输出字符串中的特定字符的位置。使用索引、切片、内置函数是最常见的方法。下面将详细介绍这几种方法的使用。
一、使用索引
在 Python 中,字符串可以看作是字符的序列,每个字符都有一个对应的索引。索引从 0 开始递增,负数索引则从字符串末尾开始递减。例如,字符串 "hello" 的索引如下:
- h -> 0
- e -> 1
- l -> 2
- l -> 3
- o -> 4
通过索引,我们可以轻松获取字符串中特定位置的字符。使用索引的方法简单且高效。
string = "hello"
index = 2
character = string[index]
print(f"The character at index {index} is {character}")
二、使用切片
切片是另一种从字符串中获取字符的方法。切片允许我们指定一个范围来获取子字符串。切片的语法是 string[start:stop:step]
,其中 start
是开始索引,stop
是结束索引,step
是步长。
切片在获取多个字符时非常有用,但对于获取单个字符,索引更加简洁。
string = "hello"
character = string[2:3]
print(f"The character at index 2 is {character}")
三、内置函数
Python 提供了一些内置函数来处理字符串。虽然没有直接获取特定索引字符的函数,但我们可以利用 enumerate
来遍历字符串并获取字符的索引。
string = "hello"
target_index = 2
for index, character in enumerate(string):
if index == target_index:
print(f"The character at index {index} is {character}")
break
四、实战案例:查找字符位置
了解了基本方法后,我们可以进一步探讨如何在实际应用中使用这些技术。例如,我们可能需要查找某个字符首次出现的位置。
string = "hello world"
target_char = 'o'
position = string.find(target_char)
print(f"The character '{target_char}' first appears at index {position}")
五、更多高级用法
我们还可以结合多种方法,实现更加复杂的字符查找功能。例如,查找所有出现某个字符的索引。
string = "hello world"
target_char = 'l'
indices = [i for i, char in enumerate(string) if char == target_char]
print(f"The character '{target_char}' appears at indices {indices}")
六、错误处理
在编写程序时,我们还需要考虑错误处理。例如,当索引超出范围时,需要捕获并处理异常。
string = "hello"
try:
index = 10
character = string[index]
print(f"The character at index {index} is {character}")
except IndexError:
print(f"Index {index} is out of range for string '{string}'")
通过本文的介绍,我们详细探讨了在 Python 中如何输出字符的第几位,并通过多个示例演示了不同方法的使用。希望这些内容能对您有所帮助,助您更好地掌握 Python 的字符串处理技巧。
相关问答FAQs:
如何在Python中访问字符串的特定字符?
在Python中,可以使用索引来访问字符串中的特定字符。字符串的索引从0开始,因此要获取第n个字符,可以使用字符串名后面跟着[n-1]的语法。例如,如果你有一个字符串s = "Hello"
,要获取第二个字符,可以使用s[1]
,这将返回'e'。
Python中如何处理字符串长度以避免索引错误?
在访问字符串的字符时,确保索引不超出字符串的长度是非常重要的。可以使用len()
函数来获取字符串的长度。例如,使用len(s)
可以得知字符串s
的长度。如果需要安全地访问字符,可以在访问之前检查索引是否在有效范围内,避免出现IndexError
。
有没有方法可以在Python中循环输出字符串中的每个字符及其索引?
可以使用enumerate()
函数结合循环来遍历字符串中的每个字符及其对应的索引。示例代码如下:
s = "Hello"
for index, char in enumerate(s):
print(f"Index {index}: Character '{char}'")
这段代码会输出每个字符及其在字符串中的位置,方便进行调试和字符分析。
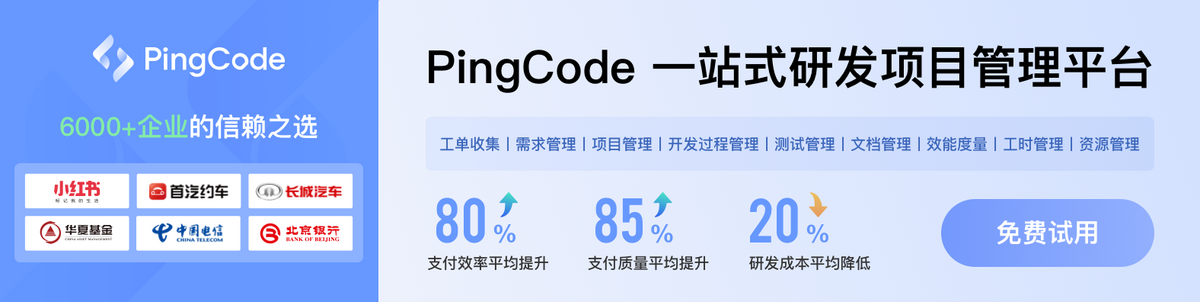