在Python中减少字符串长度的方法有:裁剪字符串、使用字符串切片、正则表达式替换、压缩字符串、移除不必要的空白字符等。 其中,使用字符串切片是最常见和简便的方法。通过切片,我们可以轻松获取字符串的子字符串,从而减少字符串的长度。
使用字符串切片的方法如下:
string = "Hello, World!"
shortened_string = string[:5] # 获取前5个字符
print(shortened_string) # 输出: Hello
字符串切片可以通过指定起始和结束位置,获取子字符串。Python支持负索引,可以方便地从字符串末尾开始计数,灵活性非常高。
接下来,我们将详细介绍在Python中减少字符串长度的各种方法。
一、裁剪字符串
裁剪字符串是指通过删除字符串的某些部分来减少字符串的长度。常用的方法包括删除前缀、后缀以及中间部分的内容。
1、删除前缀
删除前缀可以使用字符串的lstrip()
方法,它会删除字符串开头的指定字符,默认删除空白字符。
string = " Hello, World!"
shortened_string = string.lstrip()
print(shortened_string) # 输出: "Hello, World!"
2、删除后缀
删除后缀可以使用字符串的rstrip()
方法,它会删除字符串结尾的指定字符,默认删除空白字符。
string = "Hello, World! "
shortened_string = string.rstrip()
print(shortened_string) # 输出: "Hello, World!"
3、删除前后空白字符
删除前后空白字符可以使用字符串的strip()
方法,它会删除字符串两端的空白字符。
string = " Hello, World! "
shortened_string = string.strip()
print(shortened_string) # 输出: "Hello, World!"
二、使用字符串切片
字符串切片是通过索引指定字符串的起始和结束位置,获取子字符串的方法。字符串切片非常灵活,可以根据需要获取字符串的任意部分。
1、获取前n个字符
通过切片获取字符串的前n个字符。
string = "Hello, World!"
shortened_string = string[:5]
print(shortened_string) # 输出: "Hello"
2、获取后n个字符
通过切片获取字符串的后n个字符。
string = "Hello, World!"
shortened_string = string[-5:]
print(shortened_string) # 输出: "orld!"
3、获取中间部分字符
通过切片获取字符串的中间部分字符。
string = "Hello, World!"
shortened_string = string[7:12]
print(shortened_string) # 输出: "World"
三、正则表达式替换
正则表达式可以用来匹配和替换字符串中的模式,从而减少字符串的长度。Python中的re
模块提供了强大的正则表达式功能。
1、删除所有空白字符
使用正则表达式删除字符串中的所有空白字符。
import re
string = "Hello, World!"
shortened_string = re.sub(r'\s+', '', string)
print(shortened_string) # 输出: "Hello,World!"
2、删除特定模式
使用正则表达式删除字符串中的特定模式。
import re
string = "Hello, 123 World!"
shortened_string = re.sub(r'\d+', '', string)
print(shortened_string) # 输出: "Hello, World!"
四、压缩字符串
字符串压缩可以使用一些算法来减少字符串的长度,例如使用Huffman编码或RLE(运行长度编码)。
1、Huffman编码
Huffman编码是一种常见的无损压缩算法,可以用来压缩字符串。以下是一个简单的Huffman编码示例:
from collections import Counter, Heapq
class Node:
def __init__(self, freq, char=None):
self.freq = freq
self.char = char
self.left = None
self.right = None
def __lt__(self, other):
return self.freq < other.freq
def build_huffman_tree(s):
freq = Counter(s)
heap = [Node(freq[char], char) for char in freq]
heapq.heapify(heap)
while len(heap) > 1:
left = heapq.heappop(heap)
right = heapq.heappop(heap)
parent = Node(left.freq + right.freq)
parent.left = left
parent.right = right
heapq.heappush(heap, parent)
return heap[0]
def build_huffman_codes(node, code='', codes={}):
if node.char:
codes[node.char] = code
else:
build_huffman_codes(node.left, code + '0', codes)
build_huffman_codes(node.right, code + '1', codes)
return codes
def huffman_encode(s):
root = build_huffman_tree(s)
codes = build_huffman_codes(root)
return ''.join(codes[char] for char in s), codes
string = "Hello, World!"
encoded_string, huffman_codes = huffman_encode(string)
print(encoded_string) # 输出: 压缩后的二进制字符串
2、RLE(运行长度编码)
RLE是一种简单的无损压缩算法,通过记录字符及其重复次数来压缩字符串。
def rle_encode(s):
encoding = ''
i = 0
while i < len(s):
count = 1
while i + 1 < len(s) and s[i] == s[i + 1]:
i += 1
count += 1
encoding += s[i] + str(count)
i += 1
return encoding
string = "aaabbbcc"
encoded_string = rle_encode(string)
print(encoded_string) # 输出: "a3b3c2"
五、移除不必要的空白字符
在实际应用中,字符串中可能包含很多不必要的空白字符,通过移除这些空白字符可以有效减少字符串的长度。
1、移除多余空格
使用字符串的split()
和join()
方法,可以移除字符串中的多余空格。
string = "Hello, World! How are you?"
shortened_string = ' '.join(string.split())
print(shortened_string) # 输出: "Hello, World! How are you?"
2、移除换行符
使用字符串的replace()
方法,可以移除字符串中的换行符。
string = "Hello,\nWorld!\nHow\nare\nyou?"
shortened_string = string.replace('\n', ' ')
print(shortened_string) # 输出: "Hello, World! How are you?"
通过以上几种方法,我们可以在Python中有效地减少字符串的长度。无论是通过裁剪、切片、正则表达式替换,还是通过压缩和移除不必要的空白字符,都可以根据具体需求选择合适的方法来实现。
相关问答FAQs:
如何在Python中截断字符串以减少其长度?
在Python中,可以使用切片操作来截断字符串。通过指定开始和结束索引,可以方便地获取字符串的子串。例如,如果想将字符串“Hello, World!”截断为前5个字符,可以使用以下代码:short_string = original_string[:5]
,这将得到“Hello”。
有哪些方法可以将字符串压缩为更短的形式?
除了直接截断,Python还提供了多种方法来压缩字符串。例如,可以使用str.replace()
方法来替换字符串中的某些部分,或者通过正则表达式re.sub()
来删除不必要的字符。此外,使用zlib
库可以对字符串进行压缩,以减少其占用的空间。
如何在Python中处理长度超过限制的字符串?
当处理长度超过特定限制的字符串时,可以使用条件判断来确保字符串符合要求。可以通过if len(string) > limit:
来检查字符串长度,并在超过限制时进行截断或替换。例如,可以结合上述的切片和替换方法,确保字符串在输出时不会超出预定的长度限制。
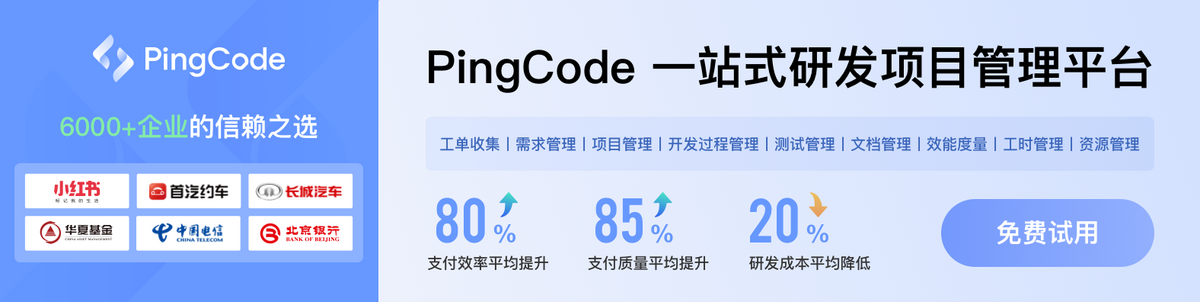