在 Python 中实现多线程进度条并行的几种方法有:使用 threading
模块管理线程、使用 tqdm
库实现进度条、以及使用 concurrent.futures
模块来简化线程管理。下面将详细解释如何使用这些方法来实现多线程进度条的并行。
使用多线程可以提高程序的效率,特别是在 I/O 密集型任务中。然而,在多线程中实现进度条并行需要一些技巧。接下来,我们将详细介绍如何使用 threading
模块和 tqdm
库来实现这一目标,并提供完整的代码示例。
一、使用 threading
模块管理线程
threading
是 Python 标准库中的一个模块,提供了创建和管理线程的基本功能。我们可以使用这个模块来创建和管理多个线程,并通过共享变量或队列来跟踪进度。
1. 创建线程
首先,我们需要创建多个线程来执行不同的任务。可以使用 threading.Thread
类来创建线程。以下是一个简单的示例:
import threading
import time
def worker(thread_id, progress):
for i in range(10):
time.sleep(0.1) # 模拟工作
progress[thread_id] = i + 1
threads = []
progress = [0] * 5 # 进度列表
for i in range(5):
t = threading.Thread(target=worker, args=(i, progress))
threads.append(t)
t.start()
for t in threads:
t.join()
print('All threads completed')
2. 跟踪进度
为了跟踪每个线程的进度,可以使用一个共享的列表或字典。每个线程在完成一定量的工作后,更新相应的进度值。
import threading
import time
def worker(thread_id, progress):
for i in range(10):
time.sleep(0.1) # 模拟工作
progress[thread_id] = i + 1
print(f'Thread {thread_id} progress: {progress[thread_id]}/10')
threads = []
progress = [0] * 5 # 进度列表
for i in range(5):
t = threading.Thread(target=worker, args=(i, progress))
threads.append(t)
t.start()
for t in threads:
t.join()
print('All threads completed')
二、使用 tqdm
库实现进度条
tqdm
是一个用于显示进度条的 Python 库。它可以与 threading
模块结合使用,以实现多线程进度条的并行。
1. 安装 tqdm
首先,需要安装 tqdm
库。可以使用 pip
来安装:
pip install tqdm
2. 使用 tqdm
显示进度条
可以将 tqdm
与 threading
模块结合使用,以显示多线程任务的进度条。以下是一个示例:
import threading
import time
from tqdm import tqdm
def worker(thread_id, progress, pbar):
for i in range(10):
time.sleep(0.1) # 模拟工作
progress[thread_id] = i + 1
pbar.update(1)
threads = []
progress = [0] * 5 # 进度列表
total_iterations = 50 # 总迭代次数(5个线程,每个10次)
pbar = tqdm(total=total_iterations)
for i in range(5):
t = threading.Thread(target=worker, args=(i, progress, pbar))
threads.append(t)
t.start()
for t in threads:
t.join()
pbar.close()
print('All threads completed')
三、使用 concurrent.futures
模块
concurrent.futures
是 Python 3.2 引入的一个标准库模块,提供了高级的异步并行执行功能。可以使用 ThreadPoolExecutor
来管理线程池,并使用 tqdm
显示进度条。
1. 使用 ThreadPoolExecutor
ThreadPoolExecutor
提供了一个简化的接口来管理线程池。可以使用 submit
方法来提交任务,并使用 as_completed
方法来跟踪任务的完成情况。
from concurrent.futures import ThreadPoolExecutor, as_completed
from tqdm import tqdm
import time
def worker(thread_id):
for i in range(10):
time.sleep(0.1) # 模拟工作
return thread_id
total_iterations = 50 # 总迭代次数(5个线程,每个10次)
pbar = tqdm(total=total_iterations)
with ThreadPoolExecutor(max_workers=5) as executor:
futures = [executor.submit(worker, i) for i in range(5)]
for future in as_completed(futures):
thread_id = future.result()
pbar.update(10)
pbar.close()
print('All threads completed')
2. 跟踪进度
在上面的示例中,使用 as_completed
方法来跟踪每个线程的完成情况,并在每个线程完成后更新进度条。
四、实践案例
为了更好地理解如何在实际应用中使用多线程进度条并行,我们将举一个实际案例。假设我们需要下载多个文件,并希望显示每个文件的下载进度。
import threading
import time
import requests
from tqdm import tqdm
def download_file(url, thread_id, progress, pbar):
response = requests.get(url, stream=True)
total_size = int(response.headers.get('content-length', 0))
chunk_size = 1024
with open(f'file_{thread_id}.bin', 'wb') as file:
for data in response.iter_content(chunk_size):
file.write(data)
progress[thread_id] += len(data)
pbar.update(len(data))
pbar.write(f'Thread {thread_id} completed')
urls = [
'https://example.com/file1.bin',
'https://example.com/file2.bin',
'https://example.com/file3.bin',
'https://example.com/file4.bin',
'https://example.com/file5.bin'
]
threads = []
progress = [0] * len(urls) # 进度列表
total_size = sum(int(requests.head(url).headers.get('content-length', 0)) for url in urls)
pbar = tqdm(total=total_size)
for i, url in enumerate(urls):
t = threading.Thread(target=download_file, args=(url, i, progress, pbar))
threads.append(t)
t.start()
for t in threads:
t.join()
pbar.close()
print('All downloads completed')
在这个示例中,我们使用 requests
库来下载文件,并使用 tqdm
显示进度条。每个线程负责下载一个文件,并在下载过程中更新进度条。
总结
在 Python 中实现多线程进度条并行的方法包括使用 threading
模块管理线程、使用 tqdm
库实现进度条、以及使用 concurrent.futures
模块简化线程管理。通过结合这些工具,可以高效地管理多线程任务,并实时显示进度。希望本文能够帮助你更好地理解和应用多线程进度条并行的技术。
相关问答FAQs:
在Python中,如何实现多线程进度条的可视化?
实现多线程进度条的可视化可以通过使用threading
模块结合tqdm
库来完成。tqdm
允许我们轻松创建进度条,并且可以与多线程一起使用。您可以在每个线程中更新进度条,并在主线程中显示进度。这种方式可以有效地反馈任务的执行情况。
使用多线程时,如何保证进度条的线程安全?
为了保证进度条的线程安全,可以使用threading.Lock
来确保在更新进度条时不会发生数据竞争。在每个线程执行任务时,获取锁并更新进度条,完成后释放锁。这种方法可以防止多个线程同时更新进度条,从而导致显示混乱。
在多线程环境中,如何处理进度条的更新逻辑?
在多线程环境中,可以通过设定一个共享变量来记录完成的任务数量。每个线程完成一个任务后,更新这个共享变量,然后调用进度条更新的方法。可以使用Queue
来实现任务的分配和进度的更新,确保所有线程都能有效地反馈其状态。
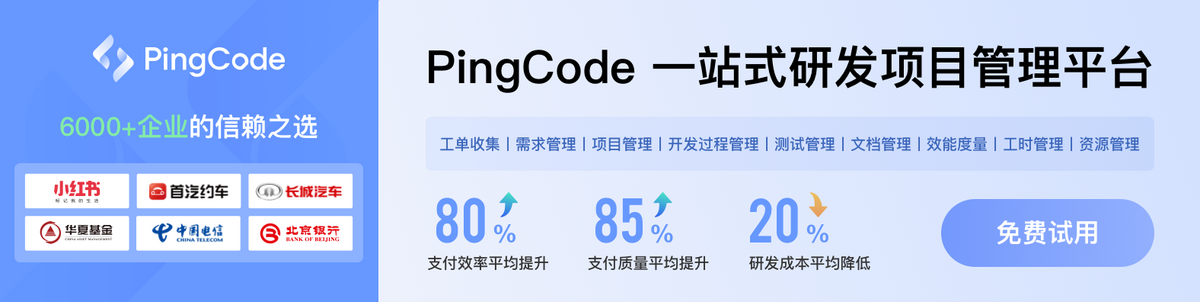