Python做空间插值分析的方法有:SciPy库、NumPy库、PyKrige库、GDAL库和Pyresample库。其中,SciPy库的插值方法较为全面,适合大多数应用场景。接下来,我们详细介绍如何使用SciPy库进行空间插值分析。
一、SciPy库
SciPy库是一个广泛使用的Python科学计算库,提供了多种插值方法,如线性插值、样条插值、径向基函数插值等。以下是使用SciPy库进行空间插值的一些步骤:
1、安装SciPy库
首先确保安装了SciPy库,可以使用以下命令安装:
pip install scipy
2、读取和准备数据
我们需要准备插值分析的数据,通常包括已知数据点的坐标和对应的数值。可以使用NumPy库来读取和准备数据:
import numpy as np
示例数据
points = np.array([[0, 0], [1, 1], [2, 2], [3, 3]])
values = np.array([0, 1, 4, 9])
3、选择插值方法
SciPy库提供了多种插值方法,可以根据实际需求选择合适的方法:
from scipy.interpolate import griddata
定义插值点
grid_x, grid_y = np.mgrid[0:3:100j, 0:3:100j]
线性插值
grid_z0 = griddata(points, values, (grid_x, grid_y), method='linear')
最近邻插值
grid_z1 = griddata(points, values, (grid_x, grid_y), method='nearest')
样条插值
grid_z2 = griddata(points, values, (grid_x, grid_y), method='cubic')
4、可视化结果
可以使用Matplotlib库将插值结果可视化:
import matplotlib.pyplot as plt
plt.subplot(131)
plt.imshow(grid_z0.T, extent=(0, 3, 0, 3), origin='lower')
plt.title('Linear')
plt.subplot(132)
plt.imshow(grid_z1.T, extent=(0, 3, 0, 3), origin='lower')
plt.title('Nearest')
plt.subplot(133)
plt.imshow(grid_z2.T, extent=(0, 3, 0, 3), origin='lower')
plt.title('Cubic')
plt.show()
二、NumPy库
NumPy库是Python中一个强大的数值计算库,可以用来处理多维数组和矩阵。虽然NumPy本身并不提供插值功能,但它可以与其他库结合使用,进行高效的数据处理和插值计算。
1、安装NumPy库
确保安装了NumPy库,可以使用以下命令安装:
pip install numpy
2、读取和准备数据
我们需要准备插值分析的数据,通常包括已知数据点的坐标和对应的数值:
import numpy as np
示例数据
points = np.array([[0, 0], [1, 1], [2, 2], [3, 3]])
values = np.array([0, 1, 4, 9])
3、选择插值方法
NumPy库可以与SciPy库结合使用,进行插值计算:
from scipy.interpolate import griddata
定义插值点
grid_x, grid_y = np.mgrid[0:3:100j, 0:3:100j]
线性插值
grid_z0 = griddata(points, values, (grid_x, grid_y), method='linear')
三、PyKrige库
PyKrige库是一个专门用于克里金插值的Python库,适合空间统计分析。克里金插值是一种基于地质统计学的插值方法,广泛应用于地球科学领域。
1、安装PyKrige库
确保安装了PyKrige库,可以使用以下命令安装:
pip install pykrige
2、读取和准备数据
准备插值分析的数据,通常包括已知数据点的坐标和对应的数值:
import numpy as np
示例数据
points = np.array([[0, 0], [1, 1], [2, 2], [3, 3]])
values = np.array([0, 1, 4, 9])
3、选择插值方法
使用PyKrige库进行克里金插值:
from pykrige.ok import OrdinaryKriging
定义插值点
grid_x = np.linspace(0, 3, 100)
grid_y = np.linspace(0, 3, 100)
克里金插值
OK = OrdinaryKriging(points[:, 0], points[:, 1], values, variogram_model='linear')
z, ss = OK.execute('grid', grid_x, grid_y)
4、可视化结果
可以使用Matplotlib库将插值结果可视化:
import matplotlib.pyplot as plt
plt.imshow(z, extent=(0, 3, 0, 3), origin='lower')
plt.title('Kriging')
plt.show()
四、GDAL库
GDAL库是一个用于处理地理空间数据的开源库,提供了强大的数据处理功能。虽然GDAL库本身不提供插值功能,但它可以与其他库结合使用,进行高效的数据处理和插值计算。
1、安装GDAL库
确保安装了GDAL库,可以使用以下命令安装:
pip install GDAL
2、读取和准备数据
准备插值分析的数据,通常包括已知数据点的坐标和对应的数值:
import numpy as np
示例数据
points = np.array([[0, 0], [1, 1], [2, 2], [3, 3]])
values = np.array([0, 1, 4, 9])
3、选择插值方法
GDAL库可以与SciPy库结合使用,进行插值计算:
from scipy.interpolate import griddata
定义插值点
grid_x, grid_y = np.mgrid[0:3:100j, 0:3:100j]
线性插值
grid_z0 = griddata(points, values, (grid_x, grid_y), method='linear')
五、Pyresample库
Pyresample库是一个用于重采样和插值的Python库,适合处理地球科学数据。它提供了多种插值方法,如最近邻插值、双线性插值等。
1、安装Pyresample库
确保安装了Pyresample库,可以使用以下命令安装:
pip install pyresample
2、读取和准备数据
准备插值分析的数据,通常包括已知数据点的坐标和对应的数值:
import numpy as np
示例数据
points = np.array([[0, 0], [1, 1], [2, 2], [3, 3]])
values = np.array([0, 1, 4, 9])
3、选择插值方法
使用Pyresample库进行插值计算:
from pyresample import geometry, kd_tree
定义插值点
grid_x, grid_y = np.mgrid[0:3:100j, 0:3:100j]
创建源和目标网格
source_grid = geometry.GridDefinition(lons=points[:, 0], lats=points[:, 1])
target_grid = geometry.GridDefinition(lons=grid_x, lats=grid_y)
最近邻插值
result = kd_tree.resample_nearest(source_grid, values, target_grid, radius_of_influence=100000)
4、可视化结果
可以使用Matplotlib库将插值结果可视化:
import matplotlib.pyplot as plt
plt.imshow(result, extent=(0, 3, 0, 3), origin='lower')
plt.title('Nearest Neighbor')
plt.show()
总结
Python提供了多种方法进行空间插值分析,主要包括SciPy库、NumPy库、PyKrige库、GDAL库和Pyresample库。根据实际需求选择合适的方法,并结合数据准备和可视化工具,可以高效地完成空间插值分析。
相关问答FAQs:
空间插值分析在Python中需要哪些库和工具?
进行空间插值分析,Python用户通常会使用一些强大的库,如SciPy、NumPy、Pandas以及专门用于地理空间数据处理的Geopandas和Rasterio。此外,Matplotlib可以用于可视化插值结果,这些工具能够帮助用户轻松处理和分析空间数据。
空间插值分析的常用方法有哪些?
在Python中,常见的空间插值方法包括反距离加权(IDW)、克里金(Kriging)、样条插值(Spline Interpolation)等。每种方法都有其适用的场景和优缺点,选择合适的方法取决于数据的性质和分析目标。
如何在Python中实现空间插值的可视化?
可视化是分析结果的重要部分。在Python中,可以使用Matplotlib和Seaborn库进行插值结果的可视化。通过绘制插值后的热图、等高线图等,用户可以直观地理解空间分布特征,从而做出更有意义的解读和决策。
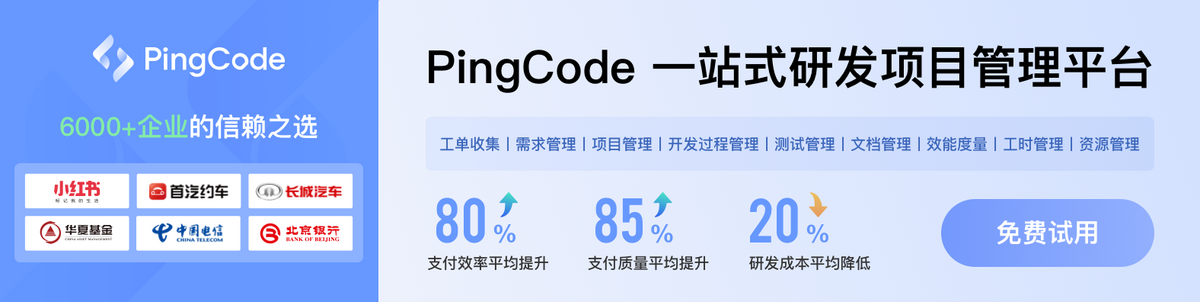